
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
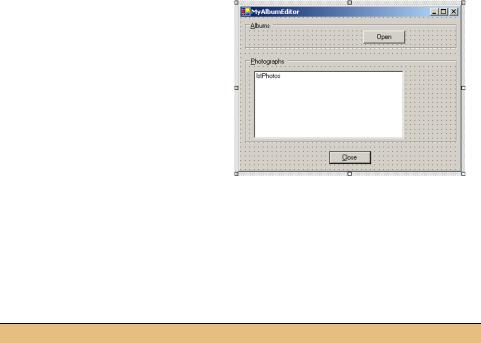
|
|
CREATE THE CONTROLS FOR OUR NEW APPLICATION |
(continued) |
|||
|
|
|
|
|
|
|
|
|
|
Action |
|
Result |
|
|
|
|
|
|
|
|
8 |
Set the properties for the MainForm |
|
|
|||
|
form. |
|
|
|
|
|
|
|
Settings |
|
|
||
|
|
|
|
|
|
|
|
|
Property |
Value |
|
|
|
|
|
AcceptButton |
btnClose |
|
|
|
|
|
Size |
400, 300 |
|
|
|
|
|
Text |
MyAlbumEditor |
|
|
|
|
|
Note: When you enter the new Size |
|
|
||
|
|
setting, note how the controls auto- |
|
|
||
|
|
matically resize within the form based |
|
|
||
|
|
on the assigned Anchor settings. |
|
|
||
|
|
|
|
|
|
|
Our form is now ready. You can compile and run if you like. Before we talk about this in any detail, we will add some code to make our new ListBox display the photographs in an album.
Some of the new code added by the following steps mimics code we provided for our MyPhotos application. This is to be expected, since both interfaces operate on photo album collections.
DISPLAY THE CONTENTS OF AN ALBUM IN THE LISTBOX CONTROL
|
Action |
Result |
|
|
|
9 |
In the MainForm.cs file, indicate we |
. . . |
|
are using the Manning.MyPhotoAlbum |
using Manning.MyPhotoAlbum; |
|
namespace. |
|
|
|
|
10 |
Add some member variables to track |
private PhotoAlbum _album; |
|
the current album and whether it has |
private bool _bAlbumChanged = false; |
|
changed. |
|
|
|
|
11 |
Override the OnLoad method to |
protected override void OnLoad |
|
initialize the album. |
(EventArgs e) |
|
Note: The OnLoad method is called a |
{ |
|
// Initialize the album |
|
|
single time after the form has been |
_album = new PhotoAlbum(); |
|
created and before the form is initially |
base.OnLoad(e); |
|
displayed. This method is a good place |
|
|
} |
|
|
to perform one-time initialization for |
|
|
a form. |
|
|
|
|
12 |
Add a Click handler for the Close |
private void btnClose_Click |
|
button to exit the application. |
(object sender, System.EventArgs e) |
|
|
{ |
|
|
Close(); |
|
|
} |
|
|
|
LIST BOXES |
319 |

DISPLAY THE CONTENTS OF AN ALBUM IN THE LISTBOX CONTROL (continued)
|
Action |
Result |
|
|
|
13 |
Add a CloseAlbum method to close a |
private void CloseAlbum() |
|
previously opened album. |
{ |
|
How-to |
if (_bAlbumChanged) |
|
{ |
|
|
Display a dialog to ask if the user |
_bAlbumChanged = false; |
|
wants to save any changes they |
DialogResult result |
|
have made. |
|
|
= MessageBox.Show("Do you want " |
|
|
|
+ "to save your changes to " |
|
|
+ _album.FileName + '?', |
|
|
"Save Changes?", |
|
|
MessageBoxButtons.YesNo, |
|
|
MessageBoxIcon.Question); |
|
|
if (result == DialogResult.Yes) |
|
|
{ |
|
|
_album.Save(); |
|
|
} |
|
|
} |
|
|
_album.Clear(); |
|
|
} |
|
|
|
14 |
Override the OnClosing method to |
protected override void OnClosing |
|
ensure the album is closed on exit. |
(CancelEventArgs e) |
|
|
{ |
|
|
CloseAlbum(); |
|
|
} |
|
|
|
15 |
Add a Click handler for the Open button |
private void btnOpen_Click |
|
to open an album and assign it to the |
(object sender, System.EventArgs e) |
|
ListBox. |
{ |
|
CloseAlbum(); |
|
|
|
|
|
How-to |
using (OpenFileDialog dlg |
|
a. Close any previously open album. |
|
|
= new OpenFileDialog()) |
|
|
b. Use the OpenFileDialog class to |
|
|
{ |
|
|
allow the user to select an album. |
dlg.Title = "Open Album"; |
|
c. Use the PhotoAlbum.Open method |
dlg.Filter = "abm files (*.abm)" |
|
+ "|*.abm|All Files (*.*)|*.*"; |
|
|
to open the file. |
dlg.InitialDirectory |
|
d. Assign the album’s file name to the |
= PhotoAlbum.DefaultDir; |
|
|
|
|
title bar of the form. |
try |
|
e. Use a separate method for updating |
{ |
|
the contents of the list box. |
if (dlg.ShowDialog() |
|
== DialogResult.OK) |
|
|
|
|
|
|
{ |
|
|
_album.Open(dlg.FileName); |
|
|
this.Text = _album.FileName; |
|
|
UpdateList(); |
|
|
} |
|
|
} |
|
|
catch (Exception) |
|
|
{ |
|
|
MessageBox.Show("Unable to open " |
|
|
+ "album\n" + dlg.FileName, |
|
|
"Open Album Error", |
|
|
MessageBoxButtons.OK, |
|
|
MessageBoxIcon.Error); |
|
|
} |
|
|
} |
|
|
} |
|
|
|
320 |
CHAPTER 10 LIST CONTROLS |
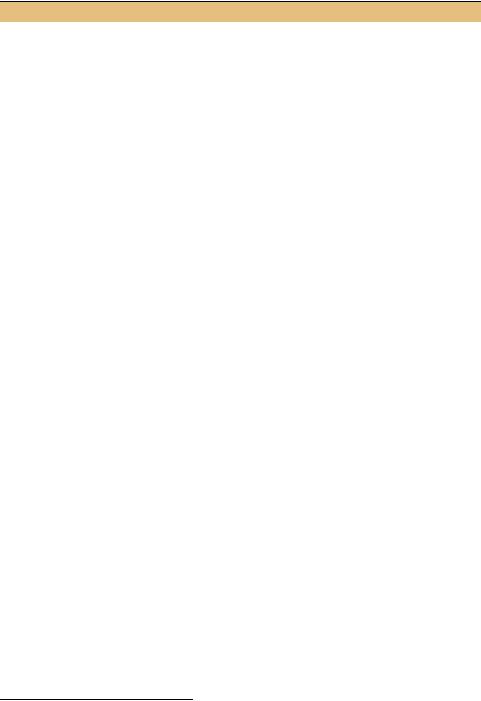
DISPLAY THE CONTENTS OF AN ALBUM IN THE LISTBOX CONTROL (continued)
|
Action |
Result |
|
|
|
16 |
Implement a protected UpdateList |
protected void UpdateList() |
|
method to initialize the ListBox |
{ |
|
control. |
lstPhotos.DataSource = _album; |
|
} |
|
|
|
|
|
|
|
That’s it! No need to add individual photographs one by one or perform other complicated steps to fill in the list box. Much of the code is similar to code we saw in previous chapters. The one exception, the UpdateList method, simply assigns the DataSource property of the ListBox control to the current photo album.
protected void UpdateList()
{
lstPhotos.DataSource = _album;
}
The DataSource property is part of the data binding support in Windows Forms. Data binding refers to the idea of assigning one or more values from some source of data to the settings for one or more controls. A data source is basically any array of objects, and in particular any class that supports the IList interface.1 Since the PhotoAlbum class is based on IList, each item in the list, in this case each Photograph, is displayed by the control. By default, the ToString property for each contained item is used as the display string. If you recall, we implemented this method for the Photograph class in chapter 5 to return the file name associated with the photo.
Compile and run your code to display your own album. An example of the output is shown in figure 10.2. In the figure, an album called colors.abm is displayed, with each photograph in the album named after a well-known color. Note how the GroupBox controls display their keyboard access keys, namely Alt+A and Alt+P. When activated, the focus is set to the first control in the group box, based on the assigned tab order.
1 We will discuss data binding more generally in chapter 17.
LIST BOXES |
321 |
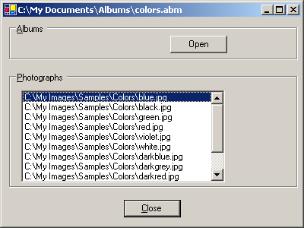
Figure 10.2
By default, the ListBox control displays a scroll bar when the number of items to display exceeds the size of
the box.
You will also note that there is a lot of blank space in our application. Not to worry. These spaces will fill up as we progress through the chapter.
TRY IT! The DisplayMember property for the ListBox class indicates the name of the property to use for display purposes. In our program, since this property is not set, the default ToString property inherited from the Object class is used. Modify this property in the UpdateList method to a property specific to the Photograph class, such as “FileName” or “Caption.” Run the program again to see how this affects the displayed photographs.
The related property ValueMember specifies the value returned by members such as the SelectedValue property. By default, this property will return the object instance itself.
10.1.2HANDLING SELECTED ITEMS
As you might expect, the ListBox class supports much more than the ability to display a collection of objects. Particulars of this class are summarized in .NET Table 10.2. In the MyAlbumEditor application, the list box is a single-selection, single-column list corresponding to the contents of the current album. There are a number of different features we will demonstrate in our application. For starters, let’s display the dialogs we
created in chapter 9.
The album dialog can be displayed using a normal button. For the
Dlg dialog, we would like to display the properties of the photograph that are currently selected in the list box. As you may recall, this dialog displays the photograph at the current position within the album, which seemed quite reasonable for our MyPhotos application. To make this work here, we will need to modify the current position to correspond to the selected item.
322 |
CHAPTER 10 LIST CONTROLS |

.NET Table 10.2 ListBox class
The ListBox class represents a list control that displays a collection as a scrollable window. A list box can support single or multiple selection of its items, and each item can display as a simple text string or a custom graphic. This class is part of the System.Windows.Forms namespace, and inherits from the ListControl class. See .NET Table 10.1 on page 316 for a list of members inherited by this class.
|
DefaultItemHeight |
The default item height for an owner-drawn ListBox |
Public |
|
object. |
|
|
|
Static Fields |
NoMatches |
The value returned by ListBox methods when no |
|
|
matches are found during a search. |
|
|
|
|
DrawMode |
Gets or sets how this list box should be drawn. |
|
ItemHeight |
Gets or sets the height of an item in the list box. |
|
Items |
Gets the collection of items to display. |
|
MultiColumn |
Gets or sets whether this list box should support |
|
|
multiple columns. Default is false. |
Public |
SelectedIndices |
Gets a collection of zero-based indices for the items |
|
selected in the list box. |
|
Properties |
SelectedItem |
Gets or sets the currently selected object. |
|
||
|
SelectedItems |
Gets a collection of all items selected in the list. |
|
SelectionMode |
Gets or sets how items are selected in the list box. |
|
Sorted |
Gets or sets whether the displayed list should be |
|
|
automatically sorted. |
|
TopIndex |
Gets the index of the first visible item in the list. |
|
|
|
|
BeginUpdate |
Prevents the control from painting its contents while |
|
|
items are added to the list box. |
|
ClearSelected |
Deselects all selected items in the control. |
Public |
FindString |
Returns the index of the first item with a display value |
|
beginning with a given string. |
|
Methods |
GetSelected |
Indicates whether a specified item is selected. |
|
||
|
IndexFromPoint |
Returns the index of the item located at the specified |
|
|
coordinates. |
|
SetSelected |
Selects or deselects a given item. |
|
|
|
|
DrawItem |
Occurs when an item in an owner-drawn list box requires |
|
|
painting. |
Public |
MeasureItem |
Occurs when the size of an item in an owner-drawn list |
Events |
|
box is required. |
|
SelectedIndex- |
Occurs whenever a new item is selected in the list box, |
|
Changed |
for both single and multiple selection boxes. |
|
|
|
LIST BOXES |
323 |