
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
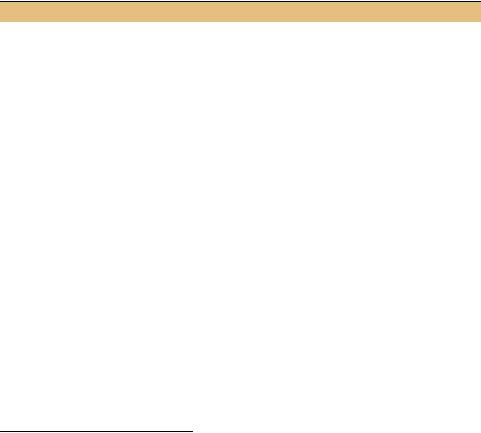
These changes implement the correct functionality for the button. When the button is pushed, a Click event for the menuPixelData menu is performed, which displays the dialog. When the button is unpushed,1 the dialog is hidden using the Hide method. In this later case we ensure that the dialog exists and is shown before trying to hide it. The AssignPixelToggle method adjusts the image and the tool tip to reflect the new state of the button.
You can run the program to see the button in action. If you do, you may notice that there are two problems we still need to address:
•The button is not pushed when the pixel data dialog is displayed using the View menu item.
•The button is not unpushed when the dialog is closed manually.
For the first problem, we simply need to adjust the button in the Click event handler for this menu. Let’s do this before we discuss the second problem.
UPDATE THE TOGGLE BUTTON WHEN THE PIXEL DATA MENU IS SELECTED
|
Action |
Result |
|
|
|
4 |
Locate the menuPixelData_Click |
private void menuPixelData_Click |
|
event handler in the MainForm.cs |
(object sender, System.EventArgs e) |
|
code window. |
{ |
|
|
|
|
|
|
5 |
Update this method to adjust the |
if (_dlgPixel == null |
|
toggle button settings. |
|| _dlgPixel.IsDisposed) |
|
|
{ |
|
|
_dlgPixel = new PixelDlg(); |
|
|
_dlgPixel.Owner = this; |
|
|
} |
|
|
_nPixelDlgIndex = _album.CurrentPosition; |
|
|
Point p = pnlPhoto.PointToClient( |
|
|
Form.MousePosition); |
|
|
UpdatePixelData(p.X, p.Y); |
|
|
AssignPixelToggle(true); |
|
|
_dlgPixel.Show(); |
|
|
} |
|
|
|
Our second problem, that of the user closing the pixel data dialog by hand, is more problematic. Since this dialog is a nonmodal window, this dialog can be closed at any time. So we need a mechanism for notifying our main window whenever the dialog is closed.
If you recall, and as shown in step 5 in the previous table, the MainForm form is defined as the owner of the PixelDlg form. This ensures that both windows are
1I know, I know. There is no such word as “unpushed.” You know what I mean. I thought about the word “released,” but unpushed seems much more packed with meaning.
428 |
CHAPTER 13 TOOLBARS AND TIPS |

shown when either window is displayed or minimized. We can take advantage of this relationship to ensure that our main window is notified when the pixel dialog is closed.
The trick is to force the MainForm window to activate whenever the PixelDlg dialog is closed. Our main form will then receive an Activated event, at which time we can update our button. Since the MainForm class derives directly from Form, we can handle this event by overriding the protected OnActivated method.
The following steps implement this mechanism.
UPDATE THE TOGGLE BUTTON WHEN THE PIXELDLG FORM IS CLOSED
|
Action |
Result |
|
|
|
6 |
In the PixelDlg.cs code window, |
protected override void OnClosing |
|
override the OnClosing method to |
(CancelEventArgs e) |
|
activate the owner of the dialog, if any. |
{ |
|
Visible = false; |
|
|
|
|
|
Note: Since the dialog may not be |
if (this.Owner != null) |
|
fully closed here if the Main- |
Owner.Activate(); |
|
|
|
|
Form.OnActivated method runs |
base.OnClosing(e); |
|
immediately, we set the Visible |
} |
|
property to false to ensure the cor- |
|
|
rect behavior occurs. |
|
|
Also note that overriding the |
|
|
OnClosed method instead does not |
|
|
work because the Owner property is |
|
|
no longer valid once the dialog has |
|
|
been closed. |
|
|
|
|
7 |
Back in the MainForm.cs code |
protected override void |
|
window, override the OnActivated |
OnActivated(EventArgs e) |
|
method. |
{ |
|
|
|
|
|
|
8 |
If the pixel dialog does not exist, |
// Update toggle button if required |
|
then make sure our button is not |
if (_dlgPixel == null |
|
pushed down. |
|| _dlgPixel.IsDisposed) |
|
{ |
|
|
|
|
|
|
AssignPixelToggle(false); |
|
|
} |
|
|
|
9 |
Otherwise, set the button state |
else |
|
based on the Visible property of |
AssignPixelToggle(_dlgPixel.Visible); |
|
the pixel dialog. |
base.OnActivated(e); |
|
|
|
|
|
} |
|
|
|
This code ensures that whenever the user closes the PixelDlg form, the main form is activated and the toggle toolbar button immediately updated. Compile and run the application to ensure that it works as expected.
TRY IT! Add two new menus to the top of the View menu called menuToolBar and menuStatusBar. Implement these menus to show and hide the corresponding controls in the application. Use the Visible property inherited from the Control class to identify the control’s current state and set it to the opposite one. If you are careful, you can implement a single Click
TOOLBAR BUTTONS |
429 |

handler for both menus by using the sender parameter and observing that both objects are Control instances. When you run the program with these changes, note how the control shows or hides their contained buttons or panels as well.
This completes our discussion of toolbars. We now move on to the mostly unrelated but similarly named ToolTip class.
13.4TOOL TIPS
You never know when a good tip might come in handy. In Windows applications, tool tips provide short and quick explanations of the purpose of a control or other object. A number of classes provide their own tool tip mechanism through a ToolTipText property, in particular the StatusBarPanel, TabPage, and ToolBarButton classes. For classes derived from the Control object, the ToolTip class handles this logic in a general fashion.
.NET Table 13.5 ToolTip class
The ToolTip class is a component that provides a small popup window for a control. This window normally contains a short phrase describing the purpose of the control, and appears whenever the mouse hovers over the control for a configurable amount of time. This class is part of the System.Windows.Forms namespace, and supports the IExtenderProvider interface. The ToolTip class derives from the
System.ComponentModel.Component class.
|
Active |
Gets or sets whether the ToolTip is currently active. When |
|
|
|
false, no tool tips will appear. The default is true. |
|
|
AutomaticDelay |
Gets or sets the default delay time in milliseconds. |
|
|
|
Whenever this property is set, the AutoPopDelay, |
|
|
|
InitialDelay, and ReshowDelay properties are initialized. |
|
|
|
The default is 500. |
|
|
AutoPopDelay |
Gets or sets the time in milliseconds before a displayed tool |
|
|
|
tip will disappear. The default is ten times the |
|
Public |
|
AutomaticDelay setting. |
|
|
|
||
Properties |
InitialDelay |
Gets or sets the time in milliseconds before a tool tip will |
|
|
|||
|
|
appear when the mouse is stationary. The default is the |
|
|
|
AutomaticDelay setting. |
|
|
ReshowDelay |
Gets or sets the time in milliseconds after the first tool tip is |
|
|
|
displayed before subsequent tool tips are displayed as the |
|
|
|
mouse moves from one assigned control to another. The |
|
|
|
default is one-fifth (1/5) the AutomaticDelay setting. |
|
|
ShowAlways |
Gets or sets whether to display the tool tip for an inactive |
|
|
|
control. The default is false. |
|
|
|
|
|
|
GetToolTip |
Retrieves the tool tip string associated with a given control. |
|
Public |
RemoveAll |
Removes all tool tip strings defined in this component. |
|
Methods |
|||
|
|
||
|
SetToolTip |
Associates a tool tip string with a given control. |
|
|
|
|
430 |
CHAPTER 13 TOOLBARS AND TIPS |