
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
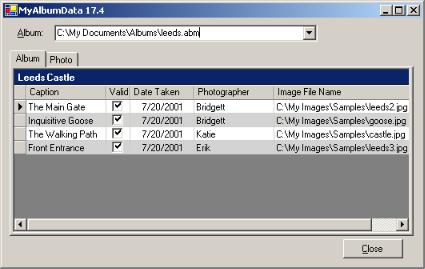
Figure 17.1 Our new application will include a tab control with a data grid on one tab page and a set of data-bound controls on a second tab page.
We begin with the DataGrid control, which displays a data source in tabular format. We will look at this class in some detail, and discuss the concept of simple data binding later in the chapter.
17.1DATA GRIDS
A data grid is just that: a grid in which data is displayed. The DataGrid class encapsulates this concept, allowing various collections of data to be displayed and manipulated by a user. The concept of data binding is central to the DataGrid class, as data is typically displayed in the control by binding an existing database table or collection class to the data grid object.
As shown in figure 17.1, the DataGrid class displays data as a set of rows and columns. The grid in general represents a specific collection of data, and this case represents a PhotoAlbum instance. Each row, in turn, represents a specific item in the overall collection, and each column represents a specific field that can be assigned to each item. In our application, each row will represent a Photograph object, and each column a possible property of a photograph.
There are a number of terms related to DataGrid controls. A summary of these is shown in figure 17.2 as they relate to our application. An overview of the DataGrid class is provided in .NET Table 17.1.
DATA GRIDS |
565 |

b
|
|
|
G |
|
|
c |
|||
d |
|
F |
|
|
E |
||||
|
Figure 17.2
This chapter will discuss many of the terms and classes related to data grids.
bCaption
Displays a short string describing the table.
cDataGridTableStyle class
Used to customize the appearance and behavior of tables.
DataGridColumnStyle class
Used to customize the order, appearance and behavior of columns. The framework supports text and boolean columns by default.
dRow Header
The area in front of a row. The small triangle indicates the current item.
eGrid Lines
The color and style of lines are configurable.
fCell
Refers to an individual value within the grid.
gColumn Header
Shows the name of each column.
The ListView class discussed in chapter 14 can also be used to present a table of information. The Windows Forms namespace provides explicit classes to represent the rows and columns in a list view. As you may recall, each item, or row, in the list is represented by the ListViewItem class instance, and each column by a ListViewSubItem instance and is presented based on a ColumnHeader instance.
As illustrated by figure 17.2, the DataGrid class takes a somewhat different approach. The contents of the grid are contained in a single collection, such as an array, a photo album, or a database table. Classes exist to configure the style in which the provided data is displayed, including colors, column ordering, and other properties. We will discuss the details of these style classes later in the chapter.
566 |
CHAPTER 17 DATA BINDING |

.NET Table 17.1 DataGrid class
The DataGrid class represents a control that displays a collection of data as a grid of rows and columns. The data displayed and the style in which it is presented is fully configurable. This class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited by this class.
|
AllowNavigation |
Gets or sets whether navigation is permitted. |
|
AlternatingBackColor |
Gets or sets the background color to use on |
|
|
every other row in the grid to create a ledger- |
|
|
like appearance. |
|
CaptionText |
Gets or sets the text to appear in the caption |
|
|
area. |
|
CaptionVisible |
Gets or sets whether the caption is visible. |
|
|
Other properties related to this and other grid |
|
|
areas are also provided. |
|
CurrentCell |
Gets or sets a DataGridCell structure |
|
|
representing the cell in the grid that has the |
|
|
focus. |
Public Properties |
CurrentRowIndex |
Gets or sets the index of the selected row. |
|
DataMember |
Gets or sets which list in the assigned data |
|
|
source should be displayed in the grid. |
|
DataSource |
Gets or sets the source of data for the grid. |
|
Item |
Gets or sets the value of a cell. This property is |
|
|
the C# indexer for this class. |
|
ReadOnly |
Gets or sets whether the grid is in read-only |
|
|
mode. |
|
RowHeaderWidth |
Gets or sets the width of row headers in pixels. |
|
TableStyles |
Gets the collection of DataGridTableStyle |
|
|
objects specifying display styles for various |
|
|
tables that may be displayed by the grid. |
|
|
|
|
BeginEdit |
Attempts to begin an edit on the grid. |
|
HitTest |
Returns location information within the grid of |
|
|
a specified point on the screen. This works |
Public Methods |
|
much like the HitTest method in the |
|
MonthCalendar class. |
|
|
SetDataBinding |
Assigns the DataSource and DataMember |
|
|
properties to the given values at run time. |
|
Unselect |
Deselects a specified row. |
|
|
|
|
CurrentCellChanged |
Occurs when the current cell has changed. |
|
DataSourceChanged |
Occurs when a new data source is assigned. |
Public Events |
Navigate |
Occurs when the user navigates to a new |
|
||
|
|
table. |
|
Scroll |
Occurs when the user scrolls the data grid. |
|
|
|
DATA GRIDS |
567 |

17.1.1CREATING THE MYALBUMDATA PROJECT
While the DataGrid class includes numerous members for customizing the appearance and behavior of the control, it is possible to create a very simple grid with only a few lines of code. We will begin with such an application, and enhance it over the course of the chapter.
The following table lays out the creation and initial layout of our new application.
CREATE THE MYALBUMDATA PROJECT
|
|
|
|
|
Action |
|
|
|
Result |
|||
|
|
|
|
|
|
|
|
|
|
|||
1 |
|
Create a new project and solution in |
The new solution is shown in the Solution Explorer |
|||||||||
|
|
Visual Studio .NET called |
window, with the default Form1.cs [Design] window |
|||||||||
|
|
“MyAlbumData.” |
|
|
|
|
|
displayed. |
||||
|
|
|
|
|
|
|
|
|
|
|||
2 |
|
Rename the Form1.cs file and |
|
|||||||||
|
|
related class file to our standard |
|
|||||||||
|
|
MainForm class and assign some |
|
|||||||||
|
|
initial settings for this form. |
|
|||||||||
|
|
|
|
|
Settings |
|
||||||
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
Property |
|
|
|
Value |
|
||||
|
|
|
(Name) |
|
MainForm |
|
||||||
|
|
|
Size |
|
|
450, 300 |
|
|
|
|||
|
|
|
Text |
|
MyAlbumData |
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
3 |
|
Drag a Label, ComboBox, |
|
|||||||||
|
|
DataGrid, and Button control onto |
|
|||||||||
|
|
the form. Arrange these controls as |
|
|||||||||
|
|
shown in the graphic. |
|
|
|
|
||||||
|
|
|
|
|
Settings |
|
|
|
|
|||
|
|
|
|
|
|
|
||||||
|
|
Control |
Property |
|
Value |
|
||||||
|
|
Label |
|
Text |
|
|
&Album |
|
||||
|
|
ComboBox |
|
(Name) |
|
|
cmbxAlbum |
|
||||
|
|
|
Anchor |
|
|
Top, Left, Right |
|
|||||
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
(Name) |
|
|
gridPhoto- |
|
|||
|
|
DataGrid |
|
|
|
|
|
Album |
|
|||
|
|
|
Anchor |
|
|
Top, Bottom, |
|
|||||
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
|
|
|
Left, Right |
|
||
|
|
|
|
|
(Name) |
|
|
btnClose |
|
|||
|
|
Button |
|
Anchor |
|
|
Bottom, Right |
|
||||
|
|
|
|
|
Text |
|
|
&Close |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
4 |
|
Create a Click event handler for |
private void btnClose_Click |
|||||||||
|
|
the Close button to shut down the |
(object sender, System.EventArgs e) |
|||||||||
|
|
application. |
|
|
|
|
|
|
|
{ |
||
|
|
|
|
|
|
|
|
|
Close(); |
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
568 |
CHAPTER 17 DATA BINDING |