
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
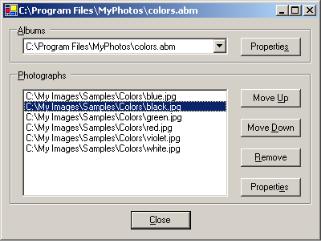
Note that the ListView and TreeView classes can also be used with collections of objects. These classes are covered in chapters 14 and 15.
We will take a slightly different approach to presenting the list controls here. Rather than using the MyPhotos application we have come to know and love, this chapter will build a new application for displaying the contents of an album, using the existing MyPhotoAlbum.dll library. This will demonstrate how a library can be reused to quickly build a different view of the same data. Our new application will be called MyAlbumEditor, and is shown in figure 10.1.
Figure 10.1
The MyAlbumEditor application does not include a menu or status bar.
10.1LIST BOXES
A list box presents a collection of objects as a scrollable list. In this section we look at the ListControl and ListBox classes. We will create a list box as part of a new MyAlbumEditor application that displays the collection of photographs in a
Album object. We will also support the ability to display our PhotoEditDlg dialog box for a selected photograph.
Subsequent sections in this chapter will extend the capabilities of this application with multiple selections of photographs and the use of combo boxes.
10.1.1CREATING A LIST BOX
The ListBox and ComboBox controls both present a collection of objects. A list box displays the collection as a list, whereas a combo box, as we shall see, displays a single item, with the list accessible through an arrow button. In the window in figure 10.1, the photo album is displayed within a ComboBox, while the collection of photographs is displayed in a ListBox. Both of these controls are derived from the ListControl class, which defines the basic collection and display functionality required in both controls. A summary of this class appears in .NET Table 10.1.
LIST BOXES |
315 |

.NET Table 10.1 ListControl class
The ListControl class is an abstract class for presenting a collection of objects to the user. You do not normally inherit from this class; instead the derived classes ListBox and ComboBox are normally used.
This class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited by this class.
|
DataSource |
Gets or sets the data source for this |
|
|
|
control. When set, the individual items |
|
|
|
cannot be modified. |
|
|
DisplayMember |
Gets or sets the property to use when |
|
|
|
displaying objects in the list control. If |
|
|
|
none is set or the setting is not a valid |
|
|
|
property, then the ToString property is |
|
|
|
used. |
|
Public Properties |
SelectedIndex |
Gets or sets the zero-based index of the |
|
|
|||
|
|
object selected in the control. |
|
|
SelectedValue |
Gets or sets the value of the object |
|
|
|
selected in the control. |
|
|
ValueMember |
Gets or sets the property to use when |
|
|
|
retrieving the value of an item in the list |
|
|
|
control. By default, the object itself is |
|
|
|
retrieved. |
|
|
|
|
|
|
GetItemText |
Returns the text associated with a given |
|
Public Methods |
|
item, based on the current |
|
|
|
DisplayMember property setting. |
|
|
|
|
|
|
DataSourceChanged |
Occurs when the DisplaySource |
|
Public Events |
|
property changes |
|
DisplayMemberChanged |
Occurs when the DisplayMember |
||
|
|||
|
|
property changes. |
|
|
|
|
Let’s see how to use some of these members to display the list of photographs contained in an album. The following steps create a new MyAlbumEditor application. We will use this application throughout this chapter to demonstrate how various controls are used. Here, we will open an album and display its contents in a ListBox using some of the members inherited from ListControl.
316 |
CHAPTER 10 LIST CONTROLS |
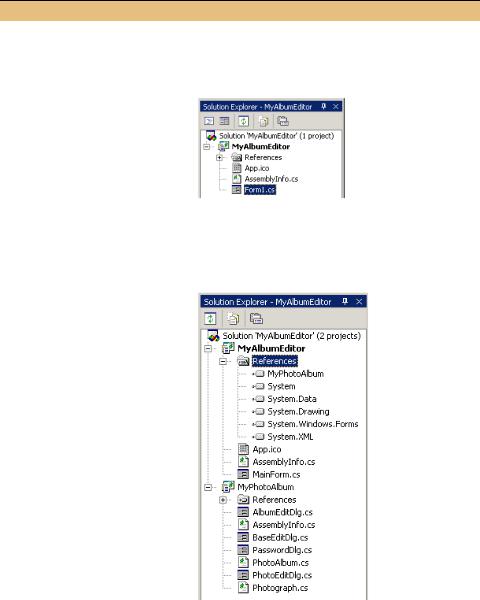
CREATE THE MYALBUMEDITOR PROJECT
|
Action |
Result |
|
|
|
1 |
Create a new project called |
The new project appears in the Solution Explorer window, |
|
“MyAlbumEditor.” |
with the default Form1 form shown in the designer |
|
How-to |
window. |
|
|
|
|
Use the File menu, or the |
|
|
keyboard shortcut |
|
|
Ctrl+Shift+N. Make sure you |
|
|
close your existing solution, if |
|
|
any. |
|
|
|
|
2 |
Rename the Form1.cs file to |
|
|
MainForm.cs. |
|
|
|
|
3 |
In the MainForm.cs source file, |
public class MainForm:System.Windows.Forms.Form |
|
rename the C# class to |
{ |
|
MainForm. |
... |
|
|
|
|
|
|
4 |
Add the MyPhotoAlbum |
|
|
project to the solution. |
|
|
How-to |
|
|
a. Right-click on the MyAlbu- |
|
|
mEditor solution. |
|
|
b. Select Existing Project… |
|
|
from the Add menu. |
|
|
c. In the Add Existing Project |
|
|
window, locate the MyPho- |
|
|
toAlbum directory. |
|
|
d. Select the MyPhotoAl- |
|
|
bum.csproj file from within |
|
|
this directory. |
|
|
|
|
5 |
Reference the MyPhotoAlbum |
|
|
project within the |
|
|
MyAlbumEditor project. |
|
|
How-to |
|
|
Right-click the References |
|
|
item in the MyAlbumEditor |
|
|
project and display the Add |
|
|
Reference dialog. |
|
|
|
|
These steps should be familiar to you if you have been following along from the beginning of the book. Since we encapsulated the PhotoAlbum and Photograph classes in a separate library in chapter 5, these objects, including the dialogs created in chapter 9, are now available for use in our application. This is quite an important point, so I will say it again. The proper encapsulation of our objects in the MyPhoto-
LIST BOXES |
317 |
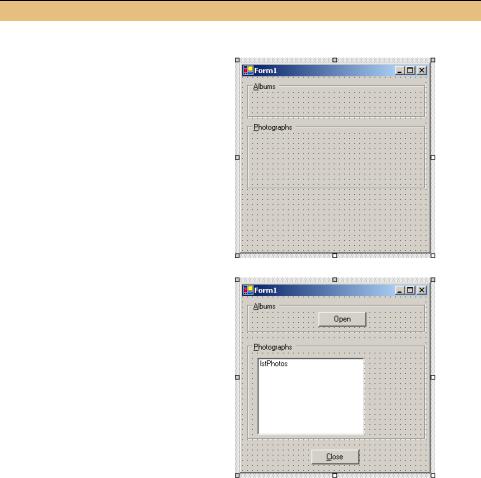
Album library in chapters 5 and 9 makes the development of our new application that much easier, and permits us to focus our attention on the list controls.
With this in mind, let’s toss up a couple of buttons and a list so we can see how control works.
Set the version number of the MyAlbumEditor application to 10.1.
CREATE THE CONTROLS FOR OUR NEW APPLICATION
|
|
|
|
Action |
|
|
|
|
Result |
|
|
|
|
|
|
|
|
|
|
|
|
6 |
Drop two GroupBox controls onto the |
|
||||||||
|
form. |
|
|
|
|
|
|
|
||
|
How-to |
|
|
|
|
|
|
|
||
|
As usual, drag them from the Toolbox |
|
||||||||
|
window. |
|
|
|
|
|
|
|
||
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
GroupBox |
|
Property |
|
|
Value |
|
||
|
|
First |
|
Anchor |
|
Top, Left, Right |
|
|||
|
|
|
|
Text |
|
|
&Albums |
|
||
|
|
Second |
|
Anchor |
|
|
Top, Bottom, |
|
||
|
|
|
|
|
|
|
Left, Right |
|
||
|
|
|
|
Text |
|
&Photo-graphs |
|
|||
|
|
|
|
|
|
|
|
|
|
|
7 |
Drop a Button control into the Albums |
|
||||||||
|
group box, a Listbox control into the |
|
||||||||
|
Photographs group box, and a Button |
|
||||||||
|
control at the base of the form. |
|
||||||||
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
Control |
Property |
|
Value |
|
||||
|
|
Open Button |
(Name) |
|
btnOpen |
|
||||
|
|
|
|
Anchor |
|
Top, Right |
|
|||
|
|
|
|
Text |
|
&Open |
|
|||
|
|
ListBox |
(Name) |
|
lstPhotos |
|
||||
|
|
|
|
Anchor |
|
Top, Bottom, |
|
|||
|
|
|
|
|
|
|
Left, Right |
|
||
|
|
Close Button |
(Name) |
|
btnClose |
|
||||
|
|
|
|
Anchor |
|
Bottom |
|
|||
|
|
|
|
Text |
|
&Close |
|
|||
|
|
|
|
|
|
|
|
|
|
Note: A couple points to note here. First, the |
|
|
|
|
|
|
|
|
|
|
Anchor settings define the resize behavior of |
|
|
|
|
|
|
|
|
|
|
the controls within their container. Note that |
|
|
|
|
|
|
|
|
|
|
the Button and ListBox here are anchored |
|
|
|
|
|
|
|
|
|
|
within their respective group boxes, and not |
|
|
|
|
|
|
|
|
|
|
to the Form itself. |
|
|
|
|
|
|
|
|
|
|
Second, since our application will not have |
|
|
|
|
|
|
|
|
|
|
a menu bar, we use the standard Close button |
|
|
|
|
|
|
|
|
|
|
as the mechanism for exiting the application. |
|
|
|
|
|
|
|
|
|
|
|
318 |
CHAPTER 10 LIST CONTROLS |