
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
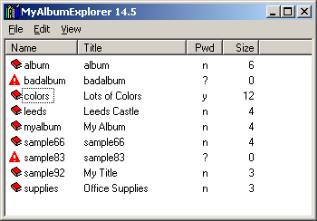
14.2THE LISTVIEW CLASS
This section begins our examination of list views by creating our new application and displaying a list view control within its main window. An overview of the ListView class appears in .NET Table 14.2.
Our initial application is shown in figure 14.2. This window displays the default, or Large Icons, view. Creating this application will require three separate tasks. First we will create the new project, then add the Menu components and ListView control required, and finally populate the ListView with the available set of albums.
Figure 14.2 MyAlbumExplorer will use a book graphic for individual albums. Note how a separate icon is used when an album cannot be opened.
14.2.1CREATING THE MYALBUMEXPLORER PROJECT
We discussed the steps for creating a new project in Visual Studio in chapter 2 and again in chapter 10. Since we have already seen this a couple of times, the following table will gloss over many of the details and just hit the highlights. We will also set an icon for our Form and application, as we discussed at the end of chapter 12.
THE LISTVIEW CLASS |
443 |
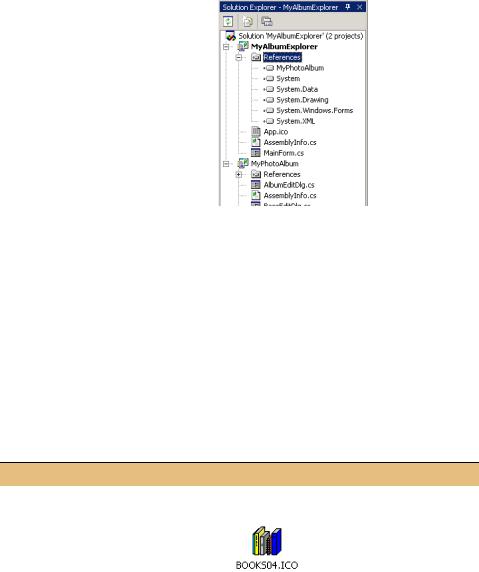
|
CREATE THE MYALBUMEXPLORER PROJECT |
|
|
|
|
|
Action |
Result |
|
|
|
1 |
Create a new Windows Application |
|
|
project called “MyAlbumExplorer.” |
|
|
|
|
2 |
Rename the Form1.cs file and |
|
|
Form1 class name to MainForm.cs |
|
|
and MainForm, respectively. |
|
|
|
|
3 |
Add the project MyPhotoAlbum to |
|
|
the solution. |
|
|
|
|
4 |
Reference this project within the |
|
|
MyAlbumExplorer project. |
|
|
|
|
5 |
In the MainForm.cs code window, |
protected override void OnLoad(EventArgs e) |
|
override the OnLoad method to |
{ |
|
display the version number in the |
// Assign title bar |
|
Version v = new Version(Application. |
|
|
title bar. |
|
|
ProductVersion); |
|
|
|
this.Text = String.Format( |
|
|
"MyAlbumExplorer {0:#}.{1:#}", |
|
|
v.Major, v.Minor); |
|
|
} |
|
|
|
This creates a solution for our new application. We will also establish an icon for the Form as well as the generated application file. This uses the term common image directory, which as you’ll recall is our shorthand for the graphics files provided with Visual Studio .NET. By default, these can be found in “C:\Program Files\Microsoft Visual Studio .NET\Common7\Graphics.”
Set the version number of the MyAlbumExplorer application to 14.2.
DEFINE ICONS FOR THE FORM AND APPLICATION
|
Action |
Result |
|
|
|
6 |
In the MainForm.cs [Design] window, |
|
|
set the Icon property for the Form to |
|
|
use the icon file “icons/Writing/ |
|
|
BOOKS04.ICO” in the common image |
|
|
directory. |
|
|
|
|
7 |
Delete the existing “App.ico” icon file |
|
|
for the MyAlbumExplorer project. |
|
|
|
|
444 |
CHAPTER 14 LIST VIEWS |
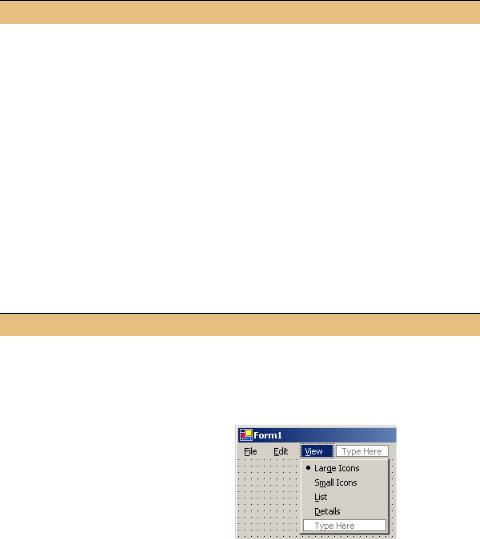
DEFINE ICONS FOR THE FORM AND APPLICATION (continued)
|
Action |
Result |
|
|
|
8 |
Set the Application Icon setting for the |
The icon is presented to the Windows operating |
|
MyAlbumExplorer project to use the |
system to represent the application. |
|
BOOKS04.ICO icon as well. |
|
|
How-to |
|
|
Right-click on the project name in |
|
|
Solution Explorer and select the |
|
|
Properties item to display the |
|
|
appropriate dialog. |
|
|
|
|
With these tasks out of the way, we are ready to add a ListView control to our form.
14.2.2CREATING A LIST VIEW
This section will drop some menu objects and a list view control onto our form so we can examine and manipulate these controls in Visual Studio .NET. These steps will also create some menus we will use as we move through the chapter.
ADD A MENU AND LIST VIEW TO OUR FORM
|
|
|
Action |
|
|
Result |
|
|
|
|
|
|
|
1 |
Add a MainMenu object to the form in |
|
||||
|
the MainForm.cs [Design] window. |
|
||||
|
|
|
|
|
|
|
2 |
Create the following top-level menus. |
This graphic is the result of steps 2 and 3. |
||||
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
Menu |
Property |
Value |
|
|
|
|
File |
(Name) |
menuFile |
|
|
|
|
|
Text |
&File |
|
|
|
|
Edit |
(Name) |
menuEdit |
|
|
|
|
|
Text |
&Edit |
|
|
|
|
View |
(Name) |
menuView |
|
|
|
|
|
Text |
&View |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
THE LISTVIEW CLASS |
445 |

ADD A MENU AND LIST VIEW TO OUR FORM (continued)
|
|
|
|
|
|
Action |
Result |
|||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||
3 |
Create four menus underneath the |
Note: The View menus allow the user to alter |
||||||||||||
|
View menu. |
|
|
|
|
|
|
|
|
how the ListView appears. To match the |
||||
|
|
|
|
|
|
Settings |
style used by Windows Explorer, we set the |
|||||||
|
|
|
|
|
|
RadioCheck property to true so that a small |
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Menu |
Property |
|
Value |
circle is used as the check mark. |
||||||||
|
|
Large |
(Name) |
|
menuLargeIcons |
|
||||||||
|
|
Icons |
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
Checked |
|
True |
|
||||||
|
|
|
|
|
RadioCheck |
|
True |
|
||||||
|
|
|
|
|
|
Text |
|
Lar&ge Icons |
|
|||||
|
|
Small |
(Name) |
|
menuSmallIcons |
|
||||||||
|
|
Icons |
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
RadioCheck |
|
True |
|
||||||
|
|
|
|
|
|
Text |
|
S&mall Icons |
|
|||||
|
|
List |
(Name) |
|
menuList |
|
||||||||
|
|
|
|
|
RadioCheck |
|
True |
|
||||||
|
|
|
|
|
|
Text |
|
&List |
|
|||||
|
|
Details |
(Name) |
|
menuDetails |
|
||||||||
|
|
|
|
|
RadioCheck |
|
True |
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
4 |
Add an Exit menu underneath the File |
private void menuExit_Click |
||||||||||||
|
menu, along with an appropriate |
(object sender, System.EventArgs e) |
||||||||||||
|
Click event handler to close the |
{ |
||||||||||||
|
Close(); |
|||||||||||||
|
form. |
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
} |
||||
|
|
|
|
|
|
Settings |
|
|||||||
|
|
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
Property |
|
|
Value |
|
||||||
|
|
|
|
(Name) |
|
menuExit |
|
|||||||
|
|
|
|
|
Text |
|
|
|
|
E&xit |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
5 |
Establish appropriate Size and Text |
|
||||||||||||
|
properties for the MainForm form. |
|
||||||||||||
|
|
|
|
|
|
Settings |
|
|||||||
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
Property |
|
|
|
Value |
|
|||||
|
|
|
|
|
Size |
|
|
|
400, 300 |
|
|
|
|
|
|
|
|
|
|
Text |
|
|
MyAlbumExplorer |
|
|||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
446 |
CHAPTER 14 LIST VIEWS |