
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
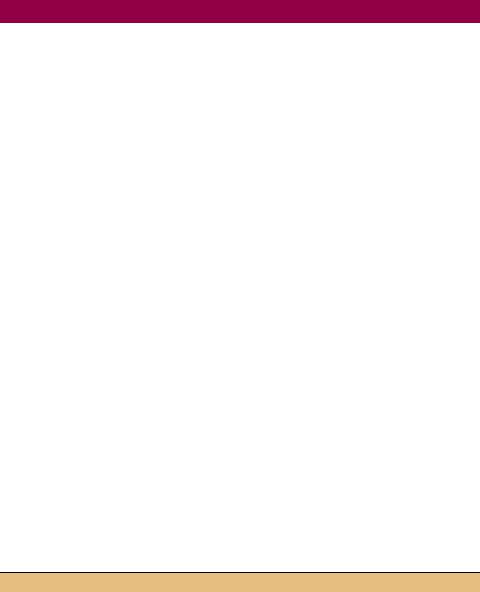
.NET Table 4.3 StatusBarPanel class
The StatusBarPanel class is a component that appears as a panel within a StatusBar control. This class is part of the System.Windows.Forms namespace, and inherits from the System.ComponentModel.Component class. A panel must be associated with a StatusBar instance with its ShowPanels property set to true in order to appear on a form.
|
Alignment |
Gets or sets the HorizontalAlignment for the |
|
|
|
panel’s text. |
|
|
AutoSize |
Gets or sets how the panel is sized within the |
|
|
|
status bar. |
|
|
BorderStyle |
Gets or sets the type of border to display for the |
|
|
|
panel, if any. |
|
|
MinWidth |
Gets or sets the minimum width for the panel. |
|
Public Properties |
Parent |
Gets the StatusBar object that contains this |
|
|
|||
|
|
panel. |
|
|
Style |
Gets or sets the style used to draw the panel. |
|
|
Text |
Gets or sets the text for the panel. |
|
|
ToolTipText |
Gets or sets the tool tip for the panel. |
|
|
Width |
Gets the current width or sets the default width |
|
|
|
for the panel. |
|
|
|
|
|
|
BeginInit |
Begins initialization of the panel when used |
|
Public Methods |
|
within a form or other component. |
|
EndInit |
Ends initialization of the panel when used within |
||
|
|||
|
|
a form or other component. |
|
|
|
|
4.3.2ASSIGNING PANEL TEXT
With our panels defined, we simply set the Text property value for each panel to have the text displayed by the application. This only works for panels with their
Style property set to Text, of course. We will look at our owner-drawn panel in section 4.4. Since our panels only have meaning after an image is loaded, we assign their values as part of the Click event handler for the Load button, as indicated by the following steps.
SET THE TEXT TO APPEAR IN THE PANELS
|
Action |
Result |
|
|
|
1 |
In the menuLoad_Click |
private void menuLoad_Click |
|
method, set the ShowPanels |
(object sender, System.EventArgs e) |
|
property to false while the |
{ |
|
. . . |
|
|
image is loading. |
|
|
try |
|
|
|
{ |
|
|
statusBar1.ShowPanels = false; |
|
|
|
116 |
CHAPTER 4 STATUS BARS |

SET THE TEXT TO APPEAR IN THE PANELS (continued)
|
Action |
Result |
|
|
|
2 |
Initialize the sbpnlFileName |
statusBar1.Text = "Loading " + dlg.FileName; |
|
and sbpnlImageSize panels |
pbxPhoto.Image = new Bitmap(dlg.OpenFile()); |
|
after the image is success- |
|
|
|
|
|
fully loaded. |
statusBar1.Text = "Loaded " + dlg.FileName; |
|
|
this.sbpnlFileName.Text = dlg.FileName; |
|
|
this.sbpnlImageSize.Text |
|
|
= String.Format("{0:#} x {1:#}", |
|
|
pbxPhoto.Image.Width, |
|
|
pbxPhoto.Image.Height); |
|
|
|
3 |
Set the ShowPanels property |
statusBar1.ShowPanels = true; |
|
to true so the panel text will |
} |
|
appear. |
. . . |
|
} |
|
|
|
|
|
|
|
Look again at the new try block.
try |
|
{ |
Disable the |
statusBar1.ShowPanels = false; |
b panels |
statusBar1.Text = "Loading " + dlg.FileName;
pbxPhoto.Image = new Bitmap(dlg.OpenFile());
statusBar1.Text = "Loaded " + dlg.FileName; this.sbpnlFileName.Text = dlg.FileName; this.sbpnlImageSize.Text
=String.Format("{0:#} x {1:#}", pbxPhoto.Image.Width, pbxPhoto.Image.Height);
statusBar1.ShowPanels = true;
}
cCreate image size string
Two items are worth noting in this code:
bThe ShowPanels property is set to false while an image is loading so that the StatusBar.Text property setting will appear, and set to true after the image is loaded and the panels are set.
cThe Format method used here is a static method provided by the String class for constructing a string. We could spend a chapter covering this and other features available in C# strings generally and the .NET System.String class specifically, but instead will assume you can look this one up in the documentation. In the code shown here, the "{0:#} x {1:#}" string indicates that two parameters are required, both of them integers.
Build and run the application to see these panels in action. Resize the window to see how the panels react. You will notice that the first panel resizes automatically along with the window, while the second two panels maintain their initial size. This is consistent with the AutoSize settings we used for these objects.
STATUS BAR PANELS |
117 |
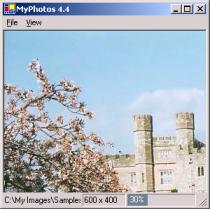
4.4OWNER-DRAWN PANELS
So what about this owner-drawn panel? Text panels do not need to worry about drawing their text onto the panel, as the .NET Framework handles this internally. There are some cases where text just will not do, and these situations requiring manual drawing of the panel.
Drawing of panels and other objects in .NET are handled through use of the System.Drawing namespace, sometimes referred to as GDI+ since it is based on an update to the graphical drawing interface provided by Microsoft. Components such as menus, status bars, and tabs that contain drawable components support a DrawItem event that occurs when an item in the component should be drawn. Controls derived from the Control class provide a Paint event for this purpose. Both types of drawing make use of the Graphics class discussed in this section in order to draw the item.
This section will examine how owner-drawn status bar panels are supported, and draw the sbpnlImagePercent panel for our application. A similar discussion would apply to owner-drawn menu items or other objects supporting the DrawItem event. The result of our changes is shown in figure 4.5.
Figure 4.5
The third status bar panel here indicates that 30 percent of the image is visible in the window.
As you can see in the figure, when the image is displayed in Actual Size mode, the third panel will show a numeric and visual representation of how much of the image is displayed. Before we draw this panel, let’s take a closer look at the DrawItem event.
4.4.1THE DRAWITEM EVENT
The DrawItem event is used by a number of classes to draw an item contained within some sort of larger collection. For instance, the MenuItem, ListBox, and ComboBox classes all include a DrawItem event for custom drawing of their contents. These classes use the DrawItemEventArgs class to provide the data associated with the event. The StatusBar class uses a derived version of this class, but the bulk of the drawing information is in the base class. An overview of this base class is provided in .NET Table 4.4.
118 |
CHAPTER 4 STATUS BARS |

.NET Table 4.4 DrawItemEventArgs class
The DrawItemEventArgs class is an event object used when handling DrawItem events in a number of classes. This class is part of the System.Windows.Forms namespace, and inherits from the System.EventArgs class. Practically, this class is used to manually draw list box items, menu items, status bar panels and other objects.
The StatusBarDrawItemEventArgs class extends this class for use with StatusBar objects. This class includes a public Panel property to indicate which panel requires drawing.
|
Bounds |
Gets the Rectangle of the area to be drawn |
|
|
|
with respect to the entire graphical area for the |
|
|
|
object. |
|
|
Font |
Gets a suggested Font to use for any text. |
|
|
|
Typically, this is the parent’s Font property. |
|
|
ForeColor |
Gets a suggested Color to use for foreground |
|
|
|
elements, such as text. Typically, this is |
|
|
|
SystemColors.WindowText, or |
|
|
|
SystemColors.HighlightText if the object |
|
Public Properties |
|
is selected. |
|
Graphics |
Gets the Graphics object to use for painting |
||
|
|||
|
|
the item. |
|
|
Index |
Gets the index of the item to be painted. The |
|
|
|
exact meaning of this property depends on the |
|
|
|
object. |
|
|
State |
Gets additional state information on the object, |
|
|
|
using the DrawItemState enumeration. |
|
|
|
Examples include whether the item is |
|
|
|
selected, enabled, has the focus, or is checked |
|
|
|
(for menus). |
|
|
|
|
|
|
DrawBackground |
Draws the Bounds rectangle with the default |
|
Public Methods |
|
background color. |
|
|
|
||
|
DrawFocusRectangle |
Draws a focus rectangle in the Bounds area. |
|
|
|
|
For the StatusBar class, the StatusBarDrawItemEventArgs class derives from the DrawItemEventArgs class and is received by StatusBar.DrawItem event handlers. The Panel property provided by this class is useful both for identifying the panel and when the text assigned to the panel is needed.
When a DrawItem event handler is invoked, the default property values are what you might expect. The Bounds property is set to the display rectangle of the panel to draw. This rectangle is with respect to the rectangle for the containing status bar, so the upper left corner of a panel’s bounds is not (0,0). The Font and ForeColor properties are set to the font information for the StatusBar object; the Graphics property to an appropriate drawing object, the Index to the zero-based index number of the panel, and State is typically set to DrawItemState.None. The DrawItem event is called once for each panel drawn.
OWNER-DRAWN PANELS |
119 |

.NET Table 4.5 System.Drawing namespace
The System.Drawing namespace provides access to basic graphics functionality provided by the graphical device interface (GDI+). The classes in this namespace are used when drawing to any display device such as a screen or printer, and to represent drawing primitives such as rectangles and points.
|
Brush |
An abstract class representing an object used to fill the interior |
|
|
of a graphical shape. For example, the |
|
|
Graphics.FillRectangle method uses a brush to fill a |
|
|
rectangular area on a drawing surface. Classes derived from |
|
|
this class include the SolidBrush and TextureBrush classes. |
|
Brushes |
A sealed class that provides Brush objects for all standard |
|
|
colors. For example, the Brushes.Red property can be used to |
|
|
fill shapes with a solid red color. |
|
Font |
Represents a font that defines how text is drawn. This includes |
|
|
the font style and size as well as the font face. |
|
Graphics |
Represents a GDI+ drawing surface. Members are provided to |
|
|
draw shapes, lines, images, and other objects onto the drawing |
|
|
surface. |
|
Image |
An abstract class for image objects such as Bitmap. |
Classes |
Pen |
Represents an object used to draw lines and curves. A pen can |
|
|
draw a line in any color and specify various styles such as line |
|
|
widths, dash styles, and ending shapes (such as arrows). For |
|
|
example, the Graphics.DrawRectangle method uses a pen |
|
|
to draw the outline of a rectangular area on a drawing surface. |
|
Region |
Represents the interior of a graphics shape composed of |
|
|
rectangles and paths. |
|
SystemColors |
A sealed class that provides Color objects for the colors |
|
|
configured in the local Windows operating system. For |
|
|
example, the SystemColors.Control property returns the |
|
|
color configured for filling the surface of controls. Similar |
|
|
classes also exist for Brush, Pen, and Icon objects based on |
|
|
the local system configuration. |
|
Color |
Stores a color value. A number of static colors are defined, |
|
|
such as Color.Red, or a custom color can be created from an |
|
|
alpha component value and a set of RGB values. |
|
|
|
|
Point |
A two-dimensional point as an integral x and y coordinate. |
|
PointF |
A two-dimensional point as a floating point x and y coordinate. |
|
Rectangle |
Stores the location and size of a rectangular region within a |
Structures |
|
two-dimensional area. All coordinates are integral values. |
|
|
|
|
Size |
Represents the size of a rectangular region as an integral width |
|
|
and height. |
|
SizeF |
Represents the size of a rectangular region as a floating point |
|
|
width and height. |
|
|
|
120 |
CHAPTER 4 STATUS BARS |