
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
C# keywords |
(continued) |
|
|
|
|
|
|
|
|
Keyword |
|
Description |
Example |
See also |
|
|
|
|
|
unsafe |
|
Indicates an unmanaged |
See example for stackalloc keyword. |
|
|
|
region of code, in which |
|
|
|
|
pointers are permitted and |
|
|
|
|
normal runtime verification is |
|
|
|
|
disabled. |
|
|
ushort |
|
Denotes an unsigned 16-bit |
ushort apprxCircum |
uint; |
|
|
integer value. A cast is |
ushort radius = (ushort)7; |
ulong |
|
|
required to convert an int or |
ushort pi = (ushort)314; |
|
|
|
apprxCircum = (ushort)2 |
|
|
|
|
uint value to ushort. |
|
|
|
|
* pi * radius / (ushort)100; |
|
|
using |
|
As a directive, indicates a |
using System.Windows.Forms; |
section 1.2.1 on |
|
|
namespace from which types |
using App = Application; |
page 15 |
|
|
do not have to be fully |
public void Main() |
|
|
|
qualified. |
|
|
|
|
{ |
|
|
|
|
Alternatively, indicates a |
Form f = new MainForm(); |
|
|
|
shortcut, or alias, for a given |
App.Run(f); |
|
|
|
class or namespace name. |
} |
|
|
|
|
|
|
|
|
As a statement, defines a |
using (OpenFileDialog dlg |
discussion in |
|
|
scope for a given expression or |
= new OpenFileDialog()) |
section 8.2.1, |
|
|
type. At the end of this scope, |
{ |
page 233 |
|
|
// Do something with dlg |
||
|
|
the given object is disposed. |
|
|
|
|
} |
|
|
virtual |
|
Declares that a method or |
See example for override keyword. |
override; |
|
|
property member may be |
|
section 9.1.1, |
|
|
overridden in a derived class. |
|
page 265 |
|
|
At runtime, the override of a |
|
|
|
|
type member is always |
|
|
|
|
invoked. |
|
|
volatile |
|
Indicates that a field may be |
// Read/Write x anew for each line. |
|
|
|
modified in a program at any |
volatile double x = 70.0; |
|
|
|
time, such as by the operating |
int num = x; |
|
|
|
x = x * Sqrt(x); |
|
|
|
|
system or in another thread. |
|
|
|
|
|
|
|
void |
|
Indicates that a method does |
See examples for override and protected key- |
examples |
|
|
not return a value. |
words. |
throughout text |
while |
|
As a statement, executes a |
Photograph p = _album.FirstPhoto; |
for; |
|
|
statement or block of |
while (p != null) |
foreach |
|
|
statements until a given |
{ |
|
|
|
// Do something with Photograph |
|
|
|
|
expression is false. |
|
|
|
|
p = _album.NextPhoto; |
|
|
|
|
|
} |
|
|
|
In a do-while loop, specifies |
See example for do keyword. |
do; |
|
|
the condition that will |
|
|
|
|
terminate the loop. |
|
|
|
|
|
|
|
A.4 SPECIAL FEATURES
This section presents some noteworthy features of the C# language. These topics did not fit in previous sections of this appendix, but are important concepts for
SPECIAL FEATURES |
667 |

programming in the language. The topics covered are exceptions, arrays, the Main entry point, boxing, and documentation.
Readers more familiar with C# will recognize certain features omitted from this discussion and the book in general. These include attributes, reflection, and the preprocessor. These features, while important, were considered beyond the scope of this book, and are not required in many Windows Forms applications. A brief discussion of attributes is provided in chapter 2 as part of a discussion on the AssemblyInfo.cs file.
A.4.1 EXCEPTIONS
An exception is a type of error. Exceptions provide a uniform type-safe mechanism for handling system level and application level error conditions. In the .NET Framework, all exceptions inherit from the System.Exception class. Even system-level errors such as divide-by-zero and null references have well-defined exception classes.
If a program or block of code ignores exceptions, then exceptions are considered unhandled. By default, an unhandled exception immediately stops execution of a program.2 This ensures that code which ignores exceptions does not continue processing when an error occurs. Code that does not ignore exceptions is said to handle exceptions, and must indicate the specific set of exception classes that are handled by the code. An exception is said to be handled or caught if a block of code can continue processing after an exception occurs. Code which generates an exception is said to throw the exception.
The try keyword is used to indicate a block of code that handles exceptions. The catch keyword indicates which exceptions to explicitly handle. The finally keyword is used to indicate code that should be executed regardless of whether an exception occurs.
Code that handles one or more exceptions in this manner uses the following format:
try
<try-block>
<catch-blocks>opt
<finally-block>opt
where
• <try-block> is the set of statements, enclosed in braces, that should handle exceptions.
• <catch-blocks> is optional, and consists of one or more catch blocks as defined below.
2 Well, most of the time. If an unhandled exception occurs during the execution of a static constructor, then a TypeInitializationException is thrown rather than the program exiting. In this case, the original exception is included as the inner exception of the new exception.
668 |
APPENDIX A C# PRIMER |
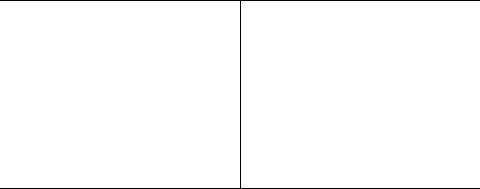
• <finally-block> is optional, and consists of the finally keyword followed by the set of statements, enclosed in braces, that should execute whether or not an exception occurs.
The format of a try block allows for one or more catch blocks, also called catch clauses, to define which exceptions to process. These are specified with the catch keyword in the following manner:
catch <exception>opt
<catch-block>
where
• <exception> is optional, and indicates the exception this catch clause will handle. This must be a class enclosed in parenthesis with an optional identifier that the block will use to reference this exception. If no class is provided, then all exceptions are handled by the clause.
• <catch-block> is the set of statements, enclosed in braces, that handles the given exception.
For example, one use for exceptions is to handle unexpected conversion errors, such as converting a string to an integer. The following side-by-side code contrasts two ways of doing this:
// A string theString requires conversion int version = 0;
try
{
version = Convert.ToInt32(theString);
}
catch
{
version = 0;
}
If any exception occurs while converting the string to an int, then the catch clause will set version to 0. For example, if the theString variable is null, an ArgumentException will occur, and version will still be set to 0.
// A string theString requires conversion int version = 0;
try
{
version = Convert.ToInt32(theString);
}
catch (FormatException)
{
version = 0;
}
The catch clause will set version to 0 only if a FormatException exception occurs while converting the string to an int. Any other exception is unhandled and will exit the program if not handled by a previous method.
When an exception occurs in a program that satisfies more than one catch block within the same try block, the first matching block is executed. For this reason, the more distinct exceptions should appear first in the list of catch blocks. As an example, consider the IOException class, which is thrown when an unexpected I/O error occurs. This class derives from the Exception class. The following code shows how an exception block might be written to handle exceptions that might occur while reading a file:
// Open some file system object
FileStream f = new FileStream(...);
SPECIAL FEATURES |
669 |
try
{
//Code that makes use of FileStream object
}
catch (IOException ioex)
{
//Code that handles an IOException
//This code can use the "ioex" variable to reference the exception
}
catch (Exception ex)
{
// Code that handles any other exception
}
Additional examples of exceptions appear throughout the book, beginning in section 2.3.2 on page 58.
A.4.2 ARRAYS
An array is a data structure consisting of a collection of variables, all of the same type. Arrays are built into C# and may be one-dimensional or many-dimensional. Each dimension of an array has an associated integral length. Arrays are treated as reference types. In the .NET Framework, the System.Array class serves as the base class for all array objects. More information on the Array and related ArrayList class can be found in chapter 5.
A standard array for any type is constructed using square brackets in the following manner:
<type> [ <dimension>opt ]
where
• <type> is the non-array type for the array. A non-array type is any type that is not an array.
• <dimension> is zero or more commas ‘,’ indicating the dimensions of the array.
Note that multiple square brackets may be specified to have variable length array elements. An example of this is shown below. To reference a value in an array, square brackets are again used, with an integer expression from zero (0) to one less than the length of the array. If an array index is outside of the valid range of the array, an IndexOutOfRangeException object is thrown as an exception.
Some examples of arrays and additional comments on the use of arrays are given below. Note that the Length property from the System.Array class determines the number of elements in an array, and the foreach keyword can be used on all arrays to enumerate the elements of the array.
// an uninitialized array defaults to null
int[] a;
670 |
APPENDIX A C# PRIMER |