
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index

In our tree view for the MyAlbumExplorer application, we would like to represent each album as a node in the tree, with each album containing a node for each photograph in that album. Since albums can appear in any directory, we might also wish to indicate where a set of albums is located. We will do this by generating a tree structure similar to the one shown in figure 15.6. This tree was generated in Visual Studio to illustrate the hierarchy we will employ. The ListView control in this figure is totally unrelated to the contents of our tree. This is not what we ultimately want, but it is okay for now.
Figure 15.6
In the TreeView, note how the selected album employs a different icon than the unselected one
As an introduction to tree nodes, let’s create the structure shown in figure 15.6 in Visual Studio .NET. The following table details the steps required.
|
|
|
CREATE TREE NODES IN VISUAL STUDIO |
||
|
|
|
|
|
|
|
|
Action |
|
|
Result |
|
|
|
|
|
|
1 |
In the MainForm.cs [Design] |
|
|||
|
window, set the ImageList |
|
|||
|
property of the tree view to use |
|
|||
|
the existing imageListSmall |
|
|||
|
component already associated |
|
|||
|
with the form. |
|
|
|
|
|
|
|
|
|
|
2 |
Set default index values for |
|
|||
|
nodes in the tree. |
|
|
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
Property |
Value |
|
|
|
|
ImageIndex |
1 |
|
|
|
|
SelectedImageIndex |
4 |
|
|
|
|
|
|
|
|
494 |
CHAPTER 15 TREE VIEWS |

CREATE TREE NODES IN VISUAL STUDIO (continued)
|
|
|
|
|
Action |
|
|
|
|
Result |
|
|
|
|
|
|
|
|
|
|
|
||
3 |
|
Display the TreeNode Editor |
|
||||||||
|
|
dialog box for the control. |
|
||||||||
|
|
How-to |
|
|
|
|
|
|
|
||
|
|
Click the … button for the Nodes |
|
||||||||
|
|
entry in the Properties window, |
|
||||||||
|
|
as shown in the graphic for |
|
||||||||
|
|
steps 1 and 2. |
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
||
4 |
|
Create a top-level node for the |
A top-level Default Albums node appears in the TreeNode |
||||||||
|
|
tree. |
|
|
|
|
|
|
Editor. This node is shown in the graphic for step 6. |
||
|
|
How-to |
|
|
|
|
|
|
|
||
|
|
Click the Add Root button. |
|
||||||||
|
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
Property |
|
Value |
|
|||||
|
|
|
Label |
|
Default Albums |
|
|||||
|
|
|
Image |
|
books image |
|
|||||
|
|
|
Selected Image |
books image |
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
5 |
|
Add three child nodes for the |
Both nodes appear using the default indexes. |
||||||||
|
|
Default Albums node. |
Note: When you select a node, notice how the |
||||||||
|
|
How-to |
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
selected image assigned to the node is displayed in |
|||
|
|
Add each node by clicking the |
the tree. |
||||||||
|
|
Add Child button while the |
|
||||||||
|
|
Default Albums node is |
|
||||||||
|
|
selected. |
|
|
|
|
|
|
|
||
|
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
Node |
|
Property |
|
Value |
|
|||
|
|
|
First |
|
Label |
|
Album 1 |
|
|||
|
|
|
Second |
|
Label |
|
Album 2 |
|
|||
|
|
|
Third |
|
Label |
|
Album 3 |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
THE TREEVIEW CLASS |
495 |
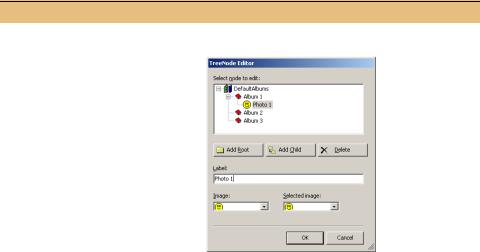
CREATE TREE NODES IN VISUAL STUDIO (continued)
|
|
Action |
Result |
||
|
|
|
|
|
|
6 |
Add a child node for the Album 1 |
|
|||
|
node. |
|
|
|
|
|
How-to |
|
|
|
|
|
Click the Add Child button while |
|
|||
|
the Album 1 node is selected. |
|
|||
|
|
Settings |
|
||
|
|
|
|
|
|
|
|
Property |
Value |
|
|
|
|
Label |
Photo 1 |
|
|
|
|
Image |
The normal face |
|
|
|
|
|
image |
|
|
|
|
Selected Image |
The smiley face |
|
|
|
|
|
image |
|
|
|
|
|
|
|
|
7 |
Click the OK button to save the |
The nodes are displayed in the designer window. |
|||
|
new nodes. |
|
|
|
|
|
|
|
|
|
|
The new nodes appear in the designer window, and are present as we saw in figure 15.6. Run the program and note how the image changes when each node is selected. Also note the plus and minus signs that appear to indicate whether a node is expanded or collapsed.
Let’s take a look at the code generated in the InitializeComponent method. The assignment of the Nodes property is shown, reformatted to be a bit more readable than the code that is generated by Visual Studio.
this.treeViewMain.Nodes.AddRange(new System.Windows.Forms.TreeNode[]
{
new System.Windows.Forms.TreeNode("Default Albums", 5, 5,
new System.Windows.Forms.TreeNode[]
{
new System.Windows.Forms.TreeNode("Album 1",
new System.Windows.Forms.TreeNode[]
{
new System.Windows.Forms.TreeNode("Photo 1", 0, 3)
}),
new System.Windows.Forms.TreeNode("Album 2"), new System.Windows.Forms.TreeNode("Album 3")
})
});
This code uses various forms of the TreeNode constructor to create the nodes in the tree. If you look carefully, you will realize that the Nodes property for the tree contains a single entry, our root Default Albums node. This root node is created to contain an array of three TreeNode objects, namely the Album 1, Album 2, and Album 3 nodes.
496 |
CHAPTER 15 TREE VIEWS |