
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf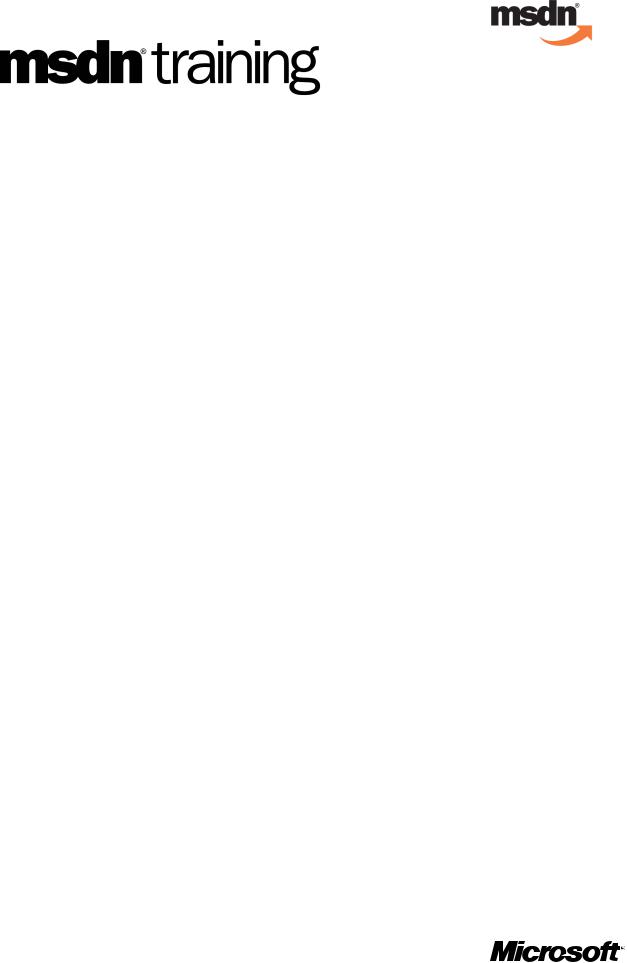
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET)
Delivery Guide
Course Number: 2349B
Part Number: X08-76381
Released: 02/2002
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places, and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, places or events is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001−2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.
Course Number: 2349B
Part Number: X08-76381
Released: 02/2002
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
iii |
|
|
|
|
Contents
Introduction |
|
Introduction.............................................................................................................. |
1 |
Course Materials ...................................................................................................... |
2 |
Prerequisites............................................................................................................. |
3 |
Course Outline ......................................................................................................... |
4 |
Microsoft Certified Professional Program ............................................................... |
9 |
Facilities................................................................................................................. |
11 |
Module 1: Overview of the Microsoft .NET Framework |
|
Overview.................................................................................................................. |
1 |
Overview of the Microsoft .NET Framework.......................................................... |
2 |
Overview of Namespaces ...................................................................................... |
13 |
Review ................................................................................................................... |
17 |
Module 2: Introduction to a Managed Execution Environment |
|
Overview.................................................................................................................. |
1 |
Writing a .NET Application..................................................................................... |
2 |
Compiling and Running a .NET Application......................................................... |
11 |
Lab 2: Building a Simple .NET Application.......................................................... |
29 |
Review ................................................................................................................... |
32 |
Module 3: Working with Components |
|
Overview.................................................................................................................. |
1 |
An Introduction to Key .NET Framework Development Technologies .................. |
2 |
Creating a Simple .NET Framework Component .................................................... |
4 |
Lab 3.1: Creating a .NET Framework Component ................................................ |
11 |
Creating a Simple Console Client.......................................................................... |
14 |
Lab 3.2: Creating a Simple Console-Based Client................................................. |
19 |
Demonstration: Creating a Windows Forms Client ............................................... |
22 |
Creating an ASP.NET Client ................................................................................. |
27 |
Lab 3.3: Calling a Component Through an ASP.NET Page.................................. |
36 |
Review ................................................................................................................... |
40 |
Module 4: Deployment and Versioning |
|
Overview.................................................................................................................. |
1 |
Introduction to Application Deployment ................................................................. |
2 |
Application Deployment Scenarios ......................................................................... |
7 |
Related Topics and Tools....................................................................................... |
31 |
Lab 4: Packaging and Deployment ........................................................................ |
37 |
Review ................................................................................................................... |
42 |
Module 5: Common Type System |
|
Overview.................................................................................................................. |
1 |
An Introduction to the Common Type System ........................................................ |
2 |
Elements of the Common Type System................................................................... |
8 |
Object-Oriented Characteristics............................................................................. |
25 |
Lab 5: Building Simple Types ............................................................................... |
39 |
Review ................................................................................................................... |
44 |

iv |
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
|
|
Module 6: Working with Types |
|
|
Overview................................................................................................................. |
1 |
|
System.Object Class Functionality ......................................................................... |
2 |
|
Specialized Constructors....................................................................................... |
12 |
|
Type Operations.................................................................................................... |
18 |
|
Interfaces............................................................................................................... |
28 |
|
Managing External Types ..................................................................................... |
34 |
|
Lab 6: Working with Types .................................................................................. |
38 |
|
Review .................................................................................................................. |
43 |
|
Module 7: Strings, Arrays, and Collections |
|
|
Overview................................................................................................................. |
1 |
|
Strings ..................................................................................................................... |
2 |
|
Terminology – Collections.................................................................................... |
20 |
|
.NET Framework Arrays....................................................................................... |
21 |
|
.NET Framework Collections ............................................................................... |
39 |
|
Lab 7: Working with Strings, Enumerators, and Collections................................ |
57 |
|
Review .................................................................................................................. |
63 |
|
Module 8: Delegates and Events |
|
|
Overview................................................................................................................. |
1 |
|
Delegates................................................................................................................. |
2 |
|
Multicast Delegates............................................................................................... |
12 |
|
Events.................................................................................................................... |
21 |
|
When to Use Delegates, Events, and Interfaces.................................................... |
31 |
|
Lab 8: Creating a Simple Chat Server................................................................... |
32 |
|
Review .................................................................................................................. |
42 |
|
Module 9: Memory and Resource Management |
|
|
Overview................................................................................................................. |
1 |
|
Memory Management Basics.................................................................................. |
2 |
|
Non-Memory Resource Management ................................................................... |
12 |
|
Implicit Resource Management ............................................................................ |
13 |
|
Explicit Resource Management ............................................................................ |
26 |
|
Optimizing Garbage Collection ............................................................................ |
36 |
|
Lab 9: Memory and Resource Management ......................................................... |
49 |
|
Review .................................................................................................................. |
56 |
|
Module 10: Data Streams and Files |
|
|
Overview................................................................................................................. |
1 |
|
Streams.................................................................................................................... |
2 |
|
Readers and Writers................................................................................................ |
5 |
|
Basic File I/O .......................................................................................................... |
8 |
|
Lab 10: Files ......................................................................................................... |
21 |
|
Review .................................................................................................................. |
26 |

Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
v |
Module 11: Internet Access |
|
Overview.................................................................................................................. |
1 |
Internet Application Scenarios................................................................................. |
2 |
The WebRequest and WebResponse Model............................................................ |
3 |
Application Protocols............................................................................................. |
16 |
Handling Errors...................................................................................................... |
25 |
Security .................................................................................................................. |
28 |
Best Practices......................................................................................................... |
35 |
Lab 11: Creating a DateTime Client/Server Application....................................... |
36 |
Review ................................................................................................................... |
41 |
Course Evaluation.................................................................................................. |
43 |
Module 12: Serialization |
|
Overview.................................................................................................................. |
1 |
Serialization Scenarios............................................................................................. |
2 |
Serialization Attributes ............................................................................................ |
4 |
Object Graph............................................................................................................ |
5 |
Serialization Process ................................................................................................ |
7 |
Serialization Example .............................................................................................. |
9 |
Deserialization Example ........................................................................................ |
10 |
Custom Serialization.............................................................................................. |
12 |
Custom Serialization Example............................................................................... |
14 |
Security Issues ....................................................................................................... |
17 |
Lab 12: Serialization .............................................................................................. |
18 |
Review ................................................................................................................... |
27 |
Module 13: Remoting and XML Web Services |
|
Overview.................................................................................................................. |
1 |
Remoting.................................................................................................................. |
2 |
Remoting Configuration Files................................................................................ |
19 |
Demonstration: Remoting...................................................................................... |
22 |
Lab 13.1: Building an Order-Processing Application by Using Remoted Servers 28 |
|
XML Web Services................................................................................................ |
36 |
Lab 13.2: Using an XML Web Service.................................................................. |
48 |
Review ................................................................................................................... |
54 |
Course Evaluation.................................................................................................. |
56 |

vi |
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
|
|
Optional Modules |
|
|
Module 14 (Optional): Threading and Asynchronous Programming |
|
|
Overview................................................................................................................. |
1 |
|
Introduction to Threading ....................................................................................... |
2 |
|
Using Threads in .NET ........................................................................................... |
9 |
|
Thread Safety........................................................................................................ |
29 |
|
Special Thread Topics........................................................................................... |
51 |
|
Asynchronous Programming in .NET................................................................... |
74 |
|
Lab 14: Working With Multithreaded Applications ............................................. |
92 |
|
Review ................................................................................................................ |
108 |
|
Module 15 (Optional): Interoperating Between Managed and |
|
|
Unmanaged Code |
|
|
Overview................................................................................................................. |
1 |
|
Integration Services................................................................................................. |
2 |
|
Platform Invoke ...................................................................................................... |
7 |
|
Lab 15.1: Calling Win32 APIs.............................................................................. |
16 |
|
Calling COM Objects from Managed Code.......................................................... |
20 |
|
Lab 15.2: Calling COM Objects ........................................................................... |
39 |
|
Calling .NET Objects from COM Objects............................................................ |
43 |
|
Review .................................................................................................................. |
55 |
|
Module 16 (Optional): Using Microsoft ADO.NET to Access Data |
|
|
Overview................................................................................................................. |
1 |
|
Overview of ADO.NET .......................................................................................... |
2 |
|
Connecting to a Data Source................................................................................. |
10 |
|
Accessing Data with DataSets .............................................................................. |
12 |
|
Using Stored Procedures....................................................................................... |
26 |
|
Lab 16: Using ADO.NET to Access Data ............................................................ |
34 |
|
Accessing Data with DataReaders ........................................................................ |
42 |
|
Binding to XML Data ........................................................................................... |
50 |
|
Review .................................................................................................................. |
56 |
|
Module 17 (Optional): Attributes |
|
|
Overview................................................................................................................. |
1 |
|
Overview of Attributes............................................................................................ |
2 |
|
Defining Custom Attributes .................................................................................. |
13 |
|
Retrieving Attribute Values .................................................................................. |
22 |
|
Demonstration: Custom Attributes ....................................................................... |
26 |
|
Lab 17: Defining and Using Attributes................................................................. |
27 |
|
Review .................................................................................................................. |
36 |
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
vii |
|
|
|
|
About This Course
This section provides you with a brief description of the course, audience, suggested prerequisites, course objectives, and information about course customization.
Description
The goal of this five day course is to help application developers understand the Microsoft® .NET Framework. In addition to offering an overview of the .NET Framework and an introduction to key concepts and terminology, the course provides a series of labs, which introduce and explain .NET Framework features that are used to code, debug, tune, and deploy applications.
Audience
This course is intended for experienced, professional software developers who work in independent software vendors (ISVs) or work on corporate enterprise development teams. Most students will be Microsoft Visual C++® (or C++) and Java developers.
Student Prerequisites
This course requires that students meet the following prerequisites:
•Students should be experienced professional developers and have a basic understanding of the C# language.
Students can meet the C# language prerequisite by taking Course 2124,
Introduction to C# Programming for the Microsoft .NET Platform.
Course Objectives
After completing this course, the student will be able to:
!List the major elements of the .NET Framework and explain how they fit into the .NET platform.
!Explain the main concepts behind the common language runtime and use the features of the .NET Framework to create a simple application.
!Create and use components in Microsoft Windows® Forms-based and ASP.NET-based applications.
!Use the deployment and versioning features of the .NET runtime to deploy multiple versions of a component.
!Create, use, and extend types by understanding the Common Type System architecture.
!Create classes and interfaces that are functionally efficient and appropriate for specific programming scenarios.
!Use the .NET Framework class library to efficiently create and manage strings, arrays, collections, and enumerators.

viiiProgramming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET)
!Use delegates and events to have an event sender object signal the occurrence of an action to an event receiver object.
!Describe and control how memory and other resources are managed in the
.NET Framework.
!Read from and write to data streams and files.
!Use the basic request/response model to send and receive data over the Internet.
!Serialize and deserialize an object graph.
!Create distributed applications through XML Web services and Object Remoting.
Programming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET) |
ix |
|
|
|
|
Course Timing
This section provides estimated course timings for all of the modules, labs, and breaks in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C#™ .NET). Your timing may vary.
Day 1
Start |
End |
Module |
|
|
|
9:00 |
9:30 |
Introduction |
|
|
|
9:30 |
10:00 |
Module 1: Overview of the Microsoft .NET Framework |
|
|
|
10:00 |
10:15 |
Break |
10:15 |
11:00 |
Module 2: Introduction to a Managed Execution Environment |
|
|
|
11:00 |
11:15 |
Lab 2: Building a Simple .NET Application |
|
|
|
11:15 |
11:45 |
Module 3: Working with Components |
|
|
|
11:45 |
12:00 |
Lab 3.1: Creating a .NET Framework Component |
|
|
|
12:00 |
1:00 |
Lunch |
1:00 |
1:15 |
Module 3: Working with Components (continued) |
|
|
|
1:15 |
1:30 |
Lab 3.2: Creating a Simple Console-Based Client |
|
|
|
1:30 |
2:00 |
Module 3: Working with Components (continued) |
|
|
|
2:00 |
2:30 |
Lab 3.3: Calling a Component Through an ASP .NET Page |
|
|
|
2:30 |
2:45 |
Break |
2:45 |
4:15 |
Module 4: Deployment and Versioning |
|
|
|
Day 2
Start |
End |
Module |
|
|
|
9:00 |
9:50 |
Lab 4: Packaging and Deployment |
|
|
|
9:50 |
10:00 |
Break |
10:00 |
11:30 |
Module 5: Common Type System |
|
|
|
11:30 |
12:30 |
Lunch |
12:30 |
1:15 |
Lab 5: Building Simple Types |
|
|
|
1:15 |
2:30 |
Module 6: Working with Types |
|
|
|
2:30 |
2:45 |
Break |
2:45 |
3:30 |
Lab 6: Working with Types |
|
|
|
3:30 |
4:00 |
Module 7: Strings, Arrays, and Collections |
|
|
|

xProgramming with the Microsoft® .NET Framework (Microsoft Visual C#™ .NET)
Day 3
Start |
End |
Module |
|
|
|
9:00 |
10:30 |
Module 7: Strings, Arrays, and Collections (continued) |
|
|
|
10:30 |
10:45 |
Break |
10:45 |
11:45 |
Lab 7: Working with Strings, Enumerators, and Collections |
|
|
|
11:45 |
12:45 |
Lunch |
12:45 |
2:00 |
Module 8: Delegates and Events |
|
|
|
2:00 |
2:15 |
Break |
2:15 |
3:30 |
Lab 8: Creating a Simple Chat Server |
|
|
|
3:30 |
4:00 |
Module 9: Memory and Resource Management |
|
|
|
Day 4
Start |
End |
Module |
|
|
|
9:00 |
10:30 |
Module 9: Memory and Resource Management (continued) |
|
|
|
10:30 |
10:45 |
Break |
10:45 |
11:45 |
Lab 9: Memory and Resource Management |
|
|
|
11:45 |
12:45 |
Lunch |
12:45 |
1:30 |
Module 10: Data Streams and Files |
|
|
|
1:30 |
2:15 |
Lab 10: Files |
|
|
|
2:15 |
2:30 |
Break |
2:30 |
3:30 |
Module 11: Internet Access |
|
|
|
3:30 |
4:15 |
Lab 11: Creating a DateTime Client/Server Application |
|
|
|
Day 5
Start |
End |
Module |
|
|
|
9:00 |
9:30 |
Module 12: Serialization |
|
|
|
9:30 |
10:15 |
Lab 12: Serialization |
|
|
|
10:15 |
10:30 |
Break |
10:30 |
11:30 |
Module 13: Remoting and Web Services |
|
|
|
11:30 |
12:30 |
Lunch |
12:30 |
1:20 |
Lab 13.1: Building an Order-Processing Application by Using |
|
|
Remoted Servers |
|
|
|
1:20 |
2:20 |
Module 13: Remoting and Web Services (continued) |
|
|
|
2:20 |
2:35 |
Break |
2:35 |
3:30 |
Lab 13.2: Using an XML Web Service |
|
|
|