
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf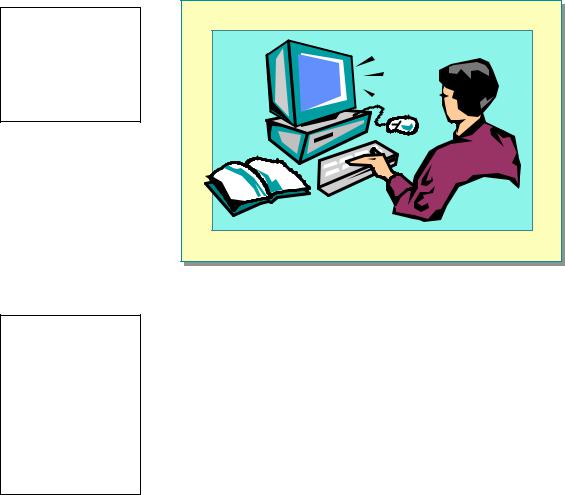
Module 3: Working with Components |
11 |
|
|
|
|
Lab 3.1: Creating a .NET Framework Component
Topic Objective
To introduce the lab.
Lead-in
In this lab, you will learn how to create a simple
.NET Framework component in C#.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
For Your Information
In this introductory module, the lab problems have intentionally been made very similar to the problems presented in the module. This approach is designed to allow the student to experience a wide range of
.NET software approaches within a very limited amount of time.
Objective
After completing this lab, you will be able to create a simple .NET Framework component in C#.
Lab Setup
Only solution files are associated with this lab. The solution files for this lab are in the folder <install folder>\Labs\Lab03.1\Solution.
Scenario
This lab is based on a small client server application scenario in which you write both the client application and server component using C#.
This lab focuses on building the server component.
Lab 3.2, “Creating a Simple Console-Based Client,” focuses on building a client program that calls the server component.
Estimated time to complete this lab: 15 minutes
12 |
Module 3: Working with Components |
Exercise 1
Creating a Component in C#
In this exercise, you will use C# to create a component that provides a wrapper for a private array of strings. The wrapper includes a read-only Count property, which returns the number of strings in the array, and a public GetString method, which takes an integer argument and returns the string in the private array at that index. The GetString method will also implement structured exception handling to ensure that the argument of the index is within range of the array.
!Specify namespace information and class declarations
1.Open Notepad and reference the System namespace.
2.Create a new namespace named CompCS. CompCS will contain the class for your component.
! Create the class implementation
1.Create a public class named StringComponent.
2.Declare a private field named StringSet of type array of string elements.
3.Create a public default constructor.
The default constructor takes no arguments.
4.Assign to the field StringSet an array of strings initialized with the following four strings:
"C# String 0",
"C# String 1",
"C# String 2",
"C# String 3"
5.Create the public GetString method, which takes an integer as an argument. That integer must be a valid index for the StringSet array.
a.If the integer is an invalid index, throw an index out of range exception.
b.If the integer is a valid index, return the StringSet string at that index.
6.Create a read-only Count property that gets the number of string elements in the StringSet array.
7.Save the file as CompCS.cs in the <install folder>\Labs\Lab03.1 folder.
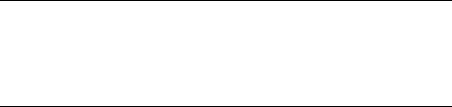
Module 3: Working with Components |
13 |
|
|
|
|
! Compile the source code
Important To use Microsoft Visual Studio .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click Start, All Programs, Microsoft Visual Studio .NET,
Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
•From a Visual Studio .NET command prompt window, enter the command to build a library named CompCS.dll from the CompCS.cs. source. You must specify that the target is a library.
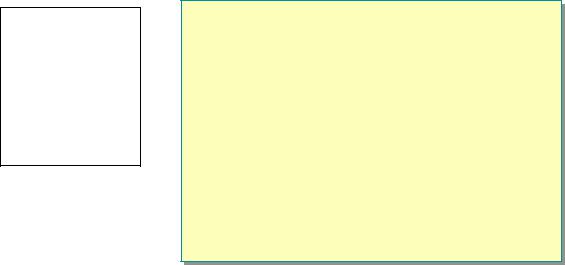
14 |
Module 3: Working with Components |
" Creating a Simple Console Client
Topic Objective
To provide an overview of the section topics.
Lead-in
In this section, you will learn how to write a simple console application that calls a .NET Framework runtime-compatible component.
!Using the Libraries
!Instantiating the Component
!Calling the Component
!Building the Client
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In this section, you will learn how to write a simple console application that calls the .NET Framework runtime-compatible component that was created in the previous section.
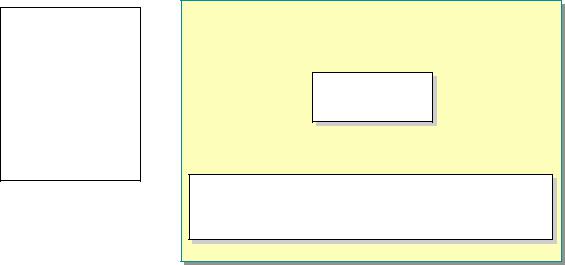
Module 3: Working with Components |
15 |
|
|
|
|
Using the Libraries
Topic Objective
To explain how to import libraries and to use namespace aliasing to remove ambiguity.
Lead-in
By using the namespaces in the program, you can reference types in the library without fully qualifying the type name.
!Reference Types Without Having to Fully Qualify the Type Name
using CompCS; using CompCS;
using CompVB; using CompVB;
!If Multiple Namespaces Contain the Same Type Name, Create a Namespace Alias to Remove Ambiguity
using CSStringComp = CompCS.StringComponent; using CSStringComp = CompCS.StringComponent;
using VBStringComp = CompVB.StringComponent; using VBStringComp = CompVB.StringComponent;
*****************************ILLEGAL FOR NON-TRAINER USE******************************
This topic explains how to reference types in your applications by using namespaces and how to use a namespace alias if you need to remove ambiguity to type references.
Using the Namespaces
By using the namespaces in the program, you can reference types in the library without fully qualifying the type name.
To use the component namespaces in C#, you must enter a using statement followed by the name of the component namespaces:
using CompCS; using CompVB;
Using an Alias
Consider a scenario where similar C# and Microsoft Visual Basic® components use the same type name (StringComponent). You must still fully qualify the type name to remove any ambiguity when referring to the GetString method and the Count property. In C#, you can create and use aliases to solve this problem.
The following C# example shows how to alias the component namespaces so that you do not have to fully qualify the type names:
using CSStringComp = CompCS.StringComponent; using VBStringComp = CompVB.StringComponent;
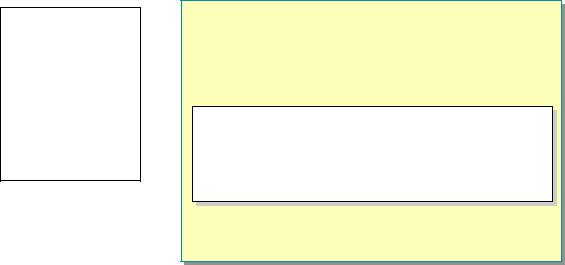
16 |
Module 3: Working with Components |
Instantiating the Component
Topic Objective
To describe how to instantiate the component in C#.
Lead-in
To instantiate the
StringComponent class, you declare a local variable of type StringComponent and create a new instance of StringComponent.
!Declare a Local Variable of Type StringComponent
!Create a New Instance of the StringComponent Class
//…
//…
using CSStringComp = CompCS.StringComponent; using CSStringComp = CompCS.StringComponent; //… //…
CSStringComp myCSStringComp = new CSStringComp myCSStringComp = new CSStringComp();
CSStringComp();
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To instantiate the StringComponent class, you declare a local variable of type StringComponent and create a new instance of StringComponent, as in the following example:
//…
using CSStringComp = CompCS.StringComponent;
class MainApp
{
public static void Main() {
CSStringComp myCSStringComp = new CSStringComp(); //…
}
}

Module 3: Working with Components |
17 |
|
|
|
|
Calling the Component
Topic Objective
To describe how to call a component after it has been instantiated.
Lead-in
After instantiating the
StringComponent class, the client can iterate over the string array in the C# or Visual Basic component and return the appropriate output.
!Iterate over All the Members of StringComponent and Output the Strings to the Console
for (int index = 0; for (int index = 0;
index < myCSStringComp.Count; index++) { index < myCSStringComp.Count; index++) {
Console.WriteLine
Console.WriteLine
(myCSStringComp.GetString(index));
}}
(myCSStringComp.GetString(index));
*****************************ILLEGAL FOR NON-TRAINER USE******************************
After instantiating the StringComponent class, the client can iterate over the string array in the component and return the appropriate output, as in the following example:
using System;
using CSStringComp = CompCS.StringComponent;
class MainApp
{
public static void Main() {
CSStringComp myCSStringComp = new CSStringComp(); // Display result strings from CS component
Console.WriteLine("Strings from CS StringComponent"); for (int index = 0; index < myCSStringComp.Count; index++) {
Console.WriteLine(myCSStringComp.GetString(index));
}
}
}

18 |
Module 3: Working with Components |
Building the Client
Topic Objective
To show how to build the client in C#.
Lead-in
To build the client, you can compile the source code from a command prompt window.
!Use the /reference Switch to Reference the Assemblies That Contain the StringComponent Class
csc /reference:CompCS.dll,CompVB.dll! csc /reference:CompCS.dll,CompVB.dll! /out:ClientCS.exe ClientCS.cs
/out:ClientCS.exe ClientCS.cs
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To build the client, you can compile the source code from a command prompt window. You must use the /reference option to reference all of the assemblies that contain the StringComponent class, as in the following example:
csc /reference:CompCS.dll,CompVB.dll!
/out:ClientCS.exe ClientCS.cs
Running a client program that first iterates over the C# component’s strings and then iterates over the Visual Basic component’s strings will produce the following output:
Strings from C# StringComponent
C# String 0
C# String 1
C# String 2
C# String 3
Strings from VB StringComponent
VB String 0
VB String 1
VB String 2
VB String 3
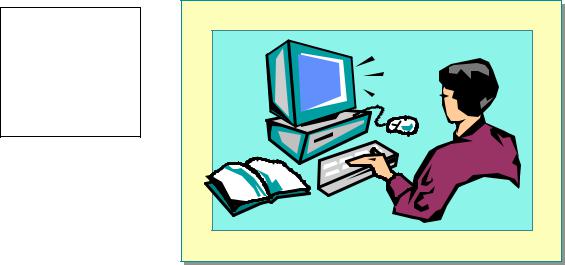
Module 3: Working with Components |
19 |
|
|
|
|
Lab 3.2: Creating a Simple Console-Based Client
Topic Objective
To introduce the lab.
Lead-in
In this lab, you will learn how to create a simple console-based client application that calls a component.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Objectives
After completing this lab, you will be able to create a simple console-based client application that calls a component.
Lab Setup
To complete this lab you will need the solution files from Lab 3.1 and a
Visual Basic component provided for you in the <install folder>\Labs\Lab03.2\ Starter\VB\Component folder. The solution files for this lab are located in <install folder>\Labs\Lab03.2\Solution.
Scenario
This lab is based on a small client/server application scenario in which both the client application and server component are written by using C#.
This lab focuses on building a simple console-based client application that calls the component that was created in Lab 3.1, “Creating a .NET Framework Component.” Also, another version of the component written in Visual Basic is provided. The client will use both the C# and Visual Basic component versions to show cross-language compatibility.
Estimated time to complete this lab: 15 minutes
20 |
Module 3: Working with Components |
Exercise 1
Creating a Client Application in C#
In this exercise, you will create a simple console application to call the C# component that you created in Lab 3.1, “Creating a .NET Framework Component,” and a Visual Basic component that is already provided. The Visual Basic component provides the same StringComponent class as the C# component.
!Specify namespace information
1.Open Notepad and import the System namespace.
2.Use the namespace of the C# component that you created in Lab 3.1, “Creating a .NET Framework Component.”
3.Use the namespace of the Visual Basic component. The namespace of the Visual Basic component is CompVB.
Because you are importing two libraries that contain the same type name but have different namespaces, use an alias directive to allow for unambiguous references to StringComponent classes.
4.Create a class in C# called MainApp.
5.Specify the program entry point.
The entry point takes no arguments and does not return a value.
!Write the code to call the C# and Visual Basic components
1.Assign to a local variable named myCSStringComp a new instance of the C# version of StringComponent.
2.Write the code to print the following string to the console:
Strings from C# StringComponent
3.Iterate over all of the members of the C# string component and output the strings to the console.
4.Repeat steps 1 through 3 for the Visual Basic version of StringComponent. Name the local variable myVBStringComp.
5.Save the file as ClientCS.cs in the <install folder>\Labs\Lab03.2 folder.