
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf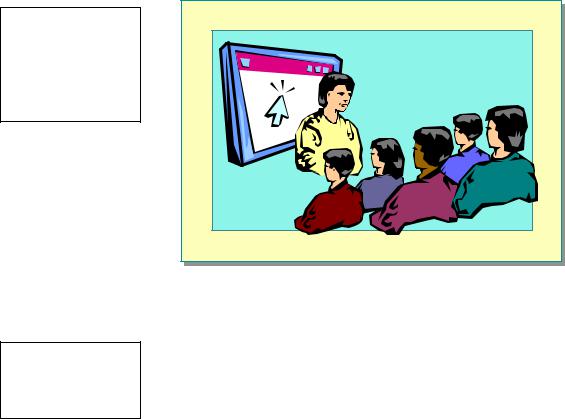
Module 2: Introduction to a Managed Execution Environment |
3 |
|
|
|
|
Demonstration: Hello World
Topic Objective
To demonstrate how to build a simple application in C#.
Lead-in
In this demonstration, you will learn how to build a simple application in C#.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Delivery Tip
As this is a short, simple demonstration, you may want to let students try it themselves.
In this demonstration, you will learn how to build a simple application in C#.
! To create the source code in C#
1.Open Notepad and type the following code:
//Allow easy reference to System namespace classes using System;
//Create class to hold program entry point
class MainApp {
public static void Main() {
// Write text to the console Console.WriteLine(“Hello World using C#!”);
}
}
2. Save the file as HelloDemoCS.cs
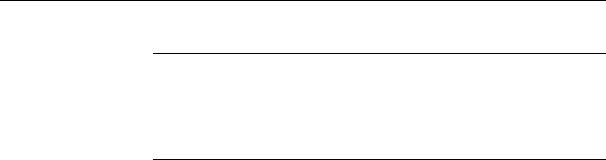
4Module 2: Introduction to a Managed Execution Environment
! To compile the source code and build an executable program
Important To use Visual Studio .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click
Start, All Programs, Microsoft Visual Studio .NET, Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
•From a Visual Studio .NET Command Prompt window, type the following syntax:
csc HelloDemoCS.cs
Running the resulting executable will generate the following output:
Hello World using C#!
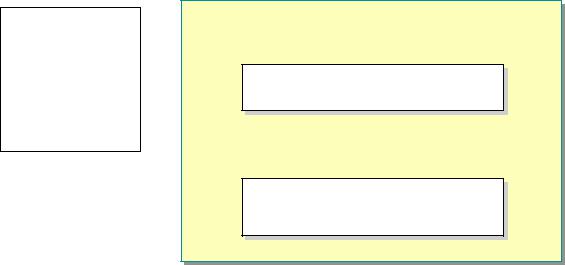
Module 2: Introduction to a Managed Execution Environment |
5 |
|
|
|
|
Using a Namespace
Topic Objective
To describe how to use namespaces in the .NET Framework.
Lead-in
You can fully reference classes in which an instance of System.IO.FileStream is declared by using C#:
! Classes Can Be Fully Referenced
// declares a FileStream object // declares a FileStream object System.IO.FileStream aFileStream; System.IO.FileStream aFileStream;
!Or the Namespace of a Class Can Be Referenced
#No need to fully qualify contained class names
using System.IO; using System.IO;
...
...
FileStream aFileStream;
FileStream aFileStream;
*****************************ILLEGAL FOR NON-TRAINER USE******************************
You can fully reference classes, as in the following example, in which an instance of System.IO.FileStream is declared by using C#:
System.IO.Filestream aFileStream;
However, it is more convenient to reference the required namespaces in your program. Using the namespace effectively disposes of the need to qualify all class library references, as in the following example:
using System.IO;
...
FileStream aFileStream;
For example, in order to have convenient access to System objects, you must use the System namespace.
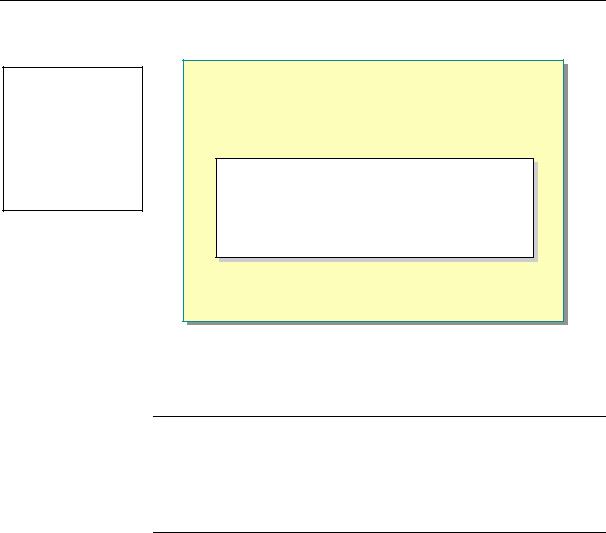
6Module 2: Introduction to a Managed Execution Environment
Defining a Namespace and a Class
Topic Objective
To describe how to define namespaces and classes in C#.
Lead-in
C# supports the creation of custom namespaces and classes within those namespaces.
!C# Supports Creation of Custom Namespaces and Classes Within Those Namespaces
namespace CompCS { namespace CompCS {
public class StringComponent { public class StringComponent {
...
}}
}}
...
*****************************ILLEGAL FOR NON-TRAINER USE******************************
C# supports the creation of custom namespaces and classes within those namespaces.
Tip The following is the general rule for naming namespaces:
CompanyName.TechnologyName
For example:
Microsoft.Office
This is merely a guideline. Third-party companies can choose other names.
Namespaces in C#
In C#, you use the namespace statement to define a new namespace, which encapsulates the classes that you create, as in the following example:
namespace CompCS {
public class StringComponent {
...
}
}
Note that a namespace may be nested in other namespaces, and a single namespace may be defined in multiple files. A single source code file may also define multiple namespaces.
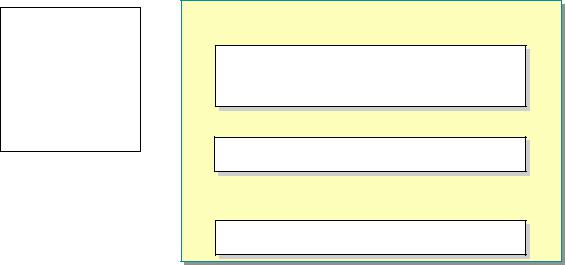
Module 2: Introduction to a Managed Execution Environment |
7 |
|
|
|
|
Entry Points, Scope, and Declarations
Topic Objective
To describe how to create program entry points in C#.
Lead-in
Every executable program must contain an external entry point, where the application begins its execution.
! In C#, the External Entry Point for a Program Is in a Class
class MainApp class MainApp
{ public static void Main() { public static void Main()
}}
{. . .} {. . .}
! C# Supports the Use of a Period As a Scope Resolution Operator
Console.WriteLine ("First String");
Console.WriteLine ("First String");
!In C#, Objects Must Be Declared Before They Can Be Used and Are Instantiated Using the New Keyword
Lib.Comp myComp = new Lib.Comp();
Lib.Comp myComp = new Lib.Comp();
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Every executable program must contain an external entry point, where the application begins its execution. In C#, all code must be contained in methods of a class.
Entry Points in C#
To accommodate the entry point code in C#, you must first specify the class, as in the following example:
class MainApp {...}
Next, you specify the entry point for your program. The compiler requires this entry point to be a public static method called Main, as in the following example:
public static void Main () {...}
Scope
C# uses the period as a scope resolution operator. For example, you use the syntax Console.WriteLine when referencing the WriteLine method of the
Console object.
Declaring and Instantiating Variables
In C#, you must declare a variable before it can be used. To instantiate the object, use the new keyword. The following example in C# shows how to declare an object of type Comp, in namespace Lib, with the name myComp:
Lib.Comp myComp = new Lib.Comp();
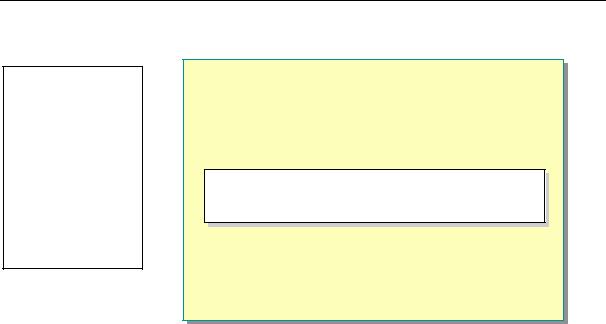
8Module 2: Introduction to a Managed Execution Environment
Console Input and Output
Topic Objective
To describe how to use Console class methods in C#.
Lead-in
You can use the runtime Console class of the System namespace for input and output to the console of any string or numeric value by using the
Read, ReadLine, Write, and WriteLine methods.
!Console Class Methods
#Read, ReadLine, Write, and WriteLine
Console.WriteLine("Hello World using C#!"); Console.WriteLine("Hello World using C#!");
*****************************ILLEGAL FOR NON-TRAINER USE******************************
You can use the common language runtime Console class of the System namespace for input and output to the console of any string or numeric value by using the Read, ReadLine, Write, and WriteLine methods.
The following example shows a C# program that outputs a string to the console:
using System;
class MainApp {
public static void Main() {
//Write text to the console Console.WriteLine(“Hello World using C#!”);
}
}
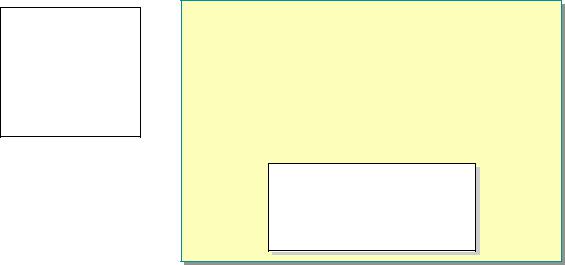
Module 2: Introduction to a Managed Execution Environment |
9 |
|
|
|
|
Case Sensitivity
Topic Objective
To describe case sensitivity issues in programming languages.
Lead-in
C++ and C# are casesensitive, but Visual Basic is not case-sensitive.
!Do Not Use Names That Require Case Sensitivity
#Components should be fully usable from both casesensitive and case-insensitive languages
#Case should not be used to distinguish between identifiers within a single name scope
!Avoid the Following
class customer {...} class customer {...} class Customer {...} class Customer {...}
void foo(int X, int x) void foo(int X, int x)
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Microsoft Visual C++® and C# are case-sensitive, but Microsoft Visual Basic® is not case-sensitive. To ensure that a program is compliant with the Common Language Specification (CLS), however, you must take special care with public names. You cannot use case to distinguish between identifiers within a single name scope, such as types within assemblies and members within types.
The following examples show situations to avoid:
! Do not have two classes or namespaces whose names differ only by case.
class customer { ... } class Customer { ... }
!Do not have a function with two parameters whose names differ only by case.
void foo(int X, int x)
This constraint enables Visual Basic (and potentially other case-insensitive languages) to produce and use components that have been created in other casesensitive languages. This constraint does not apply to your definitions of private classes, private methods on public classes, or local variables.
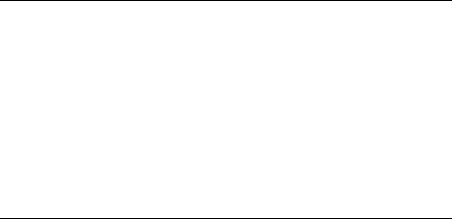
10 |
Module 2: Introduction to a Managed Execution Environment |
Note To fully interact with other objects regardless of the language they were implemented in, objects must expose to callers only those features that are common to all the languages they must interoperate with. For this reason, a set of language features has been defined, called the Common Language Specification (CLS), which includes common language features that are needed by many applications. The CLS rules define a subset of the common type system; that is, all the rules that apply to the common type system apply to the CLS, except where stricter rules are defined in the CLS. If your component uses only CLS features in the API that it exposes to other code (including derived classes), the component is guaranteed to be accessible from any programming language that supports the CLS. Components that adhere to the CLS rules and use only the features included in the CLS are said to be CLS-compliant components.
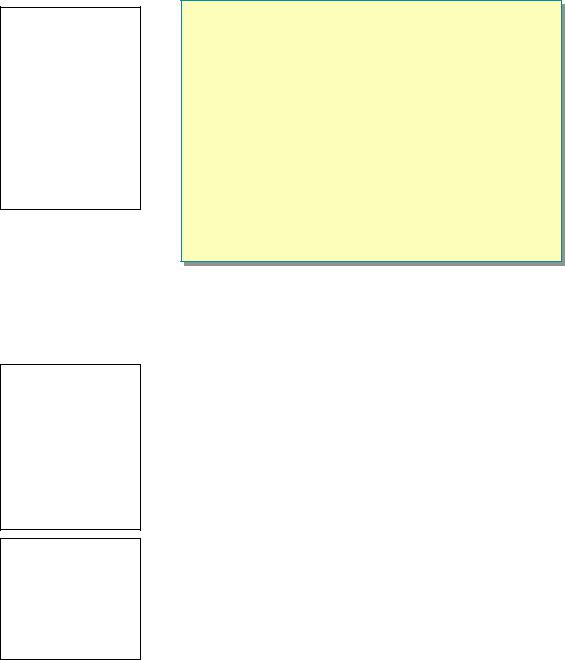
Module 2: Introduction to a Managed Execution Environment |
11 |
|
|
|
|
" Compiling and Running a .NET Application
Topic Objective
To introduce the topics in the section.
Lead-in
Most aspects of programming in the .NET Framework are the same for all compatible languages; each supported language compiler produces selfdescribing, managed Microsoft intermediate language (MSIL) code.
!Compiler Options
!The Process of Managed Execution
!Metadata
!Microsoft Intermediate Language
!Assemblies
!Common Language Runtime Tools
!Just-In-Time Compilation
!Application Domains
!Garbage Collection
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Delivery Tip
Emphasize that you are primarily introducing new concepts and terminology. Be prepared to postpone answering questions that pertain to later modules. Encourage students to start reading the .NET Framework SDK documentation.
Most aspects of programming in the .NET Framework are the same for all compatible languages; each supported language compiler produces selfdescribing, managed Microsoft intermediate language (MSIL) code. All managed code runs using the common language runtime, which provides cross-language integration, automatic memory management, cross-language exception handling, enhanced security, and a consistent and simplified programming model.
This section introduces basic concepts of a managed execution environment and presents new terminology. Many of these concepts are covered in greater detail in subsequent modules in this course, in subsequent courses, and in the .NET Framework SDK documentation.
Key Points
String literals in an application are stored and transported as clear text. Therefore, you should avoid putting sensitive information such as passwords in string literals.
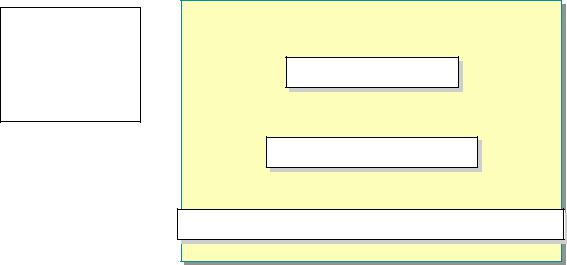
12 |
Module 2: Introduction to a Managed Execution Environment |
Compiler Options
Topic Objective
To introduce compiler options in C#.
Lead-in
The .NET Framework includes a command line compiler for C#.
! Compile Directly from a Command Prompt Window
>csc HelloDemoCS.cs >csc HelloDemoCS.cs
! Use /t to indicate target
>csc /t:exe HelloDemoCS.cs >csc /t:exe HelloDemoCS.cs
! Use /reference to reference assemblies
>csc /t:exe /reference:assemb1.dll HelloDemoCS.cs >csc /t:exe /reference:assemb1.dll HelloDemoCS.cs
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The .NET Framework includes a command line compiler for C#. The file name of the compiler is Csc.exe.
Compiling in C#
To compile the source code for the Hello World application presented in the
Hello World demonstration at the beginning of this module, type the following:
csc HelloDemoCS.cs
This syntax invokes the C# compiler. In this example, you only need to specify the name of the file to be compiled. The compiler generates the program executable, HelloDemoCS.exe.