
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdfModule 2: Introduction to a Managed Execution Environment |
13 |
|
|
|
|
Command Line Options
In C#, you can obtain the complete list of command line options by using the /? switch as follows:
csc /?
Common options include the /out switch, which specifies the name of the output file, and the /target switch, which specifies the target type. By default, the name of the output file is the name of the input file with an .exe extension. The default for the target type is an executable program.
The following example shows the use of both the /out and /t switches in C#:
csc /out:HelloDemoCS.exe /t:exe HelloDemoCS.cs
The /t switch is equivalent to the /target switch.
For more information about compiler options, see the .NET Framework SDK documentation.
Using the /reference Compilation Option
When referring to other assemblies, you must use the /reference compilation switch. The /reference compilation option allows the compiler to make information in the specified libraries available to the source that is currently being compiled.
The following example shows how to build an executable program from the command line by using the /reference compilation option.
csc /r:assemb1.dll,assemb2.dll /out:output.exe input.cs
The /r switch is equivalent to the /reference compilation switch.

14 |
Module 2: Introduction to a Managed Execution Environment |
The Process of Managed Execution
Topic Objective
To introduce fundamental concepts of compiling and executing code in a managed execution environment.
Lead-in
In the .NET Framework, the common language runtime provides the infrastructure for a managed execution environment.
Class
Class
LibrariesLibraries (MSIL and (MSIL and metadata)metadata)
EXE/DLL |
|
Source |
EXE/DLL |
CompilerCo piler |
|
(MSIL and |
Source |
|
(MSIL and |
|
Code |
metadata) |
|
Code |
metadata) |
|
|
Classl Loader
JITIT Compileril r withith optionalti l verificationrifi ti
Trusted, |
Managed |
Call to an |
|
pre-JITed |
uncompiled |
||
Native |
|||
code only |
method |
||
Code |
Executionecution
Securityec rity Checkshecks
Runtime Engine
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In the .NET Framework, the common language runtime provides the infrastructure for a managed execution environment. This topic introduces fundamental concepts of compiling and executing code in a managed execution environment and identifies new terminology.
Compiling Source Code
When you develop an application in the .NET Framework, you can write the source code in any programming language as long as the compiler that you use to compile the code targets the common language runtime. Compilation of the source code produces a managed module. The managed module is contained within a physical file also known as a portable executable (PE) file.
The file may contain the following items:
!Microsoft Intermediate Language (MSIL)
The compiler translates the source code into MSIL, a CPU-independent set of instructions that can be efficiently converted to native code.
!Type Metadata
This information fully describes types, members, and other references, and is used by the common language runtime at run time.
!A Set of Resources
For example, .bmp or .jpg files.
Module 2: Introduction to a Managed Execution Environment |
15 |
|
|
|
|
If the C# compiler’s target option is either exe or library, then the compiler produces a managed module that is an assembly. Assemblies are a fundamental part of programming with the .NET Framework. Assemblies are the fundamental units of sharing, deployment, security, and versioning in the common language runtime. The .NET common language runtime only executes MSIL code that is contained in an assembly.
If the C# compiler’s target option is module, then the compiler produces a managed module that is not an assembly, it does not contain a manifest and cannot be executed by the common language runtime. A managed module can be added to an assembly by the C# compiler, or by using the .NET’s Assembly Generation Tool, Al.exe.
Subsequent topics in this module cover MSIL, metadata, and assemblies in more detail.
Executing Code
When a user executes a managed application, the operating system loader loads the common language runtime, which then begins executing the module’s managed MSIL code. Because current host CPUs cannot execute the MSIL instructions directly, the common language runtime must first convert the MSIL instructions into native code.
The common language runtime does not convert all of the module’s MSIL code into CPU instructions at load time. Instead, it converts the instructions when functions are called. The MSIL is compiled only when needed. The component of the common language runtime that performs this function is called the just- in-time (JIT) compiler. JIT compilation conserves memory and saves time during application initialization.
For more information about the JIT compiler, see Just-In-Time Compilation in this module.
Application Domain
Operating systems and runtime environments typically provide some form of isolation between applications. This isolation is necessary to ensure that code running in one application cannot adversely affect other, unrelated applications.
Application domains provide a secure and versatile unit of processing that the common language runtime can use to provide isolation between applications. Application domains are typically created by runtime hosts, which are responsible for bootstrapping the common language runtime before an application is run.

16 |
Module 2: Introduction to a Managed Execution Environment |
Metadata
Topic Objective
To explain how metadata is used in the common language runtime.
Lead-in
Every compiler that targets the common language runtime is required to emit full metadata into every managed module.
!Declarative Information Emitted at Compile Time
!Included with All .NET Framework Files and Assemblies
!Metadata Allows the Runtime to:
#Load and locate code
#Enforce code security
#Generate native code at runtime
#Provide reflection
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Every compiler that targets the common language runtime is required to emit full metadata into every managed module. This topic explains how the common language runtime uses metadata.
Definition of Metadata
Metadata is a set of data tables, which fully describe every element that is defined in a module. This information can include data types and members with their declarations and implementations, and references to other types and members.
Metadata provides the common language runtime with all the information that is required for software component interaction. It replaces older technologies, such as Interface Definition Language (IDL) files, type libraries, and external registration. Metadata is always embedded in the .exe or .dll file containing the MSIL code. Therefore, it is impossible to separate metadata from the MSIL code.
Module 2: Introduction to a Managed Execution Environment |
17 |
|
|
|
|
Uses for Metadata
Metadata has many uses, but the following uses are most important:
!Locating and loading classes
Because metadata and MSIL are included in the same file, all type information in that file is available to the common language runtime at compile time. There is no need for header files because all types in a particular assembly are described by the assembly’s manifest.
!Enforcing security
The metadata may or may not contain the permissions required for the code to run. The security system uses permissions to prevent code from accessing resources that it does not have authority to access.
Other uses for metadata include:
!Resolving method calls.
!Setting up runtime context boundaries.
!Providing reflection capability.
For more information about metadata, see “Metadata and Self-Describing
Components” in the .NET Framework SDK documentation.
For more information about verification of type safety, see Module 4, “Deployment and Versioning,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C#™ .NET).
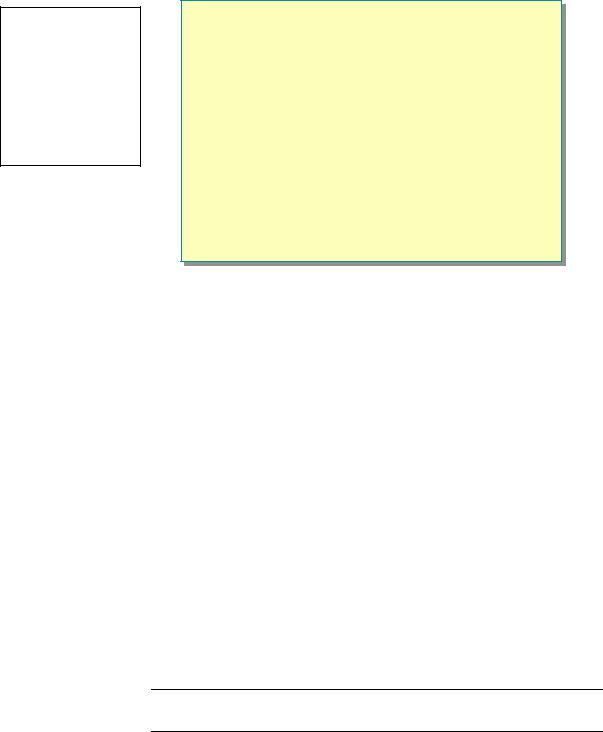
18 |
Module 2: Introduction to a Managed Execution Environment |
Microsoft Intermediate Language
Topic Objective
To introduce MSIL and JIT compilation.
Lead-in
Microsoft intermediate language, sometimes called managed code, is the set of instructions that the compiler produces as it compiles source code.
!Produced by Each Supported Language Compiler
!Converted to Native Language by the Common Language Runtime's JIT Compilers
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Microsoft intermediate language (MSIL), sometimes called managed code, is the set of instructions that the compiler produces as it compiles source code. This topic explains MSIL and the general process for converting MSIL to native code.
Compiled MSIL
Regardless of their logical arrangement, most assemblies contain code in the form of MSIL. MSIL is a CPU-independent machine language created by Microsoft in consultation with third-party compiler vendors. However, MSIL is a much higher-level language than most CPU machine languages.
MSIL contains instructions for many common operations, including instructions for creating and initializing objects, and for calling methods on objects. In addition, it includes instructions for arithmetic and logical operations, control flow, direct memory access, and exception handling.
Conversion to Native Code
Before MSIL code can be executed, it must be converted to CPU-specific or native code by a JIT compiler. The common language runtime provides an architecture-specific JIT compiler for each CPU architecture. These architecture-specific JIT compilers allow you to write managed code that can be JIT compiled and executed on any supported architecture.
Note Any managed code that calls platform-specific native APIs or libraries can only run on a specific operating system.
For more information about MSIL, see the .NET Framework SDK documentation.
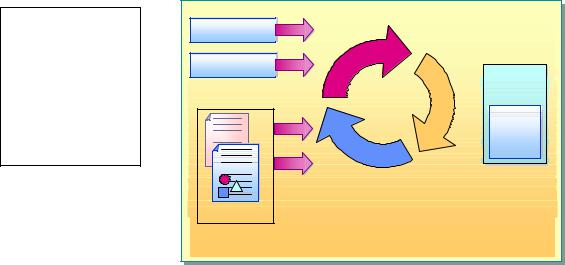
Module 2: Introduction to a Managed Execution Environment |
19 |
|
|
|
|
Assemblies
Topic Objective
This topic introduces the concept of an assembly and the role of the assembly manifest.
Lead-in
The common language runtime uses an assembly as the functional unit of sharing and reuse.
ManagedManagedModuleModule |
|
|
(MSIL(MSILandandMetadata)Metadata) |
|
|
ManagedManagedModuleModule |
|
|
(MSIL(MSILandandMetadata)Metadata) |
Assembly |
|
|
Assembly |
|
.html |
ManifestManifest |
|
|
||
.gif |
|
|
|
Multiple Managed |
|
|
Modules and |
|
Resource Files |
Resource Files |
|
Are Compiled to |
||
|
||
|
Produce an Assembly |
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The common language runtime uses an assembly as the functional unit of sharing and reuse.
Definition of an Assembly
An assembly is a unit of class deployment, analogous to a logical .dll. Each assembly consists of all the physical files that make up the functional unit: any managed modules, and resource or data files.
Conceptually, assemblies provide a way to consider a group of files as a single entity. You must use assemblies to build an application, but you can choose how to package those assemblies for deployment.
An assembly provides the common language runtime with the information that it needs to understand types and their implementations. As such, an assembly is used to locate and bind to referenced types at run time.
20 |
Module 2: Introduction to a Managed Execution Environment |
The Assembly Manifest
An assembly contains a block of data known as a manifest, which is a table in which each entry is the name of a file that is part of the assembly. The manifest includes the metadata that is needed to specify the version requirements, security identity, and the information that is used to define the scope of the assembly and resolve references to resources and classes. Because the metadata makes an assembly self-describing, the common language runtime has the information it requires for each assembly to execute.
All applications that are executed by the common language runtime must be composed of an assembly or assemblies. All files that make up an assembly must be listed in the assembly’s manifest. The manifest can be stored in singlefile assemblies or multi-file assemblies:
!Single-file assemblies
When an assembly has only one associated file, the manifest is integrated into a single PE file.
!Multi-file assemblies
When an assembly has more than one associated file, the manifest can be a standalone file, or it can be incorporated into one of the PE files in the assembly.
For more information about assemblies, see “Assemblies” in the .NET Framework SDK documentation.
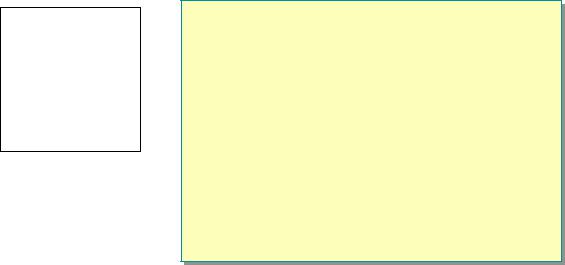
Module 2: Introduction to a Managed Execution Environment |
21 |
|
|
|
|
Common Language Runtime Tools
Topic Objective
This topic describes how the MSIL Assembler and MSIL Disassembler work.
Lead-in
The common language runtime provides two tools that you can use together to test and debug MSIL code.
!Runtime Utilities for Working with MSIL
#MSIL Assembler (ilasm.exe) produces a final executable binary
#MSIL Disassembler (ildasm.exe) inspects metadata and code of a managed binary
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The common language runtime provides two tools that you can use to test and debug MSIL code.
!The MSIL Assembler
The MSIL Assembler (Ilasm.exe) takes MSIL as text input and generates a PE file, which contains the binary representation of the MSIL code and required metadata. The basic syntax is as follows:
ilasm [options] filename [options]
!The MSIL Disassembler
You can use the MSIL Disassembler (Ildasm.exe) to examine the metadata and disassembled code of any managed module. You will use this tool to examine MSIL code in Lab 2, Building a Simple .NET Application.
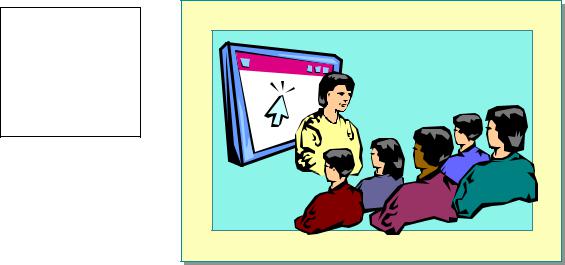
22 |
Module 2: Introduction to a Managed Execution Environment |
Demonstration: Using the MSIL Disassembler
Topic Objective
To demonstrate how the MSIL Disassembler works.
Lead-in
This demonstration shows how to use the MSIL Disassembler to view an assembly’s metadata.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
This demonstration shows how to use the MSIL Disassembler to view an assembly’s metadata.
Viewing Assembly Metadata by Using the MSIL Disassembler
In the following procedures, you will see how to use the MSIL Disassembler (Ildasm.exe) to view the contents of the HelloDemoCS.exe assembly.
!To run the MSIL Disassembler on the HelloDemoCS.exe assembly
•At a Visual Studio .NET Command Prompt window, change the directory to <install folder>\Democode\Mod02 where the sample file HelloDemoCS.exe has been copied, and type the following command:
ildasm HelloDemoCS.exe