
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf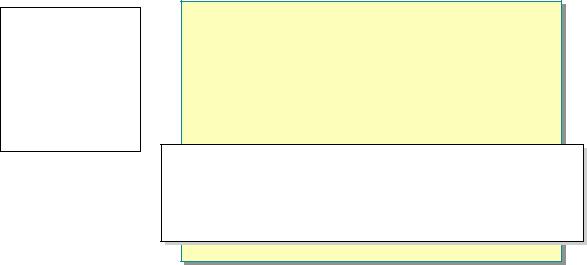
Module 5: Common Type System |
19 |
|
|
|
|
Enumerations
Topic Objective
To describe how to create enumerations, which allow developers to specify and represent simple types.
Lead-in
It is common for a variable to take on only one of a small number of values.
!.NET Framework Enumerations
#Inherit from System.Enum
#Use the enum keyword
!Bit Flags
enum SeatPreference : ushort |
|
enum SeatPreference : ushort |
|
{{ |
|
Window, //Assigned value 0 |
|
Window, //Assigned value 0 |
|
Center, //Assigned value 1 |
|
Center, //Assigned value 1 |
|
Aisle |
//Assigned value 2 |
Aisle |
//Assigned value 2 |
}} |
|
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In programming, it is common for a variable to take on only one of a small number of values. For example, the variable for an airline passenger’s seating preference has only three values: Window, Center, or Aisle. It is much easier and safer for a developer to reference such values with text, rather than numbers. The .NET Framework common language runtime supports enumerations, which allow a developer to specify and represent these simple types.
.NET Framework Enumerations
In the .NET Framework, enumerations inherit from the class System.Enum, which inherits from System.ValueType. The following example shows how to create an enumeration:
enum SeatPreference : ushort
{
Window, //Assigned value 0
Center, |
//Assigned |
value |
1 |
Aisle |
//Assigned |
value |
2 |
}
In the preceding example, the base type of the enumeration is defined as ushort. You can use any simple type for a base type, except System.Char. An enumeration will be of type int, unless otherwise specified.
The literals are specified in a comma-delimited list. Unless you specify otherwise, the first literal is assigned a value of 0, the second a value of 1, and so on.

20 Module 5: Common Type System
To use enumeration literals, you must fully specify the literal name as in the following example:
SeatPreference sp;
//Assign enumerated window type seat to sp variable sp = SeatPreference.Window; Console.WriteLine(sp.GetHashCode()); Console.WriteLine(sp.ToString());
This code generates the following output:
0 Window
The GetHashCode method will return the literal value. By using the ToString method, you can obtain the human-readable string name of the literal from a variable. This feature is useful when you need to print or save a human-readable form of the enumeration’s literals.
Also, you can use the Parse method to obtain an enumerated value from a string. This feature is useful when receiving input in a text form that requires conversion to the appropriate enumerated value. The following example shows how to convert the string “center” into an enumerated value for the
SeatPreference enumeration.
sp = (SeatPreference) System.Enum.Parse(typeof(SeatPreference), “Center”);
Module 5: Common Type System |
21 |
|
|
|
|
Bit Flags
Enumerations in the .NET Framework are also useful for storing bit flags that often involve bitwise operations, such as the bitwise AND operator (&), and the bitwise OR operator (|).
Use the Flags attribute to create an enumeration of bit flags. The following example refers to a computer-controlled weather display that uses icons to represent weather conditions. The enumeration would allow you to combine the icons in various ways to represent different conditions:
[Flags] enum WeatherDisplay : ushort
{
Sunny = 1,
Cloudy = 2,
Rain = 4,
Snow = 8,
Fog = 16
}
WeatherDisplay wd;
//Assign both Sunny and Cloudy attributes //to create partly sunny forecast
wd = WeatherDisplay.Sunny | WeatherDisplay.Cloudy; Console.WriteLine(wd.ToString());
wd = (WeatherDisplay)System.Enum.Parse(typeof(WeatherDisplay), "Rain, Snow");
Console.WriteLine(wd.GetHashCode());
This code generates the following output:
Sunny, Cloudy 12
When using the Flags attribute, you must specify the values for the literals manually.

22 Module 5: Common Type System
Interfaces
Topic Objective
To describe the uses of interfaces.
Lead-in
An interface is a contractual description of a set of related methods and properties.
!An Interface Is a Contractual Description of Methods and Properties
!An Interface Has No Implementation
!Use Casting in Client Code to Use an Interface
interface ICDPlayer interface ICDPlayer
{{ void Play(short playTrackNum); void Play(short playTrackNum); void Pause();
void Pause();
void Skip(short numTracks); void Skip(short numTracks); short CurrentTrack
short CurrentTrack
{{ get; get; set; set;
}}
}}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
An interface is a contractual description of a set of related methods and properties. An interface has a name, and it has methods and properties. For example, an interface named ICDPlayer may have methods, such as Play, Pause, and Skip. It may also have properties, such as CurrentTrack and
Time.
The following example shows how to define an interface called ICDPlayer that has the methods Play, Pause, and Skip, and has the property CurrentTrack:
interface ICDPlayer
{
void Play(short playTrackNum); void Pause();
void Skip(short numTracks); short CurrentTrack
{
get;
set;
}
}
An interface has no implementation. Any class can inherit from any interface. To inherit from an interface, a class must implement all methods, properties, and events on that interface. Thus an interface serves as a contract specifying to any user of the class that the class has implemented all methods properties, and events defined in the interface.
Module 5: Common Type System |
23 |
|
|
|
|
The following example shows how to implement the ICDPlayer interface in a class called Device.
public class Device : ICDPlayer
{
// Internal property values protected string deviceName; protected short currentTrack;
//Constructor public Device()
{
deviceName = "Default"; currentTrack = 1;
}
//Properties
public string DeviceName
{
get
{
return deviceName;
}
set
{
deviceName = value;
}
}
public short CurrentTrack
{
get
{
return currentTrack;
}
set
{
currentTrack = value;
}
}
//Methods
public void Play(short playTrackNum)
{
Console.WriteLine("Now Playing Track: {0}", playTrackNum);
currentTrack = playTrackNum;
}
public void Pause()
{
Console.WriteLine("Now Paused");
}
public void Skip(short numTracks)
{
Console.WriteLine("Skipped {0} Tracks",numTracks);
}
}

24 Module 5: Common Type System
In the preceding example, all of the methods and properties of ICDPlayer were implemented. A class may implement additional properties and methods, such as the DeviceName property in the Device class.
Client code uses an interface by casting to the interface name. The following example shows how a client can create an instance of the Device class and then use the ICDPlayer interface:
public class MainClass
{
public static void Main()
{
Device Device1 = new Device(); ICDPlayer CD1 = (ICDPlayer) Device1;
//Call Play method on ICDPlayer interface CD1.Play(1);
//Get CurrentTrack property of ICDPlayer interface Console.WriteLine("Current Track = {0}",
CD1.CurrentTrack);
//Get DeviceName property of Device object Console.WriteLine("Device Name = {0}",
Device1.DeviceName);
}
}
In the preceding example, the Device1 variable also could have been used to access methods and properties of the ICDPlayer interface.
For more information about how to separate interface methods and properties from class methods and properties, see Module 6, “Working with Types,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C# .NET).
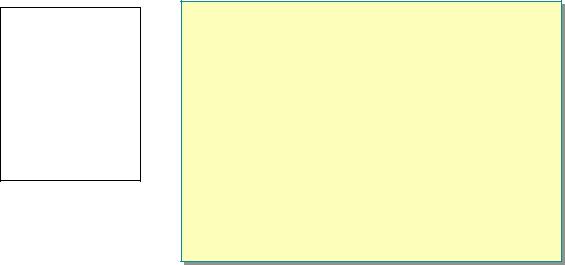
Module 5: Common Type System |
25 |
|
|
|
|
" Object-Oriented Characteristics
Topic Objective
To provide an overview of the topics covered in this section.
Lead-in
In this section, you will learn how the object-oriented characteristics of the .NET Framework common language runtime are supported.
!Abstraction
!Encapsulation
!Inheritance
!Polymorphism
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In this section, you will learn how the object-oriented characteristics of the
.NET Framework common language runtime are supported.
Everything in the common language runtime is an object that encompasses fields, methods, properties, and events. Object-oriented characteristics, such as abstraction, encapsulation, inheritance, and polymorphism, make it easier to work with code in the .NET Framework.
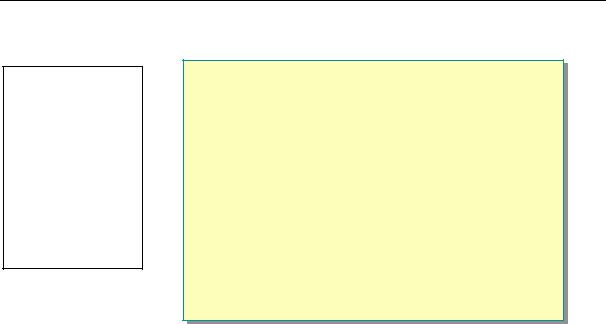
26 Module 5: Common Type System
Abstraction
Topic Objective
To describe the use and advantages of abstraction in object-oriented programming.
Lead-in
In object-oriented programming, the term abstraction refers to the process of reducing an object to its essence so that only essential elements are represented.
!Abstraction Works from the Specific to the General
!Grouping Elements Makes It Easier to Work with Complex Data Types
!Abstraction Is Supported Through Classes
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In object-oriented programming, the term abstraction refers to the process of reducing an object to its essence so that only essential elements are represented. For programmers, abstraction means working from the specific to the general.
An example of abstraction would be a phone number, such as (206) 555-1212. At the specific level, this phone number is a sequence of numbers: 2065551212. However, most people do not memorize phone numbers at this specific level. Instead they memorize such information by grouping the numbers into meaningful chunks. In this example, the phone number becomes an area code (206), prefix (555), and number (1212). This abstraction makes it easier for a person to remember the number. The number is reduced to three chunks to memorize, instead of 10 individual numbers.
Abstraction is a powerful process that allows people to group and communicate information in simpler and more meaningful ways. In the Common Type System and other object-oriented systems, the fundamental unit of abstraction is the class. Class types allow you to group different types of information, and they provide the functionality to help you operate on that information. From a design perspective, abstraction allows you to treat information and functionality as complete functional units, rather than as disparate pieces of information and operations.
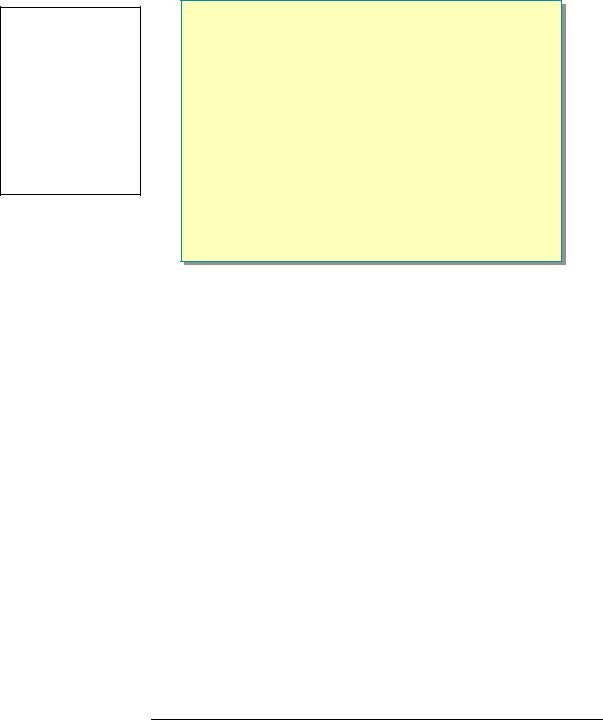
Module 5: Common Type System |
27 |
|
|
|
|
Encapsulation
Topic Objective
To explain the process of encapsulation in objectoriented programming.
Lead-in
Encapsulation, or information hiding, is the process of packaging attributes and functionality to prevent other objects from manipulating data or procedures directly.
!Encapsulation Is the Process of Hiding Internal Details of a Class
!Encapsulation Keywords
#public
#protected
#internal
#private
!Type-Level Accessibility
!Nested Classes
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In object-oriented programming, encapsulation, or information hiding, is the process of packaging attributes and functionality to prevent other objects from manipulating data or procedures directly. Encapsulation also allows the object that is requesting service to ignore the details of how the service is provided. By hiding the internal unnecessary data and functionality of a class from other classes, encapsulation provides greater flexibility in the design of classes. For example, when a sorting class performs sorts on data items, the internal data structure of the class will probably store the data items, such as a linked list. The class will also have internal functions that operate on the linked list.
If clients that use the sorting class have direct access to the linked list, they will incorporate code that relies on the linked list. In fact, if no accessor functions are written for the linked list, the clients must use the linked list to store or retrieve data items.
If the sorting class changes in the future, it may change the linked list to an array for optimization, and the clients will stop functioning correctly. At that time, the clients would require rewriting to use the array syntax. If the sorting class hid the internals of its implementation and provided accessor functions that mapped to the internal data structure, this problem could be avoided.
C# provides a set of access modifiers that vary the degree of accessibility to members of any type. The following table lists all of the access modifiers supported in C#.
Access
modifier Description
public Makes a member available to all other classes in all assemblies protected Makes a member available only to classes that inherit from this class internal Makes a member available only to other classes in the same assembly private Makes a member available only to the same class

28 Module 5: Common Type System
If no access modifier is specified, a default modifier is applied. The following table shows which modifiers are applied to different types by default.
Members of |
Default member accessibility |
enum |
public |
class |
private |
interface |
public |
struct |
private |
Type-Level Accessibility
You can specify accessibility at the type level and at the member level. Access modifiers can be applied to enumerations, classes, interfaces, and structs. However, for top-level types, internal and public are the only allowed modifiers. You cannot create a private class or a protected interface at the top level in a namespace.
The access modifier applied at the type level will supercede member-level access modifiers if the type-level access modifier is more restrictive. For example, an internal class can have a public method, but the internal modifier will make the public method internal as well.
The following example shows the effect of applying access modifiers at the class level and the member level:
public class COuter1
{
public static short MyPublicShort = 0; internal static short MyInternalShort = 0; private static short MyPrivateShort = 0;
}
public class MainClass
{
public static void Main()
{
COuter1.MyPublicShort = 1;
//Success because publicly available COuter1.MyInternalShort = 2; //Success because in same assembly COuter1.MyPrivateShort = 3;
//Failure because private only available to COuter1 class
}
}