
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf
Module 3: Working with Components |
1 |
|
|
|
|
Overview
Topic Objective
To provide an overview of the module topics and objectives.
Lead-in
In this module, you will learn how to create a small client/server application.
!An Introduction to Key .NET Framework Development Technologies
!Creating a Simple .NET Framework Component
!Creating a Simple Console Client
!Creating an ASP.NET Client
*****************************ILLEGAL FOR NON-TRAINER USE******************************
For Your Information
Tell students that the code examples used in this module are from the tutorial, “Introduction to Developing with the .NET Framework.” This tutorial is found in the
.NET Framework Software Development Kit (SDK).
In this module, you will learn how to create a small client/server application in C#. The steps that are necessary to construct, compile, and run each program are covered in detail. You will also learn how to build the client application by using the Windows Forms library and an ASP.NET page.
As in Module 2, “Introduction to a Managed Execution Environment” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C#™ .NET), the content of this module is based on the “Introduction to Developing with the .NET Framework” tutorial in the .NET Framework Software Development Kit (SDK).
After completing this module, you will be able to:
!Create a simple Microsoft® .NET Framework component in C#.
!Implement structured exception handling.
!Create a simple .NET Framework console application that calls a component.
!Create a .NET Framework client application by using the Windows Forms library.
!Create an ASP.NET page that uses the previously developed .NET Framework component to create an ASP.NET application.

2Module 3: Working with Components
An Introduction to Key .NET Framework Development Technologies
Topic Objective
To introduce the technologies that are used to develop .NET Framework applications.
Lead-in
To develop applications in the .NET Framework, you should be familiar with Windows Forms, ASP.NET Web Forms, and XML Web services.
!Windows Forms
!Web Forms
!XML Web Services
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To develop applications in the .NET Framework, you should be familiar with the following technologies: Windows Forms, ASP.NET Web Forms, and XML Web services. Your choice of technology, or technologies, depends on the type of software solution that you wish to implement. This topic briefly introduces the technologies and provides references to additional information.
Windows Forms
Windows Forms are used to develop applications in which the client computer handles most of the application processing. Classic Microsoft Win32® desktop applications, such as drawing and graphics applications, data-entry systems, point-of-sale systems, and games, are well suited to Window Forms. All of these applications rely on the power of the desktop computer for processing and for high-performance content display.
The System.Windows.Forms namespace contains the classes used to create
Windows Forms.
Module 3: Working with Components |
3 |
|
|
|
|
Web Forms
ASP.NET Web Forms are used to create applications in which the primary user interface is a browser. Obviously, you can use Web Forms to create applications that are available on the World Wide Web, such as e-commerce applications, but you will also find them helpful for creating other types of applications, such as intranet applications, which users can run by using only their browser, which is already installed on their computers.
Because Web Forms applications are platform-independent, users can interact with your application regardless of the type of browser or computer that they are using. You can also optimize Web Forms applications to use features that are built into the most recent browsers, such as Dynamic Hypertext Markup Language (DHTML) and HTML 4.0, which enhance performance and responsiveness.
The System.Web namespace contains the classes used to create Web Forms.
For more information about Windows Forms and ASP.NET Web Forms, see “Windows Forms and Web Forms Recommendations” in the .NET Framework SDK documentation.
XML Web Services
An XML Web Service is a programmable entity that resides on a Web server and is exposed through standard Internet protocols. In many respects, the programming model for creating and using XML Web services is similar to the programming model for creating and using COM-based components.
However, unlike COM, the XML Web services programming model is based on simple, open standards that are broadly supported. Instead of using binary communication methods between applications, XML Web services use communication based on the SOAP protocol to transport XML messages between applications. In an environment of integrated, programmable XML Web services, XML is the universal language for communication of raw data and information that can be understood and acted upon.
For more information about XML Web services, see Module 13, “Remoting and XML Web Services” in Course 2349B, Programming with the Microsoft
.NET Framework (Microsoft Visual C# .NET).

4Module 3: Working with Components
" Creating a Simple .NET Framework Component
Topic Objective
To provide an overview of the section topics.
Lead-in
In this section, you will learn how to use C# to create a simple component.
!Using Namespaces and Declaring the Class
!Creating the Class Implementation
!Implementing Structured Exception Handling
!Creating a Property
!Compiling the Component
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Delivery Tip
If you prefer, you can use Notepad to demonstrate the code in this section, instead of discussing the content of each slide.
In this section, you will learn how to create a simple component using C#. The component provides a wrapper for an array of strings and includes a GetString method that takes an integer and returns a string. The component includes a read-only Count property that contains the number of elements in the string array, which is used to iterate over all of the array members. The GetString method also demonstrates the use of structured exception handling.
In subsequent sections of this module, you will learn how clients use the GetString method and the Count property to determine and display the output from the component’s string array.
The simple string component that is described in this section shows the basic approach to creating reusable classes in C#.

Module 3: Working with Components |
5 |
|
|
|
|
Using Namespaces and Declaring the Class
Topic Objective
To explain how to create a new namespace that encapsulates classes.
Lead-in
To create a new namespace that encapsulates the classes that you will be creating, you use the namespace statement.
! Create a New Namespace
using System; using System;
namespace CompCS {...} namespace CompCS {...}
! Declare the Class
public class StringComponent {...} public class StringComponent {...}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To create a new namespace that encapsulates the classes that you will be creating, you use the namespace statement, as in the following example:
using System;
namespace CompCS {...}
The following statement denotes that instances of the StringComponent class will now be created by the runtime and managed in the garbage-collected heap.
public class StringComponent {...}
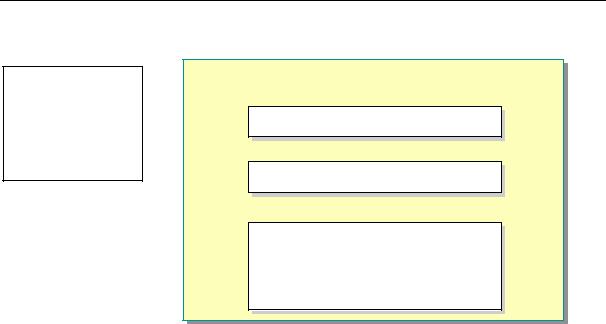
6Module 3: Working with Components
Creating the Class Implementation
Topic Objective
To explain how to create the class implementation.
Lead-in
The component provides a wrapper for an array of strings.
! Declare a Private Field of Type Array of String Elements
private string[] stringSet; private string[] stringSet;
! Create a Public Default Constructor
public StringComponent() {...} public StringComponent() {...}
! Assign the stringSet Field to an Array of Strings
stringSet = new string[] { stringSet = new string[] {
"C# String 0",
"C# String 0",
"C# String 1",
"C# String 1",
...
};
...
};
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The component provides a wrapper for an array of strings. You can declare a private field of type array of string elements, as in the following example:
private string[ ] stringSet;
The following example shows how to create a public default constructor, which executes each time a new instance of the class is created, and how to assign the stringSet field to an array of strings:
public StringComponent() { stringSet = new string[ ] {
"C# String 0",
"C# String 1",
"C# String 2",
"C# String 3"
};
}
In the preceding example, you can see that the constructor has the same name as the class and does not have a return type.

Module 3: Working with Components |
7 |
|
|
|
|
Implementing Structured Exception Handling
Topic Objective
To explain how to implement structured exception handling.
Lead-in
The component uses the GetString method to return the strings in the stringSet array.
! Implement the GetString Method
public string GetString(int index) {...} public string GetString(int index) {...}
!Create and Throw a New Object of Type IndexOutOfRangeException
if((index < 0) || (index >= stringSet.Length)) { if((index < 0) || (index >= stringSet.Length)) { throw new IndexOutOfRangeException();
}}
throw new IndexOutOfRangeException();
return stringSet[index]; return stringSet[index];
!Exceptions Caught by the Caller Using a try/catch/finally Statement
!Structured Exception Handling Replaces HRESULT-Based Error Handling in COM
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Programs must be able to uniformly handle errors and exceptions that occur during execution. The common language runtime helps you design errortolerant software by providing a platform for notifying programs of errors in a uniform manner. All .NET Framework methods indicate failure by throwing exceptions.
Traditionally, a language’s error-handling model relied on either the language’s unique way of detecting errors and locating handlers for them, or on the errorhandling mechanism that is provided by the operating system. The runtime implements exception handling with the following features:
!It handles exceptions without regard for the language that generates the exception or the language that will be called upon to handles the exception.
!It does not require any particular language syntax for handling exceptions, but allows each language to define its own syntax.
!It allows exceptions to be thrown across process boundaries and machine boundaries.
Exceptions offer several advantages over other methods of error notification. Failures do not go unnoticed. Invalid values do not continue to propagate through the system. You do not have to check return codes. Exception-handling code can be easily added to increase program reliability. Finally, the runtime’s exception handling is faster than Windows-based C++ error handling.

8Module 3: Working with Components
The component uses the GetString method to return the strings in the stringSet array. You can use a throw statement to implement structured exception handling within the GetString method, as in the following example:
public string GetString(int index) {
if ((index < 0) || (index >= stringSet.Length)) { throw new IndexOutOfRangeException();
}
return stringSet[index];
}
In the preceding example, the program evaluates whether the integer that is passed as an argument to GetString is a valid index for the stringSet array. If the index is invalid, the following statement creates and throws a new object of type IndexOutOfRangeException:
throw new IndexOutOfRangeException();
If the index is valid, the program returns the string element of the stringSet array at that particular index.
Exceptions may be caught by the caller by using a try/catch/finally statement. Place the sections of code that might throw exceptions in a try block and place code that handles exceptions in a catch block. You can also write a finally block that always runs regardless of how the try block runs. The finally block is useful for cleaning up resources after a try block. For example, in C#:
try {
//code that might throw exceptions
}
catch(Exception e) {
//place code that handles exceptions
}
finally {
//place code that runs after try or catch runs
}
In general, it is good programming practice to catch a specific type of exception rather than using the preceding general catch statement that catches any exception.
Structured exception handling replaces the HRESULT-based error-handling system that is used in COM. All .NET Framework exception-handling classes, such as IndexOutOfRangeException and user-defined exceptions, must derive from System.Exception.
For more information about exception handling, see “Handling and Throwing Exceptions” in the .NET Framework SDK documentation.
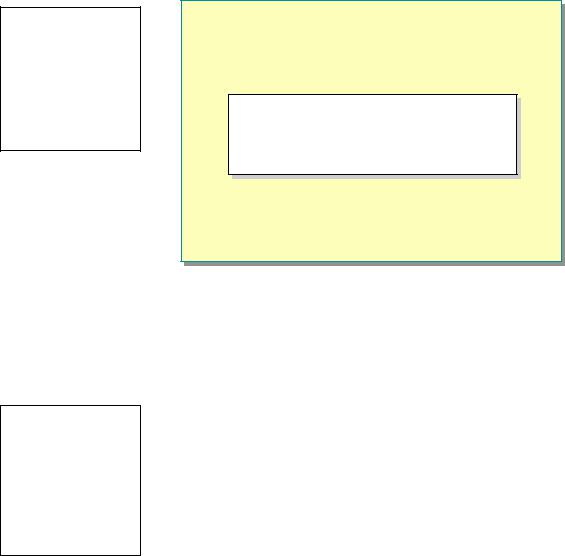
Module 3: Working with Components |
9 |
|
|
|
|
Creating a Property
Topic Objective
To explain how to create a property.
Lead-in
To provide the number of string elements in the stringSet array in C#, you create a read-only property called Count.
!Create a Read-Only Count Property to Get the Number of String Elements in the stringSet Array
public int Count { public int Count {
}}
get { return stringSet.Length; } get { return stringSet.Length; }
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To provide the number of string elements in the stringSet array, you create a read-only property called Count, as in the following example:
public int Count {
get { return stringSet.Length; }
}
For Your Information
Point out that the GetString method and Count property may be used by a client to iterate over the component’s string elements. This information will help students when they create the clients in subsequent labs.
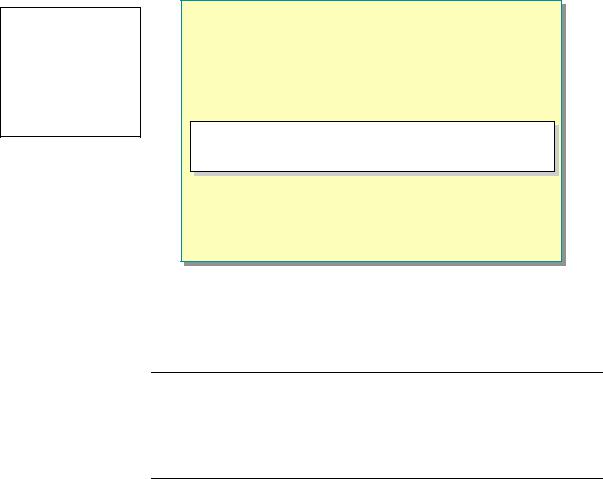
10 |
Module 3: Working with Components |
Compiling the Component
Topic Objective
To explain how to compile the source code for a component.
Lead-in
To compile the source code, first you must save the source file.
!Use the /target:library Switch to Create a DLL
#Otherwise, an executable with a .dll file extension is created instead of a DLL library
csc /out:CompCS.dll /target:library CompCS.cs csc /out:CompCS.dll /target:library CompCS.cs
*****************************ILLEGAL FOR NON-TRAINER USE******************************
To compile the source code for the C# component, first you must save the source file, and then enter the following command from a command prompt window:
Important To use Microsoft Visual Studio® .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click Start, All Programs, Microsoft Visual Studio .NET,
Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
csc /out:CompCS.dll /target:library CompCS.cs
This command directs the compiler to produce the file CompCS.dll. The /target:library switch is required to actually create a DLL library, instead of an executable.