
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf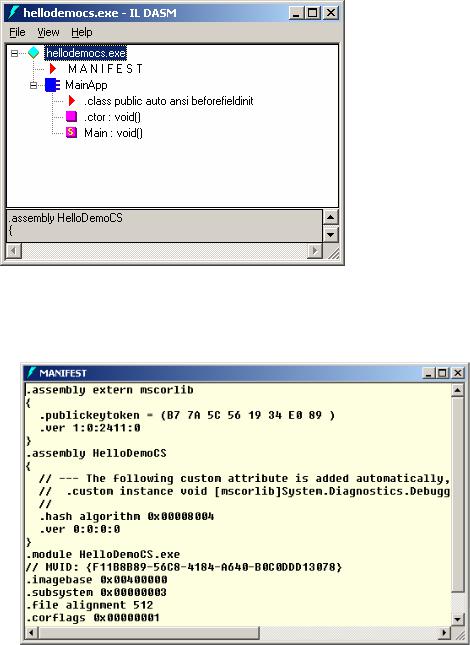
Module 2: Introduction to a Managed Execution Environment |
23 |
|
|
|
|
After you expand the MainApp icon, the MSIL Disassembler graphical user interface (GUI) displays information about the file HelloDemoCS.exe, as shown in the following illustration:
! To display the contents of the manifest
1.Double-click MANIFEST.
The MANIFEST window appears, as follows:
2. Close the Manifest window, and then close ILDASM.
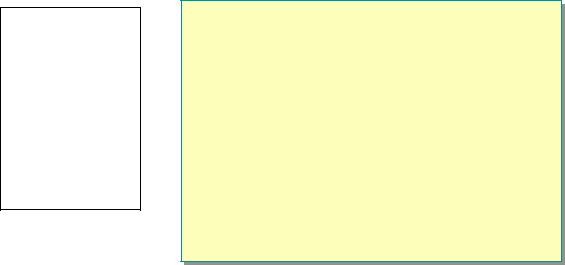
24 |
Module 2: Introduction to a Managed Execution Environment |
Just-In-Time Compilation
Topic Objective
To describe JIT compilation and introduce JIT compiler options.
Lead-in
MSIL code must be converted into native code before it can execute. Because an intermediate step is involved, the common language runtime optimizes the compilation process for efficiency.
!Process for Code Execution
#MSIL converted to native code as needed
#Resulting native code stored for subsequent calls
#JIT compiler supplies the CPU-specific conversion
*****************************ILLEGAL FOR NON-TRAINER USE******************************
As previously stated in this module, MSIL code must be converted into native code before it can execute. Because an intermediate step is involved, the common language runtime optimizes the compilation process for efficiency.
The Code Execution Process
The common language runtime compiles MSIL as needed. This just-in-time, or
JIT, compiling saves time and memory. The basic process is as follows:
1.When the common language runtime loads a class type, it attaches stub code to each method.
2.For subsequent method calls, the stub directs program execution to the common language runtime component that is responsible for compiling a method’s MSIL into native code. This component of the common language runtime is frequently referred to as the JIT compiler.
3.The JIT compiler compiles the MSIL and the method’s stub is substituted with the address of the compiled code.
Future calls to that method will not involve the JIT compiler because the compiled native code will simply execute.

Module 2: Introduction to a Managed Execution Environment |
25 |
|
|
|
|
Application Domains
Topic Objective
To describe how application domains provide application isolation.
Lead-in
Historically, process boundaries have been used to isolate applications running on the same computer.
!Historically, Process Boundaries Used to Isolate Applications
!In the Common Language Runtime, Application Domains Provide Isolation Between Applications
#The ability to verify code as type-safe enables isolation at a much lower performance cost
#Several application domains can run in a single process
!Faults in One Application Cannot Affect Other Applications
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Historically, process boundaries have been used to isolate applications running on the same computer. Each application is loaded into a separate process, which isolates the application from other applications running on the same computer.
The applications are isolated because memory addresses are process-relative; a memory pointer passed from one process to another cannot be used in any meaningful way in the target process. In addition, you cannot make direct calls between two processes. Instead, you must use proxies, which provide a level of indirection.
Managed code must be passed through a verification process before it can be run (unless the administrator has granted permission to skip the verification). The verification process determines whether the code can attempt to access invalid memory addresses or perform some other action that could cause the process in which it is running to fail to operate properly.
Code that passes the verification test is said to be type-safe. The ability to verify code as type-safe enables the common language runtime to provide as great a level of isolation as the process boundary, at a much lower performance cost.
Application domains provide a secure and versatile unit of processing that the common language runtime can use to provide isolation between applications. You can run several application domains in a single process with the same level of isolation that would exist in separate processes, but without incurring the additional overhead of making cross-process calls or switching between processes. The ability to run multiple applications within a single process dramatically increases server scalability.

26 |
Module 2: Introduction to a Managed Execution Environment |
Isolating applications is also important for application security. For example, you can run controls from several Web applications in a single browser process in such a way that the controls cannot access each other's data and resources.
The isolation provided by application domains has the following benefits:
!Faults in one application cannot affect other applications. Because type-safe code cannot cause memory faults, using application domains ensures that code running in one domain cannot affect other applications in the process.
!Individual applications can be stopped without stopping the entire process. Using application domains enables you to unload the code running in a single application.
Note You cannot unload individual assemblies or types. Only a complete domain can be unloaded.
Code running in one application cannot directly access code or resources from another application. The common language runtime enforces this isolation by preventing direct calls between objects in different application domains.
Objects that pass between domains are either copied or accessed by proxy. If the object is copied, the call to the object is local. That is, both the caller and the object being referenced are in the same application domain. If the object is accessed through a proxy, the call to the object is remote. In this case, the caller and the object being referenced are in different application domains. Crossdomain calls use the same remote call infrastructure as calls between two processes or between two computers.
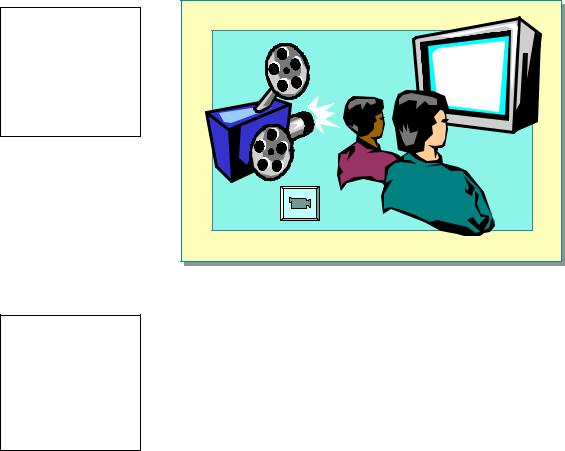
Module 2: Introduction to a Managed Execution Environment |
27 |
|
|
|
|
Multimedia: Application Loading and Single-File Assembly
Execution
Topic Objective
To describe how assembly code is executed.
Lead-in
In this demonstration, you will see how a single-file private assembly is loaded and executed.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Delivery Tip
To start the animation, click the button in the lower-left corner of the slide. The animation plays automatically. To pause or rewind the animation, click the controls in the lower-left of the screen.
Applications that are implemented by using MSIL code require the common language runtime to be installed on the user’s computer in order to run. The common language runtime manages the execution of code. For example, when an application that is implemented as a single-file private assembly is run, the following tasks are performed:
!The Microsoft Windows® loader loads the PE file.
The PE file contains a call to a function found in the file MSCorEE.dll.
!Windows loads the file MSCorEE.dll and transfers control to it to initialize the common language runtime.
!The common language runtime parses the metadata of the application assembly, and then the JIT compiler compiles the code.
!The common language runtime then locates the PE file’s managed entrypoint (the Main method in the case of a C# program), and transfers control to this entry point.
If the application calls a private assembly:
!The common language runtime uses probing to locate the referenced assembly, beginning in the application’s root directory and then traversing the subfolders until the assembly is located.
•If the assembly is not found, a TypeLoadException error occurs.
•If the assembly is found, it is loaded by the common language runtime.
The common language runtime loader parses the manifest in the referenced assembly. The JIT compiler then compiles the required code in the assembly and passes control to the called function.
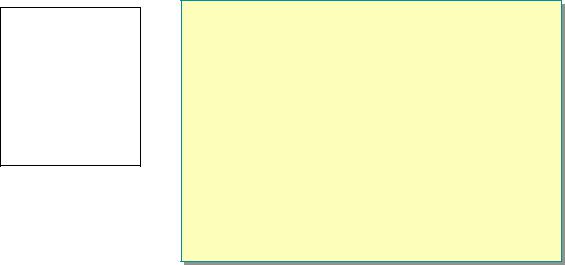
28 |
Module 2: Introduction to a Managed Execution Environment |
Garbage Collection
Topic Objective
To introduce memory and resource management in the .NET Framework.
Lead-in
Every program uses resources, such as files, screen space, network connections, and database resources.
!Garbage Collection Provides Automatic Object Memory Management in the .NET Framework
!You No Longer Need to Track and Free Object Memory
*****************************ILLEGAL FOR NON-TRAINER USE******************************
This topic introduces memory management in the .NET Framework.
For more information about memory and resource management, see Module 9, “Memory and Resource Management,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C# .NET).
Current Memory Management Model
When you create an object programmatically, you generally follow these steps:
1.Allocate memory for the object
2.Initialize the memory
3.Use the object
4.Perform cleanup on the object
5.Free the object’s memory
If you forget to free memory when it is no longer required or try to use memory after it has been freed, you can generate programming errors. The tracking and correction of such errors are complicated tasks because the consequences of the errors are unpredictable.
Memory Management in the .NET Framework
The common language runtime uses a heap called the managed heap to allocate memory for all objects. This managed heap is similar to a C-Runtime heap, however you never free objects from the managed heap. In the common language runtime, garbage collection is used to manage memory deallocation. The garbage collection process frees objects when they are no longer needed by the application.
For more information about garbage collection, see Module 9, “Memory and Resource Management,” in Course 2349B, Programming with the Microsoft
.NET Framework (Microsoft Visual C# .NET).

Module 2: Introduction to a Managed Execution Environment |
29 |
|
|
|
|
Lab 2: Building a Simple .NET Application
Topic Objective
To introduce the lab.
Lead-in
In this lab, you will learn how to write, compile, and run a simple application in C# and how to use the MSIL Disassembler to examine an assembly.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Objectives
After completing this lab, you will be able to:
!Write, compile, and run a simple application in C#.
!Use the MSIL Disassembler to examine an assembly.
Lab Setup
Only solution files are associated with this lab. The solution files for this lab are in the folder <install folder>\Labs\Lab02\Solution.
Estimated time to complete this lab: 20 minutes
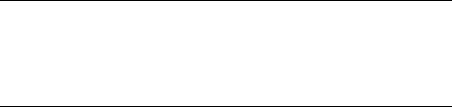
30 |
Module 2: Introduction to a Managed Execution Environment |
Exercise 1
Creating the Program in C#
In this exercise, you will create the source code for a small console application that takes user input and writes a string to the console by using C#. The lab uses the classic Hello World application to allow you to focus on basic concepts in a managed execution environment.
To help you concentrate on the syntactical aspects of this lab, you will use Notepad to create and edit the source files. From a command prompt window, you will then compile the application and test the resulting executable program.
!Write the source code
1.Open Notepad and create a class in C# called MainApp.
2.Import the System namespace.
3.Define the program entry point.
The entry point takes no arguments and does not return a value.
4.Create methods that accomplish the following:
a.Print the following text to the console: “Type your name and press Enter”.
b.Read in the resulting user input.
c.Print the text “Hello” and append to the text the value that was read in.
5.Save the file as HelloLabCS.cs in the <install folder>\Labs\Lab02 folder.
!Build and test the program
Important To use Microsoft Visual Studio .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click Start, All Programs, Microsoft Visual Studio .NET,
Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
1.From a Visual Studio .NET Command Prompt window, type the syntax to build an executable program from HelloLabCS.cs.
2.Run the resulting executable program.
Your C# program should generate the following output:
Type your name and press Enter
When you press ENTER, the program outputs the text “Hello ” and whatever text you typed as input.
Module 2: Introduction to a Managed Execution Environment |
31 |
|
|
|
|
Exercise 2
Using the MSIL Disassembler
In this exercise, you will use the MSIL Disassembler to open a single assembly and familiarize yourself with the assembly manifest.
In subsequent labs, you will explore assemblies in greater detail.
! Examine the metadata for the Hello World applications
1.Open a Visual Studio .NET Command Prompt window.
2.From the Visual Studio .NET Command Prompt window, type:
>ildasm /source
3.Open HelloLabCS.exe and double-click Manifest.
4.Note the following:
a.The externally referenced library named mscorlib
b.The assembly name HelloLabCS
c.Version information (for the HelloLabCS assembly and mscorlib)
5.Close the Manifest window, double-click MainApp, double-click Main, and view the MSIL and source code.
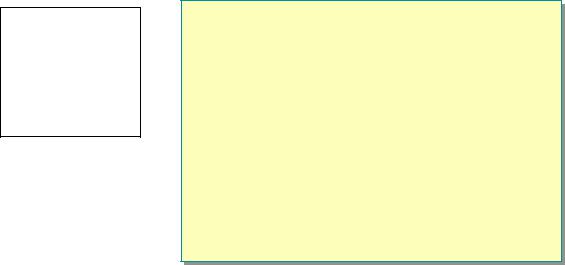
32 |
Module 2: Introduction to a Managed Execution Environment |
Review
Topic Objective
To reinforce module objectives by reviewing key points.
Lead-in
The review questions cover some of the key concepts taught in the module.
!Writing a .NET Application
!Compiling and Running a .NET Application
*****************************ILLEGAL FOR NON-TRAINER USE******************************
1.Name the root namespace for types in the .NET Framework.
The System namespace is the root namespace for types in the .NET Framework.
2.What class and methods can your application use to input and output to the console?
You can use the common language runtime’s Console class of the System namespace for input and output to the console of any string or numeric value by using the Read, ReadLine, Write, and WriteLine methods.
3.When compiling code that makes references to classes in assemblies other than mscorlib.dll what must you do?
You must use the /reference compilation switch. The /reference compilation option allows the compiler to make information in the specified libraries available to the source that is currently being compiled. The /r switch is equivalent to /reference.
4.What is the code produced by a .NET compiler called?
Microsoft intermediate language (MSIL), sometimes called managed code.