
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf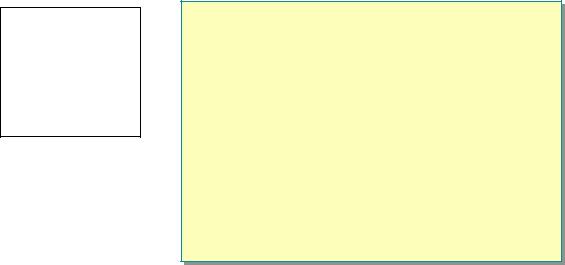
Module 1: Overview of the Microsoft .NET Framework |
17 |
|
|
|
|
Review
Topic Objective
To reinforce module objectives by reviewing key points.
Lead-in
The review questions cover some of the key concepts taught in the module.
!Overview of the Microsoft .NET Framework
!Overview of Namespaces
*****************************ILLEGAL FOR NON-TRAINER USE******************************
1.List the components of the .NET Framework.
The common language runtime, .NET Framework class library, data and XML, XML Web services and Web Forms, and Windows Forms.
2.What is the purpose of the common language runtime?
It provides an environment in which you can execute code.
3.What is the purpose of the common language specification?
It defines a set of features that all .NET languages should support.
4.What is an XML Web service?
An XML Web service is a programmable Web component that can be shared among applications on the Internet or an intranet.
5.What is a managed environment?
A managed environment is an environment that provides services, such as garbage collection, security, and other related features.
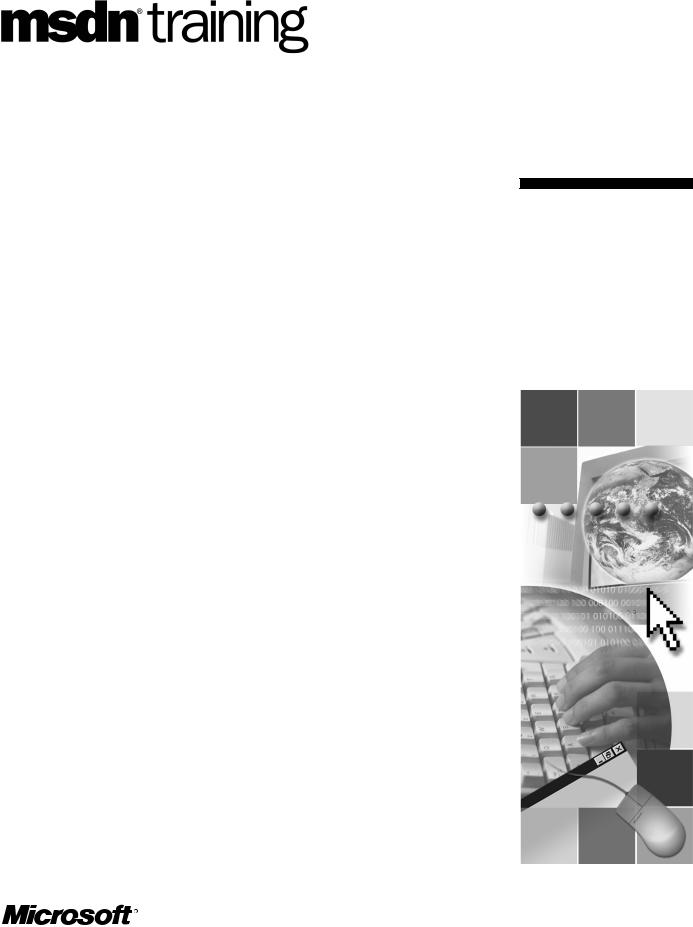
Module 2: Introduction to a Managed Execution Environment
Contents |
|
Overview |
1 |
Writing a .NET Application |
2 |
Compiling and Running a .NET Application |
11 |
Lab 2: Building a Simple .NET Application |
29 |
Review |
32 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.
Module 2: Introduction to a Managed Execution Environment |
iii |
|
|
|
|
Instructor Notes
Presentation:
45 Minutes
Lab:
20 Minutes
After completing this module, students will be able to:
!Create simple console applications in C#.
!Explain how code is compiled and executed in a managed execution environment.
!Explain the concept of garbage collection.
Materials and Preparation
This section provides the materials and preparation tasks that you need to teach this module.
Required Materials
To teach this module, you need the following materials:
!Microsoft® PowerPoint® file 2349B_02.ppt
!Sample managed module HelloDemoCS.exe
Preparation Tasks
To prepare for this module, you should:
!Read all of the materials for this module.
!Practice the demonstrations.
!Review the animation.
!Complete the lab.

iv |
Module 2: Introduction to a Managed Execution Environment |
Demonstrations
This section provides demonstration procedures that will not fit in the margin notes or are not appropriate for the student notes.
Hello World
This demonstration shows how to build a simple application in C#.
In the following procedures, use Notepad to create the simple Hello World application, and build and run the HelloDemoCS.exe application from the command line.
!To create the source code in C#
1.Open Notepad and type the following code:
//Allow easy reference to System namespace classes using System;
//Create class to hold program entry point
class MainApp {
public static void Main() {
// Write text to the console Console.WriteLine(“Hello World using C#!”);
}
}
2. Save the file as HelloDemoCS.cs.
Important To use Microsoft Visual Studio® .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click Start, All Programs, Microsoft Visual Studio .NET,
Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
Module 2: Introduction to a Managed Execution Environment |
v |
|
|
|
|
! To compile the source code and build an executable program
•From a Visual Studio .NET Command Prompt window, type the following syntax:
csc HelloDemoCS.cs
Running the resulting executable file will generate the following output:
Hello World using C#!
Viewing Assembly Metadata by Using the MSIL Disassembler
This demonstration shows how to use the Microsoft Intermediate Language (MSIL) Disassembler (Ildasm.exe) to view an assembly’s metadata.
The code for this demonstration is contained in one project and is located in <install folder>\Democode\Mod02. To demonstrate how to use the MSIL Disassembler to view the contents of the HelloDemoCS.exe assembly, follow the directions in Demonstration: Using the MSIL Disassembler in this module.
vi |
Module 2: Introduction to a Managed Execution Environment |
Multimedia
This section lists the multimedia items that are part of this module. Instructions for launching and playing the multimedia are included with the relevant slides.
Application Loading and Single-File Assembly Execution
This animation will show students how a single-file private assembly is loaded and executed.
Module Strategy
Use the following strategy to present this module:
!Writing a .NET Application
Stress the importance of understanding the process of compiling and running Microsoft .NET Framework applications, by using the simple Hello World application. Focus primarily on the compilation and execution processes.
!Compiling and Running a .NET Application
This section introduces basic concepts of a managed execution environment and presents new terminology. Many of these concepts are covered in greater detail in subsequent modules in this course, in subsequent courses, and in the .NET Framework software development kit (SDK) documentation.
Emphasize that you are primarily introducing new concepts and terminology. Be prepared to postpone answering questions that pertain to information that is covered in later modules. Encourage students to start reading the .NET Framework SDK documentation.
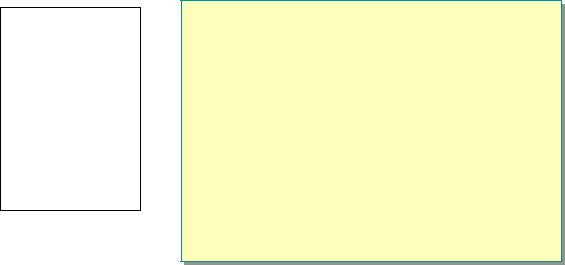
Module 2: Introduction to a Managed Execution Environment |
1 |
|
|
|
|
Overview
Topic Objective
To provide an overview of the module topics and objectives.
Lead-in
This module introduces the concept of managed execution and shows you how to quickly build applications that use the Microsoft .NET Framework common language runtime environment.
!Writing a .NET Application
!Compiling and Running a .NET Application
*****************************ILLEGAL FOR NON-TRAINER USE******************************
This module introduces the concept of managed execution and shows you how to quickly build applications that use the Microsoft® .NET Framework common language runtime environment. A simple Hello World version of a console application illustrates most of the concepts that are introduced in this module.
Because this course is an introduction to programming in the .NET Framework, you should spend some time reading the .NET Framework software development kit (SDK) documentation. In fact, the labs, demonstrations, and material for this module and other modules in this course are based on several tutorials in the .NET Framework SDK.
After completing this module, you will be able to:
!Create simple console applications in C#.
!Explain how code is compiled and executed in a managed execution environment.
!Explain the concept of garbage collection.
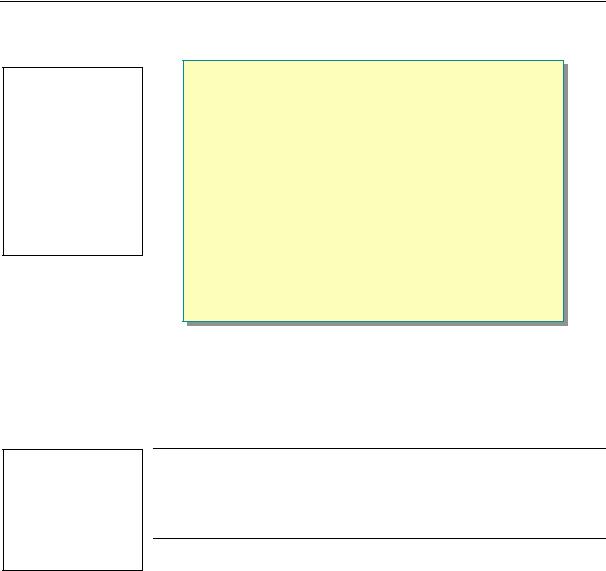
2Module 2: Introduction to a Managed Execution Environment
" Writing a .NET Application
Topic Objective
To introduce the topics in the section.
Lead-in
Because all supported languages use the Common Type System and the .NET Framework class library, and run in the common language runtime, programs in the supported languages are similar.
!Using a Namespace
!Defining a Namespace and a Class
!Entry Points, Scope, and Declarations
!Console Input and Output
!Case Sensitivity
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Delivery Tip
Stress the importance of understanding the process of compiling and running the
.NET applications. Using Visual Studio .NET at this time may obscure the underlying processes.
Because all supported languages use the Common Type System and the .NET Framework base class library, and run in the common language runtime, programs in the supported languages are similar. The most significant difference in programming with the supported languages is syntax.
Note In this module, and in Modules 3 and 4, Notepad is used as the source code editor, instead of the Microsoft Visual Studio® .NET development environment. The examples in these modules are simple enough to be compiled and built directly from a command prompt window. Working in Notepad will allow you to focus on the compilation and execution processes.