
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf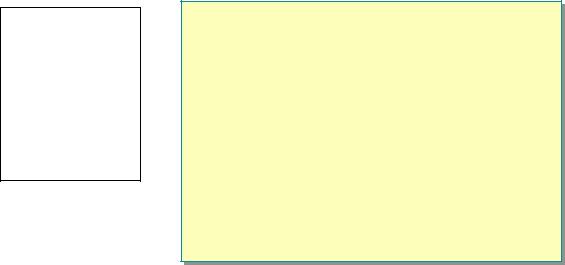
Module 5: Common Type System |
9 |
|
|
|
|
Primitive Types
Topic Objective
To explain how primitive types are used in the common language runtime.
Lead-in
Primitive types in the .NET Framework common language runtime are simple value types, commonly found in most programming languages.
!Simple Small Types Common in Most Languages
!Naming Differences
!C# Support
!Conversions
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Primitive types in the .NET Framework common language runtime are simple value types, which are commonly found in most programming languages. Primitive types are simple small types, such as Boolean types or character types.
The following table identifies all of the primitive types available in the .NET Framework common language runtime. The table lists the Microsoft intermediate language (MSIL) assembler name of the type, which you can see when you view the disassembly of managed code in the MSIL Disassembler, the name of the type in C#, the name of the type in the .NET Framework class library, and the description of the type.
Name in |
|
Name in |
|
MSIL Assembler |
Name in C# |
class library |
Description |
|
|
|
|
bool |
bool |
System.Boolean |
True/false value |
char |
char |
System.Char |
Unicode 16-bit char |
float32 |
float |
System.Single |
IEEE 32-bit float |
float64 |
double |
System.Double |
IEEE 64-bit float |
int8 |
sbyte |
System.SByte |
Signed 8-bit integer |
int16 |
short |
System.Int16 |
Signed 16-bit integer |
int32 |
int |
System.Int32 |
Signed 32-bit integer |
int64 |
long |
System.Int64 |
Signed 64-bit integer |
unsigned int8 |
byte |
System.Byte |
Unsigned 8-bit integer |
unsigned int16 |
ushort |
System.UInt16 |
Unsigned 16-bit integer |
unsigned int32 |
uint |
System.UInt32 |
Unsigned 32-bit integer |
unsigned int64 |
ulong |
System.UInt64 |
Unsigned 64-bit integer |

10 Module 5: Common Type System
The following example shows how to create and initialize the primitive System.UInt16 type in C# to store an employee’s age:
System.UInt16 age = new System.UInt16(); age = 5;
Primitive types are inherently supported in C#, allowing you to use simpler syntax, as in the following example:
ushort age = 5;
You can convert primitive types by using the Convert class. The Convert class defines static methods to convert from one primitive type to another. The following example shows how to convert a long type to an unsigned short type:
long longAge = 32; ushort ushortAge;
ushortAge = Convert.ToUInt16(longAge);
C# provides a more convenient conversion mechanism: casting. The following example shows how to explicitly cast a long type to an unsigned short type:
long longAge = 32; ushort ushortAge;
ushortAge = (ushort) longAge;
Casting in C# will automatically compile to conversion methods, such as ToUInt16. Also, you can use casting to avoid memorizing the class library names for primitive types.

Module 5: Common Type System |
11 |
|
|
|
|
Objects
Topic Objective
To explain the role of object types in the Common Type System.
Lead-in
Everything in the Common Type System is an object and inherits from
System.Object.
!Every Class Inherits from System.Object
!Objects Specify Data and Behavior
!Fields Define the Data
!Methods Define the Behavior
class Accumulator class Accumulator
{{ public float Result; public float Result;
public void Add(float amount) public void Add(float amount)
{{ Result += amount; Result += amount;
}}
}}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Everything in the Common Type System is an object and inherits from System.Object. An object type declaration specifies data and behavior. The Common Type System defines data in terms of fields and defines behavior in terms of methods. Each field or method in an object is called a member of that object.
An object is an instance of a class. The class specifies the types that are contained in a class and the class’s operations; an object is an instance containing a set of data values.
In C#, you can use the class keyword to declare an object type that is a reference type. The following example shows how to declare a class called
Accumulator.
class Accumulator
{
// Fields and methods defined here
}
Fields
Fields describe the data in an object. In the preceding example of the Accumulator class, a field is needed to store the current result that has been accumulated.
class Accumulator
{
public float Result;
}

12 Module 5: Common Type System
Methods
Methods describe the operations in an object. A method can have arguments and can also have a return type. Because the Common Type System requires strong typing, you must define a specific type for every parameter and return value.
The following example shows how to specify an Add method for the Accumulator class that takes an amount of type float and adds it to the Result field.
class Accumulator
{
public float Result;
public void Add(float amount)
{
Result += amount;
}
}
The following code is an example of client code:
Accumulator calc = new Accumulator(); calc.Result = 0;
calc.Add(5);
Console.WriteLine(calc.Result);
This code generates the following output:
5
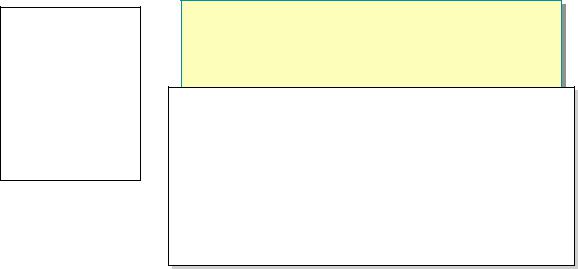
Module 5: Common Type System |
13 |
|
|
|
|
Constructors
Topic Objective
To explain how to use constructors to initialize classes.
Lead-in
If you design a class that requires special initialization code, you can use a constructor to initialize the class properly when objects are created.
!Constructors Are Used to Initialize Classes
!Constructors Can Have Zero or More Parameters
class Rectangle class Rectangle
{{ private int x1,y1,x2,y2; private int x1,y1,x2,y2;
public Rectangle(int x1, int y1, int x2,int y2) public Rectangle(int x1, int y1, int x2,int y2)
{{
}}
}}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
If you design a class that requires special initialization code, you can use a constructor to initialize the class properly when objects are created. A constructor is a method with the same name as the class. The constructor runs when a new instance of the class is created.
The following example shows how a constructor is used to initialize a class:
public class Rectangle
{
//Rectangle coordinates private int x1,y1,x2,y2;
//Initialize coordinates in constructor public Rectangle()
{
x1 = 0;
x2 = 0;
y1 = 0;
y2 = 0;
}
}
public class MainClass
{
public static void Main()
{
//Creating a new Rectangle //will call constructor Rectangle r = new Rectangle();
}
}

14 Module 5: Common Type System
Default Constructor
If you do not specify a constructor, the C# compiler will automatically provide a default constructor. The default constructor takes no parameters and calls the base class constructor.
Parameterized Constructor
You can also create a constructor with parameters as follows:
class Rectangle
{
//Rectangle coordinates private int x1,y1,x2,y2;
//Initialize coordinates in constructor
public Rectangle(int x1, int y1, int x2,int y2)
{
this.x1 = x1; this.x2 = x2; this.y1 = y1; this.y2 = y2;
}
}
public class MainClass
{
public static void Main()
{
//Must specify parameters //for this constructor
Rectangle r = new Rectangle(2,2,10,10);
}
}
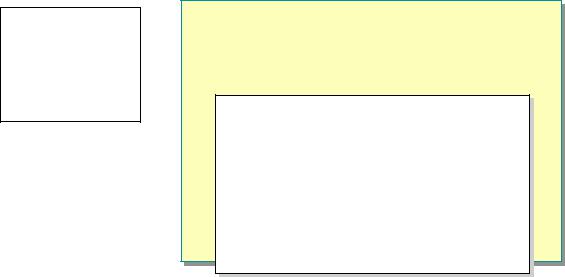
Module 5: Common Type System |
15 |
|
|
|
|
Properties
Topic Objective
To describe how to use properties.
Lead-in
Properties are values that can be stored or retrieved on a class.
!Properties Are Similar to Fields
!Properties Use get and set Accessor Methods for Managing Data Values
public float Start public float Start
{{ get get
{
{ return start; return start;
}
}
set set
{
{ if (start >= 0) start = value; if (start >= 0) start = value;
}
}}
}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Properties are values that can be stored or retrieved on a class. Like fields and methods, properties are also considered members of an object. Properties are different than fields because access to properties is controlled through get and set accessor methods. Also, properties are not necessarily stored in a single variable. They may be calculated on the fly or stored in multiple variables.
get and set Accessors
Property storage and property retrieval are defined by the get and set accessor methods. You write code in the get accessor method to determine how to retrieve a property value. You write code in the set accessor method to determine how to store a property value. By omitting the get or the set accessor, you can make a property read-only or write-only.

16 Module 5: Common Type System
The following example shows how to implement a Distance class, which can calculate the length of a trip from start to finish. The class contains three properties: Start, Finish, and Length. The Length property is read-only and is calculated on the fly.
class Distance
{
float start, finish; public float Start
{
get
{
return start;
}
set
{
if (start >= 0) start = value;
}
}
public float Finish
{
get
{
return finish;
}
set
{
if (finish >= start) finish = value;
}
}
public float Length
{
get
{
return (finish - start);
}
}
}
public class MainClass
{
public static void Main()
{
Distance d = new Distance(); d.Start = 5;
d.Finish = 10;
float length = d.Length;
}
}
Module 5: Common Type System |
17 |
|
|
|
|
In the preceding example, verification code is used in the set statements to control how the properties are set. Negative numbers are not allowed. Additional code could be written to throw an exception if invalid values are used.
The client code in MainClass does not have to use method syntax with parentheses to refer to properties.
In general, you should always use properties to expose data items to external classes. Properties allow you to encapsulate fields, so that you can change them in the future, if necessary.
For more information about encapsulation, see Encapsulation in this module.
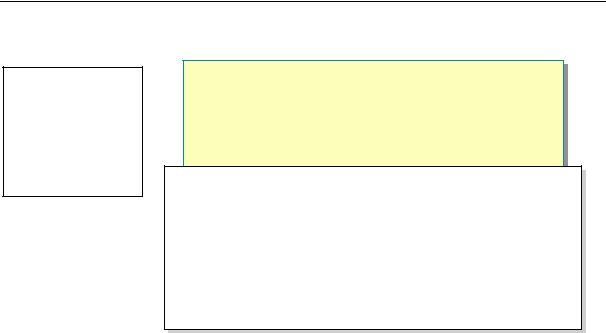
18 Module 5: Common Type System
Custom Types
Topic Objective
To describe how to build data types that use the C# struct keyword.
Lead-in
You can build your own data type by using the C# struct keyword.
!Inherit from System.ValueType
!Are Defined with the struct Keyword in C#
!Can Have Methods, Properties, and Fields
struct Employee struct Employee
{{ public string Name; public string Name; public ushort Age; public ushort Age; public DateTime HireDate;
public DateTime HireDate; public float Tenure() public float Tenure()
{{
}}
}}
*****************************ILLEGAL FOR NON-TRAINER USE******************************
You can build your own data types by using the C# struct keyword. The struct keyword will define a custom type that inherits from System.ValueType, which in turn inherits from System.Object. All value types are sealed; additional classes cannot be derived from them.
The following example shows how the C# struct keyword is used to build a simple custom type that describes an individual employee:
struct Employee
{
public string Name; public ushort Age; public DateTime HireDate;
}
In C#, structures can also have methods as in the following example:
struct Employee
{
public string Name; public ushort Age; public DateTime HireDate;
public float Tenure()
{
TimeSpan ts = DateTime.Now – HireDate; return ts.Days/(float)365;
}
}
Structures can also have properties. For example, the Employee structure could be modified so that employee age is a property. Then the set accessor could be used to verify that the age is a valid age.