
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf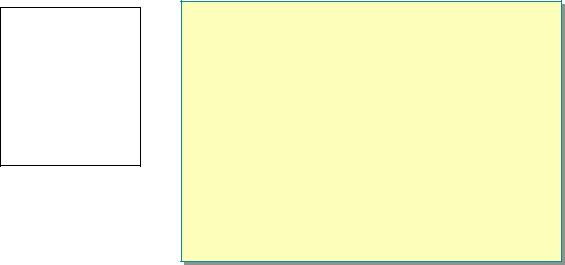
Module 4: Deployment and Versioning |
7 |
|
|
|
|
" Application Deployment Scenarios
Topic Objective
To introduce the topics in the section.
Lead-in
This section shows how to deploy a variety of applications, from simple applications to applications that make use of assembly versioning.
!A Simple Application
!A Componentized Application
!Specifying a Path for Private Assemblies
!A Strong-Named Assembly
!Deploying Shared Components
!A Versioned Assembly
!Creating Multiple Versions of a Strong-Named Assembly
!Binding Policy
!Deploying Multiple Versions of a Strong-Named Assembly
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The examples in this section show how to deploy a simple application, an application that uses a shared assembly, and an application that makes use of assembly versioning.
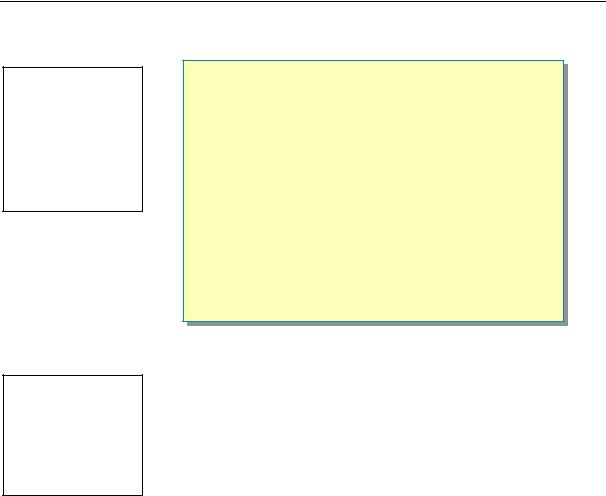
8Module 4: Deployment and Versioning
A Simple Application
Topic Objective
To explain how a simple application is deployed.
Lead-in
In Module 2, you looked at the simplest .NET Framework application, the traditional Hello World application written in C#.
!Use the Microsoft Intermediate Language Disassembler (Ildasm.exe) to Examine the Assembly Manifest
#Version information
#Imported types
#Exported types
!Deploy the Application by:
#Running the executable file directly from a file server, or
#Installing it locally by copying the file
#Uninstall the application by deleting the file
*****************************ILLEGAL FOR NON-TRAINER USE******************************
For Your Information
Point out that while Hello World is simplistic, it is extremely versatile in its ability to easily demonstrate many new concepts of the
.NET Framework, including deployment and versioning.
In Module 2, “Introduction to a Managed Execution Environment,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft
Visual C# .NET), you looked at the simplest .NET Framework application, the traditional Hello World application written in C#. The C# source code for that program, HelloDemoCS, is as follows:
using System;
class MainApp {
public static void Main() { Console.WriteLine("Hello World using C#!");
}
}
This simple executable file prints a single line to the System.Console, a type that is contained in the .NET Framework class library. It does not reference any other libraries and does not itself produce a library.
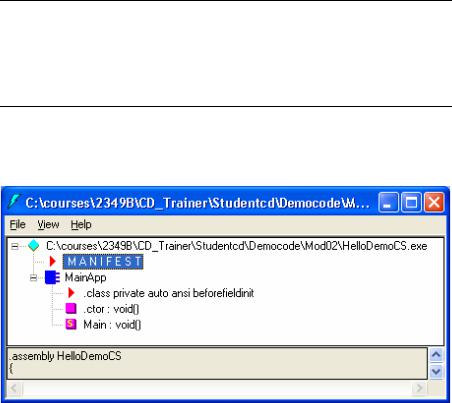
Module 4: Deployment and Versioning |
9 |
|
|
|
|
Using Microsoft Intermediate Language Disassembler to
Examine Hello World
Compiling this small application generates the HelloDemoCS.exe.
Important To use Microsoft Visual Studio® .NET tools within a command prompt window, the command prompt window must have the proper environment settings. The Visual Studio .NET Command Prompt window provides such an environment. To run a Visual Studio .NET Command Prompt window, click Start, All Programs, Microsoft Visual Studio .NET,
Visual Studio .NET Tools, and Visual Studio .NET Command Prompt.
If you run the Microsoft intermediate language (MSIL) disassembler (Ildasm.exe) against this executable file, a window appears, similar to the following illustration.
The simple Hello World application highlights a particularly important concept behind programming for the .NET Framework. The application is clearly selfdescribing: all of the information that is needed to understand the application is contained in the metadata.
The MSIL disassembler shows the classes or types that are created within the application. In the case of the Hello World application, the only class is MainApp. The MSIL disassembler also shows the methods Main and a default constructor, indicated by .ctor. The program does not have any other members. To save information about the assembly to a file, use the Dump command on the File menu.
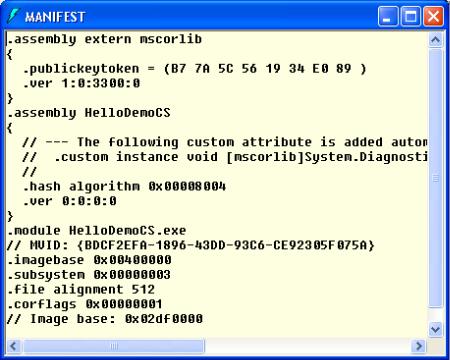
10 |
Module 4: Deployment and Versioning |
To see additional information about the application, double-click Manifest. The following window appears.
The preceding illustration shows the manifest, which contains information about the assembly, including the version (not yet set), external libraries, and types within those libraries, which the application uses.
Deployment
Deployment to computers on which the .NET runtime is installed is a simple process. The Hello World application can run directly from a file server. More advanced programs may involve security issues.
In the simple Hello World case, no files are placed on the workstation, no entries are made in the system registry, and, in effect, there is no affect on the client workstation. There is also nothing to clean up because there is nothing to remove on the client workstation.
As you would expect, HelloDemoCS.exe can also be copied to a local volume. In this scenario, you can remove the program by deleting the file, and as before, nothing would remain on the workstation.
Whether you run HelloDemoCS.exe from a file server or copy it to a local volume, running this application will not break another program, and no other application can cause HelloDemoCS.exe to stop functioning.
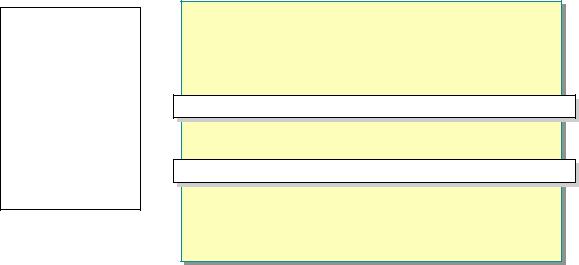
Module 4: Deployment and Versioning |
11 |
|
|
|
|
A Componentized Application
Topic Objective
To introduce compapp, a componentized application, which uses multiple assemblies.
Lead-in
The Hello World application that is discussed in the preceding topic is completely trivial and hardly representative of even the simplest real-world application.
! Assembly Component to Be Used by Application
# Assembly Stringer.dll is built from Stringer.cs as follows:
csc /target:library Stringer.cs csc /target:library Stringer.cs
! Client Needs to Reference Assembly
csc /reference:Stringer.dll Client.cs csc /reference:Stringer.dll Client.cs
! Deployment by File Server or Local Copy
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The Hello World application that is discussed in the preceding topic is completely trivial and hardly representative of even the simplest real-world application. This topic covers compapp, a componentized application, which uses multiple assemblies.
12 |
Module 4: Deployment and Versioning |
Creating Stringer.dll
The client, Client.exe, calls types that are contained in a component assembly that is named Stringer (Stringer.dll). The source code for the Stringer assembly, is located in Stringer.cs:
using System;
namespace org {
public class Stringer { private string[] StringSet;
public Stringer() {
StringSet = new string[] { "C# String 0",
"C# String 1",
"C# String 2",
"C# String 3"
};
}
public string GetString(int index) {
if ((index < 0) || (index >= StringSet.Length)) { throw new IndexOutOfRangeException();
}
return StringSet[index];
}
public int Count {
get { return StringSet.Length; }
}
}
}
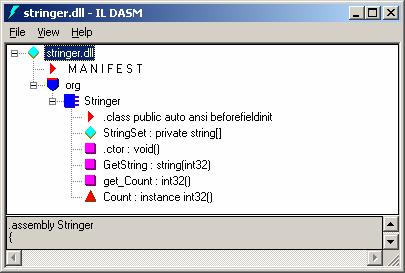
Module 4: Deployment and Versioning |
13 |
|
|
|
|
A client application that uses this component could fully qualify a reference to the Stringer class, for instance, as org.Stringer. Alternatively, a client application could include a using statement to allow easy access to the types in Stringer.dll by specifying the namespace, as shown in the Client.cs source code:
using System; using org;
class MainApp {
public static void Main() {
Stringer myStringComp = new Stringer(); string[] StringsSet = new string[4];
//Iterate over component's strings Console.WriteLine("Strings from StringComponent");
for (int index = 0; index < myStringComp.Count; index++)
{
StringsSet[index] = myStringComp.GetString(index); Console.WriteLine(StringsSet[index]);
}
//...
}
}
Building the Application
To build this componentized application, you first build the Stringer.dll assembly component. Then, you build Client.exe, importing the Stringer component by using the name of the file that contains the manifest, Stringer.dll, rather than the namespace name, which is org in this case.
csc /target:library Stringer.cs
csc /reference:Stringer.dll Client.cs
The MSIL disassembler displays the Stringer.dll and shows all of the members, as in the following illustration.
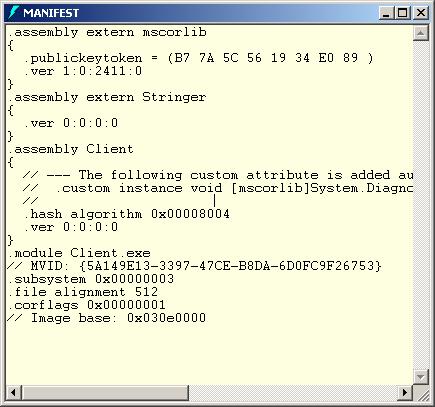
14 |
Module 4: Deployment and Versioning |
Like HelloDemoCS.exe, Client.exe contains manifest information about itself, the System library, and the types that the application uses. However, the manifest now contains information about the Stringer assembly.
A MSIL disassembler display of the manifest for Client.exe shows the reference to the Stringer assembly, as in the following illustration.
Deployment
Like the simple application, Client.exe can run directly from a file server on any workstation that has the .NET runtime installed. Client.exe and Stringer.dll can also be copied to a local volume. To remove the application, you need only delete the two files.
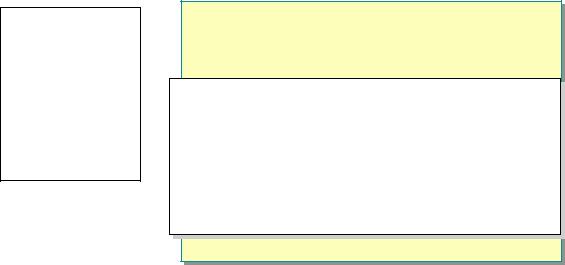
Module 4: Deployment and Versioning |
15 |
|
|
|
|
Specifying a Path for Private Assemblies
Topic Objective
To show how to use a configuration file to specify a path for private assemblies.
Lead-in
The .NET Framework provides a configuration mechanism that allows administrators to specify a directory from which to load private assemblies.
!Specifying a Directory From Which to Load Private Assemblies.
# Client.exe.config file specifies a privatePath tag
<configuration>
<configuration>
<runtime>
<runtime>
<assemblyBinding
<assemblyBinding
xmlns="urn:schemas-microsoft-com:asm.v1"> xmlns="urn:schemas-microsoft-com:asm.v1">
<probing privatePath=“MyStringer"/> <probing privatePath=“MyStringer"/>
</assemblyBinding>
</assemblyBinding>
</runtime>
</runtime>
</configuration>
</configuration>
! Configuration File’s XML Tags Are Case-Sensitive
*****************************ILLEGAL FOR NON-TRAINER USE******************************
The preceding Client.exe example has one important weakness: both Client.exe and Stringer.dll reside in the same directory. In the real world, an administrator may wish to use a directory structure to manage assemblies. The .NET Framework provides a configuration mechanism that allows administrators to specify a directory from which to load private assemblies.
Locating Stringer.dll and Client.exe in Separate Directories
Using the preceding Client example, all of the source code is the same, but for illustration purposes the build process has been modified so that the component application’s Stringer.dll is built in its own subdirectory named MyStringer.
cd \compapp
csc /target:library /out:MyStringer\Stringer.dll! MyStringer\Stringer.cs
csc /reference:MyStringer\Stringer.dll Client.cs
Using a Configuration File to Locate Assemblies at Run Time
Although the /reference: compile option locates an assembly in a directory when compiling the program, a separate XML-based application configuration file is required at run time to support assemblies that are located in other directories. For client executable files like the examples that are covered in this module, the configuration file resides in the same directory as the executable file. The configuration file has the complete name of the executable file with an additional extension of .config.
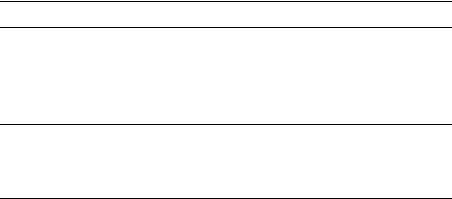
16 |
Module 4: Deployment and Versioning |
The Client.exe configuration file is called Client.exe.config. It specifies a privatePath attribute, as shown in the following example:
<configuration>
<runtime>
<assemblyBinding xmlns="urn:schemas-microsoft-com:asm.v1"> <probing privatePath="MyStringer"/>
</assemblyBinding>
</runtime>
</configuration>
Important The configuration file’s XML tags are case-sensitive.
When this configuration file is placed in the same directory as the executable file, at run time, the .NET Framework uses the privatePath attribute to determine where to look for components—in addition to looking in the application directory.
Note When loading an assembly, the runtime also searches for a private path that is equal to the name of the assembly. If the Stringer.dll assembly was located in a subdirectory named Stringer, instead of MyStringer, then a configuration file would be unnecessary.
Deployment
Like the simple application, the revised Client.exe can run directly from a file server on any workstation that has the .NET runtime installed. Client.exe, Stringer.dll, and the application’s .config file can also be copied to a local volume, using the same relative directory structure. Deleting the files and directory effectively removes the application.
While they are not used in the preceding example, it is important to know that in addition to application configuration files, the .NET Framework also supports separate user and Computer Configuration Files for many common configuration settings.