
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf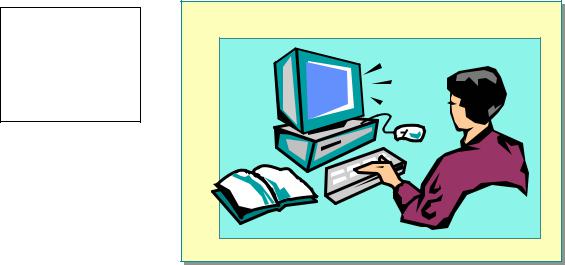
Module 5: Common Type System |
39 |
|
|
|
|
Lab 5: Building Simple Types
Topic Objective
To introduce the lab.
Lead-in
In this lab, you will create simple types and enumerations, and work with inheritance.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Objectives
After completing this lab, you will be able to:
!Create enumerations.
!Create custom value types as structures.
!Inherit from a base class and override properties and methods.
!Create constructors.
!Use appropriate levels of encapsulation through the private and public access modifiers.
Prerequisites
Before working on this lab, you must have:
!Knowledge about how to use Microsoft Visual Studio® .NET for creating and working with console applications.
!Knowledge about the C# language.
!Knowledge about the System.Console namespace for interacting with the console.
Estimated time to complete this lab: 45 minutes

40 Module 5: Common Type System
Exercise 1
Creating an Enumeration
In this exercise, you will create a simple program that uses an enumeration to reference weekdays. You will enumerate the weekdays, Monday through Friday, as a type. Then you will write code to accept a day from the user, match the day to the enumerated type, and write a response back to the user.
!Create an enumeration
1.Open the Days project located in the folder <install folder>\Labs\Lab05\ Starter\Days.
2.Open the Main.cs file.
3.Create an enumeration called Weekdays. The enumeration should be of type int and should enumerate the literals Monday, Tuesday, Wednesday,
Thursday, and Friday.
!Use an enumeration
1.Locate the Main method of the MainClass class.
2.In the Main method, write code to perform the following tasks:
a.Write a question to the console that reads, “What day of the week is it?”
b.Use the Console.ReadLine method to get the user’s response.
c.Determine which day the user selected by parsing the Weekdays enumeration. Store the correct Weekdays literal in a variable of type
Weekdays.
d.Use a switch statement on the mapped response to determine which day of the week it is. Based on the response, write a string to the user. For example, for Monday, write “Monday is the first weekday.” For Tuesday, write “Tuesday is the second weekday,” and so on.
3.Compile and test the code. Run the program and type in a weekday. You should get an appropriate response based on the day you entered.
Module 5: Common Type System |
41 |
|
|
|
|
Exercise 2
Creating a Custom Value Type
In this exercise, you will create a custom value type or structure to represent a complex number. Complex numbers have a real part and an imaginary part. You will design the structure to store the real and imaginary parts, and you will perform addition on complex numbers.
! Create a structure
1.Open the Complex project located in the folder <install folder>\Labs\ Lab05\Starter\Complex.
2.Open the Main.cs file.
3.Create a new structure called Complex.
a.Create a public member variable called Real of type int.
b.Create a public member variable called Imaginary of type int.
4.Create an overloaded Addition operator (+) for the Complex structure. The overloaded operator should look as follows:
public static Complex operator +(Complex c1, Complex c2)
{
}
a.In the Addition operator method, create a variable called result of type Complex to hold the result of the addition.
b.Add c1.Real to c2.Real and store the result in result.Real.
c.Add c1.Imaginary to c2.Imaginary and store the result in result.Imaginary.
d.Return the result.
! Use the structure
1.Locate the Main method in the MainClass class.
2.In the Main method, write code to create a variable called num1 of type
Complex.
3.Create a second variable called num2 of type Complex.
4.Initialize num1 and num2 with real and imaginary parts. For example, num1 could have values of 2 and 3 for the real and imaginary parts. num2 could have values of 3 and 4 for the real and imaginary parts.
5.Add the two numbers together, and print both the real and imaginary parts.
6.Compile the program and run it. Verify that the result is correct. Using the values in step 4, you should see the results of 5 and 7 for the real and imaginary parts.

42 Module 5: Common Type System
Exercise 3
Creating Objects
In this exercise, you will derive new classes from base classes and then use the derived classes. You will work with a base class called Polygon. The class represents the geometric characteristics of polygons.
Polygons have a number of sides. Thus the Polygon class defines a NumSides property but does not implement it. The derived classes implement the NumSides property. For example, a Triangle class could be created that overrides the NumSides property and initializes it to 3. A Pentagon class could be created that overrides the NumSides property and initializes it to 5.
The Polygon class also implements a Degrees property that returns the sum of all the angles in the polygon. For example, the angles in a triangle always add up to 180 degrees. This simple calculation is based on the number of sides in the polygon, and so the derived class must implement the NumSides property.
The Polygon class also defines an abstract method called Area that will return the area of the polygon. The derived class must override this method to calculate the correct area.
!Derive a new class
1.Open the Shapes project located in the folder <install folder>\Labs\Lab05\ Starter\Shapes.
2.Open the Polygon.cs file and study the code for the Polygon class. Notice that it is an abstract class that has an abstract property called NumSides, a property called Degrees, and an abstract method called Area that returns the area inside the polygon.
3.Open the Main.cs file.
4.Create a new class called Square that inherits from Polygon.
a.Create private member variables called x1, y1, and size of type int to store the Cartesian coordinates of the upper-left corner and the size of the square, which is the length of one side.
b.Create a private member variable called numSides of type ushort to store the number of sides that the square has.
c.Create a constructor that accepts three integer parameters (x1, y1, size) to construct a new square. Initialize the private member variables to the values passed as parameters. Initialize numSides to 4.
d.Override the NumSides property in the base class to return the numSides variable.
e.Create read/write properties called X1, and Y1 respectively that map to the private variables x1, and y1.
f.Create two read-only properties called X2 and Y2. These represent the lower-right coordinate of the square and are calculated by adding size to x1 or y1 respectively.
g.Override the Area method to return the area of the square. The area can be calculated with the following formula: (size)2.
Module 5: Common Type System |
43 |
|
|
|
|
! Use the derived class
1.Locate the Main method in the MainClass class.
2.In the Main method, write code to create a new Square object. Initialize the square with values for the upper-left corner and size, such as 1, 1, and 4.
3.Write the number of degrees in the square to the console by printing the Degrees property.
4.Write the area to the console by calling the Area method.
5.Write the x1, y1, x2, y2 coordinates to the console by using the properties of the square object.
6.Compile the program and test it. Verify that all the methods and properties work as expected. Given a square with upper-left coordinates of 1, 1, and a size of 4, the number of degrees is 360, the area is 16, and the lower-right coordinates are 5, 5.
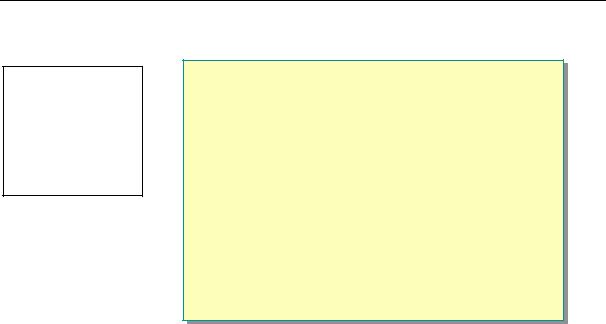
44 Module 5: Common Type System
Review
Topic Objective
To reinforce module objectives by reviewing key points.
Lead-in
The review questions cover some of the key concepts taught in the module.
!An Introduction to the Common Type System
!Elements of the Common Type System
!Object-Oriented Characteristics
*****************************ILLEGAL FOR NON-TRAINER USE******************************
1.What are the differences between value types and reference types?
Value types are allocated on the stack, assigned as copies, and passed by value.
Reference types are allocated on the heap, assigned as references, and passed by reference.
2.What are the differences between fields and properties?
A field is a data value in a class that can be directly accessed and manipulated by other classes.
A property is a value in a class that is accessed through get and set accessor methods. The actual data value of a property may be stored in the class instance, or calculated when accessed.
3.How do you create an enumeration in C#?
Use the enum keyword:
enum name : type
{
…literals…
}
Module 5: Common Type System |
45 |
|
|
|
|
4.What is an interface?
An interface is a contractual description of a set of related methods and properties.
5.How is encapsulation supported by the .NET Framework?
Encapsulation is supported through access modifiers, such as public, protected, internal, and private.
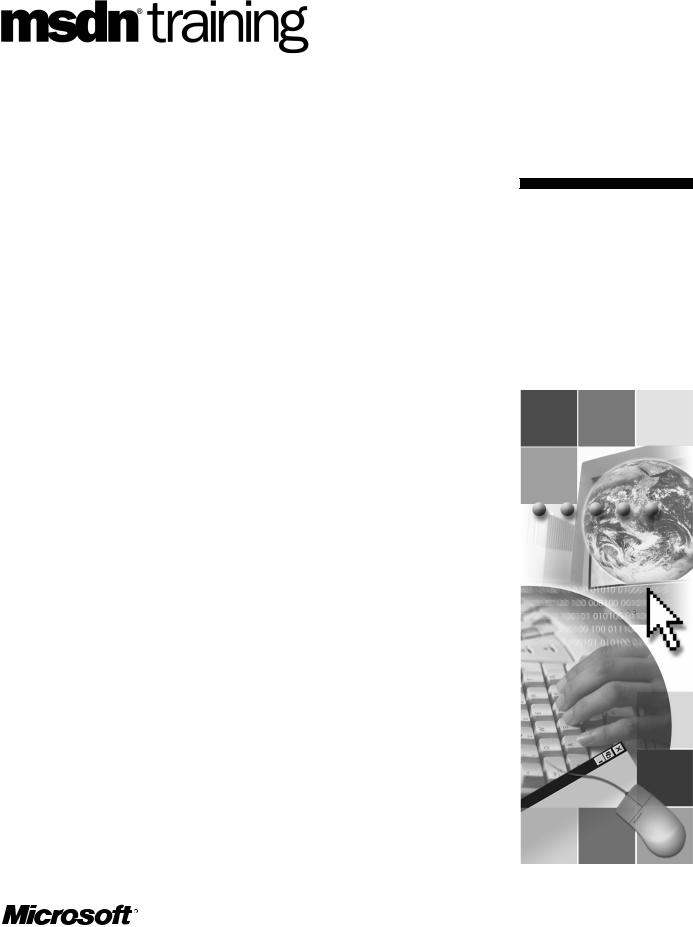
Module 6:
Working with Types
Contents |
|
Overview |
1 |
System.Object Class Functionality |
2 |
Specialized Constructors |
12 |
Type Operations |
18 |
Interfaces |
28 |
Managing External Types |
34 |
Lab 6: Working with Types |
38 |
Review |
43 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.