
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdfModule 7: Strings, Arrays, and Collections |
49 |
|
|
|
|
public static void printTable(Hashtable table) { Console.WriteLine ("Current list of employees:\n"); Console.WriteLine ("ID\tName");
Console.WriteLine ("--\t----"); foreach (DictionaryEntry d in table) {
Console.WriteLine ("{0}\t{1}", d.Key, d.Value);
}
}
}
The preceding code example displays the following output or similar output to the console:
Current list of employees:
ID |
Name |
-- |
---- |
1254 |
Brian |
6839 |
Seth |
3948 |
Rajesh |
1930 |
Lakshan |
0123 |
Jay |
0569 |
Brad |
9341 |
Kristian |
Search for employee by key, enter ID ==-> 111 |
|
Employee 111 not found. |
Search for employee by value, enter name ==-> Jay
Found Jay in |
the list. |
|
Current |
list |
of employees: |
ID |
Name |
|
-- |
---- |
|
1254 |
Brian |
|
6839 |
Seth |
|
3948 |
Rajesh |
|
1930 |
Lakshan |
|
0123 |
Jay |
|
0569 |
Brad |
|
9341 |
Kristian |
Remove employee by key, enter ID ==-> 9341
Current |
list |
of employees: |
ID |
Name |
|
-- |
---- |
|
1254 |
Brian |
|
6839 |
Seth |
|
3948 |
Rajesh |
|
1930 |
Lakshan |
|
0123 |
Jay |
|
0569 |
Brad |
|
Press Return |
to exit. |
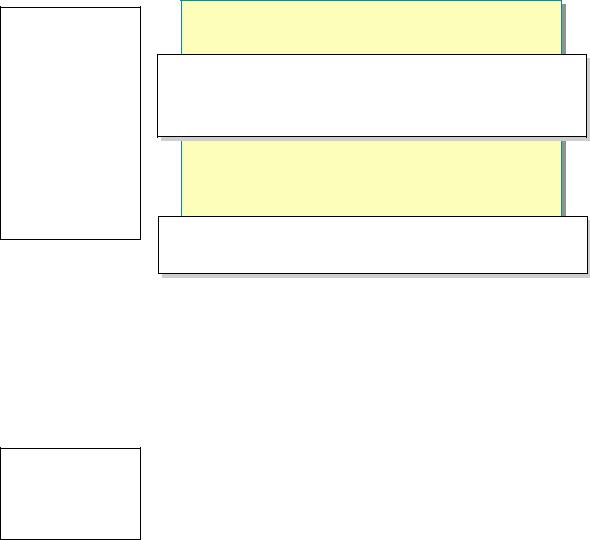
Module 7: Strings, Arrays, and Collections |
51 |
|
|
|
|
The following example shows how to create a SortedList, add an entry, modify an entry’s value, and print out the SortedList’s keys and values.
using System;
using System.Collections;
public class SamplesSortedList {
public static void Main() {
//Create and initialize a new SortedList. SortedList mySL = new SortedList(); mySL.Add("First", 1);
mySL.Add("Second", 2); mySL.Add("Third", 3);
//Display the properties and values of the SortedList. Console.WriteLine( "mySL" );
Console.WriteLine( " Count: {0}", mySL.Count ); Console.WriteLine( " Capacity: {0}", mySL.Capacity ); Console.WriteLine( " Keys and Values:" ); PrintKeysAndValues( mySL );
//increment the value of the entry whose key is "Third" mySL["Third"] = (Int32)mySL["Third"] + 1; PrintKeysAndValues( mySL );
}
public static void PrintKeysAndValues( SortedList myList ){ Console.WriteLine( "\t-KEY-\t-VALUE-" );
for ( int i = 0; i < myList.Count; i++ ) { Console.WriteLine( "\t{0}:\t{1}",
myList.GetKey(i), myList.GetByIndex(i) );
}
Console.WriteLine();
}
}
The preceding code example displays the following output to the console:
mySL
Count: 3
Capacity: 16 Keys and Values:
-KEY- -VALUE- First: 1 Second: 2 Third: 3
-KEY- -VALUE-
First: 1
Second: 2
Third: 4
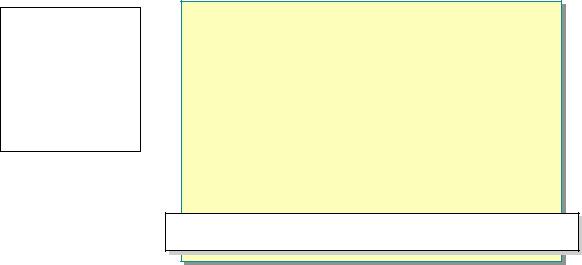
Module 7: Strings, Arrays, and Collections |
53 |
|
|
|
|
Choosing A Collection Class
Choosing the right Collections class must be done carefully. Using the wrong collection can unnecessarily restrict how you use it.
Consider the following questions:
!Do you need temporary storage?
•If yes, consider Queue or Stack.
•If no, consider the other collections.
!Do you need to access the elements in a certain order, such as first-in-first- out, last-in-first-out, or randomly?
•The Queue offers first-in, first-out access.
•The Stack offers last-in, first-out access.
•The rest of the collections offer random access.
!Do you need to access each element by index?
•ArrayList and StringCollection offer access to their elements by the zero-based index.
•Hashtable, SortedList, ListDictionary, and StringDictionary offer access to their elements by the key of the element.
•NameObjectCollectionBase and NameValueCollection offer access to their elements either by the zero-based index or by the key of the element.
!Will each element contain just one value or a key-singlevalue pair or a keymultiplevalues combination?
•One value: Use any of the collections based on IList.
•Key-singlevalue pair: Use any of the collections based on IDictionary.
•Key-multiplevalues combination: Consider using or deriving from the
NameValueCollection class in the Collections.Specialized namespace.
!Do you need to sort the elements differently from how they were entered?
•Hashtable sorts the elements by the hash code of the key.
•SortedList sorts the elements by the key, based on an IComparer implementation.
•ArrayList provides a Sort method that takes an IComparer implementation as a parameter.
!Do you need fast searches and retrieval of information?
•ListDictionary is faster than Hashtable for small collections of ten items or less.
!Do you need collections that accept only strings?
•StringCollection (based on IList) and StringDictionary (based on IDictionary) are in the Collections.Specialized namespace.
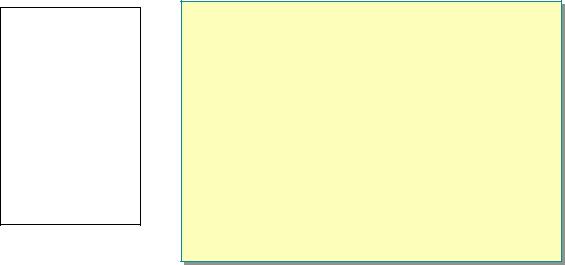
Module 7: Strings, Arrays, and Collections |
55 |
|
|
|
|
The System.Collections classes provide a simple and powerful way to implement collections. Carefully evaluate whether the use of these classes in your programs imposes a significant enough performance penalty to warrant taking the further action that is described in the following sections.
Strongly-Typed Collections
One way to provide compile-time type-checking and improve the performance of collection code is to use strongly-typed collections. The System.Collections.Specialized namespace contains string-specific collection classes, as described in the following table.
Class |
Description |
NameValueCollection |
Represents a sorted collection of associated String keys |
|
and String values that can be accessed with the hash |
|
code of the key or with the index. |
StringCollection |
Represents a collection of strings. |
StringDictionary |
Implements a hash table with the key strongly-typed to |
|
be a string, rather than an object. |
StringEnumerator |
Supports a simple iteration over a StringCollections. |
Creating Custom Collection Classes
You can also create your own strongly-typed collection by creating your own custom collection class. Your class can reuse System.Collections functionality by inheriting from the appropriate System.Collections class. You create the type-specific class with methods and properties whose parameters and return types are type-specific.
By using C#’s explicit interface implementation mechanism, which is discussed in Module 6, “Working with Types,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C#™ .NET), a class can implement both non-interface and interface members of the same name. Explicit interface method implementations are frequently used when you implement such interfaces as IEnumerable, IEnumerator, ICloneable, IComparable, ICollection, IList, and IDictionary. By having both sets of members, clients that expect type-specific or System.Object parameters and return values can use the custom class.
Techniques for Handling Boxing and Unboxing
Instead of creating a type-specific collection class, you can remove the overhead of boxing and unboxing by using a reference type, rather than a value type, for the items in a collection. To accomplish this, you can wrap a value type in a class, as shown in Module 6, Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C# .NET).
In some cases, you can reduce boxing and unboxing by using interfaces. Value types can implement interfaces, but because interfaces are referenced types, you can only have an interface reference to a boxed value type.
You can define an interface for your value type that has the methods and properties that are needed to manipulate the value type. The value type instance must still be boxed when it is inserted into a generic System.Collections object, but your code will be able to manipulate the item’s value through its interface without unboxing the item.
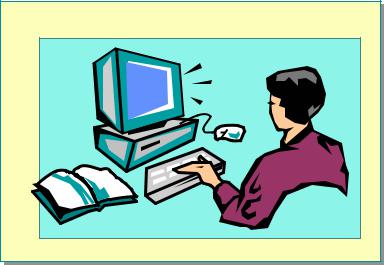
Module 7: Strings, Arrays, and Collections |
57 |
|
|
|
|
Lab 7: Working with Strings, Enumerators, and Collections
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Objectives
After completing this lab, you will be able to:
!Create an application that parses and formats strings.
!Create an application that uses SortedList collection objects.
Lab Setup
Starter and solution files are associated with this lab. The starter files are in the folder <install folder>\Labs\Lab07\Starter, and the solution files are in the folder <install folder>\Labs\Lab07\Solution.
Scenario
In this lab, you are provided with a Visual Studio .NET console application as a starting point. The application, named WordCount, parses a test string into words and then determines the number of unique words and the number of times each word occurs. The results of this analysis are formatted and displayed on the console.
The application is a modified version of the .NET Framework SDK sample,
Word Count.
Estimated time to complete this lab: 60 minutes

58 |
Module 7: Strings, Arrays, and Collections |
Exercise 1
Sorting Words Alphabetically
In this exercise, you will create a class that breaks up the test string into words and then stores each word and the number of its occurrences in a SortedList. By default, the sort order will be based on the alphabetical ordering of the word keys.
!Modify the WordCount class to sort words alphabetically
1.Open the WordCount project in the starter directory by using Visual Studio
.NET.
2.Open the WordCount.cs file.
3.In the WordCounter class, create a member named wordCounter that is an instance of the SortedList class.
4.Create a read-only property named UniqueWords that returns the number of items in wordCounter.
5.Create a public method named GetWordsAlphabeticallyEnumerator that takes no arguments and returns a wordCounter enumerator object of type
IDictionaryEnumerator.
6.Create a public method named CountStats that takes two out parameters of type Int64 named numWords and numChars and returns a Boolean.
7.Implement the CountStats method to break up the test string, create the alphabetically sorted list, and return the word count statistics.
a.Initialize the CountStats method’s out parameters to 0.
b.Use the String.Split method to break up testString into individual words that are stored in an array of type string named Words.
c.Assign to the out parameter numWords the number of words that are obtained in step 7.b.
d.For each non-empty string in the array Words:
i.Add the number of characters in the word string to numChars.
ii.If wordCounter does not already contain the string, add a new entry whose key is the string and whose value is 1. The value represents the number of occurrences of the word. Otherwise, increment by one the value of the existing entry.
Tip For information about and examples of the IDictionary methods named Add and Contains, see Dictionaries and Demonstration: Hashtable in this module. For an example of how to modify the value of an entry by using the [] Operator, see SortedList in this module.
iii. Return true.