
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdfModule 7: Strings, Arrays, and Collections |
59 |
|
|
|
|
! Modify the Application class
1.In the Application class's Main method, create an instance of
WordCounter named wc.
2.Create two variables of type Int64 named NumWords and NumChars.
3.Call the wc object’s CountStats method to assign the word and character counts to NumWord and NumChars respectively.
4.Output to the console the test string WordCounter.testString.
5.Output to the console a two-column header labeled Words and Chars. Use a tab to separate the columns.
6.Output to the console the number of words, and format this output to occupy five characters, followed by a tab and the number of characters.
! Test the WordCount application
•Build and run the application. You should see output that is similar to the following:
For string
Hello world |
|
|
hello here |
i am where are you hello you |
|
Words |
Chars |
|
11 |
41 |
|

60 |
Module 7: Strings, Arrays, and Collections |
Exercise 2
Sorting Words by Number of Occurrences
In this exercise, you will create a nested class that implements the
IComparable interface and whose CompareTo method will result in a
SortedList that is ordered on the basis of the number of occurrences of a word.
!Modify the WordCount class to sort words by occurrences
1.Add a nested class named WordOccurrence that inherits from
IComparable.
2.Add a private member of type int named occurrences.
3.Add a private member of type String named word.
4.Add a constructor that takes two parameters. The first parameter is of type int, and the second parameter is of type String. The constructor assigns the first value to occurrences and the second value to word.
5.Add a public method CompareTo that takes an Object parameter and returns an int.
The code should follow the CompareTo design pattern and return a value that results in a sort of words that is ordered by the occurrences of each word in ascending order. Less frequently occurring words should be followed by more frequently occurring words.
In the case in which the number of occurrences of the instance and the Object parameter are the same, the sort should be alphabetical by using the
String.Compare method.
Tip For an example of implementing the CompareTo method, see Demonstration: Sorting and Enumerating an Array in this module.
6.Add two public read-only properties named Occurrences and Word that return the fields occurrences and word respectively. End the nested class definition.
7.Back in the WordCounter class itself, add a public method named
GetWordsByOccurrenceEnumerator that returns an object of type IDictionaryEnumerator. Implement GetWordsByOccurrenceEnumerator as follows:
a.Create a new SortedList named sl.
b.Iterate through the alphabetically-sorted list named wordCounter by using an enumerator that is obtained by calling the
GetWordsAlphabeticallyEnumerator method.
i.For each alphabetical list entry, create a new object of type WordOccurence that is initialized with the alphabetically sorted list entry’s Value and Key.
ii.Add to sl a new entry whose key field is the new WordOccurence object and whose value field is set to null.
c.Return an enumerator of sl of type IDictionaryEnumerator.
Module 7: Strings, Arrays, and Collections |
61 |
|
|
|
|
! Modify the Application class
1.Output the words to be sorted alphabetically by adding code to the Application class’s Main method to:
a.Obtain an IDictionaryEnumerator object named de by calling the wc object’s GetWordsAlphabeticallyEnumerator method.
b.Display a message to inform the user that the output that is generated will display word usage sorted alphabetically. Also inform the user of the number of unique words.
c.Iterate over the collection and output each entry’s value and key, formatting them so that the value is displayed in the first column and the key is displayed in the second column.
2.Output the words sorted by occurrence by adding code to:
a.Assign to de an IDictionaryEnumerator object by calling the wc object’s GetWordsByOccurrenceEnumerator method.
b.Display a message to inform the user that the output that is generated will display word usage sorted by occurrence. Also inform the user of the number of unique words.
c.Iterate over the collection. For each entry, obtain the key field’s WordCounter.WordOccurrence object and output to the console the
Occurrences property of the WordCounter.WordOccurrence object in the first column and the object’s Word property in the second column.
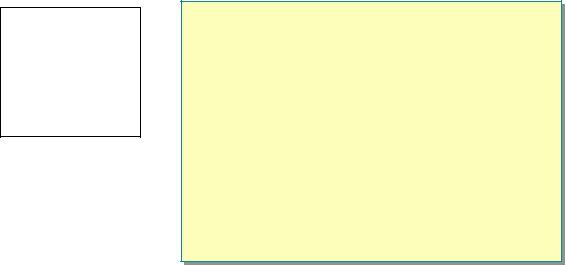

64Module 7: Strings, Arrays, and Collections
5.Create an array that contains the integers 1, 2, and 3. Then use the C# foreach statement to iterate over the array and output the numbers to the console.
int[ ] numbers = {1, 2, 3}; foreach (int i in numbers) {
System.Console.WriteLine("Number: {0}", i);
}
6.What is the name of the interface that is implemented by classes that contain an ordered collection of objects that can be individually indexed? Name the System.Collections classes that implement this interface.
The IList interface is implemented by Array, ArrayList, StringCollection, and TreeNodeCollection.
7.What is the name of the interface for collections of associated keys and values? Name the System.Collections classes that implement this interface.
The IDictionary interface is implemented by Hashtable, DictionaryBase, and SortedList.
8.Generic collection classes require runtime type-casting of their items to obtain the true type of the items in the collection classes. Name the issues raised by runtime casting.
Type-checking cannot be done at compile time. Performance overhead of casting.
In the case of collection of value types, boxing and unboxing operations.

Module 8:
Delegates and Events
Contents |
|
Overview |
1 |
Delegates |
2 |
Multicast Delegates |
12 |
Events |
21 |
When to Use Delegates, Events, and |
|
Interfaces |
31 |
Lab 8: Creating a Simple Chat Server |
32 |
Review |
42 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.