
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdfModule 6: Working with Types |
39 |
|
|
|
|
Exercise 1
Overriding System.Object Methods
In this exercise, you will create a structure that represents an office in a building. The Office structure stores the size, building number, and room number of an office. You will override appropriate methods and operators to support retrieving hash codes and comparing different offices.
You will also create a structure called OfficeUnit that is similar to the Office structure. The only difference between the OfficeUnit structure and the Office structure is that OfficeUnit represents size as an enumerated type with the values small, medium, or large. The Office structure represents size as square footage. You will write an explicit conversion operator to convert values of type Office to type OfficeUnit.
! Implement a hash code
1.Open the Offices project located in the <install folder>\Labs\ Lab06\Starter\Offices folder.
2.Open the Office.cs file.
3.Locate the Office structure.
4.Override the GetHashCode method. Generate and return a hash code by using the following algorithm:
HashCode = Building * 10000 + Room
! Provide a string representation
1.Override the ToString method.
2.Generate and return a string that contains the building number, followed by a forward slash, followed by the room number. You can use the following code to build and return the string.
return String.Concat(Building,"/",Room);
! Implement equality
1.Override the Equals method.
2.Cast the object parameter to type Office.
3.Compare the size, room, and building numbers of the parameter to this. If the values are all equal, return true. If they are not equal, return false.
4.Override the == and != operators. Implement them by calling the Equals method.

40Module 6: Working with Types
!Implement conversion
1.Define an explicit conversion operator to convert from type Office to
OfficeUnit.
2.Convert the size from square footage to an OfficeSizes value according to the following table. The OfficeSizes enumeration is already defined in the start code for you.
Square Footage |
OfficeSizes |
<= 64 |
Small |
>64 and <100 |
Medium |
>=100 |
Large |
3.Assign the Building and Room values to the new type and return an instance of the new type.
!Test
1.Open the Main.cs file.
2.Create two offices called o1 and o2. Assign different sizes, room numbers, and building numbers to each office variable.
3.Use the == operator to compare the two offices. Print the results to the console.
4.Call GetHashCode on both offices and print the results.
5.Call ToString on both offices and print the results.
6.Create an OfficeUnit and assign it one of the offices. Use explicit conversion to assign the office.
7.Print all of the values of the OfficeUnit.
8.Compile and run the program.
The two offices should not be equal. They should have different hash codes. The ToString method should print the building and room numbers, not the class names. Finally, the printed enumerated size should match the square footage appropriately.
Module 6: Working with Types |
41 |
|
|
|
|
Exercise 2
Implementing an Explicit Interface
In this exercise, you will create an interface called IRange, which provides properties and methods to select and print a range. You will also modify a class called TextEntry to inherit from IRange. This combination allows text in the TextEntry class to be selected as a range and printed.
The TextEntry class already implements a Print method. Because the IRange interface also has a Print method, you will need to explicitly implement IRange in the TextEntry class to avoid a name conflict.
! Create an explicit interface
1.Open the Interfaces project located in the <install folder>\Labs\ Lab06\Starter\Interfaces folder.
2.Open the TextEntry.cs file.
3.Define an interface called IRange that has the following properties.
Property Name |
Type |
RangeBegin |
uint |
RangeEnd |
uint |
4. Define the following methods for IRange.
Method Name |
Return Type |
Parameters |
Select |
void |
uint rb, uint re |
void |
none |
5.Modify the TextEntry class to inherit from IRange.
6.In the TextEntry class, create private fields named rangeBegin and rangeEnd to store the RangeBegin and RangeEnd data values. Use the uint type for both fields.
7.Implement the IRange RangeBegin property.
a.Implement the get accessor by returning the value of rangeBegin.
b.Implement the set accessor by storing the value in rangeBegin. Check to ensure that the new value is less than the length of the text field, and that the value of rangeEnd is not less than rangeBegin.
8.Implement the IRange RangeEnd property.
a.Implement the get accessor by returning the value of rangeEnd.
b.Implement the set accessor by storing the value in rangeEnd. Check to ensure that the new value does not exceed the length of the text field, and that the value of rangeEnd is not less than rangeBegin.
9.Implement the IRange Select method. Ensure that the re parameter is not less than the rb parameter, and that the new values are not greater than the length of the text field. Assign rb to rangeBegin and re to rangeEnd.
10.Implement the IRange Print method. Using the rangeBegin and rangeEnd fields, create a text range from the text field by using the SubString method. Then print the resulting string to the console.

42Module 6: Working with Types
!Use the interface
1.Open the Main.cs file.
2.In the MainClass class, create an instance of the TextEntry class called myEntry.
3.Create an interface of type IRange named r and cast myEntry to r.
4.Initialize the Text property on myEntry with a value, such as “The quick brown fox jumped over the gate.”
5.Select a range by using the interface variable r. For example, the values 4 and 10 will select the word “quick.”
6.Call the Print method on myEntry.
7.Call the Print method on r.
8.Compile and run the program. You should see the entire Text property printed to the console, followed by the range of text you selected.
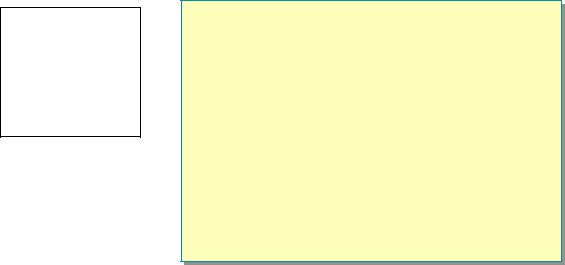

44Module 6: Working with Types
6.When should you use implicit conversions, and when should you use explicit conversions?
Use implicit conversions for improved readability and use. Use explicit conversions when the conversion could cause a loss of data or throw an exception.
7.When do boxing operations occur?
Boxing occurs when a value type is converted to an Object type. Unboxing occurs when the value is retrieved from an Object type.
8.How do you explicitly implement an interface?
Do not use the public access modifier. Use the name of the interface in front of the field or method names. For example, void IFoo.DoSomething().

Module 7: Strings,
Arrays, and Collections
Contents |
|
Overview |
1 |
Strings |
2 |
Terminology – Collections |
20 |
.NET Framework Arrays |
21 |
.NET Framework Collections |
39 |
Lab 7: Working with Strings, Enumerators, |
|
and Collections |
57 |
Review |
63 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.