
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdfModule 5: Common Type System |
iii |
|
|
|
|
Instructor Notes
Presentation:
90 Minutes
Lab:
45 Minutes
After completing this module, students will be able to:
!Describe the difference between value types and reference types.
!Explain the purpose of each element in the type system, including values, objects, and interfaces.
!Explain how object-oriented programming concepts, such as abstraction, encapsulation, inheritance, and polymorphism, are implemented in the Common Type System.
Materials and Preparation
This section provides the materials and preparation tasks that you need to teach this module.
Required Materials
To teach this module, you need the Microsoft® PowerPoint® file 2349B_05.ppt.
Preparation Tasks
To prepare for this module, you should:
!Read all of the materials for this module.
!Complete the lab.
Module Strategy
Use the following strategy to present this module:
!An Introduction to the Common Type System
Cover the basic architecture of the Common Type System. Introduce System.Object as the root type for all types in the Microsoft .NET Framework common language runtime. Explain how all types in the Common Type System are either reference types or value types.
!Elements of the Common Type System
In this section, cover the primitive types, objects, properties, and other elements of the Common Type System. The information will not be new to students with a C++ object-oriented background, so you can cover these topics quickly or omit most of the material in this section. This decision will depend on the student’s experience.
!Object-Oriented Characteristics
Discuss how the object-oriented characteristics of the .NET Framework common language runtime are supported. If the class is already experienced in object-oriented techniques, you can omit this section.
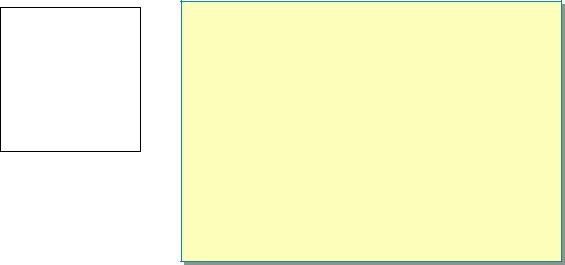
Module 5: Common Type System |
1 |
|
|
|
|
Overview
Topic Objective
To provide an overview of the module topics and objectives.
Lead-in
In this module, you will learn about the Common Type System and object-oriented programming.
!An Introduction to the Common Type System
!Elements of the Common Type System
!Object-Oriented Characteristics
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In this module, you will learn about the Common Type System. Specifically, you will learn to differentiate between value types and reference types. You will also examine how classes, interfaces, properties, methods, events, and values are represented in the Microsoft® .NET Framework.
After completing this module, you will be able to:
!Describe the difference between value types and reference types.
!Explain the purpose of each element in the type system, including values, objects, and interfaces.
!Explain how object-oriented programming concepts, such as abstraction, encapsulation, inheritance, and polymorphism, are implemented in the Common Type System.
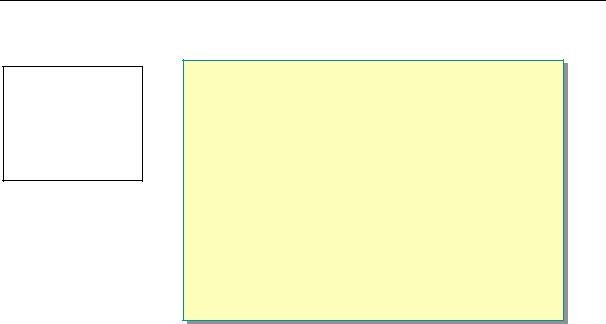
2Module 5: Common Type System
" An Introduction to the Common Type System
Topic Objective
To provide an overview of the section topics.
Lead-in
In this section, you will learn about the architecture of the Common Type System.
!Common Type System Architecture
!Value Types vs. Reference Types
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In this section, you will learn about the Common Type System architecture. Specifically, you will learn how types are specified and represented in the .NET Framework common language runtime, and you will learn the difference between value types and reference types.
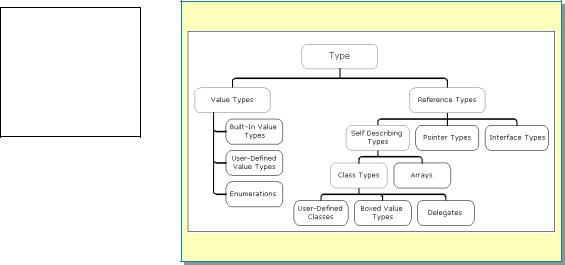
Module 5: Common Type System |
3 |
|
|
|
|
Common Type System Architecture
Topic Objective
To describe the basic architecture of the Common Type System.
Lead-in
A type defines characteristics for a set of values.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
A type defines characteristics for a set of values. For example, the set of whole numbers between negative 32768 and positive 32767 is called the short type in C#, or System.Int16 in the .NET Framework class library. The short type has a physical representation, which, in this case, is a 16-bit value. The short type also defines operations allowed on the type, such as addition and subtraction.
System.Object
System.Object is the root type for all types in the .NET Framework common language runtime. For this reason, any type you use will have System.Object as its base class. System.Object has the following methods.
Methods |
Description |
Public Equals |
Overloaded. Determines whether two Object |
|
instances are equal. |
Public GetHashCode |
Serves as a hash function for a particular type; |
|
suitable for use in hashing algorithms and data |
|
structures, such as a hash table. |
Public GetType |
Gets the type of the object. |
Public ReferenceEquals |
Determines whether the specified Object instances |
|
are the same instance. |
Public ToString |
Returns a string that represents the current object. |
Protected Finalize |
Overridden. Allows an Object to attempt to free |
|
resources and perform other cleanup operations |
|
before the Object is reclaimed by garbage collection. |
Protected MemberwiseClone |
Creates a shallow copy of the current Object. |
For more information about the methods of System.Object, see Module 6, “Working with Types,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft Visual C#™ .NET).

4Module 5: Common Type System
Value Types
Value types are any type that inherits from System.ValueType. Value types are typically simple types, such as integer values or long values. Value types are special because they are allocated on the stack.
New value types are defined by using the struct or enum keywords. You cannot create value types by using the class keyword. System.ValueType inherits from System.Object, which means that all value types can behave like
System.Object.
The type system defines primitive types, which are Byte, Int16, Int32, Int64,
Single, Double, Boolean, Char, Decimal, IntPtr, and String. There are also built-in types such as System.Array available.
Reference Types
Reference types are any type that inherits from System.Object and not System.ValueType. Reference types are analogous to pointers in C++, but there are subtle differences in the way reference types and pointers work. Reference types are allocated on the heap. Reference types also must be garbage collected. One example of a reference type is the System.IO file class, which represents a file in the system.
Fields, Methods, and Properties
The Common Type System defines fields, methods, and properties that are available to all value types and reference types. A field is a data value stored in a class. For example, Name and Age could be fields describing an employee stored in an Employee structure.
A method is an operation that can be performed on a class. For example, a Tenure method could calculate how long an employee has been employed.
Properties are a convenient way to expose data values to external objects and code. For example, the Name and Age fields could be exposed as properties, which would allow the Employee structure to control how the name and age are stored and retrieved.
Interfaces
Interfaces are the only types that do not inherit from System.Object. Interfaces have no implementation; they merely provide a description of methods, properties, and events. Any class that inherits from an interface must implement all of the methods, properties, and events in that interface. Interfaces provide a mechanism for specifying contractual relationships between classes. Interfaces also provide a means of implementing multiple inheritance. For more information about inheritance, see Module 6, “Working with Types,” in Course 2349B, Programming with the Microsoft .NET Framework (Microsoft
Visual C# .NET).
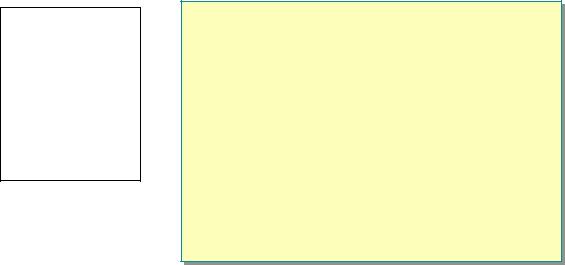
Module 5: Common Type System |
5 |
|
|
|
|
Value Types vs. Reference Types
Topic Objective
To explain the difference between value types and reference types.
Lead-in
With the exception of interfaces, all types in the Common Type System are divided into two categories: value types and reference types.
!Value Types Are Primitive or User-Defined Structures
#Allocated on stack
#Assigned as copies
#Default behavior is pass by value
!Reference Types Are Objects That Are:
#Allocated on heap using the new keyword
#Assigned as references
#Passed by reference
*****************************ILLEGAL FOR NON-TRAINER USE******************************
With the exception of interfaces, all types in the Common Type System are divided into two categories: value types and reference types.
Value Types
Value types represent primitive or user-defined structures. Value types are allocated on the stack and are removed from the stack when the method call in which they were declared returns. When a value type is passed as an argument to a method, it is passed by value, unless the ref keyword is used. All value types must inherit from System.ValueType.

6Module 5: Common Type System
An integer is an example of a value type. The following example shows an integer value type and its effect on method calls and assignment operations.
using System;
public class MainClass
{
public static void Main()
{
//Create a new integer with value 5 int a = 5;
//Create a new uninitialized integer int b;
//Initialize b by copying the value 5 into b b = a;
//Increase the value of b by 5 b += 5;
Console.WriteLine("{0}, {1}", a, b); Add5(b);
Console.WriteLine("{0}, {1}", a, b);
}
public static void Add5(int num)
{
num += 5;
}
}
This code generates the following output:
5, 10 5, 10
In the preceding example, the variables a and b represent two separate instances of the integer type. The Assignment operator (=) copies the value from one variable to another. When the Add5 method is called, the = operator updates a copy of the integer, not the original integer, because a copy of the value is pushed onto the stack when passing value types.
Module 5: Common Type System |
7 |
|
|
|
|
Reference Types
Reference types are a reference to a value. Reference types are allocated on the heap and will remain on the heap until they are garbage collected. Reference types must be allocated by using the new keyword. When you pass reference types as parameters, the reference is pushed onto the stack, and the method call works with the actual value, not with a copy of the value. All reference types inherit from System.Object.
The following example shows the effect of assignments and method calls when you use the StringBuilder class, which is a reference type:
using System; using System.Text;
public class MainClass
{
public static void Main()
{
//Create a new StringBuild instance //with the string "Bob"
StringBuilder name1 = new StringBuilder("Bob"); StringBuilder name2;
//Make name2 reference the same StringBuilder instance name2 = name1;
name2.Append("by");
Console.WriteLine("Values are {0}, {1}", name1, name2); Possessive(name2);
Console.WriteLine("Values are {0}, {1}", name1, name2);
}
public static void Possessive(StringBuilder name)
{
name.Append("'s");
}
}
This code generates the following output:
Values are Bobby, Bobby
Values are Bobby’s, Bobby’s
In this example, name1 and name2 always refer to the same StringBuilder instance on the heap. Thus, whenever a change is made to the underlying instance, both variables are affected. Also, name2 is passed by reference to the Possessive method, so that when the Possessive method makes a change to the instance, both name2 and name1 are affected.
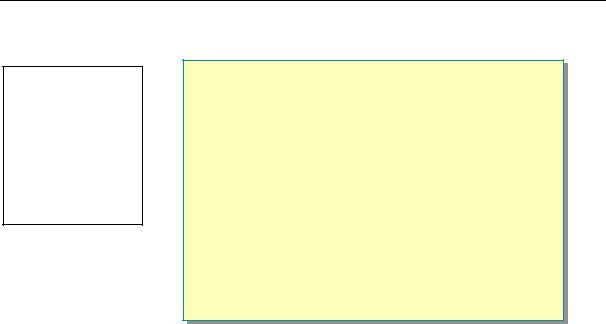
8Module 5: Common Type System
" Elements of the Common Type System
Topic Objective
To provide an overview of the section topics and objectives.
Lead-in
In this section, you will learn about primitive types, objects, properties, and other elements of the Common Type System.
!Primitive Types
!Objects
!Constructors
!Properties
!Custom Types
!Enumerations
!Interfaces
*****************************ILLEGAL FOR NON-TRAINER USE******************************
In this section, you will learn about primitive types, objects, properties, and other elements of the Common Type System.