
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf

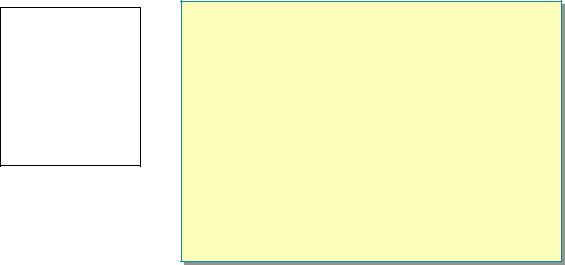
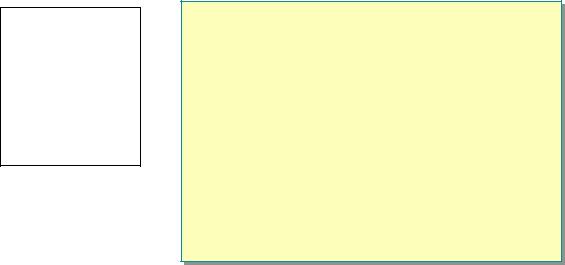

Module 7: Strings, Arrays, and Collections |
23 |
|
|
|
|
Methods and Properties of System.Array
Some of the methods and properties for the System.Array class are described in this topic. Many of these methods are used to implement the class’s interfaces.
System.Array implements the interfaces that are described in the following table.
Interface |
Use |
ICloneable |
Supports cloning, which creates a new instance of a class with the |
|
same value as an existing instance. |
IList |
Represents a collection of objects that can be individually |
|
indexed. |
ICollection |
Defines size, enumerators, and synchronization methods for all |
|
collections. |
IEnumerable |
Exposes the enumerator, which supports a simple iteration over a |
|
collection. |
The interfaces that are listed in the preceding table are supported not only by arrays but also by many of the System.Collections classes. Subsequent topics in this module discuss specific interfaces and the classes that implement them.
The following tables describe some of the public members that are available through the System.Array class.
Static Method |
Use |
BinarySearch Overloaded. Searches a one-dimensional sorted Array for a value, using a binary search algorithm.
CreateInstance Overloaded. Initializes a new instance of the Array class.
Sort Overloaded. Sorts the elements in one-dimensional Array objects.
Property |
Use |
IsFixedSize |
Gets a value that indicates whether the Array has a fixed size. |
IsReadOnly |
Gets a value that indicates whether the Array is read-only. |
Length |
Gets the total number of elements in all of the dimensions of the |
|
Array. |
Rank |
Gets the rank (number of dimensions) of the Array. |
Note The IsFixedSize and IsReadOnly properties are always false unless they are overridden by a derived class.


Module 7: Strings, Arrays, and Collections |
27 |
|
|
|
|
The following example shows a complete C# program that declares and instantiates arrays as discussed in this topic.
using System;
class DeclareArraysSample
{
public static void Main()
{
//Single-dimensional array int[] numbers = new int[5];
//Multidimensional array string[,] names = new string[5,4];
//Array-of-arrays (jagged array) byte[][] scores = new byte[5][];
//Create the jagged array
for (int i = 0; i < scores.Length; i++)
{
scores[i] = new byte[i+3];
}
// Print length of each row
for (int i = 0; i < scores.Length; i++)
{
Console.WriteLine("Length of row {0} is {1}", i, scores[i].Length);
}
}
}
The preceding program displays the following output:
Length of row 0 is 3
Length of row 1 is 4
Length of row 2 is 5
Length of row 3 is 6
Length of row 4 is 7
Initializing Arrays
C# provides simple and straightforward ways to initialize arrays when they are declared, by enclosing the initial values in curly braces ( {} ).
Important If an array is not initialized when it is declared, array members are automatically initialized to the default initial value for the array type.