
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf
Module 9: Memory and Resource Management |
51 |
|
|
|
|
! Add explicit resource management
Tip For detailed explanations about and code examples for adding explicit resource management, see The IDisposable Interface and the Dispose method in this module.
1.Modify the SentenceFormatter and SimpleTextBuffer classes to inherit from IDisposable.
2.For each of these classes, add a private member of type bool named disposed to indicate whether the object’s non-memory resources have been released. Initialize the value of disposed to false.
3.For each of these classes, add a protected virtual void Dispose method that takes a single bool parameter named disposing. If the object’s disposed flag indicates that the object has not already been disposed:
a.If the parameter disposing indicates that the object is not in finalization mode, then propagate the Dispose/Close method call through any containment hierarchies.
Tip Propagating the Dispose/Close method call through a containment hierarchy is discussed in the topic The IDisposable Interface and the Dispose Method in Module 9.
Note The SentenceFormatter should send its text buffer to its contained
SimpleTextBuffer object named aSimpleTextBuffer before it calls that
SimpleTextBuffer object’s Close method.
b. Free the object’s internal state and set disposed to indicate that the object has been disposed.
Multiple calls to protected virtual void Dispose should not throw an exception.
4.For both classes, add a public void Dispose method that takes no arguments. This method calls the protected virtual void Dispose method created in step 3 with true as its argument followed by a call to the GC class’s method to suppress finalization.
5.For both classes, modify the Close method to call the public void Dispose method.
6.For the SentenceFormatter and SimpleTextBuffer methods, which require resources that are freed by their classes’ protected virtual void Dispose method, add an initial check that throws an ObjectDisposedException if those resources have been released.
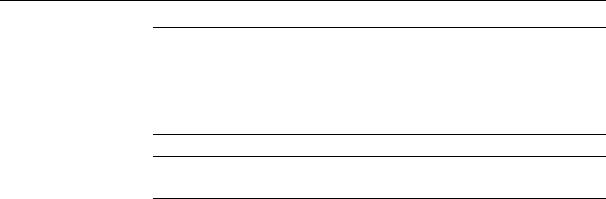
52 Module 9: Memory and Resource Management
Tip When the SentenceFormatter’s protected virtual void Dispose method is called, it frees its sentenceBuffer and aSimpleTextBuffer resources. The SentenceFormatter’s SentenceCount property does not use either of these resources and therefore does not need to check if these resources have been released. The ProcessInput method uses both these resources and therefore must check whether these resources have been released.
Note Explicit resource management will be investigated further in the next exercise.
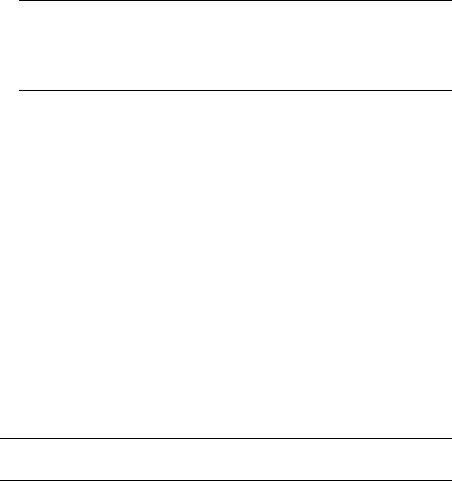
Module 9: Memory and Resource Management |
53 |
|
|
|
|
Exercise 2
Programming for Implicit Resource Management
In this exercise, you will modify the Memory and Resource Management application to incorporate implicit resource management.
! Add implicit resource management
1.Add destructors to the SentenceFormatter and SimpleTextBuffer classes.
These destructors should free only their object’s internal state by calling the protected virtual void Dispose method with false. Add code to the destructors to output to the console the name of the class and the fact that the destructor was executed.
Note Even though you have inserted code to do implicit resource management, the program is still explicitly managing its resources through the following method call in the Main method:
aSentenceFormatter.Close();
2.Build and run the Memory and Resource Management application.
As in the preceding exercise, the following text should appear in the console output:
Enter text, when finished enter an empty line
3. Enter the following text, and then enter an empty line, as prompted: hello world.are you out there?
Text similar to the following should appear in the console output:
SimpleTextBuffer:
hello world
are you out there?
Completed Sentences 1, Output Characters 29
hit enter to exit program
Note With explicit resource management, the output is identical to the output in Exercise 1. All the characters you entered are output and counted.
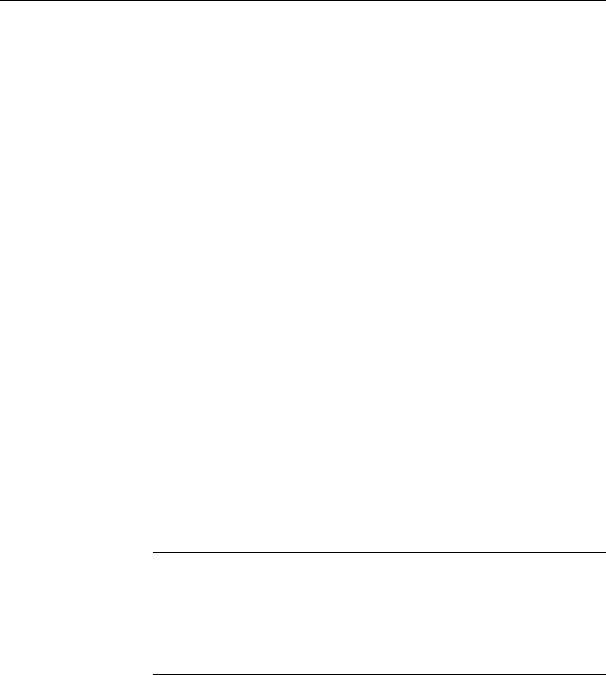
54Module 9: Memory and Resource Management
!Test implicit resource management performance
1.In the Main method, add comments to the beginning of the following line of code so that the Close method will not be invoked:
aSentenceFormatter.Close();
2.In the preceding step there is a statement after the code that outputs the sentence and character count to the console. After this Console.WriteLine statement, add code to assign the SimpleTextBuffer and SentenceFormatter objects to null, invoke garbage collection on all generations, and wait for pending finalizers.
3.Build and run the Memory and Resource Management application.
As in the preceding exercise, the following text should appear in the console output:
Enter text, when finished enter an empty line
4. Enter the following text, followed by an empty line, as prompted: hello world.are you out there?
Text similar to the following should appear in the console output:
Completed Sentences 1, Output Characters 11
SentenceFormatter destructor called
SimpleTextBuffer:
hello world
SimpleTextBuffer destructor called
hit enter to exit program
Note Observe the difference in the number of output characters when using explicit and implicit resource management. Explicit resource management outputs all the characters you entered because it propagates the Close/Dispose method call down the containment hierarchy, thereby flushing all of the buffers. Implicit resource management cannot safely do this propagation because of the nondeterministic order of destructor calls. Therefore, not all of the characters you entered are output.
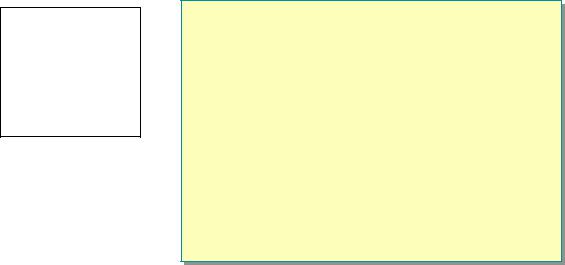

56Module 9: Memory and Resource Management
4.State the purpose of weak references.
Weak references are useful in applications that have large amounts of easily reconstructed data that should be maintained to improve performance. A weak reference allows garbage collection to collect these objects if memory in the managed heap is low.
5.Explain how and why generations are used by garbage collection.
When garbage collection is invoked to free heap space, its performance is improved because it only compacts the section of the managed heap that contains generation 0 objects. Typically, the newer an object is, the shorter its lifetime will be. Therefore, sufficient space is usually freed when generation 0 is compacted. If sufficient space cannot be obtained when generation 0 is compacted, garbage collection will compact the older generations.
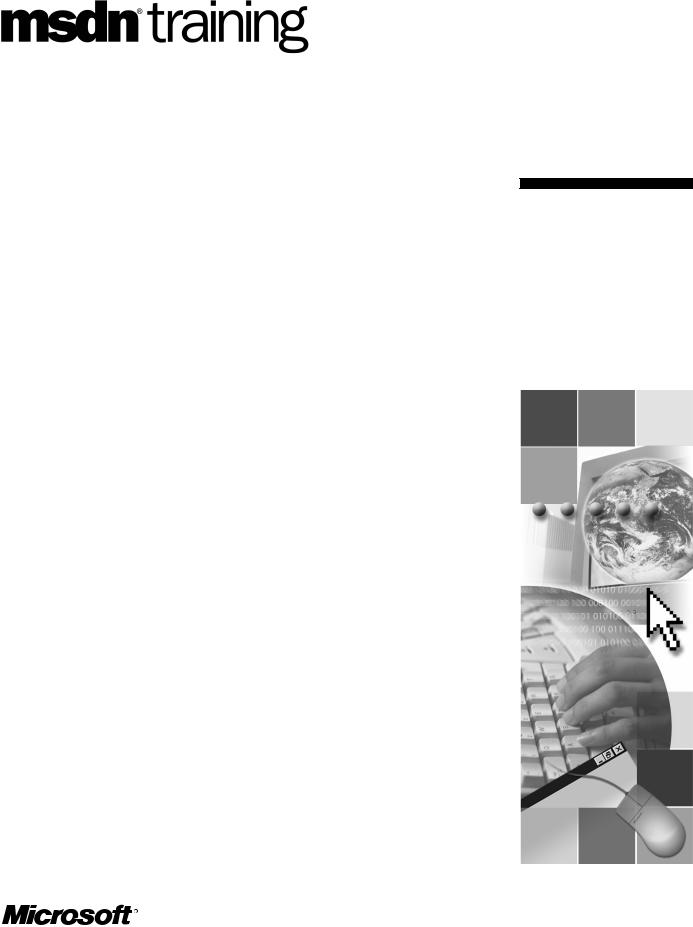
Module 10: Data
Streams and Files
Contents |
|
Overview |
1 |
Streams |
2 |
Readers and Writers |
5 |
Basic File I/O |
8 |
Lab 10: Files |
21 |
Review |
26 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.