
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf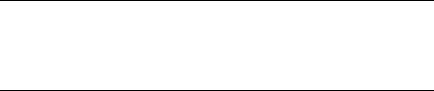
Module 12: Serialization |
21 |
|
|
|
|
Note You can open a binary file using Visual Studio .NET by running Microsoft Windows® Explorer, Explorer.exe, and navigating to the binary file’s icon. Then either right-click on the icon and choose Open With Microsoft Visual Studio .NET, or drag and drop the icon into a running Visual Studio .NET application’s left-hand File View pane.
6.Using Visual Studio .NET, open and visually examine the contents of the Linkedlist.bin file in the bin\Debug subdirectory, and note the serialized list data’s format, structure, and size.
! To create the basic Serialization application in SOAP format
1.Add code to the SaveListToDisk and LoadListFromDisk methods to use the SoapFormatter to write to and read the LinkedList parameter to and from a file named Linkedlist.soap.
2.Build and execute the application, and confirm that the console output remains correct.
3.Using Visual Studio .NET, open and visually examine the contents of the Linkedlist.soap file in the bin\Debug subdirectory, and note the serialized list data’s format and size. Compare this list data’s format, structure, and size to the Linkedlist.bin list data’s format, structure, and size.
Module 12: Serialization |
23 |
|
|
|
|
3.In the Ser class add a static public method named Scope5 that takes no arguments and returns void. Within Scope5, add code to:
a.Create two objects of type Node named n0 and n1 that are initialized to values 0 and 1 respectively.
b.Write out a message to the console that states that the application is entering Scope5 and creating a graph cycle.
c.Assign n1 to the NextNode field of n0.
d.Assign n0 to the NextNode field of n1.
e.Create an array of type Node that is initialized to contain references to the three objects: n0, n1, and n0. Note that index 0 and 2 refer to the same object.
f.Call the SaveArrayToDisk method and pass in the array of type Node.
g.Write out a message to the console that states that the application is leaving Scope5.
4.In the Ser class, add a static public method named Scope6 that takes no arguments and returns void. Within Scope6, add code to:
a.Write out a message to the console that states that the application is entering Scope6 and creating a graph cycle.
b.Assign to a variable named nodes of type array of Node the array that is returned by the LoadArrayFromDisk method. The array references the objects: n0, n1, and n0 that were created in step 1. Note that index 0 and 2 refer to the same object.
c.Write out a message to the console that states that the value of n0 is changing to 42 and that this change should result in a change in the value in both locations in the array.
d.Assign the integer 42 to the Value field of nodes[0].
e.Write out a message to the console that states that the array’s structure should be preserved during serialization and deserialization.
f.Call SaveArrayToDisk to persist nodes to the disk.
g.Assign to a variable named nodes2 of type array of Node the array that is returned by the LoadArrayFromDisk method.
h.Write out a message to the console that states that the value of n0 is being incremented.
i.Increment by 1 the value of the first element of nodes2.
j.Call SaveArrayToDisk, and pass nodes2.
k.Write out a message to the console that states that the application is leaving Scope6.
5.In the Main method of Ser, and after the call to the Scope4 method, add calls to Scope5 and Scope6.
6.Build the Serialization application.
7.Step through the application in the Visual Studio .NET debugger, and note console output similar to the following output:
Module 12: Serialization |
25 |
|
|
|
|
Entering Scope 5
Creating a circular reference:
n0's NextNode is n1 and n1's NextNode is n0
Also adding in a third node, n2, that is another reference to n0
Node 0 Value: 0 Value of NextNode Ref: 1
Node 1 Value: 1 Value of NextNode Ref: 0
Node 2 Value: 0 Value of NextNode Ref: 1
Serializing Array to file ..
Leaving Scope 5
Entering Scope 6
Deserializing Array from file ..
Node 0 Value: 0 Value of NextNode Ref: 1
Node 1 Value: 1 Value of NextNode Ref: 0
Node 2 Value: 0 Value of NextNode Ref: 1
changing value of node n0 to 42
should change value in both locations in array
array's structure should be preserved during serialization and deserialization
Node 0 Value: 42 Value of NextNode Ref: 1
Node 1 Value: 1 Value of NextNode Ref: 42
Node 2 Value: 42 Value of NextNode Ref: 1
Serializing Array to file ..
Deserializing Array from file ..
Node 0 Value: 42 Value of NextNode Ref: 1
Node 1 Value: 1 Value of NextNode Ref: 42
Node 2 Value: 42 Value of NextNode Ref: 1
incrementing value of node n0
Node 0 Value: 43 Value of NextNode Ref: 1
Node 1 Value: 1 Value of NextNode Ref: 43
Node 2 Value: 43 Value of NextNode Ref: 1
Serializing Array to file ..
Leaving Scope 6

26Module 12: Serialization
8.Using Visual Studio .NET, open and visually examine the Array.soap file in the bin\Debug subdirectory, and note the serialized array data’s format, structure, and size.
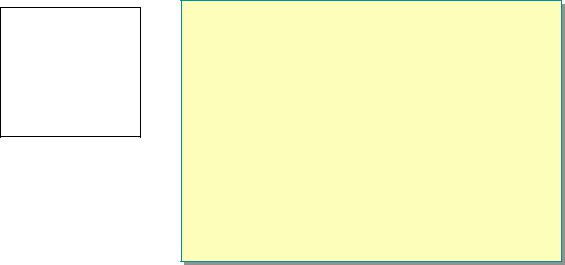

Module 13: Remoting
and XML Web Services
Contents |
|
Overview |
1 |
Remoting |
2 |
Remoting Configuration Files |
19 |
Demonstration: Remoting |
22 |
Lab 13.1: Building an Order-Processing |
|
Application by Using Remoted Servers |
28 |
XML Web Services |
36 |
Lab 13.2: Using an XML Web Service |
48 |
Review |
54 |
Course Evaluation |
56 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.