
C# ПІДРУЧНИКИ / c# / MS Press - Msdn Training Programming Net Framework With C#
.pdf
Module 11: Internet Access |
39 |
|
|
|
|
k.Close the TcpClient object.
l.Write out to a console window the following message: Press Enter to exit.
m.Do a read from the console to wait for the Enter key and exit the application.
7.Build the client application.
! Test the client and server applications
1.Ensure that there are no server applications running on the computer using port 14, for example, other instances of the server application.
Tip You can display your computer’s current TCP/IP network connections by running the program netstat in a command prompt window. The following example shows that port 7 is in use by a process with PID 1504:
C:\>netstat -o -n -a
Active Connections
Proto |
Local Address |
Foreign Address |
State |
PID |
TCP |
0.0.0.0:7 |
0.0.0.0:0 |
LISTENING |
1504 … |
2.Run the server application in a Visual Studio .NET Command Prompt window or in the Visual Studio .NET debugger.
3.Run the client application in another Visual Studio .NET Command Prompt window or in another Visual Studio .NET debugger. Do not specify any runtime arguments.
4.Note that the client application returns with the proper error message, as follows:
Please specify a server name in the command line
5.Run the client application as directed in step 3 with a runtime argument that contains a nonexistent server name. For example, assuming that there is no computer named foo on the network, enter the following text in a
Visual Studio .NET Command Prompt window:
datetimeclient foo

40Module 11: Internet Access
6.Note that the client application returns with the proper error message, for example:
Cannot find server: foo
…
7.Run the client application as directed in step 3 with a runtime argument that contains the name of the computer on which the Datetimeserver application is running. If the server application is running on your current computer, specify the local computer as follows:
datetimeclient localhost
Note that the client application outputs the number of bytes received and the current date and time string retrieved from the server.
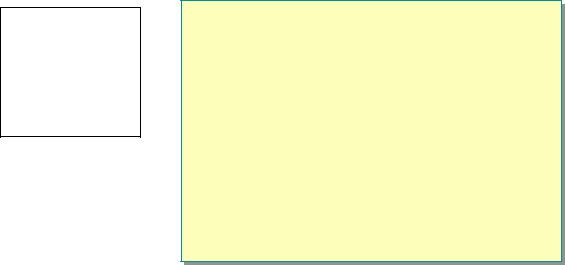

42Module 11: Internet Access
5.Name the type of Web-specific exceptions that are thrown by the
GetResponse method.
WebException
6.State how a WebRequest can be made to use the Secure Socket Layer (SSL) protocol.
URI begins with https.
7.Name at least three authentication methods that are supported by the .NET Framework.
Basic Digest Negotiate NTLM Kerberos
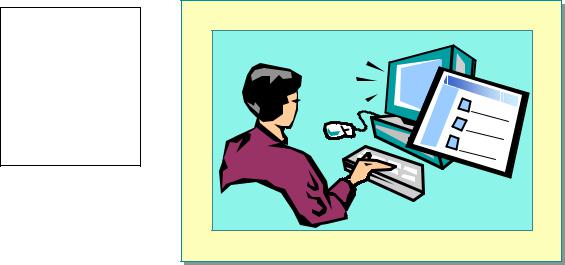
Module 11: Internet Access |
43 |
|
|
|
|
Course Evaluation
Topic Objective
To direct students to a Web site to complete a course evaluation.
Lead-in
Between now and the end of the course, you can go to the Web site listed on this page to complete a course evaluation.
*****************************ILLEGAL FOR NON-TRAINER USE******************************
Your evaluation of this course will help Microsoft understand the quality of your learning experience.
At a convenient time before the end of the course, please complete a course evaluation, which is available at http://www.metricsthatmatter.com/survey/.
Microsoft will keep your evaluation strictly confidential and will use your responses to improve your future learning experience.

Module 12: Serialization
Contents |
|
Overview |
1 |
Serialization Scenarios |
2 |
Serialization Attributes |
4 |
Object Graph |
5 |
Serialization Process |
7 |
Serialization Example |
9 |
Deserialization Example |
10 |
Custom Serialization |
12 |
Custom Serialization Example |
14 |
Security Issues |
17 |
Lab 12: Serialization |
18 |
Review |
27 |
Information in this document, including URL and other Internet Web site references, is subject to change without notice. Unless otherwise noted, the example companies, organizations, products, domain names, e-mail addresses, logos, people, places and events depicted herein are fictitious, and no association with any real company, organization, product, domain name, e-mail address, logo, person, place or event is intended or should be inferred. Complying with all applicable copyright laws is the responsibility of the user. Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual property.
2001-2002 Microsoft Corporation. All rights reserved.
Microsoft, ActiveX, BizTalk, IntelliMirror, Jscript, MSDN, MS-DOS, MSN, PowerPoint, Visual Basic, Visual C++, Visual C#, Visual Studio, Win32, Windows, Windows Media, and
Window NT are either registered trademarks or trademarks of Microsoft Corporation in the U.S.A. and/or other countries.
The names of actual companies and products mentioned herein may be the trademarks of their respective owners.