
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
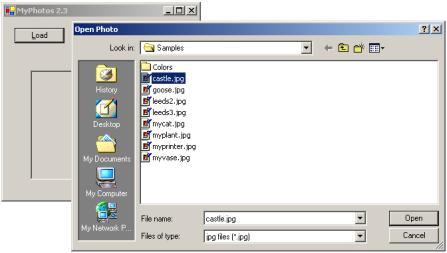
When you have finished reviewing the code, compile and run the program as before. As in chapter 1 for version 1.2 of the MyForm application, this version displays our controls but does not allow you to do anything with them. Enabling the user to load an image is our next topic.
2.3Loading files
Now that the controls are on the form, we can load an image into the PictureBox control using the OpenFileDialog class. Up until this point we really haven’t typed any C# code for our MyPhotos application. We simply set values via Visual Studio and let the environment do the work on our behalf. In this section we finally get our hands dirty. The result of our labors will allow a file to be selected as shown in figure 2.2.
Figure 2.2 The dialog used to select a file in our application. This dialog is created using the OpenFileDialog class.
There are a couple of topics worth discussing here. First we will discuss how to support the dialog shown in figure 2.2. Then we will discuss how to handle the case where the user selects an invalid file.
2.3.1Event handlers in Visual Studio .NET
As discussed in chapter 1, an event is a predefined action that a program can respond to, such as a user clicking a button or resizing a window. In chapter 1 we handled the event that occurs when the user clicks on the Load button. Here we will do the same using Visual Studio rather than a text editor.
As before, the Load button handler will allow the user to select a file and then load a Bitmap image of the file into our PictureBox control. If you recall, this involves
54 |
CHAPTER 2 GETTING STARTED WITH VISUAL STUDIO .NET |

setting a Click event handler for the button and using the OpenFileDialog class to prompt the user for an image to load.
Let’s duplicate our code from chapter 1 in Visual Studio. Our code for the event handler will be identical to that already shown and discussed, so if you skipped ahead and missed this discussion, go back to chapter 1
Set the version number of the application to 2.3.
IMPLEMENT A CLICK HANDLER FOR THE BTNLOAD BUTTON
|
Action |
Result |
|
|
|
1 |
Display the MainForm.cs |
|
|
[Design] window (the |
|
|
Windows Forms Designer |
|
|
window). |
|
|
|
|
2 |
Add a Click event handler for |
The MainForm.cs source code window is displayed with a |
|
the Load button. |
new btnLoad_Click method added. |
|
How-to |
protected void btnLoad_Click(object sender, |
|
Double-click the Load button. |
System.EventArgs e) |
|
{ |
|
|
|
|
|
|
} |
|
|
Note: Visual Studio uses the naming convention for |
|
|
all event handlers consisting of the variable name, fol- |
|
|
lowed by an underscore, followed by the event name. |
|
|
|
3 |
Add our code to handle the |
protected void btnLoad_Click(object sender, |
|
Click event. |
System.EventArgs e) |
|
|
{ |
|
How-to |
OpenFileDialog dlg = new OpenFileDialog(); |
|
Cut and paste your previous |
dlg.Title = "Open Photo"; |
|
code, or enter the code shown |
|
|
dlg.Filter = "jpg files (*.jpg)|*.jpg" |
|
|
here by hand. |
+ "|All files (*.*)|*.*"; |
|
|
if (dlg.ShowDialog() == DialogResult.OK) |
|
|
{ |
|
|
pbxPhoto.Image = new Bitmap(dlg.OpenFile()); |
|
|
} |
|
|
dlg.Dispose(); |
|
|
} |
|
|
Note: Some of these lines do not fit this table. The |
|
|
dlg.Filter line, in particular, should be a single |
|
|
string. Here and throughout the book, we will refor- |
|
|
mat the code to fit the table in a way that is equiva- |
|
|
lent to the code in the online examples. |
|
|
|
LOADING FILES |
55 |
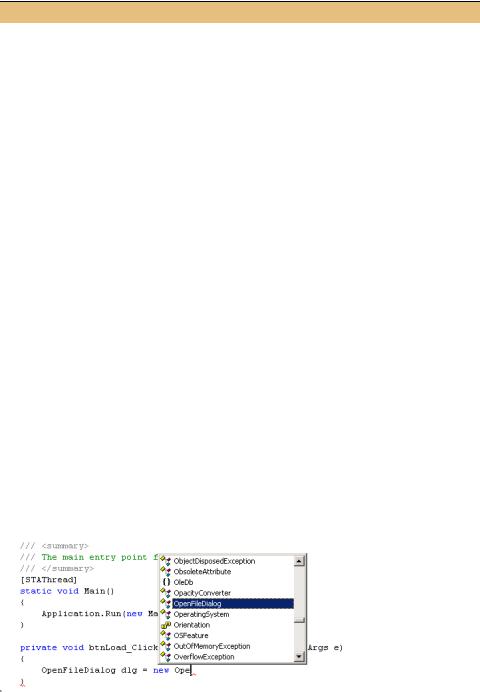
IMPLEMENT A CLICK HANDLER FOR THE BTNLOAD BUTTON (continued)
|
Action |
Result |
|
|
|
4 |
Set the SizeMode property for |
When an image is displayed, the entire image will now be |
|
the PictureBox control to |
stretched and distorted to fit within the box. |
|
StretchImage. |
Note: In the Properties window, notice how nonde- |
|
How-to |
|
|
fault properties for a control are displayed in bold |
|
|
a. Display the designer |
type. |
|
window. |
|
|
b. Right-click the PictureBox |
|
|
control. |
|
|
c. Select Properties. |
|
|
d. Locate the SizeMode prop- |
|
|
erty. |
|
|
e. Set its value to Stretch- |
|
|
Image. |
|
|
|
|
Before we discuss the code here, it is worth calling attention to the statement completion feature of Visual Studio .NET, both what it is and how to disable it. If you typed in the above code by hand, then you probably noticed how Visual Studio pops up with class member information as you type. Figure 2.3 shows what you might see after entering part of the first line of the btnLoad_Click method. After you type “new,” Visual Studio pops up a list of possible classes. The list changes to reflect the characters you type, so that after typing “Ope” the list will look something like the figure. At this point, you can press the Enter key to have Visual Studio automatically finish your typing with the highlighted entry.
Notice in this figure how Visual Studio uses a different icon for namespaces, structures, classes, and enumerations. In the figure, OleDB is a namespace, OpenFileDialog is a class, and Orientation is an enumeration type. We will not discuss these types here, other than OpenFileDialog. A structure type is not shown in this figure, but you can scroll through the list in Visual Studio to find a structure such as Point or Size.
Figure 2.3 An example of statement completion for the new keyword in Visual Studio after typing the letters “Ope.”
56 |
CHAPTER 2 GETTING STARTED WITH VISUAL STUDIO .NET |
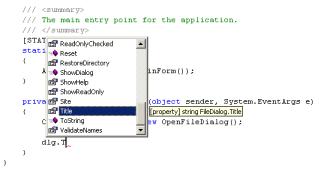
The feature applies to variables and classes as well. As another example, when you begin typing the next line to set the Title property of the dialog box, you may see something like figure 2.4. Here Visual Studio displays the class properties, methods, and events available to the dlg variable. These correspond to the members of the
OpenFileDialog class.
Once again note how Visual Studio uses different icons for different types. In the figure, ShowDialog is a method and Title is a property. You can scroll through the dialog to locate an event such as Disposed or FileOk in order to see its icon.
You will notice other statement completion popups as you type as well. One particularly nice feature is that signatures of methods are displayed as you type, and you can step through the various overloaded versions of a method using the arrow keys. In addition, as you will see in chapter 5, Visual Studio automatically picks up the classes and structures defined in your solution and incorporates them into these popup menus. Any documentation provided by <summary> tags within these classes is included as well, providing an automated forum for conveying important comments about a particular member to other programmers.
Figure 2.4
An example of statement completion for a class variable after typing the letter “T” for an OpenFileDialog class instance. Notice the small popup indicating that Title is declared as a string property in the FileDialog class.
Of course, like any feature, all these popup windows require a certain amount of CPU and system resources. If you are running Visual Studio on a slower machine, or do not want such windows popping up, you can turn statement completion off in the Options dialog box. Click the Options item under the top-level Tools menu to display this dialog. Click the Text Editor settings, select the C# item, followed by the General item. This dialog is shown in figure 2.5.
As you can see in the figure, you can disable the automatic listing of members, the display of parameter information, or both of these features. Other option settings are available here as well, of course. Feel free to look around and use the ever-ready Help button for any questions you may have.
LOADING FILES |
57 |