
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
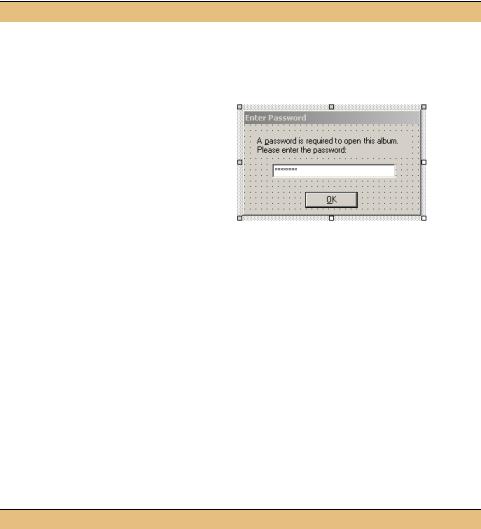
CREATE A PASSWORD DIALOG
|
|
|
Action |
|
|
Result |
|
|
|
|
|
|
|
4 |
Create a new Windows Form called |
The PasswordDlg.cs file is created and added to |
||||
|
PasswordDlg in the MyPhotoAlbum |
the project, and its design window is displayed. |
||||
|
project. |
|
|
|
|
|
|
|
|
|
|
|
|
5 |
Create the form as shown, using the |
|
||||
|
following settings. |
|
|
|
||
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
Control |
Property |
Value |
|
|
|
|
Label |
Text |
as shown |
|
|
|
|
TextBox |
(Name) |
txtPassword |
|
|
|
|
|
Password-Char |
* |
|
|
|
|
Button |
(Name) |
btnOk |
|
|
|
|
|
DialogResult |
OK |
Note: The PasswordChar property setting for |
|
|
|
|
Text |
&OK |
||
|
|
|
the TextBox control masks the user’s entry |
|||
|
|
Form |
AcceptButton |
btnOk |
||
|
|
with the given character. When this property |
||||
|
|
|
ControlBox |
False |
||
|
|
|
is set, the Clipboard cut and copy operations |
|||
|
|
|
FormBorderStyle |
FixedDialog |
are disabled (the paste operation is still per- |
|
|
|
|
ShowInTaskbar |
False |
mitted). |
|
|
|
|
Size |
262, 142 |
|
|
|
|
|
Text |
Enter |
|
|
|
|
|
|
Password |
|
|
|
|
|
|
|
|
|
6 |
Create a Password property to retrieve |
public string Password |
||||
|
the value of the txtPassword control. |
{ |
||||
|
|
|
|
|
|
get { return txtPassword.Text; } |
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
We will use this dialog to request a password when an album is opened. The point here is to illustrate the PasswordChar property, and not to create a secure mechanism for handling passwords.
ENFORCE (INSECURE) PASSWORD MECHANISM
|
Action |
Result |
|
|
|
7 |
In the PhotoAlbum.cs code |
using System.Windows.Forms; |
|
window, indicate that this |
|
|
class uses the Windows |
|
|
Forms namespace. |
|
|
|
|
BUTTON CLASSES |
297 |

ENFORCE (INSECURE) PASSWORD MECHANISM (continued)
|
Action |
Result |
|
|
|
8 |
Use the new PasswordDlg |
public void Open(string fileName) |
|
form in the |
{ |
|
PhotoAlbum.Open method |
. . . |
|
try |
|
|
to receive a password from |
|
|
{ |
|
|
the user. |
. . . |
|
|
// Check for password |
|
|
if (_password != null && _password.Length > 0) |
|
|
{ |
|
|
using (PasswordDlg dlg = new PasswordDlg()) |
|
|
{ |
|
|
dlg.Text = String.Format( |
|
|
"Opening album {0}", |
|
|
Path.GetFileName(_fileName)); |
|
|
if ((dlg.ShowDialog() == DialogResult.OK) |
|
|
&& (dlg.Password != _password)) |
|
|
{ |
|
|
throw new ApplicationException( |
|
|
"Invalid password provided"); |
|
|
} |
|
|
} |
|
|
} |
|
|
. . . |
|
|
} |
|
|
|
Our PhotoAlbum class is now ready. Each PhotoAlbum instance supports the new title, password, and display option settings. These settings are saved in the album file, and in the Open method an album cannot be loaded unless the proper password is provided.3
Let’s get back to the matter at hand and create our Album Properties dialog.
9.3.3CREATING THE ALBUMEDITDLG PANEL AREA
With our new infrastructure in place, we are ready to create the AlbumEditDlg form. This section will inherit the new dialog from our base form, and add controls to the panel at the top of the form.
3 Of course, the password mechanism here is quite insecure. A user can examine the album file by hand in order to discern the password. We could make this scheme more secure by using the password as a scrambling mechanism on the file. For example, rather than storing the password string in the file, call GetHashCode on the password string and XOR each character in the file with this hash code and the byte-sum of the password characters. The scrambled result is then stored in the file. The validity of the password is checked by using it to unscramble the version number of the file to see if it makes sense. If so, then the password is presumed to be valid. Again, this is not a totally secure mechanism, but it is slightly better then that shown in the text. For more information on security in .NET in general and the System.Security namespace in particular, see the book .NET Security by Tom Cabanski from Manning Publications.
298 |
CHAPTER 9 BASIC CONTROLS |

The Panel control inherited from the BaseEditDlg form will display the album file and the title in our new AlbumEditDlg form. The following steps create the new form class and the controls contained by the panel.
CREATE THE ALBUMEDITDLG FORM AND ITS PANEL CONTROLS
|
|
|
|
Action |
|
|
Result |
|
|
|
|
|
|
|
|
1 |
Derive a new AlbumEditDlg form |
|
|||||
|
from the BaseEditDlg form. |
|
|||||
|
|
|
|
Settings |
|
|
|
|
Set the Text property for the new |
|
|||||
|
dialog to “Album Properties.” |
|
|||||
|
|
|
|
|
|
|
|
2 |
Add two labels to the left side of |
The new controls are contained by the Panel control, |
|||||
|
the form. |
|
|
|
rather than by the Form object. |
||
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
Label |
|
Property |
Value |
|
|
|
|
label1 |
|
Text |
Album &File |
|
|
|
|
|
|
TextAlign |
MiddleRight |
|
|
|
|
label2 |
|
Text |
&Title |
|
|
|
|
|
|
TextAlign |
MiddleRight |
|
|
|
|
|
|
|
|
|
|
3 |
Add the two text boxes to the |
|
|||||
|
form. Resize and position the |
|
|||||
|
controls and the panel as shown |
|
|||||
|
in the graphic. |
|
|
|
|||
|
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
TextBox |
Property |
Value |
|
||
|
|
Album File |
(Name) |
txtAlbumFile |
|
||
|
|
|
|
ReadOnly |
True |
|
|
|
|
|
|
Text |
|
|
|
|
|
Title |
(Name) |
txtTitle |
|
||
|
|
|
|
Text |
|
|
|
|
|
|
|
|
|
|
|
BUTTON CLASSES |
299 |

With these steps completed, we are ready to add our button controls.
9.3.4USING RADIO BUTTONS
The bottom part of our dialog will contain some radio and check box buttons. We will begin with the radio buttons in the middle of the form.
Radio buttons display a set of mutually exclusive options. In our case, they indicate which Photograph setting should be used to represent the photograph in a main window. Radio buttons in .NET work much the same as radio buttons in other graphical environments. An overview of this class appears in .NET Table 9.6.
In our code, three radio buttons will collectively set the _displayOption setting for the album. Since these are the only radio buttons on the form, it is not necessary to group them within a container control. We will anyway, since it improves the overall appearance of the form.
.NET Table 9.6 RadioButton class
The RadioButton class represents a button that displays one of a possible set of options. Radio buttons are usually grouped together, and only one button may be checked at any one time. By default, when a radio button is clicked, it is automatically selected and all radio buttons in the same group are deselected. This behavior can be disabled using the AutoCheck property.
The parent container for this control defines its group. So if four radio buttons are contained within a form, then only one of the four buttons may be checked at any one time. Use container classes such as GroupBox and Panel to provide multiple independent groups of radio buttons on a single form.
This control is part of the System.Windows.Forms namespace, and inherits from the ButtonBase class. See .NET Table 9.4 on page 292 for members inherited from this class.
|
Appearance |
Gets or sets whether the control appears as a |
|
|
normal radio button or as a toggle button. |
|
AutoCheck |
Gets or sets the behavior of related radio buttons |
|
|
when this button is clicked. If true, then the |
|
|
framework automatically deselects all radio |
Public Properties |
|
buttons in the same group; if false, other radio |
|
buttons in the same group must be deselected |
|
|
|
|
|
|
manually. |
|
CheckAlign |
Gets or sets the alignment of the click box |
|
|
portion of the control. |
|
Checked |
Gets or sets whether the control is checked. |
|
|
|
Public Methods |
PerformClick |
Sends a Click event to the control. |
|
|
|
|
AppearanceChanged |
Occurs when the Appearance property |
Public Events |
|
changes. |
|
|
|
|
CheckedChanged |
Occurs when the Checked property changes. |
|
|
|
A Panel control could be used here, of course, but this is a good opportunity to create a GroupBox control. The GroupBox class inherits directly from the Control class to
300 |
CHAPTER 9 BASIC CONTROLS |