
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index

Our check box example does have one drawback. If the user clicks the check box, then he or she is forced to enter a password before leaving the txtAlbumPwd control. This could be a little frustrating if the user then wishes to uncheck the check box. We alleviate this a little by providing a default text string in the txtAlbumPwd control. From a book perspective, this was a good place to demonstrate the Focus method and validation events, so we will allow this little design anomaly to remain. In practice, an alternative might be to ensure that the password is nonempty as part of the
ValidPasswords method.
This completes our discussion of check boxes. The last step here is to add the logic to reset and save our dialog box values, and display the form from our MyPhotos application.
9.3.6Adding AlbumEditDlg to our main form
The final task required so that we can see our AlbumEditDlg dialog in action is to handle the reset and save logic required and link the dialog into our application. Let’s make this happen.
FINISH THE ALBUMEDITDLG FORM
|
Action |
Result |
|
|
|
1 |
In the AlbumEditDlg.cs source |
private PhotoAlbum _album; |
|
window, add a private |
|
|
PhotoAlbum variable to hold the |
|
|
album to edit. |
|
|
|
|
2 |
Modify the constructor to accept |
public AlbumEditDlg(PhotoAlbum album) |
|
a PhotoAlbum parameter. |
{ |
|
|
|
3 |
Within the constructor, set the |
. . . |
|
album variable and call |
// Initialize the dialog settings |
|
ResetSettings to initialize the |
_album = album; |
|
ResetSettings(); |
|
|
dialog’s controls. |
|
|
} |
|
|
|
|
310 |
CHAPTER 9 BASIC CONTROLS |

FINISH THE ALBUMEDITDLG FORM (continued)
|
Action |
Result |
|
|
|
4 |
Implement ResetSettings to |
protected override void ResetSettings() |
|
set the controls to their |
{ |
|
corresponding settings in the |
// Set file name |
|
txtAlbumFile.Text = _album.FileName; |
|
|
current photograph. |
|
|
|
|
|
How-to |
// Set title, and use in title bar |
|
this.txtTitle.Text = _album.Title; |
|
|
a. Assign the album file name and |
|
|
this.Text = String.Format( |
|
|
title text boxes. |
"{0} - Album Properties", |
|
b. Place the album title in the title |
txtTitle.Text); |
|
|
|
|
bar as well. |
// Set display option values |
|
c. Set the radio buttons based on |
_selectedDisplayOption |
|
= _album.DisplayOption; |
|
|
the DisplayOption setting for |
|
|
switch (_selectedDisplayOption) |
|
|
the album. |
{ |
|
d. Check the check box button if |
case PhotoAlbum.DisplayValEnum.Date: |
|
this.rbtnDate.Checked = true; |
|
|
the album contains a non- |
|
|
break; |
|
|
empty password. |
|
|
e. Assign both password text |
case PhotoAlbum.DisplayValEnum.FileName: |
|
this.rbtnFileName.Checked = true; |
|
|
boxes to the current password. |
|
|
break; |
|
|
|
case PhotoAlbum.DisplayValEnum.Caption: |
|
|
default: |
|
|
this.rbtnCaption.Checked = true; |
|
|
break; |
|
|
} |
|
|
string pwd = _album.Password; |
|
|
cbtnPassword.Checked |
|
|
= (pwd != null && pwd.Length > 0); |
|
|
txtAlbumPwd.Text = pwd; |
|
|
txtConfirmPwd.Text = pwd; |
|
|
} |
|
|
|
5 |
Implement the SaveSettings |
protected override bool SaveSettings() |
|
method to store the results after |
{ |
|
the user has clicked OK. |
bool valid = ValidPasswords(); |
|
|
|
|
How-to |
if (valid) |
|
{ |
|
|
a. Use the ValidPasswords |
|
|
_album.Title = txtTitle.Text; |
|
|
method to verify the pass- |
_album.DisplayOption |
|
words settings. |
= this._selectedDisplayOption; |
|
b. Store the new settings only if |
if (cbtnPassword.Checked) |
|
the passwords were valid. |
_album.Password = txtAlbumPwd.Text; |
|
c. Return whether the settings |
else |
|
_album.Password = null; |
|
|
were successfully stored. |
|
|
} |
|
|
|
return valid; |
|
|
} |
|
|
|
6 |
Add a TextChanged event |
private void txtTitle_TextChanged |
|
handler to the txtTitle control |
(object sender, System.EventArgs e) |
|
to update the title bar as the title |
{ |
|
this.Text = String.Format( |
|
|
text is modified. |
|
|
"{0} - Album Properties", |
|
|
|
txtTitle.Text); |
|
|
} |
|
|
|
BUTTON CLASSES |
311 |
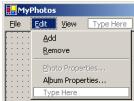
This completes the dialog. Now let’s invoke this dialog from our main application window.
Set the version number of the MyPhotos application to 9.3.
|
|
|
|
DISPLAY THE ALBUMEDITDLG FORM |
||
|
|
|
|
|
|
|
|
|
|
Action |
Result |
||
|
|
|
|
|
|
|
7 |
In the MainForm.cs [Design] |
|
||||
|
window, add a new Album |
|
||||
|
Properties menu item to the |
|
||||
|
Edit menu. |
|
||||
|
|
|
Settings |
|
||
|
|
|
|
|
|
|
|
|
Property |
|
Value |
|
|
|
|
(Name) |
|
menuAlbumProp |
|
|
|
|
Text |
|
A&lbum |
|
|
|
|
|
|
Properties |
|
|
|
|
|
|
|
|
|
8 |
Add a Click handler for this |
private void menuAlbumProp_Click |
||||
|
menu to display the |
(object sender, System.EventArgs e) |
||||
|
AlbumEditDlg form. |
{ |
||||
|
using (AlbumEditDlg dlg |
|||||
|
|
|
|
|
|
|
|
|
|
|
|
|
= new AlbumEditDlg(_album)) |
|
|
|
|
|
|
{ |
|
|
|
|
|
|
if (dlg.ShowDialog() |
|
|
|
|
|
|
== DialogResult.OK) |
|
|
|
|
|
|
{ |
|
|
|
|
|
|
// Update window with changes |
|
|
|
|
|
|
this._bAlbumChanged = true; |
|
|
|
|
|
|
SetTitleBar(); |
|
|
|
|
|
|
this.Invalidate(); |
|
|
|
|
|
|
} |
|
|
|
|
|
|
} |
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
9 |
Also, make use of the new |
protected override void OnPaint(. . .) |
||||
|
CurrentDisplayText |
{ |
||||
|
if (_album.Count > 0) |
|||||
|
property in the OnPaint |
|||||
|
{ |
|||||
|
method. |
|
|
|
||
|
|
|
|
. . . |
||
|
|
|
|
|
|
// Update the status bar. |
|
|
|
|
|
|
sbpnlFileName.Text |
|
|
|
|
|
|
= _album.CurrentDisplayText; |
|
|
|
|
|
|
. . . |
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
And we are finished. Compile and run the application to display properties for an album. Note the following aspects of the Album Properties dialog:
•This dialog can be displayed for an empty album, as opposed to the Photo Properties dialog, which requires at least one photograph in the album in order to appear.
•The title bar updates as the title changes.
•The radio buttons receive focus as a group. If you use the Tab key to move through the form, this is readily apparent. Note how the arrow keys can be used to modify the selected radio button from the keyboard.
312 |
CHAPTER 9 BASIC CONTROLS |