
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
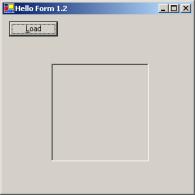
1.1.6Program execution
Before we leave this section, let’s review what we’ve learned about how our program executes within the operating system. Run the MyForm.exe program again to see this in action. When you execute this program, the Windows operating system creates and initializes a process that:
1Uses the Main method as the entry point for execution, which:
a Instantiates an instance of the class MyForm using the new keyword, which b Invokes the instance constructor for MyForm, which
c Assigns the string “Hello Form” to the title bar.
2Back in our Main method, the Application.Run method is called with the
newly created MyForm object as a parameter, and:
aDisplays MyForm as the application window, and
bWaits for and processes any messages or user interactions that occur.
3When the application window closes:
a The Application.Run method returns, and b The Main method returns, and
c The program exits.
And that is how it is done in the world of .NET.
1.2Adding controls
Let’s make our program a little more interesting by adding some controls. Throughout the course of the book, we will be building a photo viewing application, so let’s add a button for loading an image file, and a box where the image can be displayed. When we are done, our form will look like figure 1.3.
Figure 1.3
The main window shown here contains a Load button and a picture box control.
Revise your code as shown in listing 1.2. Changes from our previous code listing are shown in bold. Note that we have changed the version number of our program to 1.2
ADDING CONTROLS |
13 |
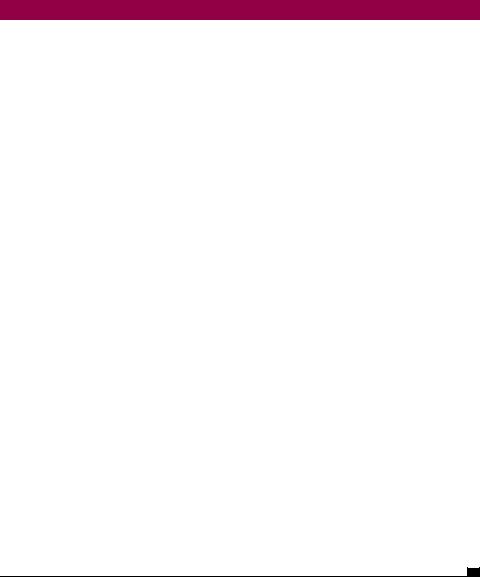
to distinguish it from our original code and to match the current section. This new version number is also displayed on the title bar. In chapter 2 we will see how to obtain the application’s version number programmatically. For now, changing it by hand will do just fine.
Listing 1.2 A Button and PictureBox control are added to the form
[assembly: System.Reflection.AssemblyVersion("1.2")]
namespace MyNamespace
{
using System;
using System.Windows.Forms;
public class MyForm : Form
{
private Button btnLoad; private PictureBox pboxPhoto;
public MyForm()
{
this.Text = "Hello Form 1.2";
//Create and configure the Button btnLoad = new Button(); btnLoad.Text = "&Load"; btnLoad.Left = 10;
btnLoad.Top = 10;
//Create and configure the PictureBox pboxPhoto = new PictureBox(); pboxPhoto.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D; pboxPhoto.Width = this.Width / 2; pboxPhoto.Height = this.Height / 2;
pboxPhoto.Left = (this.Width - pboxPhoto.Width) / 2; pboxPhoto.Top = (this.Height - pboxPhoto.Height) / 2;
//Add our new controls to the Form this.Controls.Add(btnLoad); this.Controls.Add(pboxPhoto);
}
public static void Main()
{
Application.Run(new MyForm());
}
}
}
Compile this program as before and run it to see our changes. We will walk through these changes one at a time.
14 |
CHAPTER 1 GETTING STARTED WITH WINDOWS FORMS |
1.2.1Shortcuts and fully qualified names
The first change you may notice in our new code is the using keyword at the begin-
ning of the program.
using System;
using System.Windows.Forms;
Programmers are always looking for shortcuts; and older programmers, some would say more experienced programmers, often worry that their lines may be too long for the compiler or printer to handle. The programmers at Microsoft are no exception, so while one team probably agreed that fully-qualified names are a good idea, another team probably sought a way to avoid typing them. The result is the using keyword.
The using keyword actually plays two roles in C#. The first is as a directive for specifying a shortcut, as we are about to discuss. The second is as a statement for ensuring that non-memory resources are properly disposed of. We will discuss the using keyword as a statement in chapter 6.
As a directive, using declares a namespace or alias that will be used in the current file. Do not confuse this with include files found in C and C++. Include files are not needed in C# since the assembly incorporates all of this information, making the /reference switch to the compiler sufficient in this regard. This really is just a shortcut mechanism.
In our original program in section 1.1, the Main function called the method
System.Windows.Forms.Application.Run. In our new listing the using directive allows us to shorten this call to Application.Run. The long form is called the fully qualified name since the entire namespace is specified. Imagine if you had to use the fully qualified name throughout your code. Aside from tired fingers, you would have long, cluttered lines of code. As a result, our new code is a bit easier to read:
public static void Main()
{
Application.Run(new MyForm());
}
Since Application is not a C# keyword or a globally available class, the compiler searches the System and System.Windows.Forms namespaces specified by the using directive in order to locate the System.Windows.Forms.Application class.
You can also specify an alias with the using keyword to create a more convenient representation of a namespace or class. For example,
using WF-alias = System.Windows.Forms
With this alias defined, you can then refer to the Application class as
WF-alias.Application.Run(new MyForm());
ADDING CONTROLS |
15 |

Alternatively, an alias for a specific type can be created. For example, a shortcut for the Application class can be defined with:
using MyAppAlias = System.Windows.Forms.Application
This would permit the following line in your code:
MyAppAlias.Run(new MyForm());
Typically, the using directive simply indicates the namespaces employed by the program, and this is how we use this directive in our program. For example, rather than the fully qualified names System.Windows.Forms.Button and tem.Windows.Forms.PictureBox, we simply use the Button and PictureBox names directly.
It is worth noting that there is also a Button class in the System.Web.UI.WebControls namespace. The compiler uses the correct System.Windows.Forms.Button class because of the using keyword, and because the System.Web namespace is not referenced by our program.
When we look at Visual Studio .NET in chapter 2, you will see that Visual Studio tends to use the fully qualified names everywhere. This is a good practice for a tool that generates code to guarantee that any potential for ambiguity is avoided.
1.2.2Fields and properties
Let’s go back to our use of the Button and PictureBox classes. The top of our class now defines two member variables, or fields in C#, to represent the button and the picture box on our form. Here, Button and PictureBox are classes in the Windows Forms namespace that are used to create a button and picture box control on a
Form. We will tend to use the terms class and control interchangeably for user interface objects in this book.3
public class MyForm : Form
{
private Button btnLoad;
private PictureBox pboxPhoto;
Fields, like all types in C#, must be initialized before they are used. This initialization occurs in the constructor for the MyForm class.
public MyForm()
{
// Create and configure the Button btnLoad = new Button(); btnLoad.Text = "&Load"; btnLoad.Left = 10;
btnLoad.Top = 10;
3Or, more formally, we will use the term control to refer to an instance of any class derived from the
Control class in the System.Windows.Forms namespace.
16 |
CHAPTER 1 GETTING STARTED WITH WINDOWS FORMS |

// Create and configure the PictureBox pboxPhoto = new PictureBox();
pboxPhoto.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D; pboxPhoto.Width = this.Width / 2;
pboxPhoto.Height = this.Height / 2;
pboxPhoto.Left = (this.Width - pboxPhoto.Width) / 2; pboxPhoto.Top = (this.Height - pboxPhoto.Height) / 2;
. . .
Note the use of the new keyword to initialize our two fields. Each control is then assigned an appropriate appearance and location. You might think that members such as Text, Left, BorderStyle, and so on are all public fields in the Button and PictureBox classes, but this is not the case. Public member variables in C++, as well as in C#, can be a dangerous thing, as these members can be manipulated directly by programmers without restrictions. A user might accidentally (or on purpose!) set such a variable to an invalid value and cause a program error. Typically, C++ programmers create class variables as protected or private members and then provide public access methods to retrieve and assign these members. Such access methods ensure that the internal value never contains an invalid setting.
In C#, there is a class member called properties designed especially for this purpose. Properties permit controlled access to class fields and other internal data by providing read, or get, and write, or set, access to data encapsulated by the class. Examples later in the book will show you how to create your own properties. Here we use properties available in the Button and PictureBox classes.4
We have already seen how the Text property is used to set the string to appear on a form’s title bar. For Button objects, this same property name sets the string that appears on the button, in this case “&Load.” As in previous Windows programming environments, the ampersand character ‘&’ is used to specify an access key for the control using the Alt key. So typing Alt+L in the application will simulate a click of the Load button.
Windows Forms controls also provide a Left, Right, Top, and Bottom property to specify the location of each respective side of the control. Here, the button is placed 10 pixels from the top and left of the form, while the picture box is centered on the form.
The Width and Heightproperties specify the size of the control. Our code creates a picture box approximately 1/2 the size of the form and roughly centered within it. This size is approximate because the Width and Height properties in the Form class actually represent the width and height of the outer form, from edge to edge.5
4As we will see in later chapters, the properties discussed here are inherited from the Control class.
5The ClientRectangle property represents the size of the internal display area, and could be used here to truly center the picture box on the form.
ADDING CONTROLS |
17 |