
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
The Control class extends the Component class we saw in chapter 3. All controls are components, and therefore support the IComponent and IDisposable interfaces. Controls can act as containers for other controls, although not all controls actually do so. The premier example of such a container is the Form class, which we have been using for our application window all along. The class hierarchy for the Form class is discussed in chapter 7.
All controls are also disposable. When you are finished with a control, you should call the Dispose method inherited from the Component class to clean up any nonmemory resources used by the control.
The Control class forms the basis for all windows controls in .NET, and provides many of the properties, methods, and events we have already seen such as the Left, Top, Width, and Height properties, the Invalidate method, and the Click event. An overview of the Control class is provided in .NET Table 4.1. Note that only a portion of the many members of this class are shown in the table. Consult the online documentation for the complete list of members.
The StatusBar class is just one of many controls derived from the Control class. We will look at the StatusBar class in more detail in a moment, and other control classes throughout the rest of the book.
4.2THE STATUSBAR CLASS
Now that we have seen the class hierarchy, let’s turn our attention to the StatusBar class itself. Typically, an application has only one status bar, although its contents may change as the application is used in different ways. Two types of information are normally displayed on a status bar.
•Simple text—the status bar can display a text string as feedback on the meaning of menu commands and toolbars. This is often referred to as flyby text since it displays as the cursor moves over, or flies by, the associated control. A simple string can also display status information on what the application is currently doing. For example, we will use the status bar to display a message while the application is loading a selected image. On a slower machine or for a large image, this will tell our user that the application is busy and currently unavailable.
•State or attribute information—another type of data often provided is relevant information about the application or an object displayed by the application. This information is usually divided into separate areas called status bar panels (or status bar indicators or panes). Such information can include both text and graphical data. In this chapter, we will use a status bar panel to display the image size in pixels of the displayed image.
This section will implement the first type of information to display the status bar shown in figure 4.3. As before, this chapter builds on the application constructed in the previous chapter.
THE STATUSBAR CLASS |
105 |
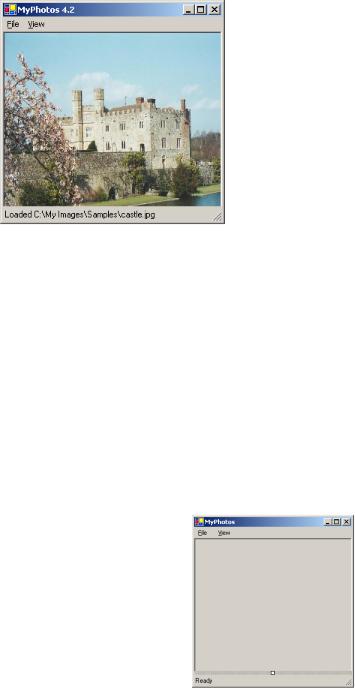
Figure 4.3
The status bar shown here uses the Text property of the StatusBar class to display a string to the user.
4.2.1ADDING A STATUS BAR
As you might expect, a status bar can be added to our application in Visual Studio by dragging one from the Toolbox window onto the form.
Set the version number of the application to 4.2.
|
|
ADD A STATUS BAR |
|
|
|
|
Action |
Result |
|
|
|
1 |
Place a status bar at the base |
The new status bar appears in the designer window. For lack |
|
of the MyPhotos application. |
of a better choice, we’ll use the default name statusBar1. |
|
How-to |
|
|
a. Display the MainForm.cs |
|
|
[Design] window. |
|
|
b. Drag a StatusBar object |
|
|
from the Toolbox window |
|
|
onto the form. |
|
|
|
|
2 |
Set the Text property for the |
|
|
StatusBar control to |
|
|
“Ready.” |
|
|
|
|
106 |
CHAPTER 4 STATUS BARS |
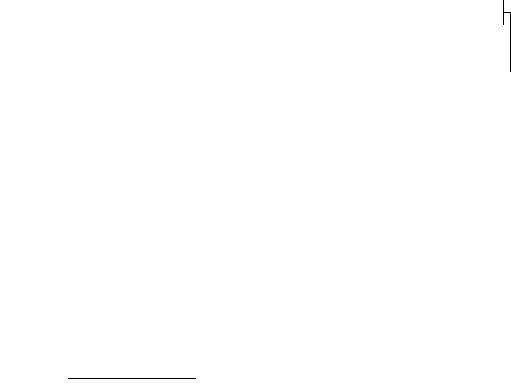
Before we interact with our new status bar, let’s take a look at the code so far. An excerpt of the code in our MainForm.cs source file is shown below.
. . .
private System.Windows.Forms.StatusBar statusBar1;
. . .
private void InitializeComponent()
{
. . .
this.statusBar1 = new System.Windows.Forms.StatusBar();
. . .
//
// statusBar1
//
this.statusBar1.Location = new System.Drawing.Point(0, 233); this.statusBar1.Name = "statusBar1";
this.statusBar1.Size = new System.Drawing.Size(292, 20);
this.statusBar1.TabIndex = 2; |
b Set the tab order |
this.statusBar1.Text = "Ready"; |
for status bar |
. . . |
|
pbxPhoto.Dock = System.Windows.Forms.DockStyle.Fill; |
|
. . . |
|
this.Controls.AddRange(new System.Windows.Forms.Control[] { |
|
|
this.statusBar1, |
|
this.pbxPhoto}); |
} |
Add the status |
|
bar before the |
|
picture boxc |
This looks very similar to code we have seen before. As usual, though, there are some points worth highlighting.
bThis line is a little strange. You do not normally tab into a status bar, so why set a tab index? Visual Studio does this to ensure that each control has a unique index, but it does not mean that you can tab to the status bar control. By default, the StatusBar sets the TabStop property (inherited from the Control class) to false. So the status bar is not a tab stop (by default), even though Visual Studio sets a TabIndex for it.
cIf you recall, the order in which controls are added establishes the z-order stack (which controls are in front or behind the others). This is important here since the pbxPhoto control takes up the entire window (with Dock set to Fill). By adding the status bar first, this insures this control is on top, docked first, and therefore visible. In the Forms
Designer window, you can right-click a control to select the Bring to Front or Send to Back item and modify the z-order.1 You might try this to verify that the status bar is hidden if you run the application with pbxPhoto at the top of the z-order.
1You can also change the order in which controls are added by rearranging their order in the InitializeComponent method. While Microsoft recommends against this, it does work.
THE STATUSBAR CLASS |
107 |

d Set the Dock property I know, there is no number 3 in the code. I’m just trying to see if you’re paying attention. The default setting for the Dock property in the Control class is DockStyles.None. The StatusBar class overrides this setting to use DockStyles.Bottom by default. This ensures the status bar appears docked at the bottom of the form. Since this is the default, Visual Studio does not set this value in the code, so there is no number 3.
A summary of the StatusBar class is shown in .NET Table 4.2. One feature noticeably missing from the StatusBar class is flyby text. In the MFC classes, menu and toolbar objects can set help messages that appear in the status bar as the cursor passes over the corresponding control. This feature may well be included in a future release of the .NET Framework.
.NET Table 4.2 StatusBar class
The StatusBar class is a control used to show a status bar on a form. This class can display either a textual string or a collection of panels in the form of StatusBarPanel objects. Whether the text or panels appear is determined by the value of the ShowPanels property. The StatusBar class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited from the Control class, and .NET Table 4.3 on page 116 for more information on the StatusBarPanel class.
|
Dock |
Gets or sets the dock setting for the control. The |
|
(inherited from |
default value for status bars is DockStyles.Bottom. |
|
|
|
|
Control) |
|
|
Panels |
Gets the StatusBarPanelCollection class |
|
|
containing the set of StatusBarPanel objects |
|
|
managed by this status bar. |
|
ShowPanels |
Gets or sets whether the panels (if true) or text (if |
|
|
false) should be displayed on the status bar. |
Public Properties |
|
Defaults to false. |
|
|
|
|
SizingGrip |
Gets or sets whether a sizing grip should be |
|
|
displayed in the corner of the status bar. This grip can |
|
|
be used to resize the form. Defaults to true. |
|
TabStop |
Gets or sets whether the control is a tab stop on the |
|
(inherited from |
form. The default value for status bars is false. |
|
Control) |
|
|
Text |
Gets or sets the text for the status bar. This is |
|
(inherited from |
displayed on the status bar only if ShowPanels is set |
|
Control) |
to false. |
|
|
|
|
DrawItem |
Occurs when an owner-drawn status bar panel must |
Public Events |
|
be redrawn. |
|
|
|
|
PanelClick |
Occurs when a panel on the status bar is clicked. |
|
|
|
108 |
CHAPTER 4 STATUS BARS |