
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index

14.5.7REDISPLAYING THE ALBUMS
As a final change, we need to give our user the opportunity to redisplay the album view. We may as well provide a menu to display the photo view as well, as an alternative to double-clicking on the album.
ALLOW USER SELECTION OF THE KIND OF OBJECT TO DISPLAY
|
|
|
Action |
|
|
Result |
|
|
|
|
|
|
|
|
|
1 |
|
In the MainForm.cs [Design] |
|
||||
|
|
window, add three menu items |
|
||||
|
|
to the bottom of the View menu. |
|
||||
|
|
|
Settings |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Menu |
Property |
|
Value |
|
|
|
|
separator |
Text |
|
- |
|
|
|
|
Albums |
(Name) |
|
menuAlbums |
|
|
|
|
|
Text |
|
&Albums |
|
|
|
|
Photos |
(Name) |
|
menuPhotos |
|
|
|
|
|
Text |
|
&Photos |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
2 |
|
Add a Click handler for the |
private void menuAlbums_Click |
||||
|
|
Albums menu. |
|
|
(object sender, System.EventArgs e) |
||
|
|
|
|
|
|
|
{ |
|
|
|
|
|
|
|
// Display albums in the list |
|
|
|
|
|
|
|
if (!_albumsShown) |
|
|
|
|
|
|
|
{ |
|
|
|
|
|
|
|
LoadAlbumData(PhotoAlbum.DefaultDir); |
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
3 |
|
Add a Click handler for the |
private void menuPhotos_Click |
||||
|
|
Photos menu. |
|
|
(object sender, System.EventArgs e) |
||
|
|
Note: This is the same as acti- |
{ |
||||
|
|
// Activate the selected album |
|||||
|
|
vating an album item. |
listViewMain_ItemActivate(sender, e); |
||||
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
4 |
|
Update the Popup handler for |
private void menuView_Popup |
||||
|
|
the View menu to enable or |
(object sender, System.EventArgs e) |
||||
|
|
disable the Photos menu as |
{ |
||||
|
|
View v = listViewMain.View; |
|||||
|
|
appropriate. |
|
|
|||
|
|
|
|
. . . |
|||
|
|
|
|
|
|
|
if (_albumsShown && listViewMain. |
|
|
|
|
|
|
|
SelectedItems.Count > 0) |
|
|
|
|
|
|
|
menuPhotos.Enabled = true; |
|
|
|
|
|
|
|
else |
|
|
|
|
|
|
|
menuPhotos.Enabled = false; |
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
14.6RECAP
This completes our discussion of the ListView class. In this chapter we discussed list views in detail, and created a new MyAlbumExplorer interface to display the collection of albums available in our default album directory. We supported all four possible views available in a ListView control, and provided support for column
RECAP |
483 |
sorting, item selection, and label editing. We finished by implementing this same support for the photos in an album, so that our application can display albums or photographs in the control.
Along the way we looked at a number of classes provided to support this control, most notably the ListViewItem, ListViewItem.ListViewSubItem, and ColumnHeader classes. We also examined the IComparer interface as a way to define how two objects should be compared, and implemented a class supporting this interface in order to sort the columns in our detailed view of the list.
The next chapter looks at a close cousin to the ListView class, namely the
TreeView control.
484 |
CHAPTER 14 LIST VIEWS |

C H A P T E R |
1 5 |
|
|
Tree views
15.1 |
Tree view basics 486 |
15.4 |
Node selection 505 |
15.2 |
The TreeView class 486 |
15.5 |
Fun with tree views 513 |
15.3 |
Dynamic tree nodes 497 |
15.6 |
Recap 524 |
In the previous chapter we created the MyAlbumExplorer application incorporating a ListView control. This program presents the default set of photo albums available and the collection of photographs contained within these albums.
In this chapter we extend this program to include a TreeView control in order to present a more traditional explorer-style interface. Specific topics we will cover in this chapter include the following:
•Exploring the TreeView class.
•Using the Splitter control to divide a container.
•Populating a tree with the TreeNode class, both in Visual Studio and programmatically.
•Selecting nodes in a tree.
•Editing the labels for a tree.
•Integrating a ListView and TreeView control into an application.
As we did for list views, we begin this chapter with a general discussion of tree views and a discussion of the terms and classes used for this control.
485
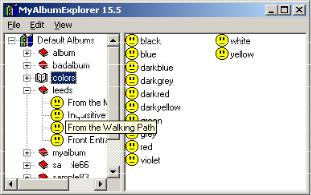
15.1TREE VIEW BASICS
The TreeView class is a close cousin of the ListView class. List views display a collection as a list, while tree views display collections as a tree. Each item in a tree view is called a tree node, or just a node. Tree nodes can contain additional nodes, called child nodes, to arbitrary levels in order to represent a hierarchy of objects in a single control. Various elements of a TreeView control are illustrated in figure 15.1.
|
b An icon taken from an |
|
ImageList instance is |
|
associated with each node. |
b |
c An alternate icon from the |
c |
ImageList can be displayed |
|
when a node is selected. |
d |
d The primary text |
associated with each |
|
|
node is called the |
|
node label. |
e |
e The TreeNode class |
represents a single |
|
|
element, or node, |
|
in the list. |
Figure 15.1 The TreeView control automatically shows the entire label in a tool tip style format when the mouse hovers over a node, as was done for the “From the Walking Path” entry in this figure.
The explorer-style interface shown in the figure and used by other applications such as Windows Explorer is a common use of the TreeView and ListView classes. In this chapter we build such an interface by extending the MyAlbumExplorer project constructed in chapter 14.
15.2THE TREEVIEW CLASS
The TreeView class is summarized in.NET Table 15.1. Like the ListView class, this class inherits directly from the Control class, and provides an extensive list of members for manipulating the objects displayed by the tree.
486 |
CHAPTER 15 TREE VIEWS |

.NET Table 15.1 TreeView class
The TreeView class represents a control that displays a collection of labeled items as a treestyle hierarchy. Typically an icon is displayed for each item in the collection to provide a graphical indication of the nature or purpose of the item. Items in the tree are referred to as nodes, and each node is represented by a TreeNode class instance. This class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited by this class.
|
CheckBoxes |
Gets or sets whether check boxes are displayed next to each |
|
|
|
node in the tree. The default is false. |
|
|
HideSelection |
Gets or sets whether a selected node remains highlighted |
|
|
|
even when the control does not have focus. |
|
|
ImageIndex |
Gets or sets an index into the tree’s image list of the default |
|
|
|
image to display by a tree node. |
|
|
ImageList |
Gets or sets an ImageList to associate with this control. |
|
|
LabelEdit |
Gets or sets whether node labels can be edited. |
|
|
Nodes |
Gets the collection of TreeNode objects assigned to the |
|
Public |
|
control. |
|
Properties |
PathSeparator |
Gets or sets the delimiter used for a tree node path, and in |
|
|
|||
|
|
particular the TreeNode.FullPath property. |
|
|
SelectedNode |
Gets or sets the selected tree node. |
|
|
ShowPlusMinus |
Gets or sets whether to indicate the expansion state of |
|
|
|
parent tree nodes by drawing a plus ‘+’ or minus ‘-‘ sign next |
|
|
|
to each node. The default is true. |
|
|
Sorted |
Gets or sets whether the tree nodes are sorted alphabetically |
|
|
|
based on their label text. |
|
|
TopNode |
Gets the tree node currently displayed at the top of the tree |
|
|
|
view control. |
|
|
|
|
|
|
CollapseAll |
Collapses all the tree nodes so that no child nodes are visible. |
|
Public |
GetNodeAt |
Retrieves the tree node at the specified location in pixels |
|
|
within the control. |
||
Methods |
|
||
|
|
||
|
GetNodeCount |
Returns the number of top-level nodes in the tree, or the total |
|
|
|
number of nodes in the entire tree. |
|
|
|
|
|
|
AfterExpand |
Occurs after a tree node is expanded. |
|
|
AfterLabelEdit |
Occurs after a tree node label is edited. |
|
Public |
BeforeCollapse |
Occurs before a tree node is collapsed. |
|
Events |
|||
|
|
||
|
BeforeSelect |
Occurs before a tree node is selected. |
|
|
ItemDrag |
Occurs when an item is dragged in the tree view. |
|
|
|
|
A TreeView object is created much like any other control in Visual Studio .NET: you simply drag the control onto the form. In our MyAlbumExplorer application, we already have a ListView on our form, so it looks like all we need to add is a tree view in order to support the interface shown in figure 15.2.
THE TREEVIEW CLASS |
487 |