
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index

P A R T 3
Advanced Windows Forms
If you have actually read this book from the beginning, then I applaud your fortitude and welcome you to the third and final part of this book. For those readers who have jumped directly to this page, I would encourage you to actually read the earlier chapters, as they build a foundation for much of the discussion that will occur in this part of the book. Of course, if you are browsing this book with the idea of buying it, then feel free to look around.
In part 3 we look at what might be considered advanced topics. If you have a firm, or at least decent, grasp of the material from part 2 of this book, then this section should be quite understandable.
Chapter 14 kicks off our discussion with the topic of “List views.” This chapter creates a new MyAlbumExplorer application incorporating a ListView control, and demonstrates various means of displaying and interacting with objects in this control.
Chapter 15 on “Tree views” extends the MyAlbumExplorer application to support a standard explorer-style interface. The Splitter and TreeView controls are discussed, and various interactions between the ListView and TreeView controls in the MyAlbumExplorer application are examined.
Chapter 16 turns to the topic of “Multiple document interfaces.” This chapter discusses the support provided by the .NET Framework for multiple document interface, or MDI, applications in Windows Forms. Here we return to our MyPhotos application from part 2 and convert it into an MDI application, using our MainForm class as the child window.
The topic of “Data binding” is taken up in chapter 17. This discusses complex data binding by way of the DataGrid control, and simple binding of data to Windows Forms controls in general. This chapter will illustrate how to provide transactional updates within a class and automatically invoke these updates from a bound control. A new MyAlbumData application is constructed over the course of this chapter.
Chapter 18 is called “Odds and ends .NET,” and completes our discussion with a review of various topics that should be of further interest. These include printing, Windows Forms timers, drag and drop, and ActiveX controls. An example for each topic is provided using the MyPhotos MDI application built in chapter 16.
Following this last chapter are four appendices with some additional information on C#, an overview of .NET namespaces, a class hierarchy chart of the Windows Forms namespace, and resources for additional information on C# and the .NET Framework.
438 |
PART 3 ADVANCED WINDOWS FORMS |

C H A P T E R |
1 4 |
|
|
List views
14.1 |
The nature of list views 440 |
14.4 |
Selection and editing 464 |
14.2 |
The ListView class 443 |
14.5 |
Item activation 472 |
14.3 |
ListView columns 453 |
14.6 |
Recap 483 |
To kick off the advanced section of the book, we take a detailed look at the ListView class. This class is used by applications such as Windows Explorer to present a collection of items in list form. We will examine this class in detail, including the following topics:
•Various styles supported by the ListView class.
•Members of the ListView class.
•Defining list view columns in Visual Studio and programmatically.
•Selecting and editing items in the list.
•Activating list view items.
•Dynamically switching the contents of a list view.
We will start from scratch here and build a new application called MyAlbumExplorer. In this chapter we will display both albums and photographs in the main window. The next chapter will add support for a TreeView control to this application to create a window much like the Windows Explorer application utilizes for file system objects.
439
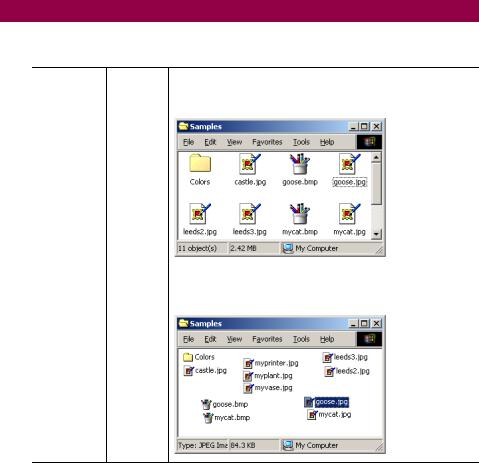
14.1THE NATURE OF LIST VIEWS
In many ways, a list view is a more glamorous version of a list box. Other than the fact that they are both controls, there is no relation from a class hierarchy perspective, but conceptually both present a scrollable list to the user. The ListBox class stores a collection of object instances, while the ListView class contains a collection of ListViewItem instances, which in turn contains a collection of ListViewSubItem objects.
Another difference is how their contents are displayed. The ListBox control displays a string associated with each object by default, and supports an owner-drawn style to display other formats. The ListView control displays its items in one of four views represented by the View enumeration, as described by .NET Table 14.1. When the Details view is displayed, the collection of subitems appears in a configured set of ColumnHeader objects associated with the control.
.NET Table 14.1 View enumeration
The View enumeration specifies the different ways the contents of a ListView control can appear. This enumeration is part of the System.Windows.Forms namespace. The following table provides an example obtained from the Windows Explorer application.
LargeIcon Each item appears as a large icon with a label below it. By default, items can be dragged around and placed at any location within the control.
Enumeration |
|
Values |
SmallIcon Each item appears as a small icon with a label at the right. By |
|
default, items can be dragged and placed at any location in the |
|
control. |
440 |
CHAPTER 14 LIST VIEWS |
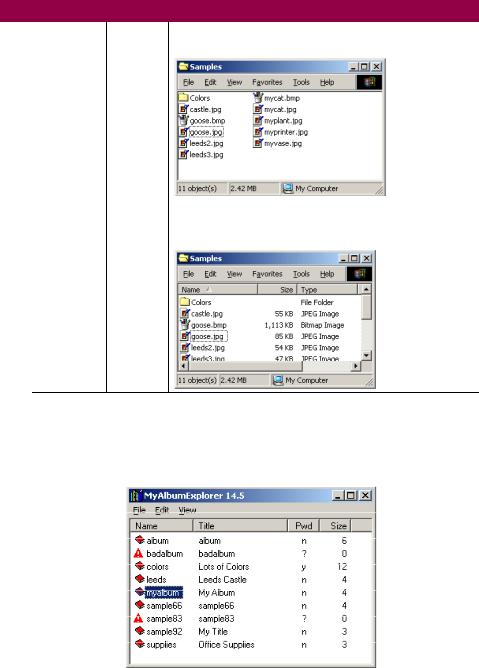
.NET Table 14.1 View enumeration
List |
Items are arranged as small icons, in columns with no headers, |
|
and with the labels on the right. |
Enumeration |
Details |
Items are arranged in columns with headers. Items appear as |
|
Values |
|||
|
small icons with the labels on the right, and additional |
||
|
|
||
|
|
information about each item appears in the columns. |
Figure 14.1 shows a Form with a ListView control displayed in the Details view mode. This figure illustrates various features and classes used by this control. We will look at these in detail as we progress through the chapter.
An icon taken from an ImageList instance is associated with each item.
The primary text associated with each item is called the item label.
The ListViewItem class represents a single item in the list.
Multiple instances of the
ListViewSubItem class represent additional information associated with each item.
The ColumnHeader class represents a single column for the list.
b
f
c
d b
c
e
d
f e
Figure 14.1 This graphic illustrates important classes and terms used for the ListView control.
THE NATURE OF LIST VIEWS |
441 |

.NET Table 14.2 ListView class
The ListView class is a control that displays a collection of labeled items as a list in one of four different views. Typically an icon is displayed for each item in the collection to provide a graphical indication of the nature or purpose of the item. Items can be displayed with large icons, small icons, in a list format, or in a detailed list format. The detailed list permits additional information about each item to appear in columns within the control. This class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited by this class.
|
Activation |
Gets or sets how an item is activated, and whether the font |
|
|
|
changes as the mouse passes over the item. |
|
|
CheckBoxes |
Gets or sets whether a check box is displayed next to each |
|
|
|
item. Default is false. |
|
|
Columns |
Gets the collection of ColumnHeader components |
|
|
|
associated with the control. |
|
|
HeaderStyle |
Gets or sets the column header style for the control. |
|
|
|
Default is ColumnHeaderStyle.Clickable. |
|
|
Items |
Gets the collection of items in the list. |
|
|
LabelEdit |
Gets or sets whether the user can edit item labels in the |
|
|
|
list. Default is false. |
|
Public |
LargeImageList |
Gets or sets the ImageList for the LargeIcon view. |
|
Properties |
|||
ListViewItemSorter |
Gets or sets an IComparer interface to use when sorting |
||
|
|||
|
|
items in the list. |
|
|
MultiSelect |
Gets or sets whether multiple items in the list may be |
|
|
|
selected at the same time. Default is false. |
|
|
SelectedItems |
Gets the collection of items selected in the list. |
|
|
SmallImageList |
Gets or sets the ImageList instance for the views other |
|
|
|
than the LargeIcon view. |
|
|
Sorting |
Gets or sets how items in the list are sorted, if at all. |
|
|
StateImageList |
Gets or sets the ImageList list for state icons. |
|
|
View |
Gets or sets the current View enumeration value for the |
|
|
|
list. Default is LargeIcon. |
|
|
|
|
|
Public |
Clear |
Removes all items and columns from the list view control. |
|
EnsureVisible |
Ensures a given item is visible, scrolling it into view if |
||
Methods |
|||
|
necessary. |
||
|
|
||
|
|
|
|
|
AfterLabelEdit |
Occurs after an item label has been edited. |
|
|
ColumnClick |
Occurs when the user clicks a column header in the |
|
|
|
Details view. |
|
Public |
ItemActivate |
Occurs when an item is activated. How this occurs |
|
Events |
|
depends on the Activation property. |
|
|
ItemDrag |
Occurs when a user begins dragging an item in the list. |
|
|
SelectedIndex- |
Occurs when the selection state of an item changes. |
|
|
Changed |
|
|
|
|
|
442 |
CHAPTER 14 LIST VIEWS |