
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index

on a form using the DateTimePicker class, as summarized in .NET Table 11.3. This class displays a date and/or time to the user, and allows the user to change the values from the keyboard or from a dropdown calendar control. The dropdown calendar is based on the MonthCalendar class, which we will examine in the next section.
.NET Table 11.3 DateTimePicker class
The DateTimePicker class represents a date and/or time value on a form. It allows the user to select a specific date and/or time, and presents this selection in a specified format. The DateTime value is presented in a text box control, with a down arrow providing access to a calendar from which an alternate date can be selected. The various parts of the DateTime value can alternately be modified using an up-down button or the arrow keys on the keyboard.
This class is part of the System.Windows.Forms namespace, and inherits from the Control class. See .NET Table 4.1 on page 104 for a list of members inherited from this class.
|
CalendarFont |
Gets or sets the Font to apply to the calendar |
|
|
portion of the control. |
|
CalendarForeColor |
Gets or sets the foreground color for the |
|
|
calendar. |
|
Checked |
When the ShowCheckBox property is true, gets |
|
|
or sets whether the check box is checked. |
|
CustomFormat |
Gets or sets the custom date-time format. |
|
Format |
Gets or sets how the date-time value is |
|
|
formatted in the control. |
Public Properties |
MaxDate |
Gets or sets the maximum date-time value for |
|
||
|
|
the control. |
|
MinDate |
Gets or sets the minimum date-time value for |
|
|
the control. |
|
ShowCheckBox |
Gets or sets whether a check box displays to the |
|
|
left of the selected date. |
|
ShowUpDown |
Gets or sets whether an up-down control is used |
|
|
to adjust the date-time value. |
|
Value |
Gets or sets the DateTime value assigned to the |
|
|
control. Default is the current date and time. |
|
|
|
|
CloseUp |
Occurs when the dropdown calendar is |
|
|
dismissed and disappears. |
Public Events |
DropDown |
Occurs when the dropdown calendar is shown. |
|
|
|
|
FormatChanged |
Occurs when the Format property changes. |
|
ValueChanged |
Occurs when the Value property changes. |
|
|
|
11.3.1DATES AND TIMES
Our Photo Properties dialog with a DateTimePicker control in place is shown in figure 11.5. As you can see, the dropdown calendar control is displayed for the object.
DATES AND TIMES |
367 |

Figure 11.5
The DateTimePicker shown here displays the Long date format, which is the default.
We can add this control to our dialog using the following steps. We will begin with the default display settings for this control, and look at how to modify these settings later in the section.
Set the version number of the MyPhotoAlbum application to 11.3.
REPLACE THE DATE TEXT BOX WITH A DATETIMEPICKER CONTROL
|
|
|
Action |
Result |
||
|
|
|
|
|
|
|
1 |
In the PhotoEditDlg.cs [Design] |
|
||||
|
window, delete the TextBox control |
|
||||
|
next to the Date Taken label. |
|
||||
|
|
|
|
|
|
|
2 |
Place a DateTimePicker control |
Note: The location of this control is shown in |
||||
|
where the text box used to be. |
figure 11.5. |
||||
|
|
Settings |
|
|||
|
|
|
|
|
|
|
|
|
Property |
|
Value |
|
|
|
|
(Name) |
|
dtpDateTaken |
|
|
|
|
TabIndex |
|
5 |
|
|
|
|
|
|
|
|
|
3 |
Locate the ResetSettings method |
protected override void ResetSettings() |
||||
|
in the MainForm.cs source file. |
{ |
||||
|
|
|
|
|
|
// Initialize the ComboBox settings |
|
|
|
|
|
|
. . . |
|
|
|
|
|
|
|
4 |
Set the Value property for the date |
Photograph photo = _album.CurrentPhoto; |
||||
|
and time control. |
if (photo != null) |
||||
|
How-to |
|
|
|
||
|
|
|
|
{ |
||
|
Use the DateTaken property. |
txtPhotoFile.Text = photo.FileName; |
||||
|
|
|
|
|
|
txtCaption.Text = photo.Caption; |
|
|
|
|
|
|
dtpDateTaken.Value = photo.DateTaken; |
|
|
|
|
|
|
cmbxPhotographer.SelectedItem |
|
|
|
|
|
|
= photo.Photographer; |
|
|
|
|
|
|
txtNotes.Text = photo.Notes; |
|
|
|
|
|
|
} |
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
368 |
CHAPTER 11 MORE CONTROLS |
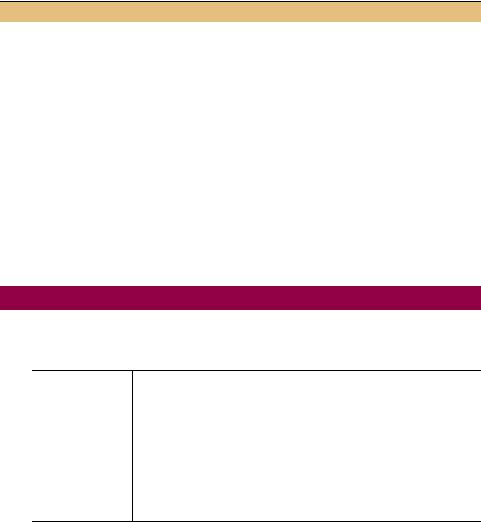
REPLACE THE DATE TEXT BOX WITH A DATETIMEPICKER CONTROL (continued)
|
Action |
Result |
|
|
|
5 |
Locate the SaveSettings method. |
protected override void SaveSettings() |
|
|
{ |
|
|
|
6 |
Set the DateTaken property to the |
Photograph photo = _album.CurrentPhoto; |
|
date-time value specified by the user. |
if (photo != null) |
|
|
|
|
|
{ |
|
|
photo.Caption = txtCaption.Text; |
|
|
photo.DateTaken = dtpDateTaken.Value; |
|
|
photo.Photographer |
|
|
= cmbxPhotographer.Text; |
|
|
photo.Notes = txtNotes.Text; |
|
|
} |
|
|
} |
|
|
|
And there you have it. One DateTimePicker control ready to work. Compile and run the application, and set the dates for your photographs as appropriate. Make sure your albums preserve the selected date after exiting and restarting the program.
.NET Table 11.4 DateTimePickerFormat enumeration
The DateTimePickerFormat enumeration specifies how to display a date-time value in a
DateTimePicker control. This enumeration is part of the System.Windows.Forms namespace. For each value, the default setting for the U.S. English culture is provided. The format codes used here correspond to the codes supported by the DateTimeFormatInfo class.
Enumeration
Values
Custom |
A custom format is used, as specified by the |
|
CustomFormat property. |
Long |
The long date format is used. In Windows, this is typically |
|
“dddd, MMMM dd, yyyy” for U.S. English environments. |
|
This is the default value. |
Short |
The short date format is used. In Windows, this is |
|
typically “MM/dd/yyyy” for U.S. English environments. |
Time |
The time format is used. In Windows, this is typically |
|
“HH:mm:ss tt” for U.S. English environments. |
|
|
You may have noticed that our control does not display the time. By default, the date and time control displays what .NET calls the long date. This includes the day of the week and month written out in the local language as well as the two-digit day and four-digit year. The format used by the control is specified by the Format property, using the DateTimePickerFormat enumeration described in .NET Table 11.4. As you can see from the table, various values allow either the date or time to be displayed in a format specified by the operating system.
11.3.2CUSTOMIZING A DATETIMEPICKER CONTROL
As can be seen in .NET Table 11.4, a custom display setting for the DateTimePicker control is used when the Format property is set to DateTimePicker-
DATES AND TIMES |
369 |
Format.Custom. The CustomFormat property contains the string value to use in this case. A number of format codes are available within this string. These codes are managed by the sealed DateTimeFormatInfo class. The following table shows a number of these codes, along with some corresponding properties in the DateTimeFormatInfo class, which can be especially useful when operating in a multi-language environment. Consult the .NET documentation for the complete list of codes and additional information on the specified properties.
Date-time codes for the DateTimeFormatInfo class
Pattern |
Description |
Default U.S. English |
DateTimeFormatInfo |
|
Values |
Property |
|||
|
|
|||
|
|
|
|
|
d |
Day of the month. |
1 to 31 |
|
|
dd |
Two-digit day of the month. |
01 to 31 |
|
|
ddd |
Abbreviated day of the week. |
Sun to Sat |
AbbreviatedDayNames |
|
dddd |
Full day of the week. |
Sunday to Saturday |
DayNames |
|
M |
Numeric month. |
1 to 12 |
|
|
MM |
Two-digit numeric month. |
01 to 12 |
|
|
MMM |
Abbreviated month name. |
Jan to Dec |
AbbreviatedMonthNames |
|
MMMM |
Full month name. |
January to December |
MonthNames |
|
y |
Year without century. |
1 to 99 |
|
|
yy |
Two-digit year without century. |
01 to 99 |
|
|
yyyy |
Four-digit century. |
0001 to 9999 |
|
|
gg |
Period or era, if any. |
B.C. or A.D. |
|
|
h |
Hour on a 12-hour clock. |
1 to 12 |
|
|
hh |
Two-digit hour on a 12-hour clock. |
01 to 12 |
|
|
H |
Hour on a 24-hour clock. |
1 to 24 |
|
|
HH |
Two-digit hour on a 24-hour clock. |
01 to 24 |
|
|
m |
Minute. |
0 to 59 |
|
|
mm |
Two-digit minute. |
00 to 59 |
|
|
s |
Second. |
0 to 59 |
|
|
ss |
Two-digit second. |
00 to 59 |
|
|
tt |
AM/PM designator. |
AM or PM |
AMDesignator and |
|
|
|
|
PMDesignator |
|
: |
Default time separator. |
: ( a colon) |
TimeSeparator |
|
/ |
Default date separator. |
/ (a slash) |
DateSeparator |
|
‘c’ |
Displays the specified character. For |
|
|
|
|
example, ‘s’ will display the |
|
|
|
|
character s rather than the number |
|
|
|
|
of seconds. |
|
|
|
|
|
|
|
Let’s modify our date and time control to display a customized value. We will include both the date and time in the display.
370 |
CHAPTER 11 MORE CONTROLS |