
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
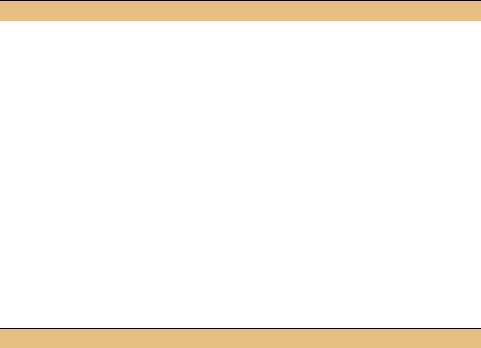
Before we do, note that we can make immediate practical use of our caption in the MainForm class. The sbpnlFileName status bar panel has previously displayed the entire path to the file, which may not fit when the window is small. The photo’s caption seems like a much better choice here.
Set the version number of the MyPhotos application to 8.3.
DISPLAY THE CAPTION VALUE IN THE STATUS BAR
|
Action |
Result |
|
|
|
6 |
Locate the OnPaint |
protected override void OnPaint |
|
method in the |
(PaintEventArgs e) |
|
MainForm.cs source code. |
{ |
|
. . . |
|
|
|
|
|
|
|
7 |
Modify the |
if (_album.Count > 0) |
|
sbpnlFileName status bar |
{ |
|
panel to display the |
. . . |
|
// Update the status bar. |
|
|
caption. |
|
|
sbpnlFileName.Text = photo.Caption; |
|
|
|
. . . |
|
|
} |
|
|
. . . |
|
|
} |
|
|
|
8.3.2Preserving caption values
Our new caption values must be saved whenever an album is saved to disk, and loaded when an album is opened. To do this, we need to create a new version of our album file, while still preserving the ability to read in our existing files. Fortunately, we established a version number for these files in chapter 6, so the changes required are not too extensive. First, let’s look at the changes to our Save method.
UPDATE THE SAVE METHOD TO STORE CAPTIONS
|
Action |
Result |
|
|
|
1 |
In the PhotoAlbum.cs file, |
private const int _CurrentVersion = 83; |
|
modify the version |
|
|
constant to be 83. |
|
|
|
|
2 |
Modify our foreach loop in |
public void Save(string fileName) |
|
the Save method to store |
{ |
|
both the file name and |
. . . |
|
// Store the data for each photograph |
|
|
caption, each on a separate |
|
|
foreach (Photograph photo in this) |
|
|
line. |
{ |
|
|
sw.WriteLine(photo.FileName); |
|
|
sw.WriteLine(photo.Caption); |
|
|
} |
|
|
} |
|
|
|
Note that the rest of our Save method works as before. In particular, the current version number is written as the first line of the file. Since we updated the constant for this number, the value written to our new album files is updated as well.
Next we need to modify our Open method to read the new file format. We will also preserve backward compatibility with our older version. This can be done by handling
MODAL DIALOG BOXES |
239 |

our previous version number 66 in addition to our new one. We continue the previous table with the following steps.
UPDATE THE OPEN METHOD TO READ CAPTIONS
|
Action |
Result |
|
|
|
3 |
Modify the switch block in |
public void Open(string fileName) |
|
the Open method to |
{ |
|
recognize both the old and |
. . . |
|
switch (version) |
|
|
current version. |
|
|
{ |
|
|
|
case 66: |
|
|
case 83: |
|
|
{ |
|
|
string name; |
|
|
|
4 |
Modify the do..while |
do |
|
loop to read the caption |
{ |
|
when a newer version of |
name = sr.ReadLine(); |
|
|
|
|
the file is opened. |
if (name != null) |
|
|
{ |
|
|
Photograph p = new Photograph(name); |
|
|
if (version == 83) |
|
|
{ |
|
|
// Also read the caption string |
|
|
p.Caption = sr.ReadLine(); |
|
|
} |
|
|
this.Add(p); |
|
|
} |
|
|
} while (name!= null); |
|
|
break; |
|
|
. . . |
|
|
} |
|
|
|
Our data layer is complete. We can add individual captions to photographs, and these captions are preserved as the album is saved and opened. Next we turn our attention to the new Form required.
8.3.3CREATING THE CAPTIONDLG FORM
With our data layer ready, we can turn to the presentation layer. This requires the dialog previously shown in figure 8.5. In this section we create a new Form class to hold the dialog, and look at what settings should be set to turn the default form into a standard dialog box. In the next section we will add some properties to this class so that our MainForm class can interact with the dialog.
In previous Windows development environments, an explicit class such as CDialog created a dialog box directly. It would certainly be possible to create a
FormDialog class in .NET derived from the Form class for this purpose, and perhaps Microsoft will do so in the future. Until this happens, you will have to create your own dialog class or modify each dialog form separately to have dialog box behavior. The following table summarizes the properties required to turn the default Form into a somewhat standard dialog box.
240 |
CHAPTER 8 DIALOG BOXES |
Turning the default Form into a dialog box
Property |
Default |
Value for |
Comments |
|
Dialog Box |
||||
|
|
|
||
|
|
|
|
|
AcceptButton |
(none) |
OK button |
For a modal dialog, set to the OK or other |
|
|
|
instance |
Button the user will click when finished. |
|
CancelButton |
(none) |
Cancel button |
For a modal dialog, set to the Cancel or |
|
|
|
instance |
other Button the user will click to abort |
|
|
|
|
dialog. |
|
FormBorderStyle |
Sizable |
FixedDialog |
This creates a fixed-sized window with a |
|
|
|
|
thick dialog-style border, and no control box |
|
|
|
|
on the title bar. Assuming the ControlBox |
|
|
|
|
setting is true, the system menu is still |
|
|
|
|
available by right-clicking on the title bar. |
|
|
|
|
This value is based on the |
|
|
|
|
FormBorderStyle enumeration. |
|
HelpButton |
False |
True or False |
Set to true if you would like the question |
|
|
|
|
mark box to appear on the title bar. The |
|
|
|
|
HelpRequested event fires when this box |
|
|
|
|
is clicked. Note that the question box only |
|
|
|
|
appears if the MaximizeBox and |
|
|
|
|
MinimumBox properties are both false. |
|
MaximizeBox |
True |
False |
Removes the Maximize button from the |
|
|
|
|
title bar. |
|
MinimizeBox |
True |
False |
Removes the Minimize button from the title |
|
|
|
|
bar. |
|
ShowInTaskBar |
True |
False |
Does not display the dialog on the |
|
|
|
|
Windows task bar. |
|
StartPosition |
WindowsDefault- |
CenterParent |
Establishes the initial position for the form. |
|
|
Location |
|
Typically, a dialog box is centered over the |
|
|
|
|
parent window. |
|
Size |
300, 300 |
(varies) |
For a fixed size dialog, set the window to an |
|
|
|
|
appropriate size. |
|
|
|
|
|
Of course, you may need to modify other properties as well, but these settings establish the appropriate features for a standard dialog box. We can use this table to create a dialog in our application.
MODAL DIALOG BOXES |
241 |

CREATE THE CAPTIONDLG CLASS
|
|
Action |
|
|
Result |
|
|
|
|
|
|
|
|
1 |
Add a new form called “CaptionDlg” |
The new file is added to the MyPhotos project and |
||||
|
to the MyPhotos project. |
a CaptionDlg.cs [Design] window displays your |
||||
|
How-to |
|
|
|
new form. |
|
|
|
|
|
|
||
|
a. Right-click the MyPhotos project |
|
||||
|
in Solution Explorer. |
|
||||
|
b. Expand the Add menu. |
|
||||
|
c. Select Add Windows Form… |
|
||||
|
under the Add menu to display |
|
||||
|
the Add New Item dialog. |
|
||||
|
d. Enter “CaptionDlg” as the name |
|
||||
|
of the form. |
|
|
|
|
|
|
e. Click the Open button. |
|
||||
|
|
|
|
|
|
|
2 |
Modify the form’s properties to |
The form in the designer window should now look |
||||
|
make this create a somewhat |
something like this. |
||||
|
standard dialog. |
|
|
|
|
|
|
|
Settings |
|
|||
|
|
|
|
|
|
|
|
|
Property |
|
Value |
|
|
|
|
FormBorderStyle |
|
FixedDialog |
|
|
|
|
MaximizeBox |
|
False |
|
|
|
|
MinimizeBox |
|
False |
|
|
|
|
ShowInTaskBar |
|
False |
|
|
|
|
Size |
|
350, 160 |
|
|
|
|
StartPosition |
CenterParent |
|
||
|
|
Text |
|
Edit Caption |
|
|
|
|
|
|
|
|
|
If you compare these settings to the previous table, you will see that we have not set the AcceptButton and CancelButton properties yet. This is because the required buttons are not yet on our form. We will look at some of the code generated in the CaptionDlg.cs file in a moment. Before we do, let’s continue our changes to add the required controls to our form.
242 |
CHAPTER 8 DIALOG BOXES |