
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
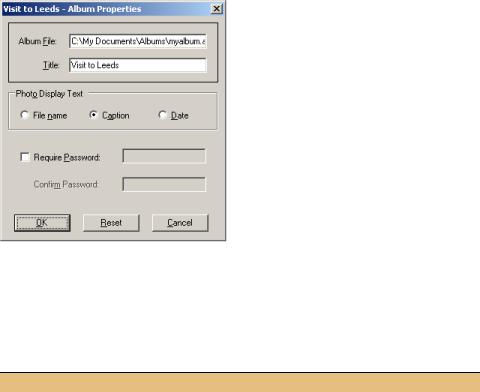
•Password—if present, a password is required to open the album. We will use a CheckBox to indicate whether a password is desired, and TextBox controls to accept and confirm the password.
A dialog to support these new settings is shown in figure 9.5. Of course, we will need some additional infrastructure in our PhotoAlbum class to support these new settings.
Figure 9.5
Note how the AlbumEditDlg modifies the Panel control inherited from BaseEditDlg as compared with the PhotoEditDlg just completed. This is possible since the panel is a protected member of the base form.
9.3.1EXPANDING THE PHOTOALBUM CLASS
In order to support title, display name, and password settings in our albums, we need to make a few changes. For starters, let’s add some variables to hold these values and properties to provide external access.
Set the version number of the MyPhotoAlbum library to 9.3.
ADD NEW SETTINGS TO THE PHOTOALBUM CLASS
|
Action |
Result |
|
|
|
1 |
In the PhotoAlbum.cs file, add |
private string _title; |
|
some variables to hold the new |
private string _password; |
|
title, password, and display option |
public enum DisplayValEnum { |
|
settings. |
|
|
FileName, Caption, Date |
|
|
|
}; |
|
|
private DisplayValEnum _displayOption |
|
|
= DisplayValEnum.Caption; |
|
|
|
BUTTON CLASSES |
293 |

ADD NEW SETTINGS TO THE PHOTOALBUM CLASS
|
Action |
Result |
|
|
|
2 |
Add properties to set and retrieve |
public string Title |
|
these values. |
{ |
|
|
get { return _title; } |
|
|
set { _title = value; } |
|
|
} |
|
|
public string Password |
|
|
{ |
|
|
get { return _password; } |
|
|
set { _password = value; } |
|
|
} |
|
|
public DisplayValEnum DisplayOption |
|
|
{ |
|
|
get { return _displayOption; } |
|
|
set { _displayOption = value; } |
|
|
} |
|
|
|
3 |
Modify the OnClear method to |
protected override void OnClear() |
|
reset these settings when the |
{ |
|
album is cleared. |
_currentPos = 0; |
|
_fileName = null; |
|
|
|
|
|
|
_title = null; |
|
|
_password = null; |
|
|
_displayOption = DisplayValEnum.Caption; |
|
|
. . . |
|
|
} |
|
|
|
Next, we need to store and retrieve these values in the Save and Open methods. This will also require us to create a new version of our file. The new version will be 93, to match the current section of the book. Continuing the previous steps:
UPDATE SAVE METHOD IN PHOTOALBUM CLASS
|
Action |
Result |
|
|
|
4 |
Change the current version setting |
private const int CurrentVersion = 93; |
|
to 93. |
|
|
|
|
5 |
Update the Save method to store |
public void Save(string fileName) |
|
the new album settings. |
{ |
|
|
. . . |
|
|
try |
|
|
{ |
|
|
sw.WriteLine |
|
|
(CurrentVersion.ToString()); |
|
|
// Save album properties |
|
|
sw.WriteLine(_title); |
|
|
sw.WriteLine(_password); |
|
|
sw.WriteLine(Convert.ToString( |
|
|
(int)_displayOption)); |
|
|
// Store each photo separately |
|
|
. . . |
|
|
} |
|
|
|
294 |
CHAPTER 9 BASIC CONTROLS |

Similar changes are required for the Open method. To make this code a little more readable, we will extract the code to read the album data into a separate method.
UPDATE OPEN METHOD IN PHOTOALBUM CLASS
|
Action |
|
Result |
|
|
|
|
6 |
Modify the Open method to use |
public void Open(string fileName) |
|
|
a new ReadAlbumData method. |
{ |
|
|
|
. . . |
|
|
|
try |
|
|
|
{ |
|
|
|
// Initialize as a new album |
|
|
|
Clear(); |
|
|
|
this._fileName = fileName; |
|
|
|
ReadAlbumData(sr, version); |
|
|
|
// Check for password |
|
|
|
// |
(we’ll deal with this shortly) |
|
|
Photograph.ReadDelegate PhotoReader; |
|
|
|
switch (version) |
|
|
|
{ |
|
|
|
|
. . . |
|
|
|
case 92: |
|
|
|
case 93: |
|
|
|
PhotoReader = |
|
|
|
new Photograph.ReadDelegate( |
|
|
|
Photograph.ReadVersion92); |
|
|
|
break; |
|
|
|
. . . |
|
|
} |
|
|
|
. . . |
|
|
|
} |
|
|
|
|
|
7 |
Implement the new |
protected void ReadAlbumData |
|
|
ReadAlbumData to read in the |
(StreamReader sr, int version) |
|
|
album-related information from |
{ |
|
|
// Initialize settings to defaults |
||
|
an open stream. |
||
|
_title = null; |
||
|
|
_password = null; |
|
|
|
_displayOption |
|
|
|
|
= DisplayValEnum.Caption; |
|
|
if (version >= 93) |
|
|
|
{ |
|
|
|
// Read album-specific data |
|
|
|
_title = sr.ReadLine(); |
|
|
|
_password = sr.ReadLine(); |
|
|
|
_displayOption = (DisplayValEnum) |
|
|
|
|
Convert.ToInt32(sr.ReadLine()); |
|
|
} |
|
|
|
// Initialize title if none provided |
|
|
|
if (_title == null || _title.Length == 0) |
|
|
|
{ |
|
|
|
_title = Path. |
|
|
|
|
GetFileNameWithoutExtension(_fileName); |
|
|
} |
|
|
|
} |
|
|
|
|
|
Our PhotoAlbum class can now store and retrieve these settings in the album file. We can make immediate use of these new settings within the PhotoAlbum class.
BUTTON CLASSES |
295 |

9.3.2USING THE NEW ALBUM SETTINGS
Before we create the Album Properties form, let’s make use of our new settings within the PhotoAlbum class. The title is not used internally, but the password and display settings are for internal use. The display option indicates which Photograph property should be displayed to represent the photo for the album. This will be used by our main form to decide which string to display on the status bar panel.
The password setting is required when opening the album. When this field is set, the Open method should prompt for the password before it reads in the file. This requires a small dialog box to request this string from the user.
We will provide support for the display option first.
SUPPORT DISPLAY TEXT OPTION
|
Action |
Result |
|
|
|
1 |
In the PhotoAlbum.cs file, add a |
public string GetDisplayText(Photograph photo) |
|
new GetDisplayText method |
{ |
|
to return the display string for a |
|
|
given Photograph object. |
|
|
|
|
2 |
Implement this method by using |
switch (this._displayOption) |
|
the DisplayOption property to |
{ |
|
determine the appropriate value |
case DisplayValEnum.Caption: |
|
default: |
|
|
to return. |
|
|
return photo.Caption; |
|
|
|
case DisplayValEnum.Date: |
|
|
return photo.DateTaken.ToString("g"); |
|
|
case DisplayValEnum.FileName: |
|
|
return Path.GetFileName(photo.FileName); |
|
|
} |
|
|
} |
|
|
|
3 |
Also add a CurrentDisplay- |
public string CurrentDisplayText |
|
Text property to return this |
{ |
|
value for the current photo. |
get { return GetDisplayText(CurrentPhoto); } |
|
} |
|
|
|
|
|
|
|
This code is fairly straightforward. One new feature is the ability to provide a formatting code to the DateTime.ToString method.
return photo.DateTaken.ToString("g");
The "g" string used here causes the short form of the associated DateTime structure to be returned. We will discuss additional formatting conventions for DateTime structures when we discuss the DateTimePicker control in chapter 11.
For the password setting, we require a new dialog to permit this string to be entered by the user when an album is opened. The following steps create the dialog for this purpose:
296 |
CHAPTER 9 BASIC CONTROLS |