
- •brief contents
- •about this book
- •The Windows Forms namespace
- •Part 1: Hello Windows Forms
- •Part 2: Basic Windows Forms
- •Part 3: Advanced Windows Forms
- •Who should read this book?
- •Conventions
- •Action
- •Result
- •Source code downloads
- •Author online
- •acknowledgments
- •about .NET
- •Casting the .NET
- •Windows Forms overview
- •about the cover illustration
- •Hello Windows Forms
- •1.1 Programming in C#
- •1.1.1 Namespaces and classes
- •1.1.2 Constructors and methods
- •1.1.3 C# types
- •1.1.4 The entry point
- •1.1.5 The Application class
- •1.1.6 Program execution
- •1.2 Adding controls
- •1.2.1 Shortcuts and fully qualified names
- •1.2.2 Fields and properties
- •1.2.3 The Controls property
- •1.3 Loading files
- •1.3.1 Events
- •1.3.2 The OpenFileDialog class
- •1.3.3 Bitmap images
- •1.4 Resizing forms
- •1.4.1 Desktop layout properties
- •1.4.2 The Anchor property
- •1.4.3 The Dock property
- •1.5 Recap
- •2.1 Programming with Visual Studio .NET
- •2.1.1 Creating a project
- •Action
- •Result
- •2.1.2 Executing a program
- •Action
- •Result
- •2.1.3 Viewing the source code
- •View the code generated by Visual Studio .NET
- •Action
- •Result
- •2.2 Adding controls
- •2.2.1 The AssemblyInfo file
- •Action
- •Results
- •2.2.2 Renaming a form
- •Action
- •Result
- •2.2.3 The Toolbox window
- •Action
- •Result
- •2.3 Loading files
- •2.3.1 Event handlers in Visual Studio .NET
- •Action
- •Result
- •2.3.2 Exception handling
- •Action
- •Result
- •Action
- •Results and Comments
- •2.4 Resizing forms
- •2.4.1 Assign the Anchor property
- •Action
- •Result
- •2.4.2 Assign the MinimumSize property
- •Action
- •Result
- •2.5 Recap
- •Basic Windows Forms
- •Menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.3 Click events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •3.5 Context menus
- •Action
- •Result
- •Action
- •Result
- •3.6 Recap
- •Status bars
- •4.1 The Control class
- •4.2 The StatusBar class
- •Action
- •Result
- •Action
- •Result
- •4.3.1 Adding panels to a status bar
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •4.5 Recap
- •Reusable libraries
- •5.1 C# classes and interfaces
- •5.2 Class libraries
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.3 Interfaces revisited
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •5.4 Robustness issues
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Common file dialogs
- •Action
- •Results
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.3 Paint events
- •Action
- •Result
- •Action
- •Result
- •6.4 Context menus revisited
- •Action
- •Result
- •Action
- •Result
- •6.5 Files and paths
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.6 Save file dialogs
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •6.7 Open file dialogs
- •Action
- •Result
- •Action
- •Result
- •6.8 Recap
- •Drawing and scrolling
- •7.1 Form class hierarchy
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •7.4 Panels
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Dialog boxes
- •8.1 Message boxes
- •Action
- •Result
- •Action
- •Result
- •8.1.4 Creating A YesNoCancel dialog
- •Action
- •Result
- •Action
- •Result
- •8.2 The Form.Close method
- •8.2.1 The relationship between Close and Dispose
- •Action
- •Result
- •8.3 Modal dialog boxes
- •Action
- •Result
- •Action
- •Result
- •8.3.2 Preserving caption values
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Basic controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.1.2 Creating a derived form
- •Action
- •Result
- •9.2 Labels and text boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •9.3.6 Adding AlbumEditDlg to our main form
- •Action
- •Result
- •Action
- •Result
- •9.4 Recap
- •List controls
- •10.1 List boxes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.2 Multiselection list boxes
- •10.2.1 Enabling multiple selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.3 Combo boxes
- •Action
- •Result
- •Action
- •Result
- •10.4 Combo box edits
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •10.5 Owner-drawn lists
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •More controls
- •Action
- •Result
- •Action
- •Result
- •11.2 Tab pages
- •Action
- •Result
- •Action
- •Result
- •11.3.1 Dates and times
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •11.5 Recap
- •A .NET assortment
- •12.1 Keyboard events
- •Action
- •Result
- •Action
- •Result
- •12.2 Mouse events
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.3 Image buttons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.4 Icons
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •12.5 Recap
- •Toolbars and tips
- •13.1 Toolbars
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •13.4.2 Creating tool tips
- •Action
- •Result
- •Action
- •Result
- •Advanced Windows Forms
- •List views
- •14.2 The ListView class
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.2.3 Populating a ListView
- •Action
- •Result
- •Action
- •14.3 ListView columns
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •14.6 Recap
- •Tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.3 Dynamic tree nodes
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.4 Node selection
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •15.5 Fun with tree views
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Multiple document interfaces
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.3 Merged menus
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.4 MDI children
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •16.5 MDI child window management
- •Action
- •Result
- •Action
- •Result
- •16.6 Recap
- •Data binding
- •17.1 Data grids
- •Action
- •Result
- •Action
- •Result
- •17.2 Data grid customization
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Odds and ends .NET
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.2 Timers
- •Action
- •Result
- •Action
- •Result
- •18.3 Drag and drop
- •Action
- •Result
- •Action
- •Result
- •18.4 ActiveX controls
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •Action
- •Result
- •18.5 Recap
- •C# primer
- •A.1 C# programs
- •A.1.1 Assemblies
- •A.1.2 Namespaces
- •A.2 Types
- •A.2.1 Classes
- •A.2.2 Structures
- •A.2.3 Interfaces
- •A.2.4 Enumerations
- •A.2.5 Delegates
- •A.3 Language elements
- •A.3.1 Built-in types
- •A.3.2 Operators
- •A.3.3 Keywords
- •A.4 Special features
- •A.4.1 Exceptions
- •A.4.2 Arrays
- •A.4.3 Main
- •A.4.4 Boxing
- •A.4.5 Documentation
- •.NET namespaces
- •B.1 System.Collections
- •B.2 System.ComponentModel
- •B.3 System.Data
- •B.4 System.Drawing
- •B.5 System.Globalization
- •B.6 System.IO
- •B.7 System.Net
- •B.8 System.Reflection
- •B.9 System.Resources
- •B.10 System.Security
- •B.11 System.Threading
- •B.12 System.Web
- •B.13 System.Windows.Forms
- •B.14 System.XML
- •Visual index
- •C.1 Objects
- •C.2 Marshal by reference objects
- •C.3 Components
- •C.4 Common dialogs
- •C.7 Event data
- •C.8 Enumerations
- •For more information
- •bibliography
- •Symbols
- •Index
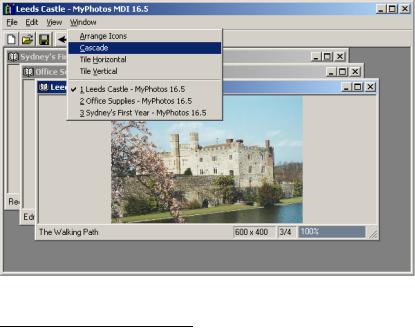
With this change, our MDI application is ready. Compile and run the application and try to find an error.1
TRY IT! We have been careful to use the IsMdiChild property in all our changes to the MainForm.cs source file to ensure that the application can run as a single document interface or a multiple document interface. Test this out by modifying the Startup Object in the properties for the MyPhotos project to use the MainForm.Main method. Recompile the application and verify that it now runs as an SDI application. Change it back to an MDI application by setting the Startup Object to ParentForm.Main.
Our final change in this chapter will be the addition of layout management for our MDI child forms.
16.5MDI CHILD WINDOW MANAGEMENT
Ultimately, an MDI application is simply a collection of Forms displayed in a parent window. The .NET Framework provides some assistance in managing these forms within this parent. In this section we will discuss child form layout and how to show the active forms in a menu. The Form class contains a LayoutMdi method for the former, while the MenuItem class contains an MdiList property for the latter. A new top-level Window menu, as shown in figure 16.9, will make use of these constructs.
Figure 16.9 The new Window menu for our application will support options related to managing child forms within the parent window.
1 Of course, if you do find an error here or elsewhere in the book, please send me an email so the correction can be posted online and the error corrected in the next edition.
MDI CHILD WINDOW MANAGEMENT |
557 |
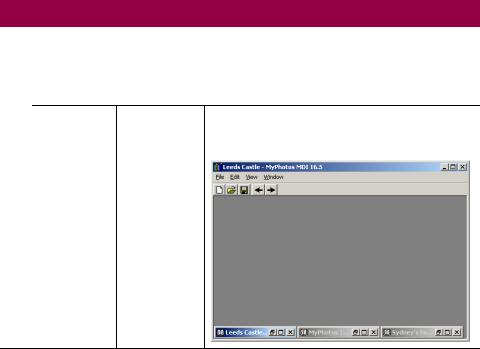
We will begin with the automatic layout of MDI child forms.
16.5.1ARRANGING MDI FORMS
In an MDI application, as well as on the Windows desktop, a number of windows are created and strewn about in various locations. It would be nice if our application permitted automatic organization of the windows at the user’s request. This would allow the user to immediately see all open windows and select the desired one.
Such support is provided by the LayoutMdi method of the Form class. This method accepts an enumeration value specifying the type of layout to apply to the MDI container, as shown by the following signature:
public void LayoutMdi( MdiLayout layoutValue );
This method is called from the MDI container form, in our case the ParentForm class. The MdiLayout enumeration is summarized in .NET Table 16.3. To demonstrate how this is used, we will create a new Windows menu containing options for each of the main layout options. An illustration of each option is shown in the table.
.NET Table 16.3 MdiLayout enumeration
The MdiLayout enumeration specifies the possible layout options for a set of MDI child forms. This is used in the LayoutMdi method of the Form class to automatically display a set of forms with the given layout mode. This class is part of the System.Windows.Forms namespace.
The following table illustrates each layout style with a set of three child forms.
ArrangeIcons |
The icons or minimized forms are arranged within the |
|
client window. Note that this has no effect on child |
|
forms that are not minimized. |
Enumeration
Values
558 |
CHAPTER 16 MULTIPLE DOCUMENT INTERFACES |
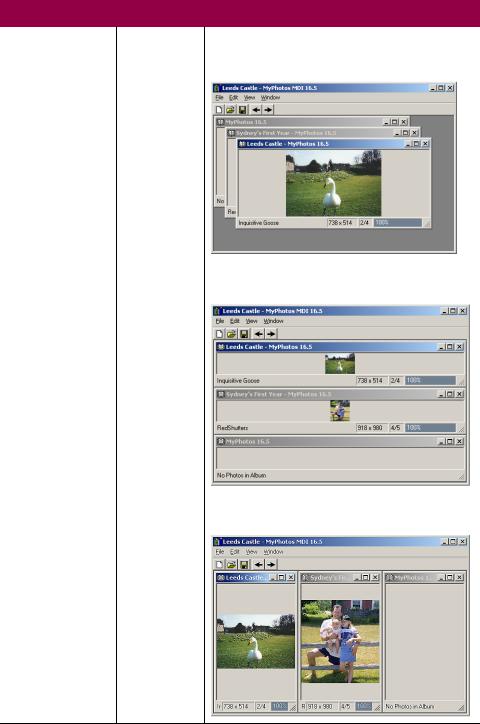
.NET Table 16.3 MdiLayout enumeration
Cascade |
The child forms are displayed on top of each other in |
|
step fashion so that only the title bar of the hidden forms |
|
is visible. |
TileHorizontal |
The client area is divided horizontally into equal sections |
|
and each open window is displayed in a section. |
Enumeration
Values
TileVertical |
The client area is divided vertically into equal sections |
|
and each open window is displayed in a section. |
MDI CHILD WINDOW MANAGEMENT |
559 |
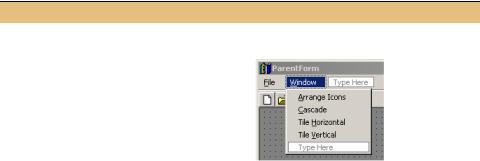
In your application, you can choose to support some or all of these options. The various layout styles are useful for quickly seeing the entire set of open windows in an MDI application, and typically appear in a Windows menu located on the MDI parent form. Since our application is nothing if not typical, we will do exactly this. The following table details the required steps.
Set the version number of the MyPhotos application to 16.5.
ADD LAYOUT MENUS TO THE PARENT FORM
|
|
|
|
|
Action |
Result |
|||||
|
|
|
|
|
|
|
|
|
|
||
1 |
In the ParentForm.cs [Design] window, add |
|
|||||||||
|
a new top-level Windows menu to the |
|
|||||||||
|
form. |
|
|
|
|
|
|
|
|
||
|
|
|
|
|
Settings |
|
|||||
|
|
|
|
|
|
|
|
|
|
||
|
|
|
Property |
|
|
Value |
|
||||
|
|
|
(Name) |
|
menuWindows |
|
|||||
|
|
|
MergeOrder |
|
3 |
|
|
|
|||
|
|
|
|
Text |
|
|
&Window |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
2 |
Add a menu item for each of the layout |
|
|||||||||
|
styles. |
|
|
|
|
|
|
|
|
||
|
|
|
|
|
Settings |
|
|||||
|
|
|
|
|
|
|
|
|
|||
|
|
Menu |
|
Property |
|
Value |
|
||||
|
|
Arrange |
|
(Name) |
|
menuArrange |
|
||||
|
|
|
|
|
Text |
|
&Arrange Icons |
|
|||
|
|
Cascade |
|
(Name) |
|
menuCascade |
|
||||
|
|
|
|
|
Text |
|
&Cascade |
|
|||
|
|
Horizontal |
|
(Name) |
|
menuTileHorizontal |
|
||||
|
|
|
|
|
Text |
|
Tile &Horizontal |
|
|||
|
|
Vertical |
|
(Name) |
|
menuTileVertical |
|
||||
|
|
|
|
|
Text |
|
Tile &Vertical |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
560 |
CHAPTER 16 MULTIPLE DOCUMENT INTERFACES |
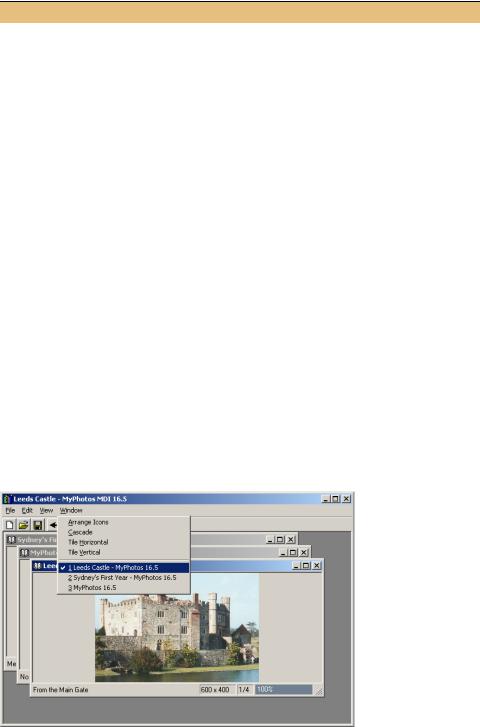
ADD LAYOUT MENUS TO THE PARENT FORM (continued)
|
Action |
Result |
|
|
|
3 |
Add a Click event handler for each menu |
private void menuArrange_Click |
|
that calls the LayoutMdi method with the |
(object sender, System.EventArgs e) |
|
corresponding MdiLayout enumeration |
{ |
|
LayoutMdi(MdiLayout.ArrangeIcons); |
|
|
value. |
|
|
} |
|
|
|
private void menuCascade_Click |
|
|
(object sender, System.EventArgs e) |
|
|
{ |
|
|
LayoutMdi(MdiLayout.Cascade); |
|
|
} |
|
|
private void menuTileHorizontal_Click |
|
|
(object sender, System.EventArgs e) |
|
|
{ |
|
|
LayoutMdi(MdiLayout.TileHorizontal); |
|
|
} |
|
|
private void menuTileVertical_Click |
|
|
(object sender, System.EventArgs e) |
|
|
{ |
|
|
LayoutMdi(MdiLayout.TileVertical); |
|
|
} |
|
|
|
This change permits the user to automatically arrange the open windows in the selected style. Compile and run the application, open a few windows, and verify that these changes work as advertised.
The other topic related to our Windows menu is that of the MdiList property.
16.5.2CREATING AN MDI CHILD LIST
It is common in MDI applications to provide a list of open windows as part of the Window menu. This permits the user to quickly jump to an open window at the click of the mouse. The .NET folks at Microsoft were kind enough to provide a quick way to do this through the MdiList property of the MenuItem class.
Figure 16.10
In the list of child forms, note how the active child form is automatically checked by the framework.
MDI CHILD WINDOW MANAGEMENT |
561 |