
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

624 Chapter 18 Applets and Multimedia
59frame.setLocationRelativeTo(null); // Center the frame
60frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
61frame.setVisible(true);
62}
63
64/** Get command-line parameters */
65private void getCommandLineParameters(String[] args) {
66// Check usage and get x, y and message
67if (args.length != 3) {
68System.out.println(
69"Usage: java DisplayMessageApp x y message");
70System.exit(0);
71}
72else {
73x = Integer.parseInt(args[0]);
74y = Integer.parseInt(args[1]);
75message = args[2];
76}
77}
78}
When you run the program as an applet, the main method is ignored. When you run it as an application, the main method is invoked. Sample runs of the program as an application and as an applet are shown in Figure 18.8.
FIGURE 18.8 The DisplayMessageApp class can run as an application and as an applet.
The main method creates a JFrame object frame and creates a JApplet object applet, then places the applet applet into the frame frame and invokes its init method. The application runs just like an applet.
The main method sets isStandalone true (line 45) so that it does not attempt to
|
retrieve HTML parameters when the init method is invoked. |
|
The setVisible(true) method (line 61) is invoked after the components are added to |
|
the applet, and the applet is added to the frame to ensure that the components will be visible. |
|
Otherwise, the components are not shown when the frame starts. |
|
Important Pedagogical Note |
omitting main method |
From now on, all the GUI examples will be created as applets with a main method. Thus you will |
|
be able to run the program either as an applet or as an application. For brevity, the main method |
|
is not listed in the text. |
18.8 Case Study: Bouncing Ball
This section presents an applet that displays a ball bouncing in a panel. Use two buttons to suspend and resume the movement, and use a scroll bar to control the bouncing speed, as shown in Figure 18.9.
Here are the major steps to complete this example:
1.Create a subclass of JPanel named Ball to display a ball bouncing, as shown in Listing 18.7.
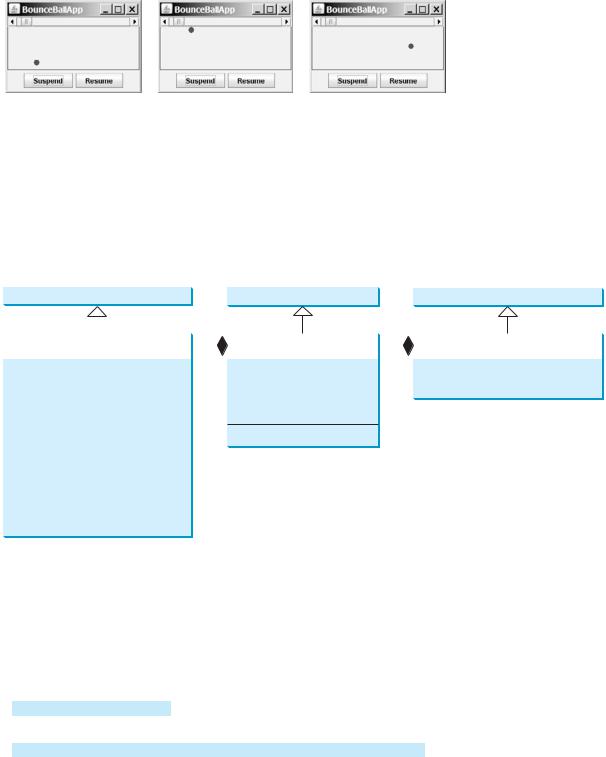
18.8 Case Study: Bouncing Ball 625
FIGURE 18.9 The ball’s movement is controlled by the Suspend and Resume buttons and the scroll bar.
2.Create a subclass of JPanel named BallControl to contain the ball with a scroll bar and two control buttons Suspend and Resume, as shown in Listing 18.8.
3.Create an applet named BounceBallApp to contain an instance of BallControl and enable the applet to run standalone, as shown in Listing 18.9.
The relationship among these classes is shown in Figure 18.10.
javax.swing.JPanel |
|
|
javax.swing.JPanel |
|
|
javax.swing.JApplet |
|||||
|
|
1 |
1 |
|
|
1 |
1 |
|
|||
|
|
|
|
|
|||||||
Ball |
|
BallControl |
BounceBallApp |
||||||||
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
-x: int |
|
|
-ball: Ball |
|
|
+BounceBallApp() |
|||||
-y: int |
|
|
-jsbDelay: JScrollBar |
|
|
+main(args: String[]): void |
|||||
-dx: int |
|
|
-jbtResume: JButton |
|
|
|
|||||
-dy: int |
|
|
-jbtSuspend: JButton |
|
|
|
-radius: int
-delay: int |
+BallControl() |
|
|
-timer: Timer |
|
|
|
+Ball() |
|
+suspend(): void |
|
+resume(): void |
|
+setDelay(delay: int): void |
|
FIGURE 18.10 BounceBallApp contains BallControl, and BallControl contains Ball.
LISTING 18.7 Ball.java
1 import javax.swing.Timer;
2 import java.awt.*;
3 import javax.swing.*;
4 import java.awt.event.*;
5
6 public class Ball extends JPanel {
7 private int delay = 10;
8
9// Create a timer with delay 1000 ms
10 private Timer timer = new Timer(delay, new TimerListener());
11
12private int x = 0; private int y = 0; // Current ball position
13private int radius = 5; // Ball radius
14private int dx = 2; // Increment on ball's x-coordinate
15private int dy = 2; // Increment on ball's y-coordinate
16
timer delay
create timer
626 Chapter 18 |
Applets and Multimedia |
||||||||
|
17 |
|
public Ball() { |
||||||
start timer |
18 |
|
|
timer.start(); |
|
||||
|
19 |
} |
|
|
|
|
|
|
|
|
20 |
|
|
|
|
|
|
|
|
timer listener |
21 |
|
private class TimerListener implements ActionListener { |
|
|||||
|
22 |
|
|
/** Handle the action event */ |
|||||
|
23 |
|
|
public void actionPerformed(ActionEvent e) { |
|||||
repaint ball |
24 |
|
|
|
repaint(); |
|
|
|
|
|
25 |
} |
|
|
|
|
|
||
|
26 |
} |
|
|
|
|
|
|
|
|
27 |
|
|
|
|
|
|
|
|
paint ball |
28 |
|
protected void paintComponent(Graphics g) { |
|
|
||||
|
|
super.paintComponent(g); |
|||||||
|
29 |
|
|
||||||
|
30 |
|
|
|
|
|
|
|
|
|
31 |
|
|
g.setColor(Color.red); |
|||||
|
32 |
|
|
|
|
|
|
|
|
|
33 |
|
|
// Check boundaries |
|||||
|
34 |
|
|
if (x < radius) dx = Math.abs(dx); |
|||||
|
35 |
|
|
if (x > getWidth() - radius) dx = -Math.abs(dx); |
|||||
|
36 |
|
|
if (y < radius) dy = Math.abs(dy); |
|||||
|
37 |
|
|
if (y > getHeight() - radius) dy = -Math.abs(dy); |
|||||
|
38 |
|
|
|
|
|
|
|
|
|
39 |
|
|
// Adjust ball position |
|||||
|
40 |
|
|
x += dx; |
|||||
|
41 |
|
|
y += dy; |
|||||
|
42 |
|
|
g.fillOval(x - radius, y - radius, radius * 2, radius * 2); |
|||||
|
43 |
} |
|
|
|
|
|
|
|
|
44 |
|
|
|
|
|
|
|
|
|
45 |
public void suspend() { |
|||||||
|
46 |
|
timer.stop(); // Suspend timer |
||||||
|
47 |
} |
|
|
|
|
|
|
|
|
48 |
|
|
|
|
|
|
|
|
|
49 |
public void resume() { |
|||||||
|
50 |
|
timer.start(); // Resume timer |
||||||
|
51 |
} |
|
|
|
|
|
|
|
|
52 |
|
|
|
|
|
|
|
|
53public void setDelay(int delay) {
54this.delay = delay;
55timer.setDelay(delay);
56}
57}
The use of Timer to control animation was introduced in §16.12, “Animation Using the Timer Class.” Ball extends JPanel to display a moving ball. The timer listener implements ActionListener to listen for ActionEvent (line 21). Line 10 creates a Timer for a Ball. The timer is started in line 18 when a Ball is constructed. The timer fires an ActionEvent at a fixed rate. The listener responds in line 24 to repaint the ball to animate ball movement. The center of the ball is at (x, y), which changes to (x + dx, y + dy) on the next display (lines 40–41). The suspend and resume methods (lines 45–51) can be used to stop and start the timer. The setDelay(int) method (lines 53–56) sets a new delay.
LISTING 18.8 BallControl.java
|
1 |
import javax.swing.*; |
|
2 |
import java.awt.event.*; |
|
3 |
import java.awt.*; |
|
4 |
|
|
5 |
public class BallControl extends JPanel { |
button |
6 |
private Ball ball = new Ball(); |

18.8 Case Study: Bouncing Ball 627
7private JButton jbtSuspend = new JButton("Suspend");
8 |
private JButton jbtResume = |
new JButton("Resume"); |
scroll bar |
|
9 |
private JScrollBar jsbDelay |
= new JScrollBar(); |
|
|
10 |
|
|
|
|
11 |
public BallControl() { |
|
|
create UI |
12// Group buttons in a panel
13JPanel panel = new JPanel();
14panel.add(jbtSuspend);
15panel.add(jbtResume);
16
17// Add ball and buttons to the panel
18ball.setBorder(new javax.swing.border.LineBorder(Color.red));
19jsbDelay.setOrientation(JScrollBar.HORIZONTAL);
20ball.setDelay(jsbDelay.getMaximum());
21setLayout(new BorderLayout());
22add(jsbDelay, BorderLayout.NORTH);
23add(ball, BorderLayout.CENTER);
24add(panel, BorderLayout.SOUTH);
25 |
|
|
|
|
26 |
// Register listeners |
|
||
27 |
jbtSuspend.addActionListener(new ActionListener() { |
register listener |
||
28 |
public void actionPerformed(ActionEvent e) { |
|
||
29 |
|
ball.suspend(); |
|
suspend |
30}
31});
32 |
jbtResume.addActionListener(new ActionListener() { |
register listener |
||
33 |
public void actionPerformed(ActionEvent e) { |
|
||
34 |
|
ball.resume(); |
|
resume |
35}
36});
37 |
jsbDelay.addAdjustmentListener(new AdjustmentListener() { |
register listener |
||
38 |
public void adjustmentValueChanged(AdjustmentEvent e) { |
|
||
39 |
|
ball.setDelay(jsbDelay.getMaximum() - e.getValue()); |
|
new delay |
40}
41});
42}
43}
The BallControl class extends JPanel to display the ball with a scroll bar and two control buttons. When the Suspend button is clicked, the ball’s suspend() method is invoked to suspend the ball movement (line 29). When the Resume button is clicked, the ball’s resume() method is invoked to resume the ball movement (line 34). The bouncing speed can be changed using the scroll bar.
LISTING 18.9 BounceBallApp.java
1 import java.awt.*;
2 import javax.swing.*;
3
4 public class BounceBallApp extends JApplet {
5 public BounceBallApp() {
6add(new BallControl());
7 |
} |
8 |
} |
add BallControl
main method omitted
The BounceBallApp class simply places an instance of BallControl in the applet. The main method is provided in the applet (not displayed in the listing for brevity) so that you can also run it standalone.