
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX
84 Chapter 3 Selections
To swap two variables number1 and number2, a temporary variable temp (line 11) is used to first hold the value in number1. The value in number2 is assigned to number1 (line 12), and the value in temp is assigned to number2 (line 13).
3.10 Problem: Computing Body Mass Index
Body Mass Index (BMI) is a measure of health on weight. It can be calculated by taking your weight in kilograms and dividing by the square of your height in meters. The interpretation of BMI for people 16 years or older is as follows:
BMI |
Interpretation |
below 16 |
seriously underweight |
16–18 |
underweight |
18–24 |
normal weight |
24–29 |
overweight |
29–35 |
seriously overweight |
above 35 |
gravely overweight |
Write a program that prompts the user to enter a weight in pounds and height in inches and display the BMI. Note that one pound is 0.45359237 kilograms and one inch is 0.0254 meters. Listing 3.5 gives the program.
LISTING 3.5 ComputeBMI.java
|
1 |
import java.util.Scanner; |
|
2 |
|
|
3 |
public class ComputeAndInterpretBMI { |
|
4 |
public static void main(String[] args) { |
|
5 |
Scanner input = new Scanner(System.in); |
|
6 |
|
|
7 |
// Prompt the user to enter weight in pounds |
|
8 |
System.out.print("Enter weight in pounds: "); |
input weight |
9 |
double weight = input.nextDouble(); |
|
10 |
|
|
11 |
// Prompt the user to enter height in inches |
|
12 |
System.out.print("Enter height in inches: "); |
input height |
13 |
double height = input.nextDouble(); |
|
14 |
|
|
15 |
final double KILOGRAMS_PER_POUND = 0.45359237; // Constant |
|
16 |
final double METERS_PER_INCH = 0.0254; // Constant |
|
17 |
|
|
18 |
// Compute BMI |
|
19 |
double weightInKilograms = weight * KILOGRAMS_PER_POUND; |
|
20 |
double heightInMeters = height * METERS_PER_INCH; |
compute bmi |
21 |
double bmi = weightInKilograms / |
|
22 |
(heightInMeters * heightInMeters); |
|
23 |
|
|
24 |
// Display result |
display output |
25 |
System.out.printf("Your BMI is %5.2f\n", bmi); |
|
26 |
if (bmi < 16) |
|
27 |
System.out.println("You are seriously underweight"); |
|
28 |
else if (bmi < 18) |
|
29 |
System.out.println("You are underweight"); |
|
30 |
else if (bmi < 24) |
|
31 |
System.out.println("You are normal weight"); |
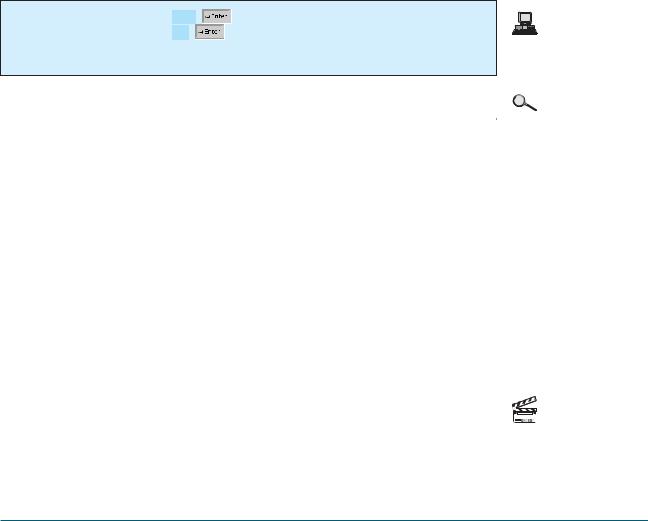
3.11 Problem: Computing Taxes 85
32else if (bmi < 29)
33System.out.println("You are overweight");
34else if (bmi < 35)
35System.out.println("You are seriously overweight");
36else
37System.out.println("You are gravely overweight");
38}
39}
Enter weight in pounds: 146
Enter height in inches: 70
Your BMI is 20.948603801493316
You are normal weight
|
line# |
weight height WeightInKilograms heightInMeters bmi |
output |
|
|
|
|
|
|
|
9 |
146 |
|
|
13 |
70 |
|
|
|
19 |
66.22448602 |
|
|
|
20 |
1.778 |
|
|
|
21 |
20.9486 |
|
|
|
25 |
|
Your BMI |
||
|
|
|
is 20.95 |
|
31 |
|
You are |
||
|
|
|
normal |
|
|
|
|
weight |
|
|
|
|
|
|
Two constants KILOGRAMS_PER_POUND and METERS_PER_INCH are defined in lines 15–16. Using constants here makes programs easy to read.
3.11 Problem: Computing Taxes
The United States federal personal income tax is calculated based on filing status and taxable income. There are four filing statuses: single filers, married filing jointly, married filing separately, and head of household. The tax rates vary every year. Table 3.2 shows the rates for 2009. If you are, say, single with a taxable income of $10,000, the first $8,350 is taxed at 10% and the other $1,650 is taxed at 15%. So, your tax is $1,082.5
Video Note
Use multiple alternative if statements
TABLE 3.2 2009 U.S. Federal Personal Tax Rates
Marginal |
|
Married Filing Jointly |
|
|
|
Tax Rate |
Single |
or Qualified Widow(er) |
Married Filing Separately |
Head of Household |
|
|
|
|
|
|
|
10% |
$0 - $8,350 |
$0 - $16,700 |
$0 - $8,350 |
$0 - $11,950 |
|
15% |
$8,351 - $33,950 |
$16,701 |
- $67,900 |
$8,351 - $33,950 |
$11,951 - $45,500 |
25% |
$33,951 - $82,250 |
$67,901 - $137,050 |
$33,951 - $68,525 |
$45,501 - $117,450 |
|
28% |
$82,251 - $171,550 |
$137,051 |
- $208,850 |
$68,525 - $104,425 |
$117,451 - $190,200 |
33% |
$171,551 - $372,950 |
$208,851 |
- $372,950 |
$104,426 - $186,475 |
$190,201 - $372,950 |
35% |
$372,951+ |
$372,951+ |
$186,476+ |
$372,951+ |
86 Chapter 3 Selections
You are to write a program to compute personal income tax. Your program should prompt the user to enter the filing status and taxable income and compute the tax. Enter 0 for single filers, 1 for married filing jointly, 2 for married filing separately, and 3 for head of household.
Your program computes the tax for the taxable income based on the filing status. The filing status can be determined using if statements outlined as follows:
if (status == 0) {
// Compute tax for single filers
}
else if (status == 1) {
// Compute tax for married filing jointly
}
else if (status == 2) {
// Compute tax for married filing separately
}
else if (status == 3) {
// Compute tax for head of household
}
else {
// Display wrong status
}
For each filing status there are six tax rates. Each rate is applied to a certain amount of taxable income. For example, of a taxable income of $400,000 for single filers, $8,350 is taxed at 10%, 133,950 - 8,3502 at 15%, 182,250 - 33,9502 at 25%, 1171,550 - 82,2502 at 28%,
1372,950 - 171,5502 at 33%, and 1400,000 - 372,9502 at 35%.
Listing 3.6 gives the solution to compute taxes for single filers. The complete solution is left as an exercise.
LISTING 3.6 ComputeTax.java
1 import java.util.Scanner;
2
3 public class ComputeTax {
4 public static void main(String[] args) {
|
5 |
// Create a Scanner |
|
|
6 |
Scanner input = new Scanner(System.in); |
|
|
7 |
|
|
|
8 |
// Prompt the user to enter filing status |
|
|
9 |
System.out.print( |
|
|
10 |
"(0-single filer, 1-married jointly,\n" + |
|
|
11 |
"2-married separately, 3-head of household)\n" + |
|
|
12 |
"Enter the filing status: "); |
|
input status |
13 |
int status = input.nextInt(); |
|
|
14 |
|
|
|
15 |
// Prompt the user to enter taxable income |
|
|
16 |
System.out.print("Enter the taxable income: "); |
|
input income |
17 |
double income = input.nextDouble(); |
|
|
18 |
|
|
|
19 |
// Compute tax |
|
|
20 |
double tax = 0; |
|
|
21 |
|
|
compute tax |
22 |
if (status == 0) { |
// Compute tax for single filers |
|
23 |
if (income <= 8350) |
|
|
24 |
tax = income * 0.10; |
|
|
25 |
else if (income <= 33950) |
|
|
26 |
tax = 8350 * 0.10 + (income - 8350) * 0.15; |
|
|
27 |
else if (income <= 82250) |
|
|
28 |
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + |
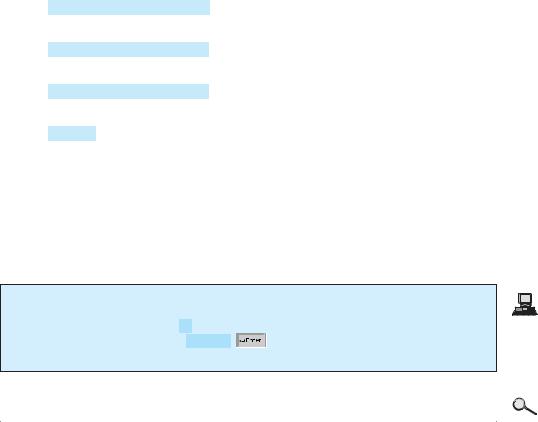
3.11 Problem: Computing Taxes 87
29(income - 33950) * 0.25;
30else if (income <= 171550)
31tax = 8350 * 0.10 + (33950 - 8350) * 0.15 +
32(82250 - 33950) * 0.25 + (income - 82250) * 0.28;
33else if (income <= 372950)
34tax = 8350 * 0.10 + (33950 - 8350) * 0.15 +
35(82250 - 33950) * 0.25 + (171550 - 82250) * 0.28 +
36(income - 171550) * 0.35;
37else
38tax = 8350 * 0.10 + (33950 - 8350) * 0.15 +
39(82250 - 33950) * 0.25 + (171550 - 82250) * 0.28 +
40(372950 - 171550) * 0.33 + (income - 372950) * 0.35;
41}
42else if (status == 1) { // Compute tax for married file jointly
43// Left as exercise
44}
45else if (status == 2) { // Compute tax for married separately
46// Left as exercise
47}
48else if (status == 3) { // Compute tax for head of household
49// Left as exercise
50}
51else {
52System.out.println("Error: invalid status");
53 |
System.exit(0); |
exit program |
54 |
} |
|
55 |
|
|
56 |
// Display the result |
|
57 |
System.out.println("Tax is " + (int)(tax * 100) / 100.0); |
display output |
58}
59}
(0-single filer, 1-married jointly, 2-married separately, 3-head of household) Enter the filing status: 0
Enter the taxable income: 400000
Tax is 117683.5
line# |
status |
income |
tax |
output |
||
|
|
|
|
|
|
|
|
13 |
0 |
|
|
|
|
17 |
|
400000 |
|
|
|
|
20 |
|
|
0 |
|
|
|
38 |
|
|
117683.5 |
|
|
|
57 |
|
|
|
Tax is 117683.5 |
||
|
|
|
|
|
|
|
The program receives the filing status and taxable income. The multiple alternative if statements (lines 22, 42, 45, 48, 51) check the filing status and compute the tax based on the filing status.
System.exit(0) (line 53) is defined in the System class. Invoking this method termi- System.exit(0) nates the program. The argument 0 indicates that the program is terminated normally.
An initial value of 0 is assigned to tax (line 20). A syntax error would occur if it had no initial value, because all of the other statements that assign values to tax are within the if