
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX
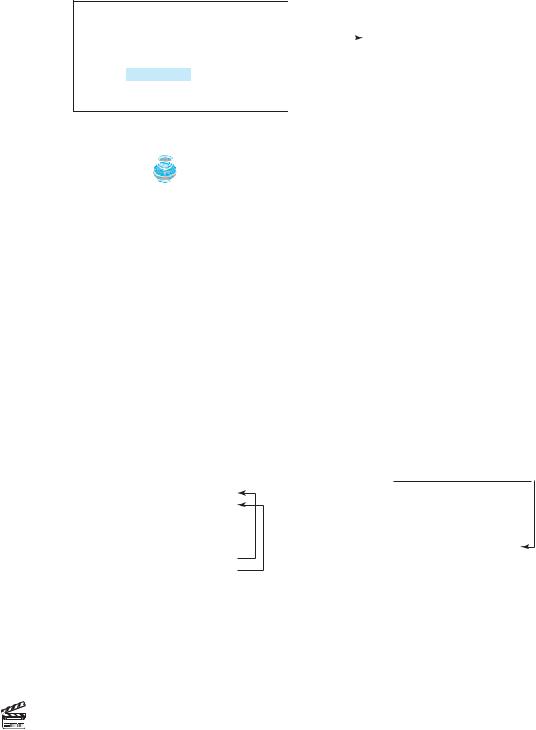
160 Chapter 5 Methods
public static int sign(int n) { if (n > 0)
return 1;
else if (n == 0) return 0;
else if (n < 0) return –1;
}
(a)
|
|
public static int sign(int n) { |
|
Should be |
if (n > 0) |
||
return 1; |
|||
|
|
||
|
|
else if (n == 0) |
|
|
|
return 0; |
|
|
|
else |
|
|
|
return –1; |
|
|
|
} |
|
|
|
|
|
|
|
(b) |
|
Note |
reusing method |
Methods enable code sharing and reuse. The max method can be invoked from any class besides |
|
TestMax. If you create a new class, you can invoke the max method using Class- |
|
Name.methodName (i.e., TestMax.max). |
5.3.1Call Stacks
stack |
Each time a method is invoked, the system stores parameters and variables in an area of mem- |
||||||||||
ory known as a stack, which stores elements in last-in, first-out fashion. When a method calls |
|||||||||||
|
|
|
|||||||||
|
|
|
another method, the caller’s stack space is kept intact, and new space is created to handle the |
||||||||
|
|
|
new method call. When a method finishes its work and returns to its caller, its associated |
||||||||
|
|
|
space is released. |
|
|
|
|
|
|
||
|
|
|
Understanding call stacks helps you to comprehend how methods are invoked. The vari- |
||||||||
|
|
|
ables defined in the main method are i, j, and k. The variables defined in the max method are |
||||||||
|
|
|
num1, num2, and result. The variables num1 and num2 are defined in the method signature |
||||||||
|
|
|
and are parameters of the method. Their values are passed through method invocation. Figure |
||||||||
|
|
|
5.3 illustrates the variables in the stack. |
|
|
|
|
||||
|
|
|
Space required for |
|
Space required for |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
the max method |
|
the max method |
|
|
|
|
|
|
|
|
|
num2: 2 |
|
result: 5 |
|
|
|
|
|
|
|
|
|
|
num2: 2 |
|
|
|
|
|
||
|
|
|
num1: 5 |
|
num1: 5 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Space required for |
|
Space required for |
|
Space required for |
|
Space required for |
|
|
|
|
|
the main method |
|
the main method |
|
the main method |
|
the main method |
|
Stack is empty |
|
|
|
k: |
|
k: |
|
k: |
|
k: 5 |
|
|
||
|
j: 2 |
|
j: 2 |
|
j: 2 |
|
j: 2 |
|
|
|
|
|
i: 5 |
|
i: 5 |
|
i: 5 |
|
i: 5 |
|
|
|
|
|
(a) The main |
(b) The max |
(c) The max method |
(d) The max method is |
(e) The main |
||||||
|
method is invoked. |
method is invoked. |
is being executed. |
finished and the return |
method is finished. |
||||||
|
|
|
|
|
|
|
value is sent to k. |
|
|
FIGURE 5.3 When the max method is invoked, the flow of control transfers to the max method. Once the max method is finished, it returns control back to the caller.
Video Note
Use void method
5.4 void Method Example
The preceding section gives an example of a value-returning method. This section shows how to define and invoke a void method. Listing 5.2 gives a program that defines a method named printGrade and invokes it to print the grade for a given score.
LISTING 5.2 TestVoidMethod.java
|
1 |
public class TestVoidMethod { |
main method |
2 |
public static void main(String[] args) { |
|
3 |
System.out.print("The grade is "); |
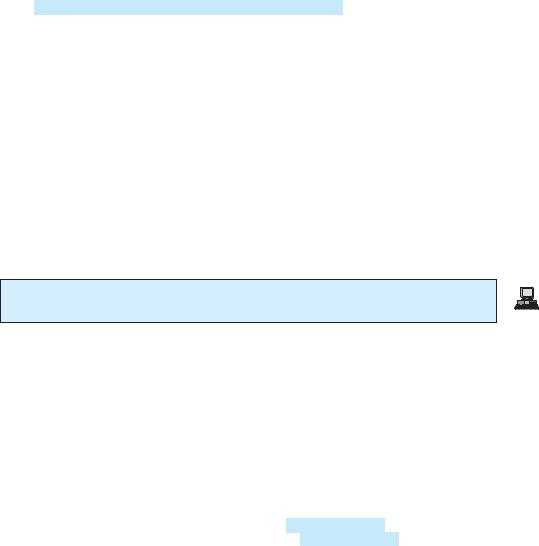
|
5.4 |
void Method Example 161 |
|
|
|
|
|
4 |
printGrade(78.5); |
|
invoke printGrade |
5 |
|
|
|
6System.out.print("The grade is ");
7 |
|
printGrade(59.5); |
printGrade method |
8 |
} |
|
|
9 |
|
|
|
10public static void printGrade(double score) {
11if (score >= 90.0) {
12System.out.println('A');
13}
14else if (score >= 80.0) {
15System.out.println('B');
16}
17else if (score >= 70.0) {
18System.out.println('C');
19}
20else if (score >= 60.0) {
21System.out.println('D');
22}
23else {
24System.out.println('F');
25}
26}
27}
The grade is C
The grade is F
The printGrade method is a void method. It does not return any value. A call to a void |
invoke void method |
method must be a statement. So, it is invoked as a statement in line 4 in the main method. |
|
Like any Java statement, it is terminated with a semicolon. |
|
To see the differences between a void and a value-returning method, let us redesign the |
void vs. value-returned |
printGrade method to return a value. The new method, which we call getGrade, returns |
|
the grade as shown in Listing 5.3. |
|
LISTING 5.3 TestReturnGradeMethod.java
1 public class TestReturnGradeMethod {
2public static void main(String[] args) {
3System.out.print("The grade is " + getGrade(78.5) );
4System.out.print("\nThe grade is " + getGrade(59.5) );
5 |
} |
|
6 |
|
|
7 |
|
public static char getGrade(double score) { |
8 |
|
if (score >= 90.0) |
9return 'A';
10else if (score >= 80.0)
11return 'B';
12else if (score >= 70.0)
13return 'C';
14else if (score >= 60.0)
15return 'D';
16else
17return 'F';
18}
19}
main method
invoke printGrade
printGrade method
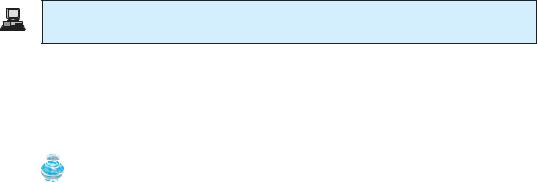
162 Chapter 5 Methods
The grade is C
The grade is F
|
The getGrade method defined in lines 7–18 returns a character grade based on the numeric |
||||
|
score value. The caller invokes this method in lines 3–4. |
||||
|
The getGrade method can be invoked by a caller wherever a character may appear. The |
||||
|
printGrade method does not return any value. It must be also invoked as a statement. |
||||
|
Note |
||||
return in void method |
A return statement is not needed for a void method, but it can be used for terminating the |
||||
|
method and returning to the method’s caller. The syntax is simply |
||||
|
return; |
||||
|
This is not often done, but sometimes it is useful for circumventing the normal flow of control in |
||||
|
a void method. For example, the following code has a return statement to terminate the method |
||||
|
when the score is invalid. |
||||
|
public static void printGrade(double score) { |
||||
|
|
if (score < 0 || score > 100) { |
|
||
|
|
|
System.out.println("Invalid |
score"); |
|
|
|
|
return; |
|
|
|
} |
|
|
|
|
|
if (score >= 90.0) { |
||||
|
|
System.out.println('A'); |
|||
|
} |
|
|
|
|
|
else if (score >= 80.0) { |
||||
|
|
System.out.println('B'); |
|||
|
} |
|
|
|
|
|
else if (score >= 70.0) { |
||||
|
|
|
System.out.println('C'); |
||
|
} |
|
|
|
|
|
else if (score >= 60.0) { |
||||
|
|
|
System.out.println('D'); |
||
|
} |
|
|
|
|
|
else { |
||||
|
|
|
System.out.println('F'); |
||
|
} |
|
|
|
|
|
} |
|
|
|
|
5.5 Passing Parameters by Values
The power of a method is its ability to work with parameters. You can use println to print any string and max to find the maximum between any two int values. When calling a method, you need to provide arguments, which must be given in the same order as their
parameter order association respective parameters in the method signature. This is known as parameter order association. For example, the following method prints a message n times:
public static void nPrintln(String message, int n) { for (int i = 0; i < n; i++)
System.out.println(message);
}
You can use nPrintln("Hello", 3) to print "Hello" three times. The nPrintln("Hello", 3) statement passes the actual string parameter "Hello" to the parameter message; passes 3 to n;
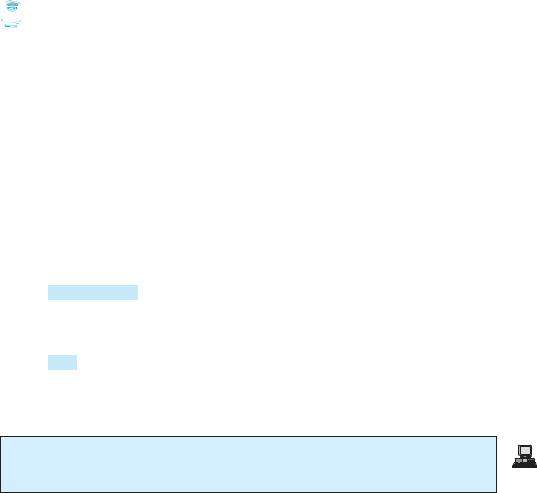
5.5 Passing Parameters by Values 163
and prints "Hello" three times. However, the statement nPrintln(3, "Hello") would be wrong. The data type of 3 does not match the data type for the first parameter, message, nor does the second parameter, "Hello", match the second parameter, n.
Caution
The arguments must match the parameters in order, number, and compatible type, as defined in the method signature. Compatible type means that you can pass an argument to a parameter without explicit casting, such as passing an int value argument to a double value parameter.
When you invoke a method with a parameter, the value of the argument is passed to the para-
meter. This is referred to as pass-by-value. If the argument is a variable rather than a literal pass-by-value value, the value of the variable is passed to the parameter. The variable is not affected, regard-
less of the changes made to the parameter inside the method. As shown in Listing 5.4, the value of x (1) is passed to the parameter n to invoke the increment method (line 5). n is incremented by 1 in the method (line 10), but x is not changed no matter what the method does.
LISTING 5.4 Increment.java
1 public class Increment {
2 public static void main(String[] args) {
3int x = 1;
4System.out.println("Before the call, x is " + x);
5increment(x);
6System.out.println("after the call, x is " + x);
7 |
} |
8 |
|
9public static void increment(int n) {
10n++;
11System.out.println("n inside the method is " + n);
12}
13}
Before the call, x is 1 n inside the method is 2 after the call, x is 1
invoke increment
increment n
Listing 5.5 gives another program that demonstrates the effect of passing by value. The program creates a method for swapping two variables. The swap method is invoked by passing two arguments. Interestingly, the values of the arguments are not changed after the method is invoked.
LISTING 5.5 TestPassByValue.java
1 public class TestPassByValue {
2/** Main method */
3 |
public static void main(String[] args) { |
|
|||
4 |
// Declare |
and initialize variables |
|
||
5 |
int num1 = |
1; |
|
|
|
6 |
int num2 = |
2; |
|
|
|
7 |
|
|
|
|
|
8 |
System.out.println("Before invoking the swap method, num1 is " + |
|
|||
9 |
|
num1 + " |
and num2 is " + num2); |
|
|
10 |
|
|
|
|
|
11 |
// Invoke the swap method to attempt to swap two variables |
false swap |
|||
12 |
|
swap(num1, |
num2); |
|
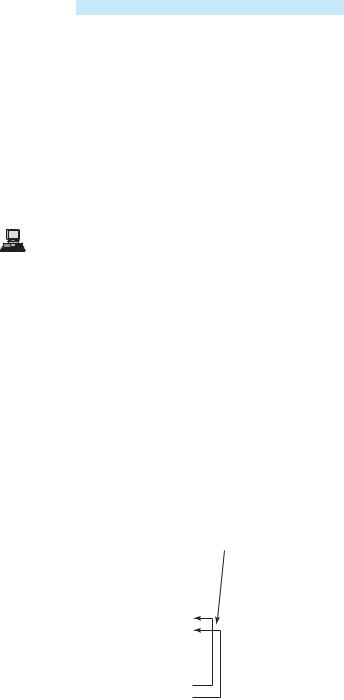
164 Chapter 5 Methods
13
14System.out.println("After invoking the swap method, num1 is " +
15num1 + " and num2 is " + num2);
16}
17
18/** Swap two variables */
19public static void swap(int n1, int n2) {
20System.out.println("\tInside the swap method");
21System.out.println("\t\tBefore swapping n1 is " + n1
22+ " n2 is " + n2);
23
24// Swap n1 with n2
25int temp = n1;
26n1 = n2;
27n2 = temp;
28
29System.out.println("\t\tAfter swapping n1 is " + n1
30+ " n2 is " + n2);
31}
32}
Before invoking the |
swap method, num1 is 1 and num2 is 2 |
Inside the swap method |
|
Before swapping |
n1 is 1 n2 is 2 |
After swapping |
n1 is 2 n2 is 1 |
After invoking the |
swap method, num1 is 1 and num2 is 2 |
|
|
Before the swap method is invoked (line 12), num1 is 1 and num2 is 2. After the swap method is invoked, num1 is still 1 and num2 is still 2. Their values have not been swapped. As shown in Figure 5.4, the values of the arguments num1 and num2 are passed to n1 and n2, but n1 and n2 have their own memory locations independent of num1 and num2. Therefore, changes in n1 and n2 do not affect the contents of num1 and num2.
Another twist is to change the parameter name n1 in swap to num1. What effect does this have? No change occurs, because it makes no difference whether the parameter and the argument have the same name. The parameter is a variable in the method with its own memory space. The variable is allocated when the method is invoked, and it disappears when the method is returned to its caller.
The values of num1 and num2 are passed to n1 and n2. Executing swap does not affect num1 and num2.
|
|
Space required for the |
|
|
|
|
|
|
swap method |
|
|
|
|
|
|
temp: |
|
|
|
|
|
|
n2: 2 |
|
|
|
|
|
|
n1: 1 |
|
|
|
|
Space required for the |
|
Space required for the |
|
Space required for the |
|
|
main method |
|
main method |
|
main method |
|
Stack is empty |
num2: 2 |
|
num2: 2 |
|
num2: 2 |
|
|
num1: 1 |
|
num1: 1 |
|
num1: 1 |
|
|
The main method |
The swap method |
|
The swap method |
The main method |
||
is invoked. |
is invoked. |
|
is finished. |
is finished. |
FIGURE 5.4 The values of the variables are passed to the parameters of the method.