
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX
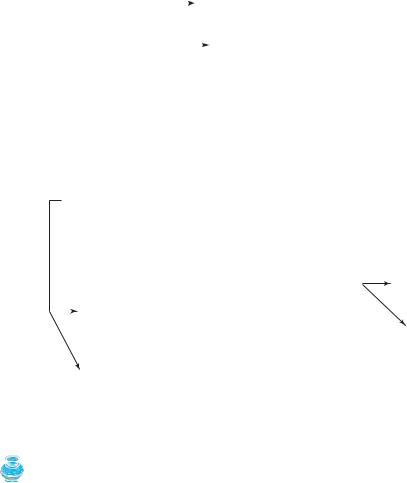
5.9 The Scope of Variables 171
5.9 The Scope of Variables
The scope of a variable is the part of the program where the variable can be referenced. A
variable defined inside a method is referred to as a local variable. local variable The scope of a local variable starts from its declaration and continues to the end of the
block that contains the variable. A local variable must be declared and assigned a value before it can be used.
A parameter is actually a local variable. The scope of a method parameter covers the entire method.
A variable declared in the initial-action part of a for-loop header has its scope in the entire loop. But a variable declared inside a for-loop body has its scope limited in the loop body from its declaration to the end of the block that contains the variable, as shown in Figure 5.5.
|
|
|
|
public static void method1() { |
||||||||
|
|
|
|
. |
|
|
|
|
|
|||
|
|
|
|
. |
|
|
|
|
|
|||
|
|
|
|
|
|
|
for (int |
|
= 1; i < 10; i++) { |
|||
|
|
|
|
i |
||||||||
|
|
|
|
. |
|
|
|
|
||||
The scope of i |
|
|
|
. |
|
; |
||||||
|
|
|
||||||||||
|
|
|
|
|
|
|
|
int |
j |
|||
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
. |
|
|
|
|
||
The scope of j |
|
|
|
. |
|
|
|
|
||||
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
. |
|
|
|
|
||
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
FIGURE 5.5 A variable declared in the initial action part of a for-loop header has its scope in the entire loop.
You can declare a local variable with the same name in different blocks in a method, but you cannot declare a local variable twice in the same block or in nested blocks, as shown in Figure 5.6.
It is fine to declare i in two |
|
|
It is wrong to declare i in two |
|||||||||||||||||
|
|
|||||||||||||||||||
nonnested blocks |
|
|
nested blocks |
|||||||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
public static void method1() { |
|
|
|
|
|
public static void method2() { |
||||||||||||
|
|
|
|
|
int x = 1; |
|
|
|
|
|
|
|
|
|
|
= 1; |
|
|||
|
|
|
|
|
int y = 1; |
|
|
|
|
|
|
|
|
int i |
|
|||||
|
|
|
|
|
for ( |
|
= 1; i < 10; i++) { |
|
|
|
|
|
|
|
|
int sum = 0; |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
int i |
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
x += i; |
|
|
|
|
|
|
|
|
for ( |
int i |
= 1; i < 10; i++) |
|||
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
sum += i; |
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
for ( |
int i |
= 1; i < 10; i++) { |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
} |
|
|
|
|
|
|||||||
|
|
|
|
|
|
y += i; |
|
|
|
|
|
|
|
|
||||||
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
FIGURE 5.6 A variable can be declared multiple times in nonnested blocks but only once in nested blocks.
Caution
Do not declare a variable inside a block and then attempt to use it outside the block. Here is an example of a common mistake:
for (int i = 0; i < 10; i++) {
}
System.out.println(i);
172 Chapter 5 Methods
The last statement would cause a syntax error, because variable i is not defined outside of the for loop.
5.10 The Math Class
The Math class contains the methods needed to perform basic mathematical functions. You have already used the pow(a, b) method to compute ab in Listing 2.8, ComputeLoan.java, and the Math.random() method in Listing 3.4, SubtractionQuiz.java. This section introduces other useful methods in the Math class. They can be categorized as trigonometric methods, exponent methods, and service methods. Besides methods, the Math class provides two useful double constants, PI and E (the base of natural logarithms). You can use these constants as Math.PI and Math.E in any program.
5.10.1Trigonometric Methods
The Math class contains the following trigonometric methods:
/** Return the trigonometric sine of an angle in radians */ public static double sin(double radians)
/** Return the trigonometric cosine of an angle in radians */ public static double cos(double radians)
/** Return the trigonometric tangent of an angle in radians */ public static double tan(double radians)
/** Convert the angle in degrees to an angle in radians */ public static double toRadians(double degree)
/** Convert the angle in radians to an angle in degrees */ public static double toDegrees(double radians)
/** Return the angle in radians for the inverse of sin */ public static double asin(double a)
/** Return the angle in radians for the inverse of cos */ public static double acos(double a)
/** Return the angle in radians for the inverse of tan */ public static double atan(double a)
The parameter for sin, cos, and tan is an angle in radians. The return value for asin, acos, and atan is a degree in radians in the range between - p/2 and p/2. One degree is equal to p/180 in radians, 90 degrees is equal to p/2 in radians, and 30 degrees is equal to p/6 in radians.
For example,
Math.toDegrees(Math.PI / 2) returns 90.0
Math.toRadians(30) returns π/6
Math.sin(0) returns 0.0
Math.sin(Math.toRadians(270)) returns -1.0
Math.sin(Math.PI / 6) returns 0.5
Math.sin(Math.PI / 2) returns 1.0
Math.cos(0) returns 1.0
Math.cos(Math.PI / 6) returns 0.866
Math.cos(Math.PI / 2) returns 0
Math.asin(0.5) returns π/6

5.10 The Math Class 173
5.10.2Exponent Methods
There are five methods related to exponents in the Math class:
/** Return e raised to the power of x (ex) */ public static double exp(double x)
/** Return the natural logarithm of x (ln(x) = loge(x)) */ public static double log(double x)
/** Return the base 10 logarithm of x (log10(x)) */ public static double log10(double x)
/** Return a raised to the power of1 b (ab) */ public static double pow(double a, double b)
/** Return the square root of x ( x) for x >= 0 */ public static double sqrt(double x)
For example,
Math.exp(1) returns 2.71828
Math.log(Math.E) returns 1.0
Math.log10(10) returns 1.0
Math.pow(2, 3) returns 8.0
Math.pow(3, 2) returns 9.0
Math.pow(3.5, 2.5) returns 22.91765
Math.sqrt(4) returns 2.0
Math.sqrt(10.5) returns 3.24
5.10.3The Rounding Methods
The Math class contains five rounding methods:
/** x is rounded up to its nearest integer. This integer is * returned as a double value. */
public static double ceil(double x)
/** x is rounded down to its nearest integer. This integer is * returned as a double value. */
public static double floor(double x)
/** x is rounded to its nearest integer. If x is equally close * to two integers, the even one is returned as a double. */
public static double rint(double x)
/** Return (int)Math.floor(x + 0.5). */ public static int round(float x)
/** Return (long)Math.floor(x + 0.5). */ public static long round(double x)
For example,
Math.ceil(2.1) returns 3.0
Math.ceil(2.0) returns 2.0
Math.ceil(-2.0) returns –2.0
Math.ceil(-2.1) returns -2.0
Math.floor(2.1) returns 2.0
Math.floor(2.0) returns 2.0
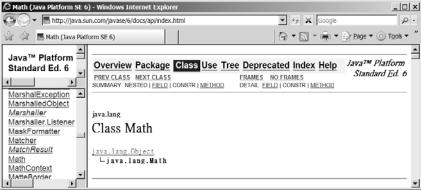
174 Chapter 5 Methods
Math.floor(-2.0) returns –2.0
Math.floor(-2.1) returns -3.0
Math.rint(2.1) returns 2.0
Math.rint(-2.0) returns –2.0
Math.rint(-2.1) returns -2.0
Math.rint(2.5) returns 2.0
Math.rint(3.5) returns 4.0
Math.rint(-2.5) returns -2.0
Math.round(2.6f) returns 3 // Returns int
Math.round(2.0) returns 2 // Returns long
Math.round(-2.0f) returns -2
Math.round(-2.6) returns -3
5.10.4The min, max, and abs Methods
The min and max methods are overloaded to return the minimum and maximum numbers between two numbers (int, long, float, or double). For example, max(3.4, 5.0) returns 5.0, and min(3, 2) returns 2.
The abs method is overloaded to return the absolute value of the number (int, long, float, and double). For example,
Math.max(2, 3) returns 3
Math.max(2.5, 3) returns 3.0
Math.min(2.5, 3.6) returns 2.5
Math.abs(-2) returns 2
Math.abs(-2.1) returns 2.1
5.10.5The random Method
You have used the random() method to generate a random double value greater than or equal to 0.0 and less than 1.0 (0 <= Math.random() < 1.0). This method is very useful. You can use it to write a simple expression to generate random numbers in any range. For example,
1int2 1Math.random1 2 * 102 ¡ Returns a random integer between 0 and 9
50 + 1int2 1Math.random1 2 * 502 ¡ Returns a random integer
|
between 50 and 99 |
|
In general, |
Returns a random number between |
|
a + Math.random1 2 |
||
* b ¡ a and a + b excluding a + b |
FIGURE 5.7 You can view the documentation for Java API online.