
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

4.9 Keywords break and continue 135
14System.out.println("PI is " + pi);
15}
16}
PI is 3.14124
The program repeatedly generates a random point (x, y) in the square in lines 7–8:
double x = Math.random() * 2.0 - 1; double y = Math.random() * 2.0 - 1;
If x2 + y2 … 1, the point is inside the circle and numberOfHits is incremented by 1. p is approximately 4 * numberOfHits / NUMBER_OF_TRIALS (line 13).
4.9 Keywords break and continue
Pedagogical Note
Two keywords, break and continue, can be used in loop statements to provide additional controls. Using break and continue can simplify programming in some cases. Overusing or improperly using them, however, can make programs difficult to read and debug. (Note to instructors: You may skip this section without affecting the rest of the book.)
You have used the keyword break in a switch statement. You can also use break in a loop break to immediately terminate the loop. Listing 4.11 presents a program to demonstrate the effect
of using break in a loop.
LISTING 4.11 TestBreak.java
1 public class TestBreak {
2 public static void main(String[] args) {
3int sum = 0;
4 int number = 0;
5
6while (number < 20) {
7number++;
8 sum += number;
9if (sum >= 100)
10 |
break; |
break |
11}
12
13System.out.println("The number is " + number);
14System.out.println("The sum is " + sum);
15}
16}
The number is 14
The sum is 105
The program in Listing 4.11 adds integers from 1 to 20 in this order to sum until sum is greater than or equal to 100. Without the if statement (line 9), the program calculates the sum of the numbers from 1 to 20. But with the if statement, the loop terminates when sum becomes greater than or equal to 100. Without the if statement, the output would be:
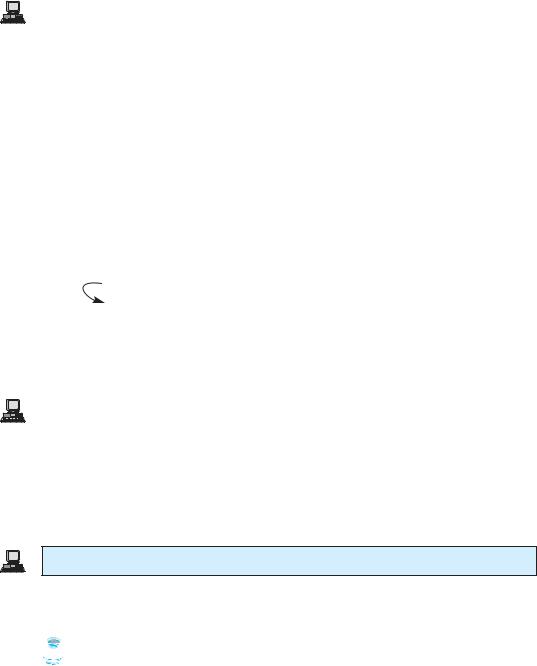
136 Chapter 4 |
|
Loops |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
The number is 20 |
|
|
|
|
|
|
The sum is 210 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
continue |
|
|
|
|
You can also use the continue keyword in a loop. When it is encountered, it ends the current |
|
|
|
|
|
|
iteration. Program control goes to the end of the loop body. In other words, continue breaks |
|
|
|
|
|
|
out of an iteration while the break keyword breaks out of a loop. Listing 4.12 presents a pro- |
|
|
|
|
|
|
gram to demonstrate the effect of using continue in a loop. |
|
|
|
|
|
|
LISTING 4.12 TestContinue.java |
|
|
1 |
public class TestContinue { |
||||
|
2 |
public static void main(String[] args) { |
||||
|
3 |
int sum = 0; |
||||
|
4 |
int number = 0; |
||||
|
5 |
|
||||
|
6 |
while (number < 20) { |
||||
|
7 |
number++; |
||||
|
8 |
if (number == 10 || number == 11) |
||||
continue |
9 |
continue; |
||||
|
10 |
sum += number; |
||||
|
11 |
} |
||||
|
12 |
|
||||
|
13 |
System.out.println("The sum is " + sum); |
||||
|
14 |
} |
||||
|
15 |
} |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
The sum is 189 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
The program in Listing 4.12 adds integers from 1 to 20 except 10 and 11 to sum. With the if statement in the program (line 8), the continue statement is executed when number becomes 10 or 11. The continue statement ends the current iteration so that the rest of the statement in the loop body is not executed; therefore, number is not added to sum when it is 10 or 11. Without the if statement in the program, the output would be as follows:
The sum is 210
In this case, all of the numbers are added to sum, even when number is 10 or 11. Therefore, the result is 210, which is 21 more than it was with the if statement.
Note
The continue statement is always inside a loop. In the while and do-while loops, the loop-continuation-condition is evaluated immediately after the continue statement. In the for loop, the action-after-each-iteration is performed, then the loop-continuation-condition is evaluated, immediately after the continue statement.
You can always write a program without using break or continue in a loop. See Review Question 4.18. In general, using break and continue is appropriate only if it simplifies coding and makes programs easier to read.
Listing 4.2 gives a program for guessing a number. You can rewrite it using a break statement, as shown in Listing 4.13.

4.9 Keywords break and continue 137
LISTING 4.13 GuessNumberUsingBreak.java
1 import java.util.Scanner;
2
3 public class GuessNumberUsingBreak {
4public static void main(String[] args) {
5// Generate a random number to be guessed
6 |
int number = (int)(Math.random() * 101); |
generate a number |
||||
7 |
|
|
|
|
|
|
8 |
Scanner input = new Scanner(System.in); |
|
||||
9 |
System.out.println("Guess a magic number between 0 and 100"); |
|
||||
10 |
|
|
|
|
|
|
11 |
|
while (true) { |
|
loop continuously |
||
12 |
|
// Prompt the user to guess the number |
|
|||
13 |
|
System.out.print("\nEnter your guess: "); |
enter a guess |
|||
14 |
|
int guess = input.nextInt(); |
|
|||
15 |
|
|
|
|
|
|
16 |
|
if (guess == number) { |
|
|||
17 |
|
System.out.println("Yes, the number is " + number); |
|
|||
18 |
|
|
break; |
|
|
break |
19}
20else if (guess > number)
21System.out.println("Your guess is too high");
22else
23System.out.println("Your guess is too low");
24} // End of loop
25}
26}
Using the break statement makes this program simpler and easier to read. However, you should use break and continue with caution. Too many break and continue statements will produce a loop with many exit points and make the program difficult to read.
Note
Some programming languages have a goto statement. The goto statement indiscriminately |
goto |
transfers control to any statement in the program and executes it. This makes your program vul- |
|
nerable to errors. The break and continue statements in Java are different from goto state- |
|
ments. They operate only in a loop or a switch statement. The break statement breaks out of |
|
the loop, and the continue statement breaks out of the current iteration in the loop. |
|
4.9.1Problem: Displaying Prime Numbers
An integer greater than 1 is prime if its only positive divisor is 1 or itself. For example, 2, 3, 5, and 7 are prime numbers, but 4, 6, 8, and 9 are not.
The problem is to display the first 50 prime numbers in five lines, each of which contains ten numbers. The problem can be broken into the following tasks:
■Determine whether a given number is prime.
■For number = 2, 3, 4, 5, 6, Á , test whether it is prime.
■Count the prime numbers.
■Print each prime number, and print ten numbers per line.
Obviously, you need to write a loop and repeatedly test whether a new number is prime. If the number is prime, increase the count by 1. The count is 0 initially. When it reaches 50, the loop terminates.

138 Chapter 4 Loops
Here is the algorithm for the problem:
Set the number of prime numbers to be printed as a constant NUMBER_OF_PRIMES;
Use count to track the number of prime numbers and set an initial count to 0;
Set an initial number to 2;
while (count < NUMBER_OF_PRIMES) { Test whether number is prime;
if number is prime {
Print the prime number and increase the count;
}
Increment number by 1;
}
To test whether a number is prime, check whether it is divisible by 2, 3, 4, up to number/2. If a divisor is found, the number is not a prime. The algorithm can be described as follows:
Use a boolean variable isPrime to denote whether
the number is prime; Set isPrime to true initially;
for (int divisor = 2; divisor <= number / 2; divisor++) { if (number % divisor == 0) {
Set isPrime to false Exit the loop;
}
}
The complete program is given in Listing 4.14.
LISTING 4.14 PrimeNumber.java
1 public class PrimeNumber {
|
2 |
public static void main(String[] args) { |
|||||
|
3 |
|
final int NUMBER_OF_PRIMES = 50; // Number of primes to display |
||||
|
4 |
|
final int NUMBER_OF_PRIMES_PER_LINE = 10; // Display 10 per line |
||||
|
5 |
|
int count = 0; // Count the number of prime numbers |
||||
|
6 |
|
int number = 2; // A number to be tested for primeness |
||||
|
7 |
|
|
|
|
|
|
|
8 |
|
System.out.println("The first 50 prime numbers are \n"); |
||||
|
9 |
|
|
|
|
|
|
|
10 |
|
// Repeatedly find prime numbers |
||||
count prime numbers |
11 |
|
while (count < NUMBER_OF_PRIMES) { |
|
|||
|
12 |
|
|
// Assume the number is prime |
|||
|
13 |
|
|
boolean isPrime = true; // Is the current number prime? |
|||
|
14 |
|
|
|
|
|
|
|
15 |
|
|
// Test whether number is prime |
|||
check primeness |
16 |
|
|
for (int divisor = 2; divisor <= number / 2; divisor++) { |
|
||
|
17 |
|
|
|
if (number % divisor == 0) { // If true, number is not prime |
||
|
18 |
|
|
|
isPrime = false; // Set isPrime to false |
||
exit loop |
19 |
|
|
|
break; // Exit the for loop |
||
|
20 |
} |
|
|
|||
|
21 |
|
|
} |
|
|
|
|
22 |
|
|
|
|
|
|
|
23 |
|
|
// Print the prime number and increase the count |
|||
print if prime |
24 |
|
|
if (isPrime) { |
|||
|
25 |
|
|
|
count++; // Increase the count |