
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX
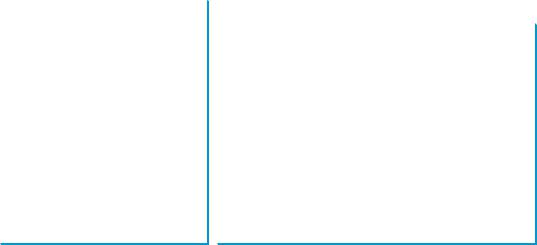
9.7 File Input and Output 325
Figure 9.16(a) shows a sample run of the program on Windows, and Figure 9.16(b), a sample run on Unix. As shown in the figures, the path-naming conventions on Windows are different from those on Unix.
9.7 File Input and Output
A File object encapsulates the properties of a file or a path but does not contain the methods for creating a file or for reading/writing data from/to a file. In order to perform I/O, you need to create objects using appropriate Java I/O classes. The objects contain the methods for reading/writing data from/to a file. This section introduces how to read/write strings and numeric values from/to a text file using the Scanner and PrintWriter classes.
9.7.1Writing Data Using PrintWriter
The java.io.PrintWriter class can be used to create a file and write data to a text file. First, you have to create a PrintWriter object for a text file as follows:
PrintWriter output = new PrintWriter(filename);
Then, you can invoke the print, println, and printf methods on the PrintWriter object to write data to a file. Figure 9.17 summarizes frequently used methods in PrintWriter.
java.io.PrintWriter |
|
|
|
|
|
+PrintWriter(file: File) |
|
Creates a PrintWriter object for the specified file object. |
+PrintWriter(filename: String) |
|
Creates a PrintWriter object for the specified file-name string. |
+print(s: String): void |
|
Writes a string to the file. |
+print(c: char): void |
|
Writes a character to the file. |
+print(cArray: char[]): void |
|
Writes an array of characters to the file. |
+print(i: int): void |
|
Writes an int value to the file. |
+print(l: long): void |
|
Writes a long value to the file. |
+print(f: float): void |
|
Writes a float value to the file. |
+print(d: double): void |
|
Writes a double value to the file. |
+print(b: boolean): void |
|
Writes a boolean value to the file. |
Also contains the overloaded |
|
A println method acts like a print method; additionally it |
println methods. |
|
prints a line separator. The line-separator string is defined |
|
|
by the system. It is \r\n on Windows and \n on Unix. |
Also contains the overloaded |
|
The printf method was introduced in §3.17, “Formatting |
printf methods. |
|
Console Output.” |
|
|
|
FIGURE 9.17 The PrintWriter class contains the methods for writing data to a text file.
Listing 9.7 gives an example that creates an instance of PrintWriter and writes two lines to the file “scores.txt”. Each line consists of first name (a string), middle-name initial (a character), last name (a string), and score (an integer).
LISTING 9.7 WriteData.java
1 |
public class WriteData { |
|
||||||
2 |
public static void main(String[] args) |
throws Exception |
{ |
throws an exception |
||||
3 |
|
|
|
|
|
|
||
|
java.io.File file = new java.io.File("scores.txt"); |
create File object |
||||||
4 |
|
if |
(file.exists()) |
{ |
file exist? |
5System.out.println("File already exists");
6System.exit(0);
7 |
} |
8 |
|
9// Create a file
10 |
java.io.PrintWriter output = new java.io.PrintWriter(file); |
create PrintWriter |
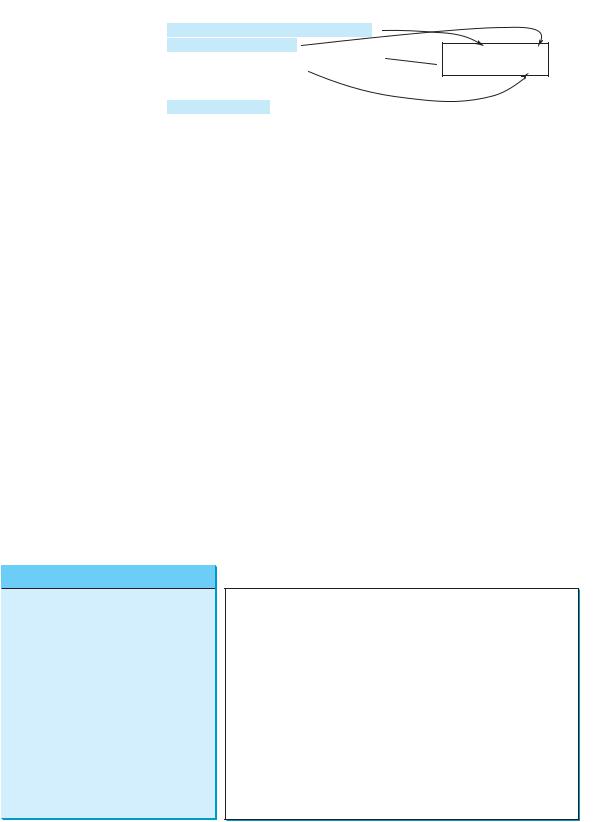
326 Chapter 9 Strings and Text I/O
print data
close file
create a file
throws Exception
print method close file
11
12// Write formatted output to the file
13output.print("John T Smith ");
14output.println(90);
15output.print("Eric K Jones ");
16output.println(85);
17
18// Close the file
19output.close();
20}
21}
John T Smith 90 scores.txt Eric K Jones 85
Lines 3–7 check whether the file scores.txt exists. If so, exit the program (line 6).
Invoking the constructor of PrintWriter will create a new file if the file does not exist. If the file already exists, the current content in the file will be discarded.
Invoking the constructor of PrintWriter may throw an I/O exception. Java forces you to write the code to deal with this type of exception. You will learn how to handle it in Chapter 13, “Exception Handling.” For now, simply declare throws Exception in the method header (line 2).
You have used the System.out.print and System.out.println methods to write text to the console. System.out is a standard Java object for the console. You can create objects for writing text to any file using print, println, and printf (lines 13–16).
The close() method must be used to close the file. If this method is not invoked, the data may not be saved properly in the file.
9.7.2Reading Data Using Scanner
The java.util.Scanner class was used to read strings and primitive values from the console in §2.3, “Reading Input from the Console.” A Scanner breaks its input into tokens delimited by whitespace characters. To read from the keyboard, you create a Scanner for System.in, as follows:
Scanner input = new Scanner(System.in);
To read from a file, create a Scanner for a file, as follows:
Scanner input = new Scanner(new File(filename));
Figure 9.18 summarizes frequently used methods in Scanner.
Creates a scanner that produces values scanned from the specified file.
Creates a scanner that produces values scanned from the specified string.
Closes this scanner.
Returns true if this scanner has more data to be read.
Returns next token as a string from this scanner.
Returns a line ending with the line separator from this scanner.
Returns next token as a byte from this scanner.
Returns next token as a short from this scanner.
Returns next token as an int from this scanner.
Returns next token as a long from this scanner.
Returns next token as a float from this scanner.
Returns next token as a double from this scanner.
Sets this scanner’s delimiting pattern and returns this scanner.
FIGURE 9.18 The Scanner class contains the methods for scanning data.
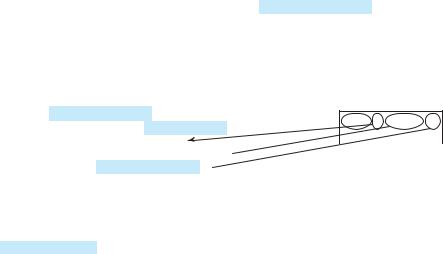
9.7 File Input and Output 327
Listing 9.8 gives an example that creates an instance of Scanner and reads data from the file “scores.txt”.
LISTING 9.8 ReadData.java
1 import java.util.Scanner;
2
3 public class ReadData {
4 public static void main(String[] args) throws Exception {
5// Create a File instance
6 |
java.io.File file = new java.io.File("scores.txt"); |
|||
7 |
|
|
|
|
8 |
// Create a Scanner |
for the file |
|
|
9 |
Scanner input = new |
Scanner(file); |
|
|
10 |
// Read data from a |
file |
|
|
11 |
scores.txt |
12while (input.hasNext()) {
13String firstName = input.next();John T Smith 90
14 |
String mi = input.next(); |
Eric K Jones 85 |
|
15String lastName = input.next();
16int score = input.nextInt();
17System.out.println(
18firstName + " " + mi + " " + lastName + " " + score);
19}
20
21// Close the file
22input.close();
23}
24}
Note that new Scanner(String) creates a Scanner for a given string. To create a Scanner to read data from a file, you have to use the java.io.File class to create an instance of the File using the constructor new File(filename) (line 6), and use new Scanner(File) to create a Scanner for the file (line 9).
Invoking the constructor new Scanner(File) may throw an I/O exception. So the main method declares throws Exception in line 4.
Each iteration in the while loop reads first name, mi, last name, and score from the text file (lines 12–19). The file is closed in line 22.
It is not necessary to close the input file (line 22), but it is a good practice to do so to release the resources occupied by the file.
9.7.3How Does Scanner Work?
The nextByte(), nextShort(), nextInt(), nextLong(), nextFloat(), nextDouble(), and next() methods are known as token-reading methods, because they read tokens separated by delimiters. By default, the delimiters are whitespace. You can use the useDelimiter-(String regex) method to set a new pattern for delimiters.
How does an input method work? A token-reading method first skips any delimiters (whitespace by default), then reads a token ending at a delimiter. The token is then automatically converted into a value of the byte, short, int, long, float, or double type for nextByte(), nextShort(), nextInt(), nextLong(), nextFloat(), and nextDouble(), respectively. For the next() method, no conversion is performed. If the token does not match the expected type, a runtime exception java.util.InputMismatchException will be thrown.
Both methods next() and nextLine() read a string. The next() method reads a string delimited by delimiters, but nextLine() reads a line ending with a line separator.
create a File
create a Scanner
has next? read items
close file
File class
throws Exception
close file
token-reading method
change delimiter
InputMismatchException
next() vs. nextLine()

328 Chapter 9 |
Strings and Text I/O |
|
Note |
line separator |
The line-separator string is defined by the system. It is \r\n on Windows and \n on Unix. To |
|
get the line separator on a particular platform, use |
|
String lineSeparator = System.getProperty("line.separator"); |
|
If you enter input from a keyboard, a line ends with the Enter key, which corresponds to the \n |
|
character. |
behavior of nextLine() |
The token-reading method does not read the delimiter after the token. If the nextLine() is |
|
invoked after a token-reading method, the method reads characters that start from this delim- |
|
iter and end with the line separator. The line separator is read, but it is not part of the string |
|
returned by nextLine(). |
input from file |
Suppose a text file named test.txt contains a line |
34 567
|
After the following code is executed, |
|
Scanner input = new Scanner(new File("test.txt")); |
|
int intValue = input.nextInt(); |
|
String line = input.nextLine(); |
|
intValue contains 34 and line contains characters ' ', '5', '6', '7'. |
input from keyboard |
What happens if the input is entered from the keyboard? Suppose you enter 34, the Enter |
|
key, 567, and the Enter key for the following code: |
|
Scanner input = new Scanner(System.in); |
|
int intValue = input.nextInt(); |
|
String line = input.nextLine(); |
|
You will get 34 in intValue and an empty string in line. Why? Here is the reason. The |
|
token-reading method nextInt() reads in 34 and stops at the delimiter, which in this case is |
|
a line separator (the Enter key). The nextLine() method ends after reading the line separa- |
|
tor and returns the string read before the line separator. Since there are no characters before |
|
the line separator, line is empty. |
9.7.4 Problem: Replacing Text
Suppose you are to write a program named ReplaceText that replaces all occurrences of a string in a text file with a new string. The file name and strings are passed as command-line arguments as follows:
java ReplaceText sourceFile targetFile oldString newString
For example, invoking
java ReplaceText FormatString.java t.txt StringBuilder StringBuffer
replaces all the occurrences of StringBuilder by StringBuffer in FormatString.java and saves the new file in t.txt.
Listing 9.9 gives the solution to the problem. The program checks the number of arguments passed to the main method (lines 7–11), checks whether the source and target files exist (lines 14–25), creates a Scanner for the source file (line 28), creates a PrintWriter for the target file, and repeatedly reads a line from the source file (line 32), replaces the text (line 33), and writes a new line to the target file (line 34). You must close the output file (line 38) to ensure that data are saved to the file properly.