
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

236 Chapter 7 Multidimensional Arrays
|
7.1 Introduction |
|
|
|
|
|
|
|
problem |
The preceding chapter introduced how to use one-dimensional arrays to store linear collec- |
|||||||
|
tions of elements. You can use a two-dimensional array to store a matrix or a table. For exam- |
|||||||
|
ple, the following table that describes the distances between the cities can be stored using a |
|||||||
|
two-dimensional array. |
|
|
|
|
|
|
|
|
|
|
|
Distance Table (in miles) |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Chicago |
Boston |
New York |
Atlanta |
Miami |
Dallas |
Houston |
|
|
|
|
|
|
|
|
|
|
Chicago |
0 |
983 |
787 |
714 |
1375 |
967 |
1087 |
|
Boston |
983 |
0 |
214 |
1102 |
1763 |
1723 |
1842 |
|
New York |
787 |
214 |
0 |
888 |
1549 |
1548 |
1627 |
|
Atlanta |
714 |
1102 |
888 |
0 |
661 |
781 |
810 |
|
Miami |
1375 |
1763 |
1549 |
661 |
0 |
1426 |
1187 |
|
Dallas |
967 |
1723 |
1548 |
781 |
1426 |
0 |
239 |
|
Houston |
1087 |
1842 |
1627 |
810 |
1187 |
239 |
0 |
7.2 Two-Dimensional Array Basics
How do you declare a variable for two-dimensional arrays? How do you create a twodimensional array? How do you access elements in a two-dimensional array? This section addresses these issues.
7.2.1Declaring Variables of Two-Dimensional Arrays and Creating
Two-Dimensional Arrays
Here is the syntax for declaring a two-dimensional array:
elementType[][] arrayRefVar;
or
elementType arrayRefVar[][]; // Allowed, but not preferred
As an example, here is how you would declare a two-dimensional array variable matrix of int values:
int[][] matrix;
or
int matrix[][]; // This style is allowed, but not preferred
You can create a two-dimensional array of 5-by-5 int values and assign it to matrix using this syntax:
matrix = new int[5][5];
Two subscripts are used in a two-dimensional array, one for the row and the other for the column. As in a one-dimensional array, the index for each subscript is of the int type and starts from 0, as shown in Figure 7.1(a).
To assign the value 7 to a specific element at row 2 and column 1, as shown in Figure 7.1(b), you can use the following:
matrix[2][1] = 7;

7.2 Two-Dimensional Array Basics 237
[0][1][2][3][4] |
|
[0][1][2][3][4] |
|
[0][1][2] |
||||||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
[0] |
|
|
|
|
|
[0] |
|
|
|
|
|
|
[0] |
|
1 |
|
2 |
3 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
[1] |
|
|
|
|
|
[1] |
|
|
|
|
|
|
[1] |
|
4 |
|
5 |
6 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
[2] |
|
|
|
|
|
[2] |
|
7 |
|
|
|
|
[2] |
|
7 |
|
8 |
9 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
[3] |
|
|
|
|
|
[3] |
|
|
|
|
|
|
[3] |
10 |
11 |
12 |
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|||||||||
[4] |
|
|
|
|
|
[4] |
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
int[][] array = { |
|||||||||
|
|
|
|
|
|
|
|
|
|
|
||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
{1, 2, |
3}, |
||||||
matrix = new int[5][5]; |
matrix[2][1] = 7; |
|||||||||||||||||||
{4, 5, 6}, |
||||||||||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
{7, 8, |
9}, |
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
{10, |
11, 12} |
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
}; |
|
|
|
|
|
|
|
|
|
(a) |
|
|
|
(b) |
|
|
|
(c) |
|
|
FIGURE 7.1 The index of each subscript of a two-dimensional array is an int value, starting from 0.
Caution
It is a common mistake to use matrix[2, 1] to access the element at row 2 and column 1. In
Java, each subscript must be enclosed in a pair of square brackets.
You can also use an array initializer to declare, create, and initialize a two-dimensional array. For example, the following code in (a) creates an array with the specified initial values, as shown in Figure 7.1(c). This is equivalent to the code in (b).
int[][] array = { |
|
|
|
int[][] array = new int[4][3]; |
{1, 2, 3}, |
|
Equivalent |
array[0][0] = 1; array[0][1] = 2; array[0][2] = 3; |
|
{4, 5, 6}, |
|
array[1][0] = 4; array[1][1] = 5; array[1][2] = 6; |
||
|
|
|
||
{7, 8, 9}, |
|
|
|
array[2][0] = 7; array[2][1] = 8; array[2][2] = 9; |
{10, 11, 12} |
|
|
|
array[3][0] = 10; array[3][1] = 11; array[3][2] = 12; |
}; |
|
|
|
|
|
|
|
|
|
(a) |
|
|
|
(b) |
7.2.2Obtaining the Lengths of Two-Dimensional Arrays
A two-dimensional array is actually an array in which each element is a one-dimensional array. The length of an array x is the number of elements in the array, which can be obtained using x.length. x[0], x[1], Á , and x[x.length-1] are arrays. Their lengths can be obtained using x[0].length, x[1].length, Á , and x[x.length-1].length.
For example, suppose x = new int[3][4], x[0], x[1], and x[2] are one-dimensional arrays and each contains four elements, as shown in Figure 7.2. x.length is 3, and x[0].length, x[1].length, and x[2].length are 4.
x |
|
x[0][0] |
x[0][1] |
x[0][2] |
x[0][3] |
x[0].length is 4 |
|
|
|
|
|||||
x[0] |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
x[1] |
|
|
|
|
|
|
x[1].length is 4 |
|
|
|
|||||
|
|
x[1][0] |
x[1][1] |
x[1][2] |
x[1][3] |
||
x[2] |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
x[2][0] |
x[2][1] |
x[2][2] |
x[2][3] |
x[2].length is 4 |
x.length is 3 |
|
||||||
|
|
|
|
|
|
||
|
|
|
|
|
|
FIGURE 7.2 A two-dimensional array is a one-dimensional array in which each element is another one-dimensional array.

238Chapter 7 Multidimensional Arrays
7.2.3Ragged Arrays
Each row in a two-dimensional array is itself an array. Thus the rows can have different lengths. An array of this kind is known as a ragged array. Here is an example of creating a ragged array:
int[][] triangleArray = { |
|
|
1 |
2 |
3 |
4 |
5 |
{1, 2, 3, 4, 5}, |
|
|
|
|
|
|
|
{2, 3, 4, 5}, |
|
|
|
|
|
|
|
|
|
2 |
3 |
4 |
5 |
|
|
{3, 4, 5}, |
|
|
|
||||
|
|
|
|
|
|
|
|
{4, 5}, |
|
|
|
|
|
|
|
{5} |
|
|
3 |
4 |
5 |
|
|
}; |
|
|
|
|
|
|
|
|
|
|
4 |
5 |
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
5 |
|
|
|
|
As can be seen, triangleArray[0].length is 5, triangleArray[1].length is 4, triangleArray[2].length is 3, triangleArray[3].length is 2, and triangleArray[4].length is 1.
If you don’t know the values in a ragged array in advance, but know the sizes, say the same as before, you can create a ragged array using the syntax that follows:
int[][] triangleArray = new int[5][] ; triangleArray[0] = new int[5]; triangleArray[1] = new int[4]; triangleArray[2] = new int[3]; triangleArray[3] = new int[2]; triangleArray[4] = new int[1];
You can now assign values to the array. For example,
triangleArray[0][3] = 50; triangleArray[4][0] = 45;
Note
The syntax new int[5][] for creating an array requires the first index to be specified. The syntax new int[][] would be wrong.
7.3 Processing Two-Dimensional Arrays
Suppose an array matrix is created as follows:
int[][] matrix = new int[10][10];
Here are some examples of processing two-dimensional arrays:
1.(Initializing arrays with input values) The following loop initializes the array with user input values:
java.util.Scanner input = new Scanner(System.in); System.out.println("Enter " + matrix.length + " rows and " +
matrix[0].length + " columns: ");
for (int row = 0; row < matrix.length ; row++) {
for (int column = 0; column < matrix[row].length ; column++) { matrix[row][column] = input.nextInt();
}
}
2.(Initializing arrays with random values) The following loop initializes the array with random values between 0 and 99:
for (int row = 0; row < matrix.length ; row++) {
for (int column = 0; column < matrix[row].length ; column++) {
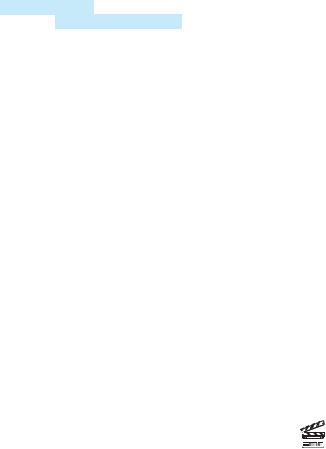
7.3 Processing Two-Dimensional Arrays 239
matrix[row][column] = (int)(Math.random() * 100);
}
}
3.(Printing arrays) To print a two-dimensional array, you have to print each element in the array using a loop like the following:
for (int row = 0; row < matrix.length ; row++) {
for (int column = 0; column < matrix[row].length ; column++) { System.out.print(matrix[row][column] + " ");
}
System.out.println();
}
4.(Summing all elements) Use a variable named total to store the sum. Initially total is 0. Add each element in the array to total using a loop like this:
int total = 0;
for (int row = 0; row < matrix.length; row++) {
for (int column = 0; column < matrix[row].length; column++) { total += matrix[row][column];
}
}
5.(Summing elements by column) For each column, use a variable named total to store its sum. Add each element in the column to total using a loop like this:
for (int column = 0; column < matrix[0].length; column++) { int total = 0;
for (int row = 0; row < matrix.length; row++) total += matrix[row][column];
System.out.println("Sum for column " + column + " is " + total);
}
6.(Which row has the largest sum?) Use variables maxRow and indexOfMaxRow to track the largest sum and index of the row. For each row, compute its sum and update maxRow and indexOfMaxRow if the new sum is greater.
int maxRow = 0;
int indexOfMaxRow = 0;
// Get sum of the first row in maxRow
for (int column = 0; column < matrix[0].length; column++) { maxRow += matrix[0][column];
}
for (int row = 1; row < matrix.length; row++) { int totalOfThisRow = 0;
for (int column = 0; column < matrix[row].length; column++) totalOfThisRow += matrix[row][column];
if (totalOfThisRow > maxRow) { maxRow = totalOfThisRow; indexOfMaxRow = row;
}
}
System.out.println("Row " + indexOfMaxRow + " has the maximum sum of " + maxRow);
Video Note
find the row with the largest sum