
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

618 Chapter 18 Applets and Multimedia
(a) |
(b) |
FIGURE 18.5 The appletviewer command runs a Java applet in the applet viewer utility.
signed applet
Video Note
Run applets standalone
18.4 Applet Security Restrictions
Java uses the so-called “sandbox security model” for executing applets to prevent destructive programs from damaging the system on which the browser is running. Applets are not allowed to use resources outside the “sandbox.” Specifically, the sandbox restricts the following activities:
■Applets are not allowed to read from, or write to, the file system of the computer. Otherwise, they could damage the files and spread viruses.
■Applets are not allowed to run programs on the browser’s computer. Otherwise, they might call destructive local programs and damage the local system on the user’s computer.
■Applets are not allowed to establish connections between the user’s computer and any other computer, except for the server where the applets are stored. This restriction prevents the applet from connecting the user’s computer to another computer without the user’s knowledge.
Note
You can create signed applets to circumvent the security restrictions. See java.sun.com/ developer/onlineTraining/Programming/JDCBook/signed.html for detailed instructions on how to create signed applets.
18.5 Enabling Applets to Run as Applications
Despite some differences, the JFrame class and the JApplet class have a lot in common. Since they both are subclasses of the Container class, all their user-interface components, layout managers, and event-handling features are the same. Applications, however, are invoked from the static main method by the Java interpreter, and applets are run by the Web browser. The Web browser creates an instance of the applet using the applet’s no-arg constructor and controls and executes the applet.
In general, an applet can be converted to an application without loss of functionality. An application can be converted to an applet as long as it does not violate the security restrictions imposed on applets. You can implement a main method in an applet to enable the applet to run as an application. This feature has both theoretical and practical implications. Theoretically, it blurs the difference between applets and applications. You can write a class that is both an applet and an application. From the standpoint of practicality, it is convenient to be able to run a program in two ways.
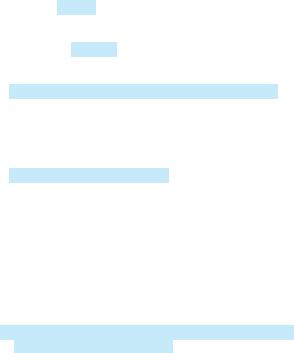
18.5 Enabling Applets to Run as Applications 619
How do you write such program? Suppose you have an applet named MyApplet. To enable it to run as an application, you need only add a main method in the applet with the implementation, as follows:
public static void main(String[] args) { // Create a frame
JFrame frame = new JFrame("Applet is in the frame");
// Create an instance of the applet MyApplet applet = new MyApplet();
// Add the applet to the frame frame.add(applet, BorderLayout.CENTER);
// Display the frame frame.setSize(300, 300);
frame.setLocationRelativeTo(null); // Center the frame frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true);
}
create frame
create applet
add applet
show frame
You can revise the DisplayLabel class in Listing 18.1 to enable it to run standalone by adding a main method in Listing 18.3.
LISTING 18.3 New DisplayLabel.java with a main Method
1 import javax.swing.*;
2
3 public class DisplayLabel extends JApplet {
4public DisplayLabel() {
5add(new JLabel("Great!", JLabel.CENTER));
6 |
} |
|
7 |
|
|
8 |
public static void main(String[] args) { |
new main method |
9// Create a frame
10 JFrame frame = new JFrame("Applet is in the frame"); 11
12// Create an instance of the applet
13DisplayLabel applet = new DisplayLabel();
15// Add the applet to the frame
16 |
frame.add(applet); |
17 |
|
18// Display the frame
19frame.setSize(300, 100);
20frame.setLocationRelativeTo(null); // Center the frame
21frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
22frame.setVisible(true);
23}
24}
When the applet is run from a Web browser, the browser creates an instance of the applet and displays it. When the applet is run standalone, the main method is invoked to create a frame (line 10) to hold the applet. The applet is created (line 13) and added to the frame (line 16). The frame is displayed in line 22.

620 Chapter 18 Applets and Multimedia
|
18.6 Applet Life-Cycle Methods |
applet container |
Applets are actually run from the applet container, which is a plug-in of a Web browser. The |
|
Applet class contains the init(), start(), stop(), and destroy() methods, known as |
|
the life-cycle methods. These methods are called by the applet container to control the execu- |
|
tion of an applet. They are implemented with an empty body in the Applet class. So, they do |
|
nothing by default. You may override them in a subclass of Applet to perform desired oper- |
|
ations. Figure 18.6 shows how the applet container calls these methods. |
|
Applet container |
Applet container |
Applet container |
|
Applet container |
|
|
|||||||||||
|
creates the applet |
invokes init() |
|
invokes start() |
|
invokes stop() |
|
|
||||||||||
Loaded |
|
|
Created |
|
|
Initialized |
|
|
Started |
|
|
|
|
Stopped |
||||
|
|
|
|
|
|
|
|
|
||||||||||
|
|
|
||||||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
Applet container |
|
Applet container |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
invokes start() |
|
invokes destroyed() |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
Applet container |
Destroyed |
|
loads the applet |
||
|
FIGURE 18.6 The applet container uses the init, start, stop, and destroy methods to control the applet.
|
18.6.1 |
The init Method |
init() |
The init method is invoked after the applet is created. If a subclass of Applet has an initia- |
|
|
lization to perform, it should override this method. The functions usually implemented in this |
|
|
method include getting string parameter values from the <applet> tag in the HTML page. |
|
|
18.6.2 |
The start Method |
start() |
The start method is invoked after the init method. It is also called when the user returns to |
|
|
the Web page containing the applet after surfing other pages. |
|
|
A subclass of Applet overrides this method if it has any operation that needs to be per- |
|
|
formed whenever the Web page containing the applet is visited. An applet with animation, for |
|
|
example, might start the timer to resume animation. |
|
|
18.6.3 |
The stop Method |
stop() |
The stop method is the opposite of the start method. The start method is called when the |
|
|
user moves back to the page that contains the applet. The stop method is invoked when the |
|
|
user leaves the page. |
|
|
A subclass of Applet overrides this method if it has any operation to be performed each |
|
|
time the Web page containing the applet is no longer visible. An applet with animation, for |
|
|
example, might stop the timer to pause animation. |
|
|
18.6.4 |
The destroy Method |
destroy() |
The destroy method is invoked when the browser exits normally to inform the applet that it |
|
|
is no longer needed and should release any resources it has allocated. The stop method is |
|
|
always called before the destroy method. |
|
|
A subclass of Applet overrides this method if it has any operation to be performed before |
|
|
it is destroyed. Usually, you won’t need to override this method unless you wish to release |
|
|
specific resources that the applet created. |
|
|
18.7 |
Passing Strings to Applets |
In §9.5, “Command-Line Arguments,” you learned how to pass strings to Java applications from a command line. Strings are passed to the main method as an array of strings. When the

18.7 Passing Strings to Applets 621
application starts, the main method can use these strings. There is no main method in an applet, however, and applets are not run from the command line by the Java interpreter.
How, then, can applets accept arguments? In this section, you will learn how to pass strings to Java applets. To be passed to an applet, a parameter must be declared in the HTML file and must be read by the applet when it is initialized. Parameters are declared using the <param> tag. The <param> tag must be embedded in the <applet> tag and has no end tag. Its syntax is given below:
<param name = parametername value = stringvalue />
This tag specifies a parameter and its corresponding string value.
Note
No comma separates the parameter name from the parameter value in the HTML code. The
HTML parameter names are not case sensitive.
Suppose you want to write an applet to display a message. The message is passed as a parameter. In addition, you want the message to be displayed at a specific location with x- coordinate and y-coordinate, which are passed as two parameters. The parameters and their values are listed in Table 18.1.
TABLE 18.1 Parameter Names and Values for the
DisplayMessage Applet
Parameter Name |
Parameter Value |
MESSAGE |
"Welcome to Java" |
X |
20 |
Y |
30 |
The HTML source file is given in Listing 18.4.
LISTING 18.4 DisplayMessage.html
<html>
<head>
<title>Passing Strings to Java Applets</title> </head>
<body>
<p>This applet gets a message from the HTML page and displays it.</p>
<applet
code = "DisplayMessage.class" width = 200
height = 50
alt = "You must have a Java 2-enabled browser to view the applet"
>
<param name = MESSAGE value = "Welcome to Java" /> <param name = X value = 20 />
<param name = Y value = 30 /> </applet>
</body>
</html>
To read the parameter from the applet, use the following method defined in the Applet class:
public String getParameter(String parametername);
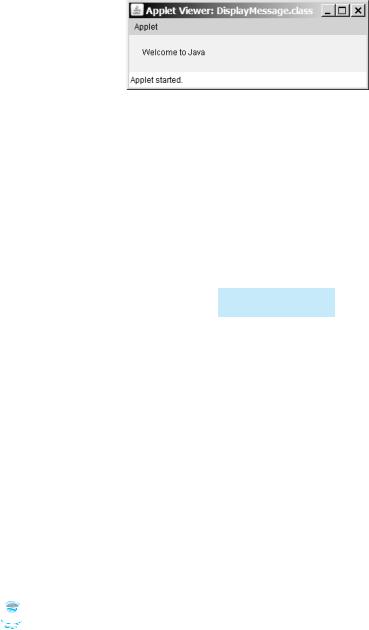
622 Chapter 18 Applets and Multimedia
This returns the value of the specified parameter.
The applet is given in Listing 18.5. A sample run of the applet is shown in Figure 18.7.
FIGURE 18.7 The applet displays the message Welcome to Java passed from the
HTML page.
LISTING 18.5 DisplayMessage.java
|
1 |
import javax.swing.*; |
|||||||
|
2 |
|
|
|
|
|
|
|
|
|
3 |
public class |
DisplayMessage extends JApplet |
{ |
|||||
|
4 |
|
/** Initialize the applet */ |
||||||
|
5 |
|
public void init() |
{ |
|
||||
|
6 |
|
// Get parameter values from the HTML file |
||||||
getParameter |
7 |
|
String message = |
getParameter("MESSAGE") |
; |
||||
|
8 |
|
int x = Integer.parseInt(getParameter("X") |
); |
|||||
|
9 |
|
int y = Integer.parseInt(getParameter("Y") |
); |
|||||
|
10 |
|
|
|
|
|
|
|
|
|
11 |
|
// Create a message panel |
||||||
|
12 |
|
MessagePanel messagePanel = new MessagePanel(message); |
||||||
|
13 |
|
messagePanel.setXCoordinate(x); |
||||||
|
14 |
|
messagePanel.setYCoordinate(y); |
||||||
|
15 |
|
|
|
|
|
|
|
|
|
16 |
|
// Add the message panel to the applet |
||||||
add to applet |
17 |
|
add(messagePanel); |
||||||
|
18 |
} |
|
|
|
|
|
|
|
|
19 |
} |
|
|
|
|
|
|
|
The program gets the parameter values from the HTML in the init method. The values are strings obtained using the getParameter method (lines 7–9). Because x and y are int, the program uses Integer.parseInt(string) to parse a digital string into an int value.
If you change Welcome to Java in the HTML file to Welcome to HTML, and reload the HTML file in the Web browser, you should see Welcome to HTML displayed. Similarly, the x and y values can be changed to display the message in a desired location.
Caution
The Applet’s getParameter method can be invoked only after an instance of the applet is created. Therefore, this method cannot be invoked in the constructor of the applet class. You should invoke it from the init method.
You can add a main method to enable this applet to run standalone. The applet takes the parameters from the HTML file when it runs as an applet and takes the parameters from the command line when it runs standalone. The program, as shown in Listing 18.6, is identical to DisplayMessage except for the addition of a new main method and of a variable named isStandalone to indicate whether it is running as an applet or as an application.

18.7 Passing Strings to Applets 623
LISTING 18.6 DisplayMessageApp.java
1 import javax.swing.*;
2 import java.awt.Font;
3 import java.awt.BorderLayout;
4
5 public class DisplayMessageApp extends JApplet {
6 private String message = "A default message"; // Message to display 7 private int x = 20; // Default x-coordinate
8 private int y = 20; // Default y-coordinate 9
10/** Determine whether it is an application */
11private boolean isStandalone = false;
12
13/** Initialize the applet */
14public void init() {
15if (!isStandalone) {
16// Get parameter values from the HTML file
17message = getParameter("MESSAGE");
18x = Integer.parseInt(getParameter("X"));
19y = Integer.parseInt(getParameter("Y"));
20}
21
22// Create a message panel
23MessagePanel messagePanel = new MessagePanel(message);
24messagePanel.setFont(new Font("SansSerif", Font.BOLD, 20));
25messagePanel.setXCoordinate(x);
26messagePanel.setYCoordinate(y);
27
28// Add the message panel to the applet
29add(messagePanel);
30}
31
32/** Main method to display a message
33@param args[0] x-coordinate
34@param args[1] y-coordinate
35@param args[2] message
36*/
37public static void main(String[] args) {
38// Create a frame
39JFrame frame = new JFrame("DisplayMessageApp");
41// Create an instance of the applet
42DisplayMessageApp applet = new DisplayMessageApp();
44// It runs as an application
45applet.isStandalone = true;
46
47// Get parameters from the command line
48applet.getCommandLineParameters(args);
49
50// Add the applet instance to the frame
51frame.add(applet, BorderLayout.CENTER);
52
53// Invoke applet's init method
54applet.init();
55applet.start();
56
57// Display the frame
58frame.setSize(300, 300);
isStandalone
applet params
standalone
command params