
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

78* of calling dispose on the window.
79*/
80public void windowClosed(WindowEvent event) {
81System.out.println("Window closed");
82}
83} );
84}
85}
16.9 Listener Interface Adapters 551
implement handler
The WindowEvent can be fired by the Window class or by any subclass of Window. Since JFrame is a subclass of Window, it can fire WindowEvent.
TestWindowEvent extends JFrame and implements WindowListener. The
WindowListener interface defines several abstract methods (windowActivated, windowClosed, windowClosing, windowDeactivated, windowDeiconified, windowIconified, windowOpened) for handling window events when the window is activated, closed, closing, deactivated, deiconified, iconified, or opened.
When a window event, such as activation, occurs, the windowActivated method is invoked. Implement the windowActivated method with a concrete response if you want the event to be processed.
16.9 Listener Interface Adapters
Because the methods in the WindowListener interface are abstract, you must implement all of them even if your program does not care about some of the events. For convenience, Java
provides support classes, called convenience adapters, which provide default implementations convenience adapter for all the methods in the listener interface. The default implementation is simply an empty
body. Java provides convenience listener adapters for every AWT listener interface with multiple handlers. A convenience listener adapter is named XAdapter for XListener. For example, WindowAdapter is a convenience listener adapter for WindowListener. Table 16.3 lists the convenience adapters.
TABLE 16.3 Convenience Adapters
Adapter |
Interface |
WindowAdapter WindowListener
MouseAdapter MouseListener
MouseMotionAdapter MouseMotionListener
KeyAdapter |
KeyListener |
ContainerAdapter ContainerListener
ComponentAdapter ComponentListener
FocusAdapter FocusListener
Using WindowAdapter, the preceding example can be simplified as shown in Listing 16.8, if you are interested only in the window-activated event. The WindowAdapter class is used to create an anonymous listener instead of WindowListener (line 15). The windowActivated handler is implemented in line 16.
LISTING 16.8 AdapterDemo.java
1 import java.awt.event.*;
2 import javax.swing.JFrame;
3
4 public class AdapterDemo extends JFrame {
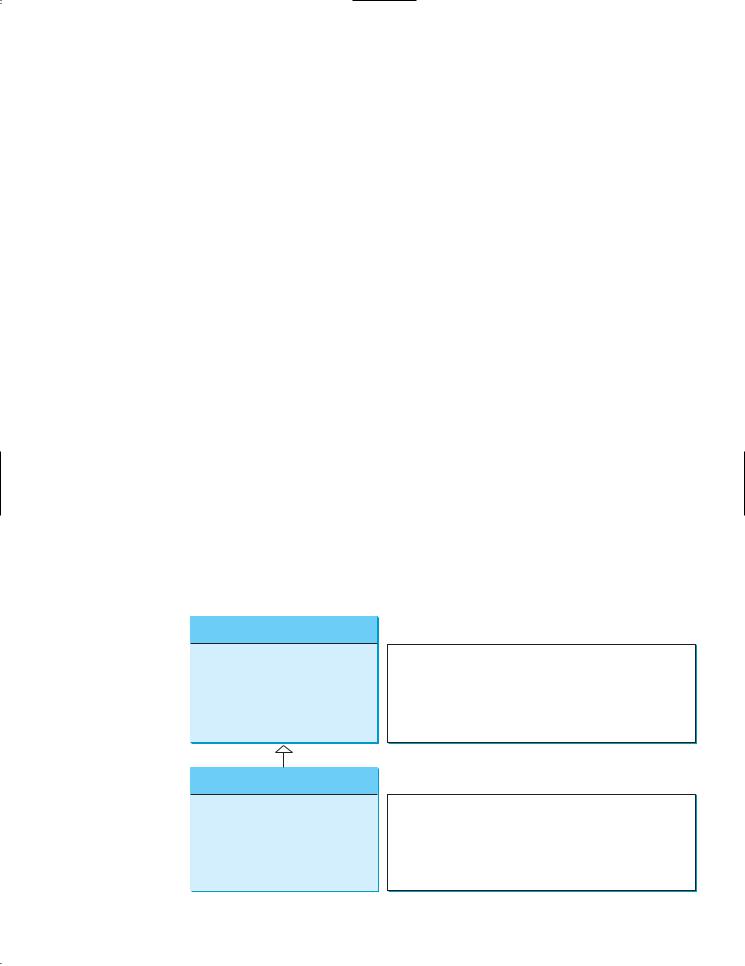
552 Chapter 16 |
Event-Driven Programming |
|||||
|
5 |
public static void main(String[] args) { |
||||
|
6 |
|
AdapterDemo frame = new AdapterDemo(); |
|||
|
7 |
|
frame.setSize(220, 80); |
|||
|
8 |
|
frame.setLocationRelativeTo(null); // Center the frame |
|||
|
9 |
|
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); |
|||
|
10 |
|
frame.setTitle("AdapterDemo"); |
|||
|
11 |
|
frame.setVisible(true); |
|||
|
12 |
} |
|
|
|
|
|
13 |
|
|
|
|
|
|
14 |
public AdapterDemo() { |
||||
register listener |
15 |
|
addWindowListener(new WindowAdapter() { |
|
||
implement handler |
16 |
|
|
public void windowActivated(WindowEvent event) { |
|
|
|
17 |
|
|
System.out.println("Window activated"); |
||
|
18 |
} |
|
|
||
|
19 |
}); |
|
|
||
|
20 |
} |
|
|
|
|
|
21 |
} |
|
|
|
|
16.10 Mouse Events
A mouse event is fired whenever a mouse is pressed, released, clicked, moved, or dragged on a component. The MouseEvent object captures the event, such as the number of clicks associated with it or the location (x- and y-coordinates) of the mouse, as shown in Figure 16.11.
Since the MouseEvent class inherits InputEvent, you can use the methods defined in the InputEvent class on a MouseEvent object.
Point class The java.awt.Point class represents a point on a component. The class contains two public variables, x and y, for coordinates. To create a Point, use the following constructor:
Point(int x, int y)
This constructs a Point object with the specified x- and y-coordinates. Normally, the data fields in a class should be private, but this class has two public data fields.
Java provides two listener interfaces, MouseListener and MouseMotionListener, to handle mouse events, as shown in Figure 16.12. Implement the MouseListener interface to
java.awt.event.InputEvent
+getWhen(): long
+isAltDown(): boolean
+isControlDown(): boolean
+isMetaDown(): boolean
+isShiftDown(): boolean
java.awt.event.MouseEvent
+getButton(): int
+getClickCount(): int
+getPoint(): java.awt.Point
+getX(): int
+getY(): int
FIGURE 16.11 The MouseEvent class encapsulates information for mouse events.

16.10 Mouse Events 553
«interface»
java.awt.event.MouseListener
+mousePressed(e: MouseEvent): void
+mouseReleased(e: MouseEvent): void
+mouseClicked(e: MouseEvent): void
+mouseEntered(e: MouseEvent): void
Invoked after the mouse button has been pressed on the source component.
Invoked after the mouse button has been released on the source component.
Invoked after the mouse button has been clicked (pressed and released) on the source component.
Invoked after the mouse enters the source component.
+mouseExited(e: MouseEvent): void |
Invoked after the mouse exits the source component. |
«interface»
java.awt.event.MouseMotionListener
+mouseDragged(e: MouseEvent): void Invoked after a mouse button is moved with a button pressed.
+mouseMoved(e: MouseEvent): void |
Invoked after a mouse button is moved without a button pressed. |
FIGURE 16.12 The MouseListener interface handles mouse pressed, released, clicked, entered, and exited events. The MouseMotionListener interface handles mouse dragged and moved events.
listen for such actions as pressing, releasing, entering, exiting, or clicking the mouse, and implement the MouseMotionListener interface to listen for such actions as dragging or moving the mouse.
16.10.1Example: Moving a Message on a Panel Using a Mouse
This example writes a program that displays a message in a panel, as shown in Listing 16.9. You can use the mouse to move the message. The message moves as the mouse drags and is always displayed at the mouse point. A sample run of the program is shown in Figure 16.13.
FIGURE 16.13 You can move the message by dragging the mouse.
LISTING 16.9 MoveMessageDemo.java
1 import java.awt.*;
2 import java.awt.event.*;
3 import javax.swing.*;
4
5 public class MoveMessageDemo extends JFrame {
6public MoveMessageDemo() {
7 // Create a MovableMessagePanel instance for moving a message 8 MovableMessagePanel p = new MovableMessagePanel
9 ("Welcome to Java");
10
11// Place the message panel in the frame
12setLayout(new BorderLayout());
13add(p);
14}
15
Video Note
Move message using the mouse
create a panel
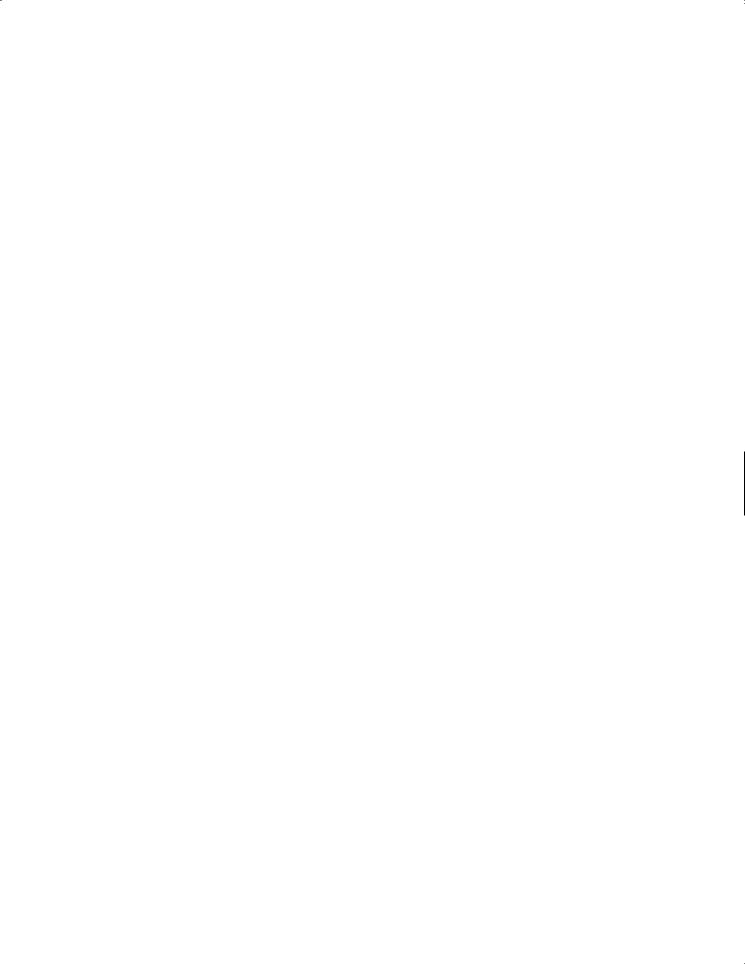
554 Chapter 16 |
Event-Driven Programming |
||||||||
|
16 |
|
/** Main method */ |
||||||
|
17 |
|
public static void main(String[] args) { |
||||||
|
18 |
|
MoveMessageDemo frame = new MoveMessageDemo(); |
||||||
|
19 |
|
frame.setTitle("MoveMessageDemo"); |
||||||
|
20 |
|
frame.setSize(200, 100); |
||||||
|
21 |
|
frame.setLocationRelativeTo(null); // Center the frame |
||||||
|
22 |
|
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); |
||||||
|
23 |
|
frame.setVisible(true); |
||||||
|
24 |
} |
|
|
|
|
|
|
|
|
25 |
|
|
|
|
|
|
|
|
|
26 |
// Inner class: MovableMessagePanel draws a message |
|||||||
inner class |
27 |
|
static class MovableMessagePanel extends JPanel { |
|
|||||
|
28 |
|
private String message = "Welcome to Java"; |
||||||
|
29 |
|
private int x = 20; |
||||||
|
30 |
|
private int y = 20; |
||||||
|
31 |
|
|
|
|
|
|
|
|
|
32 |
|
/** Construct a panel to draw string s */ |
||||||
|
33 |
|
public MovableMessagePanel(String s) { |
||||||
set a new message |
34 |
|
message = s; |
||||||
anonymous listener |
35 |
|
|
addMouseMotionListener(new MouseMotionAdapter() { |
|
||||
|
36 |
|
|
|
/** Handle mouse-dragged event */ |
||||
override handler |
37 |
|
|
|
|
public void mouseDragged(MouseEvent e) { |
|
|
|
|
38 |
|
|
|
|
// Get the new location and repaint the screen |
|||
new location |
39 |
|
|
|
|
x = e.getX(); |
|||
|
40 |
|
|
|
|
y = e.getY(); |
|||
repaint |
41 |
|
|
|
|
repaint(); |
|||
|
42 |
} |
|
|
|
||||
|
43 |
|
|
} |
); |
|
|
|
|
|
44 |
} |
|
|
|
|
|
|
|
|
45 |
|
|
|
|
|
|
|
|
|
46 |
|
/** Paint the component */ |
||||||
|
47 |
|
protected void paintComponent(Graphics g) { |
||||||
|
48 |
|
super.paintComponent(g); |
||||||
paint message |
49 |
|
g.drawString(message, x, y); |
||||||
|
50 |
} |
|
|
|
|
|
|
|
|
51 |
} |
|
|
|
|
|
|
|
|
52 |
} |
|
|
|
|
|
|
|
The MovableMessagePanel class extends JPanel to draw a message (line 27). Additionally, it handles redisplaying the message when the mouse is dragged. This class is defined as an inner class inside the main class because it is used only in this class. Furthermore, the inner class is defined static because it does not reference any instance members of the main class.
The MouseMotionListener interface contains two handlers, mouseMoved and mouseDragged, for handling mouse-motion events. When you move the mouse with the button pressed, the mouseDragged method is invoked to repaint the viewing area and display the message at the mouse point. When you move the mouse without pressing the button, the mouseMoved method is invoked.
Because the listener is interested only in the mouse-dragged event, the anonymous innerclass listener extends MouseMotionAdapter to override the mouseDragged method. If the inner class implemented the MouseMotionListener interface, you would have to implement all of the handlers, even if your listener did not care about some of the events.
The mouseDragged method is invoked when you move the mouse with a button pressed. This method obtains the mouse location using getX and getY methods (lines 39–40) in the MouseEvent class. This becomes the new location for the message. Invoking the repaint() method (line 41) causes paintComponent to be invoked (line 47), which displays the message in a new location.