
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

124 Chapter 4 Loops
Caution
Don’t use floating-point values for equality checking in a loop control. Since floating-point values numeric error are approximations for some values, using them could result in imprecise counter values and inac-
curate results.
Consider the following code for computing 1 + 0.9 + 0.8 + ... + 0.1:
double item = 1; double sum = 0;
while (item != 0) { // No guarantee item will be 0 sum += item;
item -= 0.1;
}
System.out.println(sum);
Variable item starts with 1 and is reduced by 0.1 every time the loop body is executed. The loop should terminate when item becomes 0. However, there is no guarantee that item will be exactly 0, because the floating-point arithmetic is approximated. This loop seems OK on the surface, but it is actually an infinite loop.
4.2.5Input and Output Redirections
In the preceding example, if you have a large number of data to enter, it would be cumbersome to type from the keyboard. You may store the data separated by whitespaces in a text file, say input.txt, and run the program using the following command:
java SentinelValue < input.txt
input redirection |
This command is called input redirection. The program takes the input from the file |
||||||
|
input.txt rather than having the user to type the data from the keyboard at runtime. Suppose |
||||||
|
the contents of the file are |
|
|
|
|
||
|
2 3 4 5 6 7 8 9 12 23 32 |
|
|
|
|||
|
23 |
45 67 |
89 92 12 |
34 35 |
3 |
1 |
2 4 0 |
|
The program should get sum to be 518. |
||||||
output redirection |
Similarly, there is output redirection, which sends the output to a file rather than displaying |
||||||
|
it on the console. The command for output redirection is: |
||||||
|
java ClassName > output.txt |
|
|||||
|
Input and output redirection can be used in the same command. For example, the following |
||||||
|
command gets input from input.txt and sends output to output.txt: |
||||||
|
java SentinelValue < input.txt > output.txt |
||||||
|
Please run the program and see what contents are in output.txt. |
|
4.3 The do-while Loop |
|
The do-while loop is a variation of the while loop. Its syntax is given below: |
do-while loop |
do { |
|
// Loop body; |
|
Statement(s); |
|
} while (loop-continuation-condition); |
Its execution flow chart is shown in Figure 4.2.
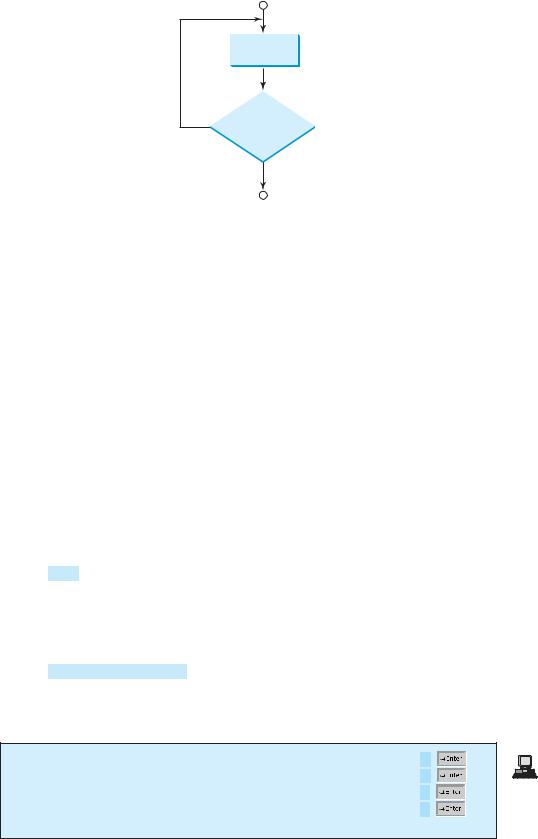
4.3 The do-while Loop 125
loop- true continuation
false
FIGURE 4.2 The do-while loop executes the loop body first, then checks the loop- continuation-condition to determine whether to continue or terminate the loop.
The loop body is executed first. Then the loop-continuation-condition is evaluated. If the evaluation is true, the loop body is executed again; if it is false, the do-while loop terminates. The difference between a while loop and a do-while loop is the order in which the loop-continuation-condition is evaluated and the loop body executed. The while loop and the do-while loop have equal expressive power. Sometimes one is a more convenient choice than the other. For example, you can rewrite the while loop in Listing 4.4 using a do-while loop, as shown in Listing 4.5:
LISTING 4.5 TestDoWhile.java
1 import java.util.Scanner;
2
3 public class TestDoWhile {
4/** Main method */
5 public static void main(String[] args) {
6int data;
7 int sum = 0;
8
9// Create a Scanner
10 Scanner input = new Scanner(System.in);
11
12// Keep reading data until the input is 0
13do {
14// Read the next data
15System.out.print(
16"Enter an int value (the program exits if the input is 0): ");
17data = input.nextInt();
18
19sum += data;
20} while (data != 0);
21
22System.out.println("The sum is " + sum);
23}
24}
Enter an int value (the program exits if the input is 0): 3
Enter an int value (the program exits if the input is 0): 5
Enter an int value (the program exits if the input is 0): 6
Enter an int value (the program exits if the input is 0): 0 The sum is 14
loop
end loop
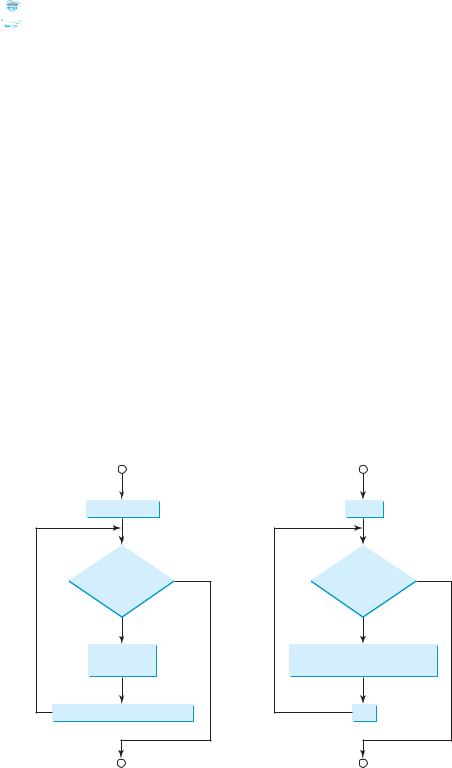
126 Chapter 4 Loops
Tip
Use the do-while loop if you have statements inside the loop that must be executed at least once, as in the case of the do-while loop in the preceding TestDoWhile program. These statements must appear before the loop as well as inside it if you use a while loop.
4.4 The for Loop
Often you write a loop in the following common form:
i = initialValue; // Initialize loop control variable while (i < endValue) {
// Loop body
...
i++; // Adjust loop control variable
}
A for loop can be used to simplify the proceding loop:
|
for (i = initialValue; i < endValue; i++) { |
|
// Loop body |
|
... |
|
} |
|
In general, the syntax of a for loop is as shown below: |
for loop |
for (initial-action; loop-continuation-condition; |
|
action-after-each-iteration) { |
|
// Loop body; |
|
Statement(s); |
|
} |
|
The flow chart of the for loop is shown in Figure 4.3(a). |
Initial-Action
loop- |
false |
|
continuation |
||
|
||
condition? |
|
|
true |
|
|
Statement(s) |
|
|
(loop body) |
|
|
action-after-each-iteration |
||
(a) |
|
i = 0 |
|
(i < 100)? |
false |
|
|
true |
|
System.out.println( |
|
"Welcome to Java"); |
|
i++ |
|
(b) |
|
FIGURE 4.3 A for loop performs an initial action once, then repeatedly executes the statements in the loop body, and performs an action after an iteration when the loop- continuation-condition evaluates to true.
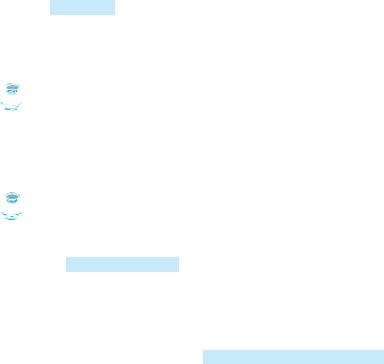
4.4 The for Loop 127
The for loop statement starts with the keyword for, followed by a pair of parentheses enclosing the control structure of the loop. This structure consists of initial-action, loop-continuation-condition, and action-after-each-iteration. The control structure is followed by the loop body enclosed inside braces. The initial-action, loop- continuation-condition, and action-after-each-iteration are separated by semicolons.
A for loop generally uses a variable to control how many times the loop body is executed
and when the loop terminates. This variable is referred to as a control variable. The initial- control variable action often initializes a control variable, the action-after-each-iteration usually
increments or decrements the control variable, and the loop-continuation-condition tests whether the control variable has reached a termination value. For example, the following for loop prints Welcome to Java! a hundred times:
int i;
for (i = 0; i < 100; i++) { System.out.println("Welcome to Java!");
}
The flow chart of the statement is shown in Figure 4.3(b). The for loop initializes i to 0, then repeatedly executes the println statement and evaluates i++ while i is less than 100.
The initial-action, i = 0, initializes the control variable, i. The loop- continuation-condition, i < 100, is a Boolean expression. The expression is evaluated right after the initialization and at the beginning of each iteration. If this condition is true, the loop body is executed. If it is false, the loop terminates and the program control turns to the line following the loop.
The action-after-each-iteration, i++, is a statement that adjusts the control variable. This statement is executed after each iteration. It increments the control variable. Eventually, the value of the control variable should force the loop-continuation-condition to become false. Otherwise the loop is infinite.
The loop control variable can be declared and initialized in the for loop. Here is an example:
for (int i = 0 ; i < 100; i++) { System.out.println("Welcome to Java!");
}
If there is only one statement in the loop body, as in this example, the braces can be omitted.
Tip
The control variable must be declared inside the control structure of the loop or before the loop. If the loop control variable is used only in the loop, and not elsewhere, it is good programming practice to declare it in the initial-action of the for loop. If the variable is declared inside the loop control structure, it cannot be referenced outside the loop. In the preceding code, for example, you cannot reference i outside the for loop, because it is declared inside the for loop.
initial-action
action-after-each- iteration
omitting braces
declare control variable
Note
The initial-action in a for loop can be a list of zero or more comma-separated variable |
for loop variations |
declaration statements or assignment expressions. For example, |
|
for (int i = 0, j = 0 ; (i + j < 10); i++, j++) { // Do something
}
The action-after-each-iteration in a for loop can be a list of zero or more commaseparated statements. For example,
for (int i = 1; i < 100; System.out.println(i), i++ );