
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
WRITE ERRORS TO THE APPLICATION LOG
hen working with exceptions, there are cases Wwhere you want to persist the error/exception
information to a durable store. You can persist errors by using the Event Log that is built into the Windows NT and 2000 operating systems. If you log error/exception information, you can analyze a reoccurring problem and understand the sequence of events that occur to cause the problem. Logging to the Event Log allows you to perform some troubleshooting without having to run the application in a debug mode.
To access the Event Log, you will have to use the System.Diagnostics namespace. With this referenced,
you can create an event log source which will give context to the entries that you write to the Event Log (source name for application and which log you want to write to – Application, Security, System, or a custom event log). With that Event Log object you will call the WriteEntry method to put entries into the event log. When writing errors to the log, you will want to classify the severity of the error. These severities will affect what icon and type classification the error is given in the event viewer.
The task below will take you through the basic steps of setting up and logging to an Event Log.
WRITE ERRORS TO THE APPLICATION LOG
|
|
|
|
|
|
|
|
class name |
Á Save the file. |
||
|
|
‡ Create string variables |
|
|
|
||
function. |
for the type of log, the source |
||
|
of the error, and the error |
||
|
message. |
° Add an if statement to check for the existence of the event log and set the
CreateEventSource property.
· Create a new EventLog variable and set the Source for the event log.

WORKING WITH ERRORS 15
You can control the severity for entries that you place in the Application Error Log. After running this sample, open the Event Viewer and note that each one has a different severity and each severity has a different icon.
Example:
string sLog = "Application";
string sSource = "MySharedPhotoAlbum";
string sErrorMsg1 = "Message for Information."; string sErrorMsg2 = "Message for Error."; string sErrorMsg3 = "Message for Warning.";
if ( !EventLog.SourceExists(sSource) ) { EventLog.CreateEventSource(sSource,sLog); }
EventLog elMain = new EventLog(); elMain.Source = sSource;
if ( elMain.Log.ToUpper() != sLog.ToUpper() ){ Console.WriteLine
("Source is not available to use!"); return;}
elMain.WriteEntry(sErrorMsg1,EventLogEntryType.Information);
elMain.WriteEntry(sErrorMsg2,EventLogEntryType.Error);
elMain.WriteEntry(sErrorMsg3,EventLogEntryType.Warning);
‚ Add an if statement to write a message if some other application is accessing
the log.
— Add the WriteEntry function to write the details of the log entry and write a message to the console about the update being successful.
± Set a debug stop.
¡ Press F5 to save, build, and run the console application.
■ A message appears about the event log being updated.
285

APPENDIX
BASIC EXAMPLES
DECLARING VARIABLES
|
|
|
|
|
|
Visual Basic |
C# |
JScript |
|
|
Dim x As Integer |
int x; |
var x : int; |
|
|
Dim x As Integer = 10 |
int x = 10; |
var x : int = 10; |
|
|
|
|
|
|
|
|
|
|
|
COMMENTS |
|
|
|
|
|
|
|
|
|
|
Visual Basic |
C# |
JScript |
|
|
‘ comment |
// comment |
// comment |
|
|
x = 1 ‘ comment |
/* multiline |
/* multiline |
|
|
Rem comment |
comment */ |
comment */ |
|
|
|
|
|
|
|
|
|
|
|
ASSIGNMENT STATEMENTS |
|
|
|
|
|
|
|
|
|
|
Visual Basic |
C# |
JScript |
|
|
nVal = 7 |
nVal = 7; |
nVal = 7; |
|
|
|
|
|
|
|
|
|
|
|
IF...ELSE STATEMENTS
Visual Basic
If nCnt <= nMax Then
nTotal += nCnt ‘ Same as nTotal = nTotal + nCnt nCnt += 1 ‘ Same as nCnt = nCnt + 1
Else
nTotal += nCnt nCnt -= 1
End If
C# |
JScript |
if (nCnt <= nMax) |
if(nCnt < nMax) { |
{ |
nTotal += nCnt; |
nTotal += nCnt; |
nCnt ++; |
nCnt++; |
} |
} |
else { |
else { |
nTotal += nCnt; |
nTotal += nCnt; |
nCnt --; |
nCnt --; |
}; |
} |
|
|
|
286

C# QUICK REFERENCE A
CASE STATEMENTS
Visual Basic
Select Case n
Case 0
MsgBox ("Zero")
‘Visual Basic exits the Select at the end of a Case.
Case 1
MsgBox ("One")
Case 2
MsgBox ("Two")
Case Else
MsgBox ("Default")
End Select
|
C# |
JScript |
|
|
switch(n) { |
switch(n) { |
|
|
case 0: |
case 0 : |
|
|
MessageBox.Show("Zero"); |
MessageBox.Show("Zero"); |
|
|
break; |
break; |
|
|
case 1: |
case 1 : |
|
|
MessageBox.Show("One"); |
MessageBox.Show("One"); |
|
|
break; |
break; |
|
|
case 2: |
case 2 : |
|
|
MessageBox.Show("Two"); |
MessageBox.Show("Two"); |
|
|
break; |
default : |
|
|
default: |
MessageBox.Show("Default"); |
|
|
MessageBox.Show("?"); |
} |
|
|
} |
|
|
|
|
|
|
FOR LOOPS |
|
|
|
|
|
|
|
|
Visual Basic |
JScript |
|
|
For n = 1 To 10 |
for (var n = 0; n < 10; n++) { |
|
|
MsgBox("The number is " & n) |
Response.Write("The number is " + n); |
|
|
Next |
} |
|
|
For Each i In iArray |
for (prop in obj){ |
|
|
Box.Show(i) |
obj[prop] = 42; |
|
|
Next i Msg |
} |
|
|
|
|
|
|
C# |
|
|
|
for (int i = 1; i <= 10; i++) |
|
|
|
MessageBox.Show("The number is {0}", i); |
|
|
|
foreach (int i in iArray) |
|
|
|
{ |
|
|
|
MessageBox.Show (i.ToString()); |
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
287
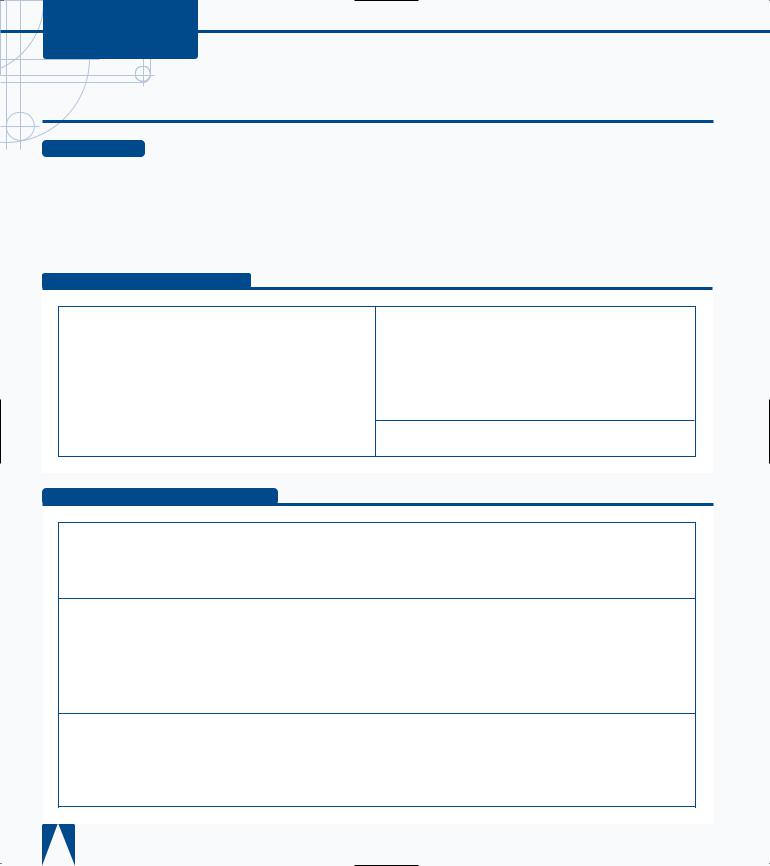
APPENDIX
BASIC EXAMPLES
WHILE LOOPS
|
|
|
|
|
|
|
Visual Basic |
|
C# |
JScript |
|
|
While n < 100 ‘ Test at start of loop |
while (n < 100) |
while (n < 100) { |
|
|
|
n += 1 |
‘ Same as n = n + 1 |
n++; |
n++; } |
|
|
End While ‘ |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
PARAMETER PASSING BY VALUE
Visual Basic
Public Sub ABC(ByVal y As Long) ‘ The argument Y is passed by value.
‘ If ABC changes y, the changes do not affect x. End Sub
ABC(x) ‘ Call the procedure
You can force parameters to be passed by value, regardless of how they are declared, by enclosing the parameters in extra parentheses.
ABC((x))
C#
//The method: void ABC(int x)
{
...
}
//Calling the method: ABC(i);
JScript
ABC(i,j);
PARAMETER PASSING BY REFERENCE
Visual Basic
Public Sub ABC(ByRef y As Long) ‘ The parameter of ABC is declared by reference: ‘ If ABC changes y, the changes are made to the value of x.
End Sub
ABC(x) ‘ Call the procedure
C#
//The method: void ABC(ref int x)
{
...
}
//Calling the method: ABC(ref i);
JScript
N/A (objects (including arrays) are passed by reference, but the object to which the variable refers to cannot be changed in the caller). Properties and methods changed in the callee are visible to the caller.
/* Reference parameters are supported for external object, but not internal JScript functions */
comPlusObject.SomeMethod(&foo);
288

C# QUICK REFERENCE A
STRUCTURED EXCEPTION HANDLING
Visual Basic |
JScript |
Try |
try { |
If x = 0 Then |
if (x == 0) { |
Throw New Exception("x equals zero") |
throw new Error(513, "x equals zero"); |
Else |
} |
Throw New Exception("x does not equal zero") |
else { |
End If |
throw new Error(514, "x does not equal zero"); |
Catch |
} |
MessageBox.Show("Error: " & Err.Description) |
} |
Finally |
catch(e) { |
MessageBox.Show("Executing finally block.") |
Response.Write("Error number: " + e.number + "<BR>"); |
End Try |
Response.Write("Error description: " + e.message + "<BR>"); |
|
} |
|
finally { |
|
Response.Write("Executing finally block."); |
|
} |
|
|
C# |
|
// try-catch-finally |
|
try |
|
{ |
|
if (x == 0)
throw new System.Exception ("x equals zero"); else
throw new System.Exception ("x does not equal zero");
}
catch (System.Exception err)
{
System.Console.WriteLine(err.Message);
}
finally
{
System.Console.WriteLine("executing finally block");
}
SET AN OBJECT REFERENCE TO NOTHING
Visual Basic |
C# |
JScript |
o = Nothing |
o = null; |
o = null; |
289