
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
HANDLING EXCEPTIONS WITH THE CATCH BLOCK
You can handle thrown exceptions with a catch block. You can insert a try/catch in all your procedures and just format a message to the user with the error that
occurred. Just formating the current exception into a message will keep your application from terminating, but it will create a frustrated user. To keep a content application user, you want to do more that just display the current error. At a minimum you should trap for common errors and display a custom message that your user can understand.
The granularity of the exception handling determines how polished your final application is and it has a large impact on the usability of the application. Errors happen
in your application, and the way they are handled is key to a good application.
To take exception handling further, you need to handle common exceptions that you know can occur. For example, the sample task below will take you through an example that is doing file access. One of the known issues with file access is attempting to access a file that does not exist. In the case of code that does file access, you want a catch block that explicitly handles the exception generated from a missing file. Inside of that catch block you write code that will collect the relative information about the failed attempt and then log that information and/or pass the information up the call stack while throwing an exception.
HANDLING EXCEPTIONS WITH THE CATCH BLOCK
Á Save the file.
‡ Create a string variable
. and initialize with a text file name.
° Create a string variable to hold a line of text.
· Add a try statement that attempts to open the file and outputs a status message to the console.
‚ Add a while loop to read through the file and output the contents of the file.
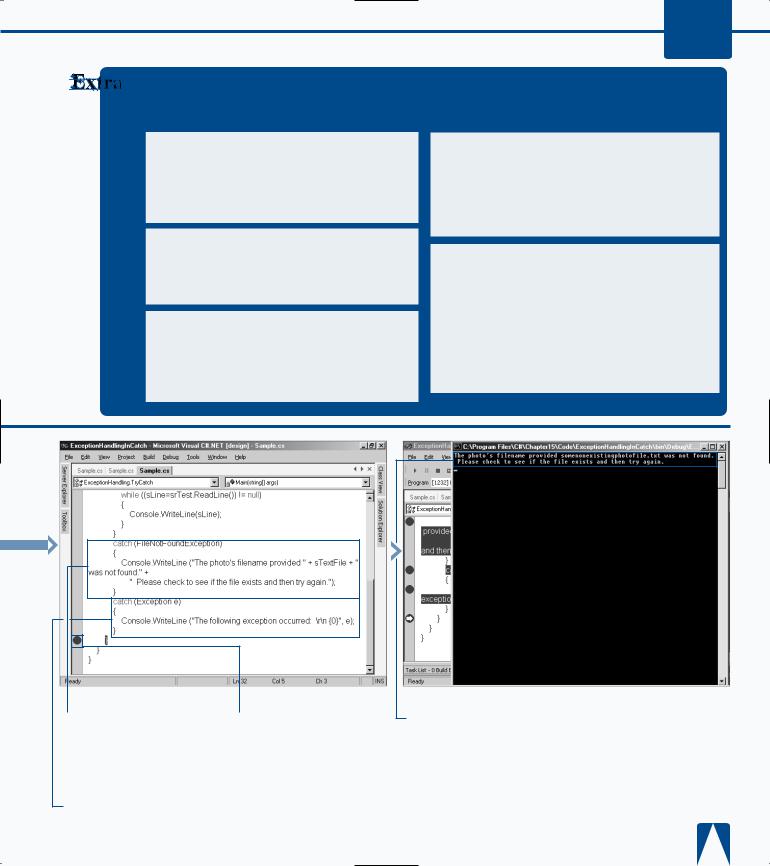
Catch blocks can be implemented several ways. Below are several sample catch blocks and a brief explanation of what each one does.
Example:
//Sample 1 – Handles all
//exception, execution continues catch
{
}
Example:
// Sample 2 – Essentially same as 1 catch (Exception e)
{
}
Example:
// Sample 3 - Rethrows exception e catch (Exception e)
{
throw (e);
}
WORKING WITH ERRORS 15
Example:
//Sample 4 – Handles only one
//specific error (all others
//will not be handled)
catch (StackOverflowException e)
{
}
Example:
//Sample 5 – Handles a specific
//error and all others go to the
//general catch statement
catch (StackOverflowException e)
{
}
catch (Exception e)
{
}
— Add a catch statement for the
FileNotFoundException and output an appropriate message if the exception was raised.
± Add a catch statement to output exceptions to the console.
¡ Add a debug stop.
™ Press F5 to save, build, and run the console application.
■ The FileNotFound Exception is raised and the message for this exception is displayed.
281

C#
USING THE FINALLY BLOCK
ou can run common code that needs to execute after Ya try/catch block by placing the code in an optional
finally block. The finally block is handy for running code that cleans up object reference and any other cleanup code that needs to run after the try and/or catch blocks. The cleanup code in the finally block can be closing a file or a connection to a database.
Finally, blocks will run no matter if an exception occurs or does not occur. You will want to place the finally block after the try and catch blocks. Note that the finally block will always execute, except for unhandled errors like
exceptions outside of the try/catch blocks or a run-time error inside the catch block.
There are cases where you might release or close resources in your try block. If this is the case, you need to validate that this has happened before closing out the resource again. Checking to see if a resource is close is necessary, because you can sometimes generate an exception if you reattempt to close a resource that is already close. To check to see if the resource is already released or not, you can check to see if the object is null ( if (object != null) { object.Close();} ).
USING THE FINALLY BLOCK
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Rename the namespace |
Á Save the file. |
||||
ExceptionHandling. |
|
‡ Create a string variable |
|||
|
|
||||
Rename the class name |
and initialize with a text file |
||||
FinallyBlock. |
name. |
||||
Add the Main function. |
|
° Create a string variable |
|||
|
|||||
|
to hold a line of text. |
· Add a try statement that attempts to open the file and outputs status messages to the console.
‚ Add a while loop to read through the file and output the contents of the file.

WORKING WITH ERRORS 15
Data access code will most likely always be in try/catch/finally blocks. If you compile this sample and run it twice, you will generate a primary key constraint error.
Example:
SqlConnection cnPubs = new SqlConnection(); SqlCommand cmdTitles = new SqlCommand(); try {
cnPubs.ConnectionString = "server=(local);uid=sa;pwd=;database=pubs";
cnPubs.Open(); String sInsertCmd =
"INSERT INTO titles(title_id, title) " + "VALUES(‘BU2222’,’Book Title’)";
cmdTitles.Connection = cnPubs; cmdTitles.CommandText = sInsertCmd; cmdTitles.ExecuteNonQuery(); }
catch (Exception e){ Console.WriteLine
("Exception occurred: \r\n {0}", e);} finally {
cmdTitles.Connection.Close(); Console.WriteLine("Cleanup Code Executed");
— Add a catch statement and output a message if the exception was raised.
± Add a finally statement to output messages to the console.
¡ Add a debug stop.
™ Press F5 to save, build, and run the console application.
■ The FileNotFound Exception is raised and the message for this exception is displayed, along with several status messages.
283